How to Get Customers with the Endear API in Python
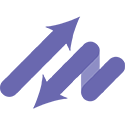
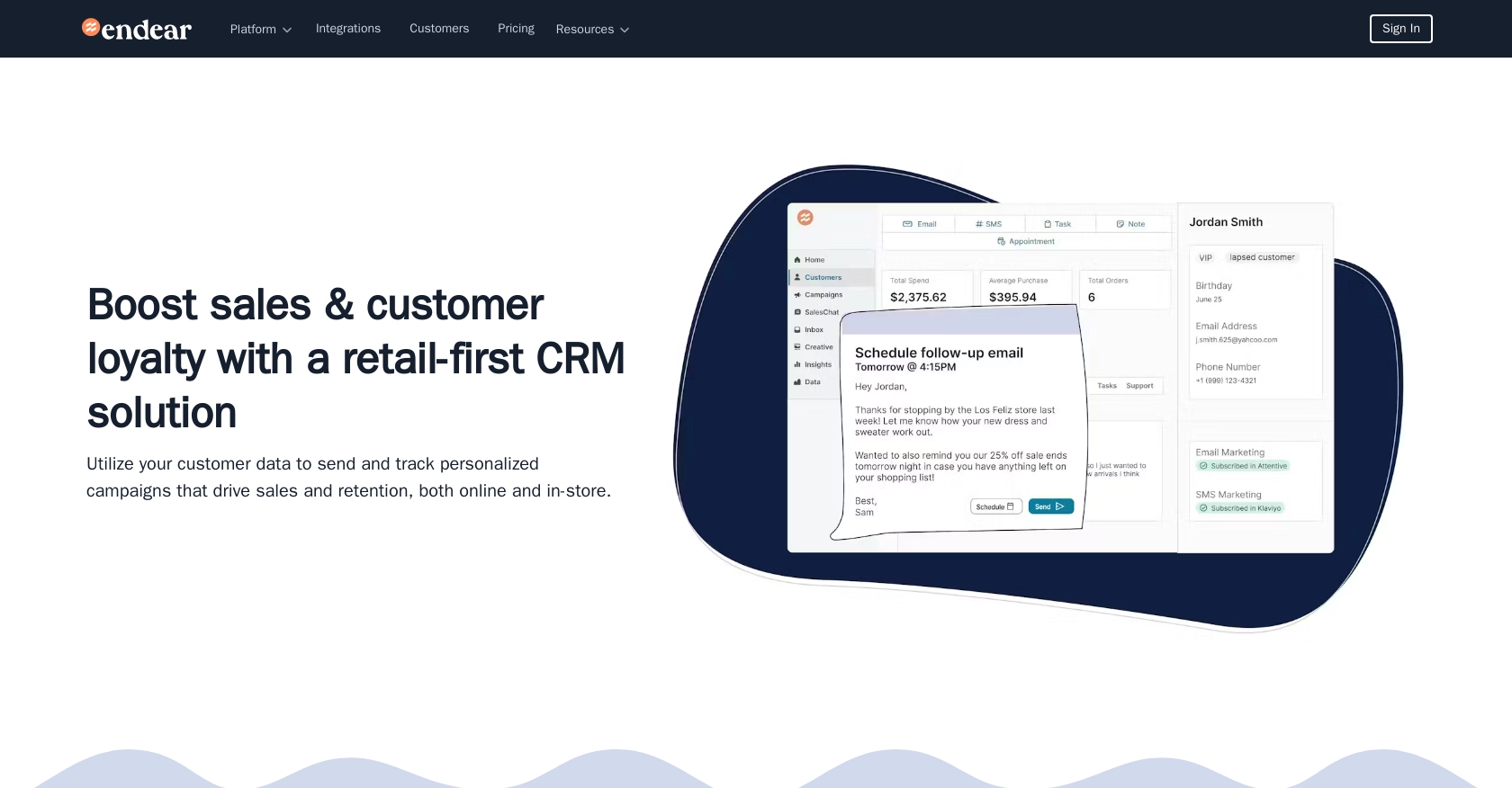
Introduction to Endear API Integration
Endear is a powerful CRM platform designed to enhance customer relationships by providing businesses with tools to manage and analyze customer data effectively. Its robust features allow businesses to personalize customer interactions and improve sales strategies.
