How to Get Companies with the Teamwork CRM API in PHP
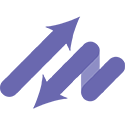
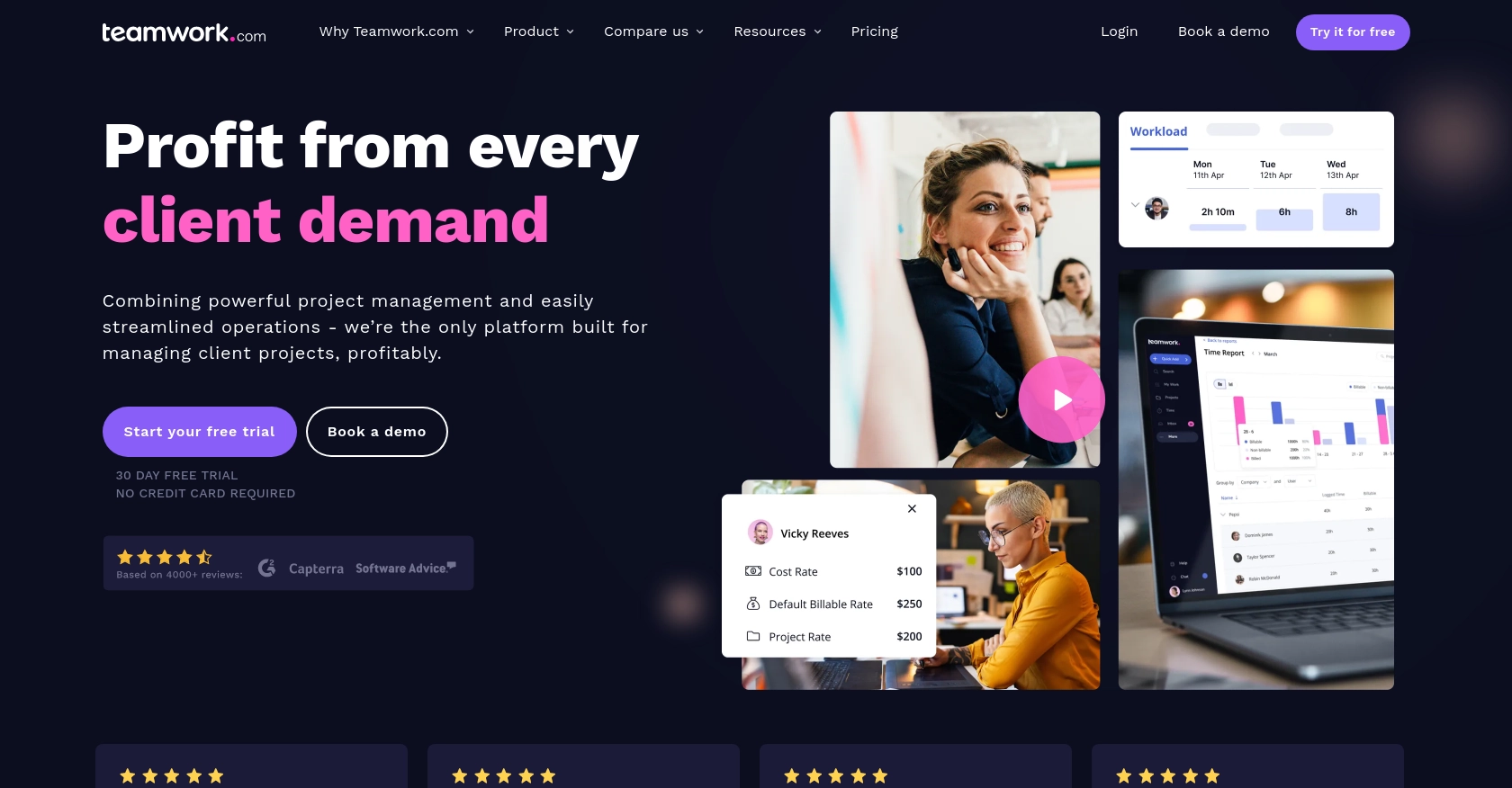
Introduction to Teamwork CRM API
Teamwork CRM is a powerful customer relationship management tool designed to help businesses manage their sales processes more effectively. With features that include deal tracking, contact management, and sales forecasting, Teamwork CRM provides a comprehensive solution for sales teams looking to streamline their workflows and improve productivity.
Integrating with the Teamwork CRM API allows developers to access and manipulate CRM data programmatically, enabling automation and customization of sales processes. For example, a developer might use the API to retrieve a list of companies from Teamwork CRM, which can then be used to generate detailed sales reports or synchronize data with other business applications.
