How to Get Accounts with the Zoho CRM API in Python
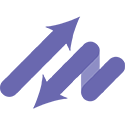
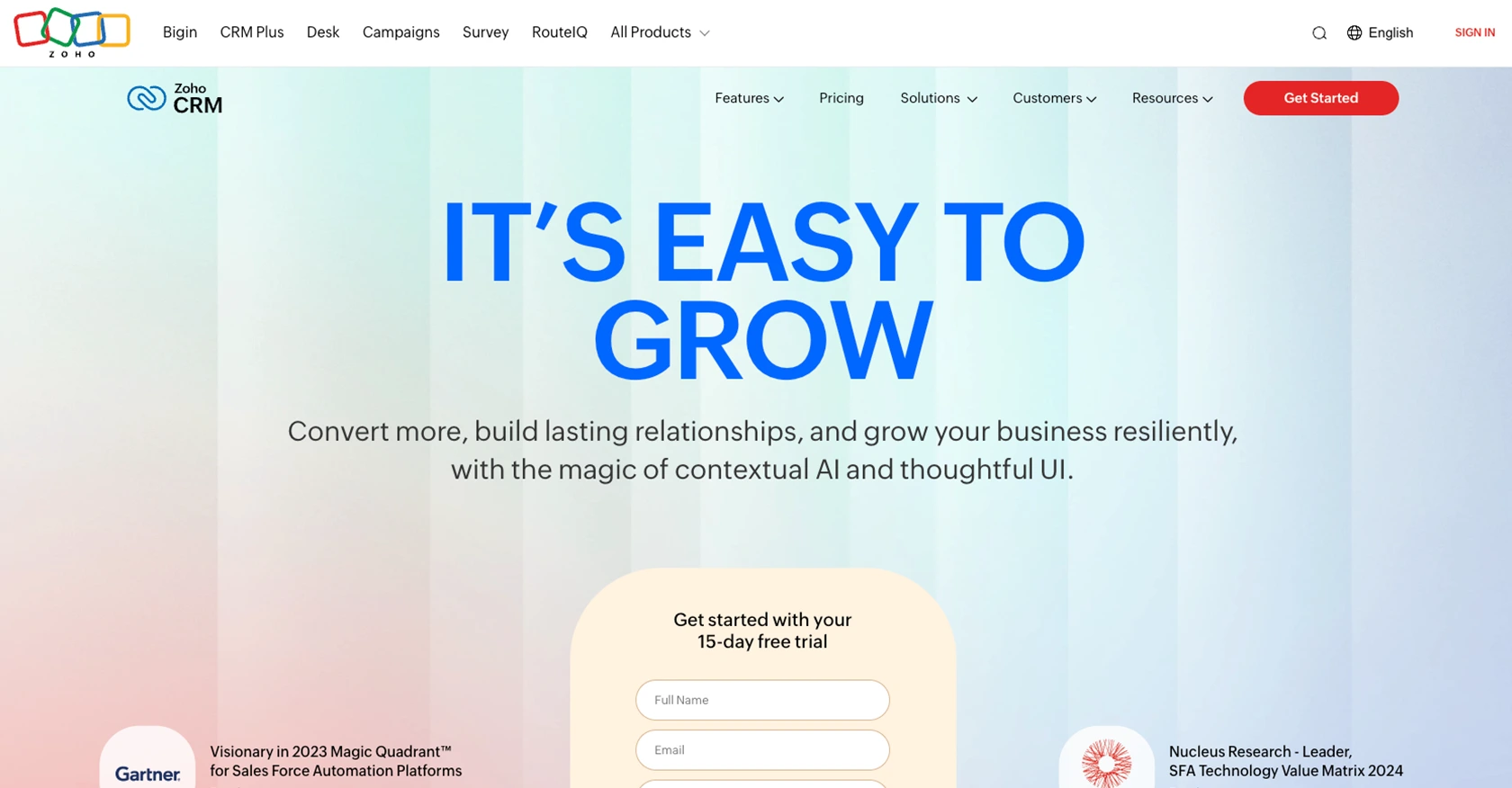
Introduction to Zoho CRM API Integration
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a unified manner. Known for its flexibility and extensive features, Zoho CRM is a popular choice for businesses looking to enhance their customer interactions and streamline operations.