Developers may want to integrate with Endear's API to access and manage customer data seamlessly. For example, a developer could use the Endear API to retrieve customer information and use it to tailor marketing campaigns or improve customer service interactions.
This article will guide you through using Python to interact with the Endear API, specifically focusing on retrieving customer data. By following these steps, you can efficiently access and manage customer information on the Endear platform using Python.
Setting Up Your Endear Test Account and API Key
Before you can start interacting with the Endear API, you'll need to set up a test account and generate an API key. This will allow you to authenticate your requests and access customer data securely.
Creating an Endear Account
If you don't already have an Endear account, you'll need to create one. Visit the Endear website and sign up for a free trial or demo account. Follow the on-screen instructions to complete the registration process. Once your account is set up, you can log in to access the Endear dashboard.
Generating an API Key for Endear Integration
To interact with the Endear API, you'll need to generate an API key. Follow these steps to create an API key:
- Log in to your Endear account and navigate to the settings page.
- Click on Integrations from the menu.
- Select Add Integration and choose API as the integration type.
- Fill in the required details and submit the form to generate your API key.
Once generated, your API key will be displayed. Make sure to copy and store it securely, as you'll need it to authenticate your API requests.
Authenticating API Requests with Your Endear API Key
With your API key ready, you can authenticate your requests to the Endear API. Here's how to include the API key in your requests:
import requests
# Set the API endpoint
url = "https://api.endearhq.com/graphql"
# Set the request headers with your API key
headers = {
"Content-Type": "application/json",
"X-Endear-Api-Key": "Your_API_Key"
}
# Example query to fetch customer data
query = """
query Edges {
searchCustomers {
edges {
node {
first_name
last_name
default_email_address
}
}
}
}
"""
# Send the request
response = requests.post(url, headers=headers, json={"query": query})
# Print the response
print(response.json())
Replace Your_API_Key
with the API key you generated earlier. This code snippet demonstrates how to set up the headers and send a POST request to the Endear API to retrieve customer data.
By following these steps, you can successfully set up your Endear test account and authenticate your API requests, allowing you to access and manage customer data efficiently.
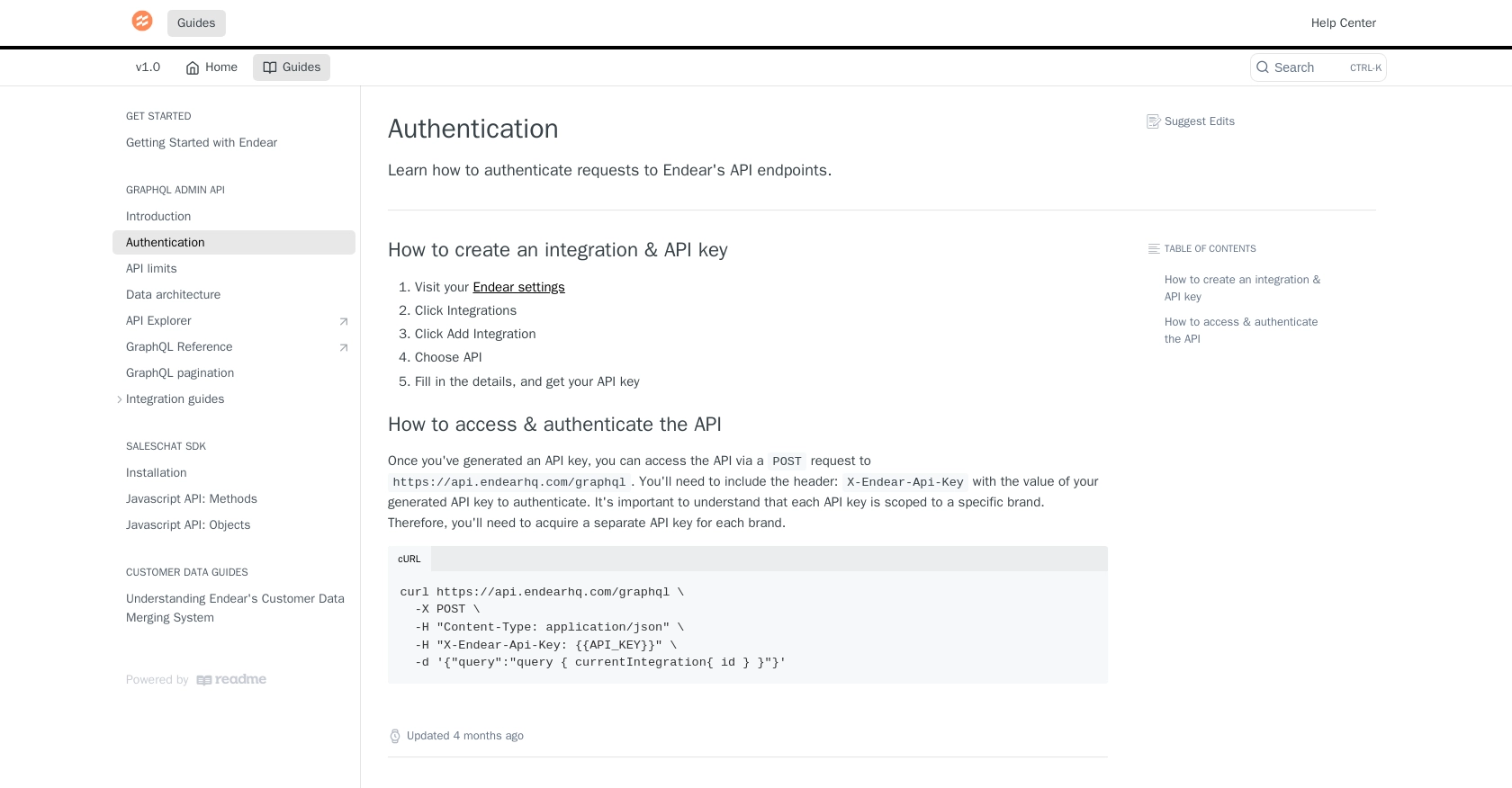
sbb-itb-96038d7