Setting Up a Teamwork CRM Test Account
Before you can start interacting with the Teamwork CRM API, you'll need to set up a test account. This allows you to safely experiment with API calls without affecting live data. Teamwork offers a free trial that you can use to access their CRM features.
Follow these steps to create your test account:
- Visit the Teamwork CRM website and sign up for a free trial account.
- Complete the registration process by providing the necessary details such as your name, email address, and company information.
- Once registered, log in to your Teamwork CRM account to access the dashboard.
Creating a Teamwork CRM App for API Access
To interact with the Teamwork CRM API, you'll need to create an app in the Teamwork Developer Portal. This will provide you with the necessary credentials for authentication.
- Navigate to the Teamwork Developer Portal.
- Log in using your Teamwork CRM account credentials.
- Go to the "Create an App" section and fill in the required details such as the app name and description.
- Once your app is created, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for authenticating your API requests.
Authenticating with Teamwork CRM API
Teamwork CRM supports OAuth 2.0 for authentication. Follow these steps to authenticate your API requests:
- Use the client ID and client secret obtained from the Developer Portal to implement the OAuth 2.0 flow.
- Generate an access token by following the OAuth 2.0 process. This token will be used in the Authorization header of your API requests.
- Include the token in your API requests as follows:
// Example of setting the Authorization header in PHP
$headers = [
"Authorization: Bearer {your_access_token}"
];
For more details on authentication, refer to the Teamwork CRM Authentication Documentation.
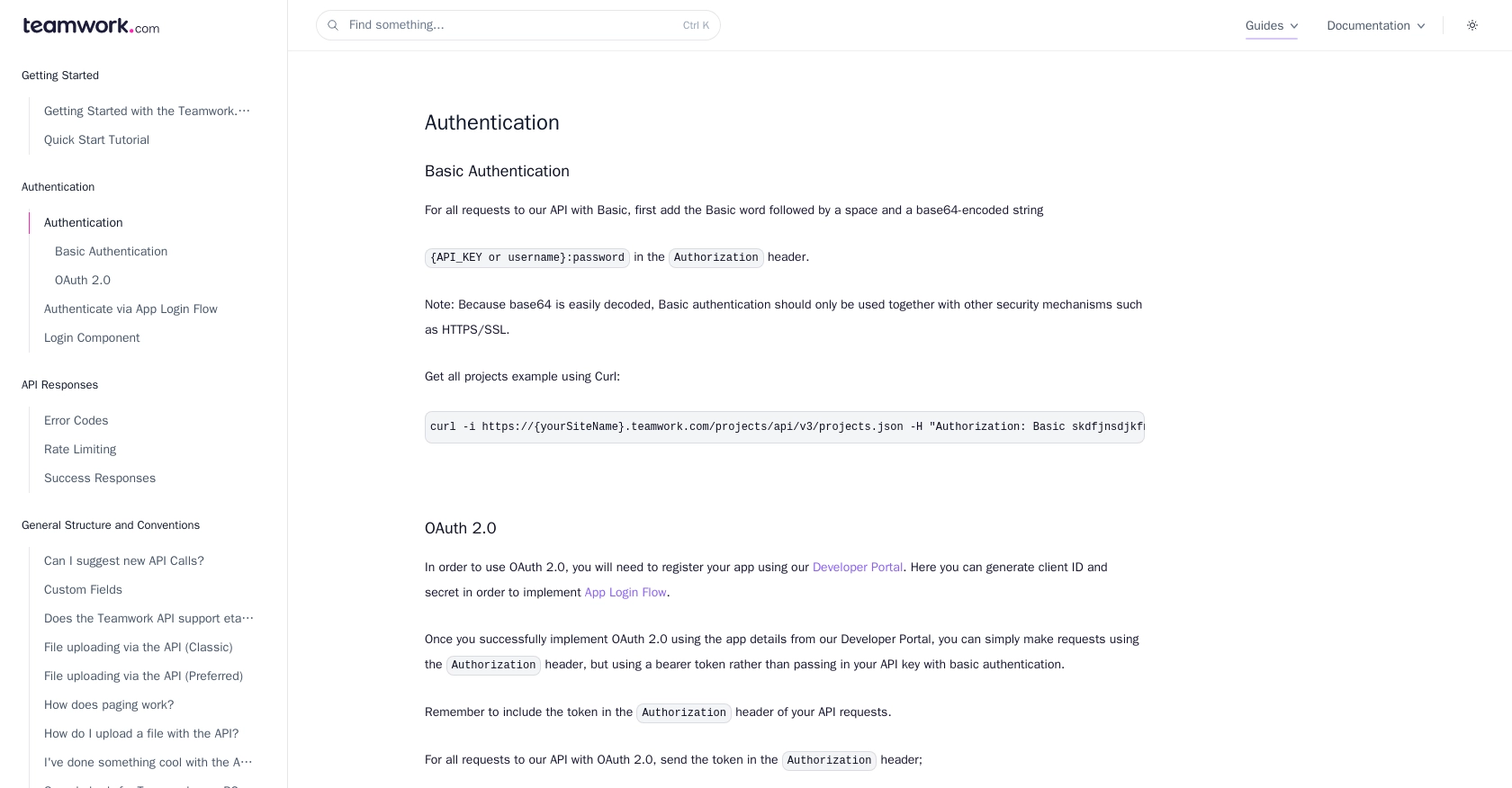
sbb-itb-96038d7
How to Make API Calls to Retrieve Companies from Teamwork CRM Using PHP
To interact with the Teamwork CRM API and retrieve a list of companies, you'll need to write a PHP script that makes an HTTP GET request to the appropriate endpoint. This section will guide you through the process, including setting up your PHP environment and handling API responses.