Developers often integrate with Zoho CRM to access and manipulate customer data, such as accounts, to automate workflows and enhance business processes. For example, a developer might use the Zoho CRM API to retrieve account information and synchronize it with other business systems, ensuring data consistency across platforms.
This article will guide you through the process of using Python to interact with the Zoho CRM API, specifically focusing on retrieving account data. By following this tutorial, you'll learn how to efficiently access and manage account information within Zoho CRM using Python.
Setting Up Your Zoho CRM Test/Sandbox Account
Before diving into the integration process, it's essential to set up a Zoho CRM test or sandbox account. This allows you to safely experiment with the API without affecting live data. Zoho CRM offers a developer-friendly environment to test integrations and ensure everything works seamlessly.
Creating a Zoho CRM Developer Account
If you don't already have a Zoho CRM account, you can sign up for a free developer account. Follow these steps to get started:
- Visit the Zoho Developer Console.
- Click on "Sign Up For Free" and fill in the required details.
- Verify your email address to activate your account.
Registering Your Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication, which is a secure and industry-standard protocol. To interact with the API, you need to register your application and obtain the necessary credentials:
- Log in to the Zoho Developer Console.
- Select "Add Client" and choose the appropriate client type (e.g., Web-based, Mobile).
- Enter the following details:
- Client Name: A unique name for your application.
- Homepage URL: The URL of your application's homepage.
- Authorized Redirect URIs: The URL where Zoho will redirect after authentication.
- Click "Create" to generate your Client ID and Client Secret.
Generating Access and Refresh Tokens
Once your application is registered, you need to generate access and refresh tokens to authenticate API requests:
- Make an authorization request to Zoho's OAuth server using the client credentials.
- Upon successful authentication, you'll receive an authorization code.
- Exchange this code for access and refresh tokens by making a POST request to Zoho's token endpoint.
Refer to the detailed steps in the Zoho CRM OAuth Overview documentation.
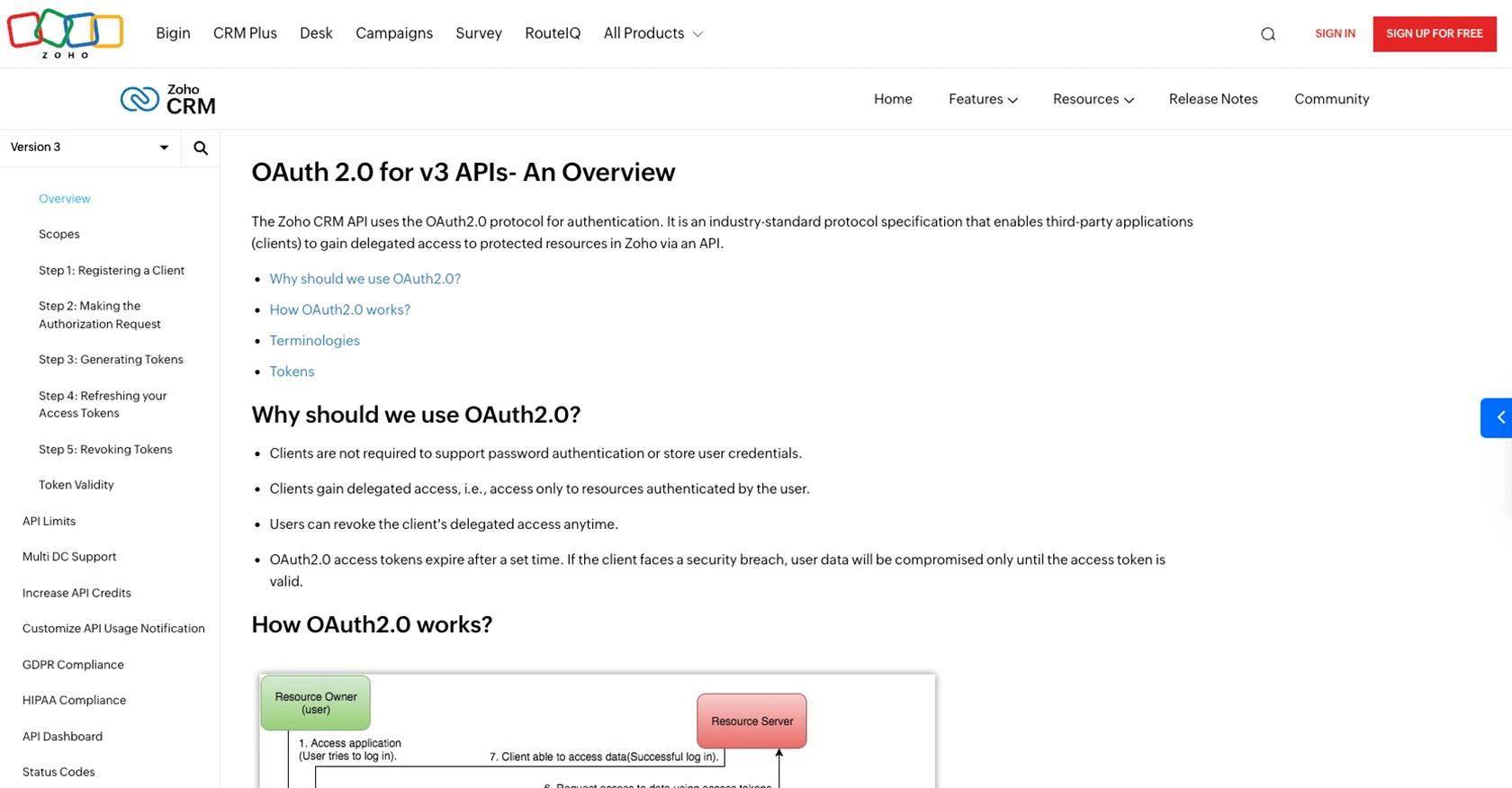
sbb-itb-96038d7
Making API Calls to Retrieve Accounts from Zoho CRM Using Python
To interact with the Zoho CRM API and retrieve account data, you'll need to use Python, a versatile and widely-used programming language. This section will guide you through the process of setting up your Python environment, installing necessary dependencies, and executing API calls to fetch account information from Zoho CRM.
Setting Up Your Python Environment for Zoho CRM API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests to the Zoho CRM API:
pip install requests
Executing the API Call to Fetch Account Data from Zoho CRM
With your environment set up, you can now proceed to make an API call to retrieve account data. Create a new Python file named get_zoho_accounts.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://www.zohoapis.com/crm/v3/Accounts"
headers = {
"Authorization": "Zoho-oauthtoken Your_Access_Token"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Parse the JSON data from the response
data = response.json()
# Loop through the accounts and print their information
for account in data.get("data", []):
print(account)
Replace Your_Access_Token
with the access token you obtained during the OAuth authentication process.
This script uses the requests
library to send a GET request to the Zoho CRM API endpoint for accounts. It then parses the JSON response and prints out the account details.
Running the Python Script and Verifying the API Response
To execute the script, run the following command in your terminal or command prompt:
python get_zoho_accounts.py
Upon successful execution, you should see the account data printed in your terminal. Verify the data by cross-referencing it with the accounts listed in your Zoho CRM sandbox account.
Handling Errors and Understanding Zoho CRM API Response Codes
While making API calls, it's crucial to handle potential errors. Zoho CRM API provides various status codes to indicate the success or failure of a request:
- 200 OK: The request was successful, and the response contains the requested data.
- 400 Bad Request: The request was malformed or contained invalid parameters.
- 401 Unauthorized: Authentication failed due to invalid or expired tokens.
- 403 Forbidden: The user does not have permission to access the requested resource.
- 404 Not Found: The requested resource could not be found.
For a comprehensive list of error codes and their meanings, refer to the Zoho CRM Status Codes documentation.