Setting Up Your PHP Environment for Teamwork CRM API Integration
Before making API calls, ensure that your PHP environment is correctly configured. You'll need PHP 7.4 or later and the cURL extension enabled to handle HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the cURL extension is enabled by checking your
php.ini
file or runningphp -m | grep curl
.
Installing Required PHP Dependencies
To simplify HTTP requests, you can use the Guzzle HTTP client. Install it using Composer:
composer require guzzlehttp/guzzle
Writing the PHP Script to Retrieve Companies from Teamwork CRM
Now, let's write a PHP script to make a GET request to the Teamwork CRM API and retrieve a list of companies.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'your_access_token'; // Replace with your actual access token
try {
$response = $client->request('GET', 'https://yourSiteName.teamwork.com/crm/v2/companies', [
'headers' => [
'Authorization' => "Bearer $accessToken",
'Accept' => 'application/json'
]
]);
$statusCode = $response->getStatusCode();
$body = $response->getBody();
$companies = json_decode($body, true);
if ($statusCode === 200) {
echo "Companies retrieved successfully:\n";
foreach ($companies['companies'] as $company) {
echo "Company Name: " . $company['name'] . "\n";
}
} else {
echo "Failed to retrieve companies. Status code: $statusCode\n";
}
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
Replace your_access_token
with the access token you obtained during the authentication process. This script uses Guzzle to send a GET request to the Teamwork CRM API endpoint for companies. It then decodes the JSON response and prints the company names.
Verifying the API Call Success in Teamwork CRM
After running the script, you should see a list of company names printed in your terminal. To verify the success of the API call, you can cross-check these companies in your Teamwork CRM dashboard.
Handling Errors and Understanding Teamwork CRM API Error Codes
It's crucial to handle potential errors when making API calls. The Teamwork CRM API may return various error codes, such as:
- 401 Unauthorized: Check your access token and ensure it's valid.
- 404 Not Found: Verify the API endpoint URL.
- 429 Rate Limit Exceeded: The API allows up to 150 requests per minute. If you exceed this, you'll receive a 429 error. Check the response headers for rate limit details.
For more information on error codes, refer to the Teamwork CRM Error Codes Documentation.
Conclusion and Best Practices for Using Teamwork CRM API in PHP
Integrating with the Teamwork CRM API using PHP can significantly enhance your ability to manage and automate sales processes. By following the steps outlined in this guide, you can efficiently retrieve company data and integrate it with other business applications.
Best Practices for Securely Storing Teamwork CRM API Credentials
When working with API credentials, it's crucial to store them securely to prevent unauthorized access. Consider the following best practices:
- Use environment variables to store sensitive information like access tokens and client secrets.
- Ensure your code repository does not contain hardcoded credentials.
- Regularly rotate your API keys and tokens to minimize security risks.
Handling Teamwork CRM API Rate Limiting
To avoid hitting the rate limit of 150 requests per minute, implement strategies such as:
- Batching requests where possible to reduce the number of API calls.
- Implementing exponential backoff for retrying requests after receiving a 429 error.
- Monitoring the
X-Rate-Limit-Remaining
header to adjust your request frequency dynamically.
For more details on rate limits, refer to the Teamwork CRM Rate Limiting Documentation.
Transforming and Standardizing Data from Teamwork CRM API
When integrating data from Teamwork CRM with other systems, ensure consistency by:
- Mapping data fields between Teamwork CRM and your application to maintain uniformity.
- Using data transformation tools or scripts to convert data formats as needed.
- Implementing validation checks to ensure data integrity during synchronization.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including Teamwork CRM, allowing you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate.
Read More
Ready to get started?