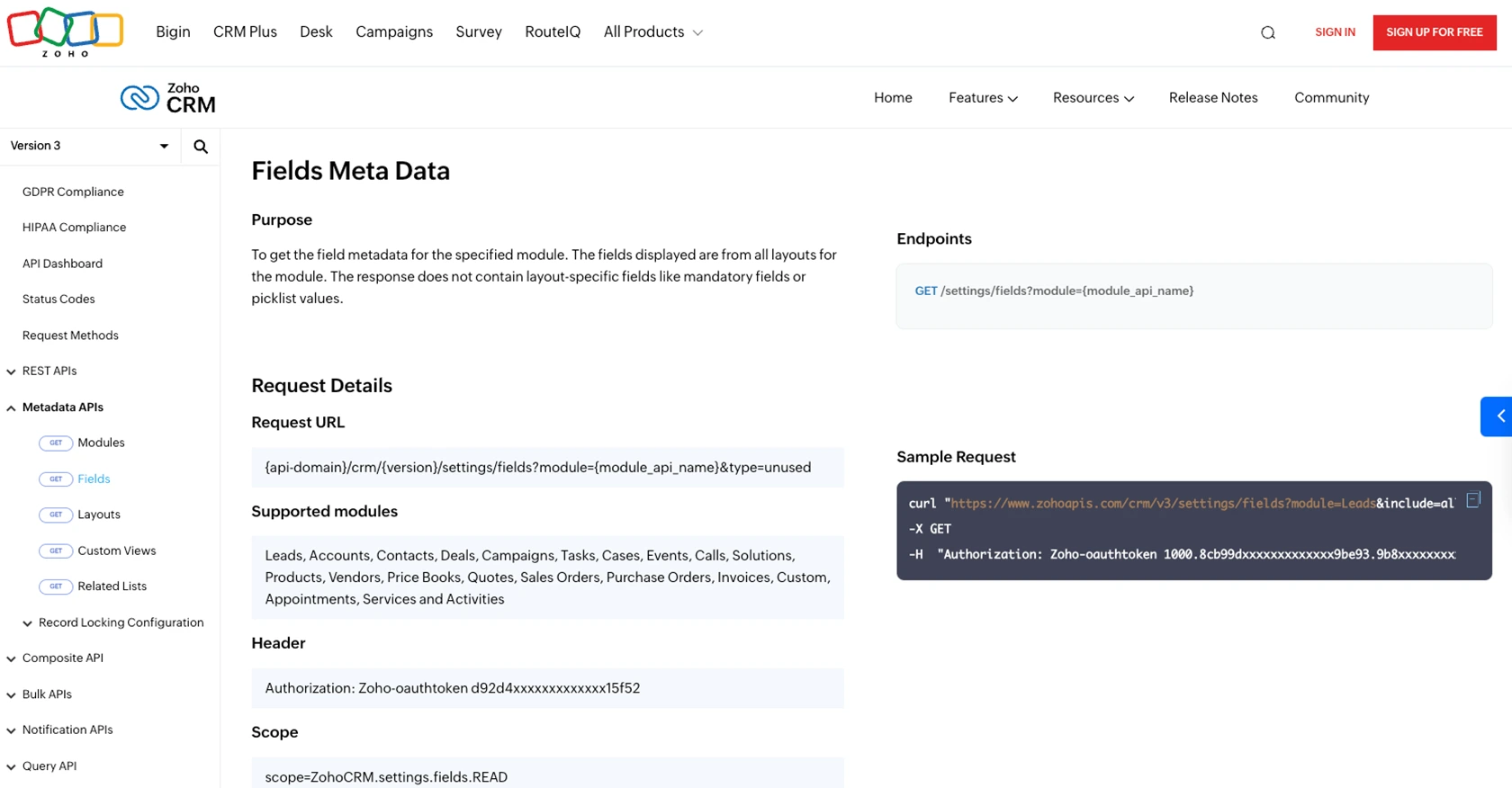
Conclusion and Best Practices for Zoho CRM API Integration
Integrating with the Zoho CRM API using Python provides a powerful way to automate and enhance your business processes. By following the steps outlined in this guide, you can efficiently retrieve account data and ensure seamless data synchronization across your systems.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Securely Store Credentials: Always store your OAuth tokens securely and avoid exposing them in public repositories or client-side code. Consider using environment variables or secure vaults for sensitive information.
- Handle Rate Limits: Zoho CRM imposes API rate limits to ensure fair usage. Monitor your API usage and implement retry mechanisms to handle rate limit errors gracefully. For more details, refer to the Zoho CRM API Limits documentation.
- Data Transformation and Standardization: When integrating data from multiple sources, ensure that data fields are transformed and standardized to maintain consistency and accuracy across systems.
- Error Handling: Implement robust error handling in your code to manage different API response codes effectively. This will help you identify and resolve issues quickly.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider leveraging Endgrate to simplify the process. Endgrate offers a unified API endpoint that connects to various platforms, including Zoho CRM, allowing you to focus on your core product while outsourcing integration complexities.
By using Endgrate, you can build once for each use case instead of multiple times for different integrations, saving time and resources. Explore the benefits of an intuitive integration experience for your customers by visiting Endgrate.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/get-records.html
Ready to get started?