Making API Calls to Retrieve Customer Data from Endear Using Python
Now that you have set up your Endear account and generated an API key, it's time to make API calls to retrieve customer data. This section will guide you through the process of using Python to interact with the Endear API effectively.
Setting Up Your Python Environment for Endear API Integration
Before making API calls, ensure you have the necessary Python environment set up. You'll need Python 3.x and the requests
library to handle HTTP requests. If you haven't installed the requests
library, you can do so using the following command:
pip install requests
Writing Python Code to Fetch Customer Data from Endear
With your environment ready, you can now write the Python code to interact with the Endear API. Below is a sample code snippet to help you get started:
import requests
# Define the API endpoint
url = "https://api.endearhq.com/graphql"
# Set the request headers with your API key
headers = {
"Content-Type": "application/json",
"X-Endear-Api-Key": "Your_API_Key"
}
# Define the GraphQL query to fetch customer data
query = """
query Edges {
searchCustomers {
edges {
node {
first_name
last_name
default_email_address
default_phone_number
default_city
}
}
}
}
"""
# Send the POST request to the Endear API
response = requests.post(url, headers=headers, json={"query": query})
# Check if the request was successful
if response.status_code == 200:
# Parse and print the JSON response
data = response.json()
for customer in data['data']['searchCustomers']['edges']:
print(customer['node'])
else:
print(f"Error: {response.status_code} - {response.text}")
Replace Your_API_Key
with the API key you generated earlier. This code sends a POST request to the Endear API, retrieves customer data, and prints it to the console.
Verifying API Call Success and Handling Errors
To ensure your API call was successful, check the response status code. A status code of 200 indicates success, while other codes may indicate errors. Refer to the Endear documentation for more information on error codes and handling.
In case of a rate limit error (HTTP 429), remember that Endear's API allows up to 120 requests per minute. Implementing a retry mechanism or exponential backoff strategy can help manage rate limits effectively.
Testing and Validating Your API Calls in the Endear Sandbox
After running your code, verify the retrieved data by cross-referencing it with the customer records in your Endear sandbox account. This ensures that the API call is fetching the correct data.
By following these steps, you can efficiently retrieve and manage customer data from Endear using Python, enhancing your application's capabilities and user experience.
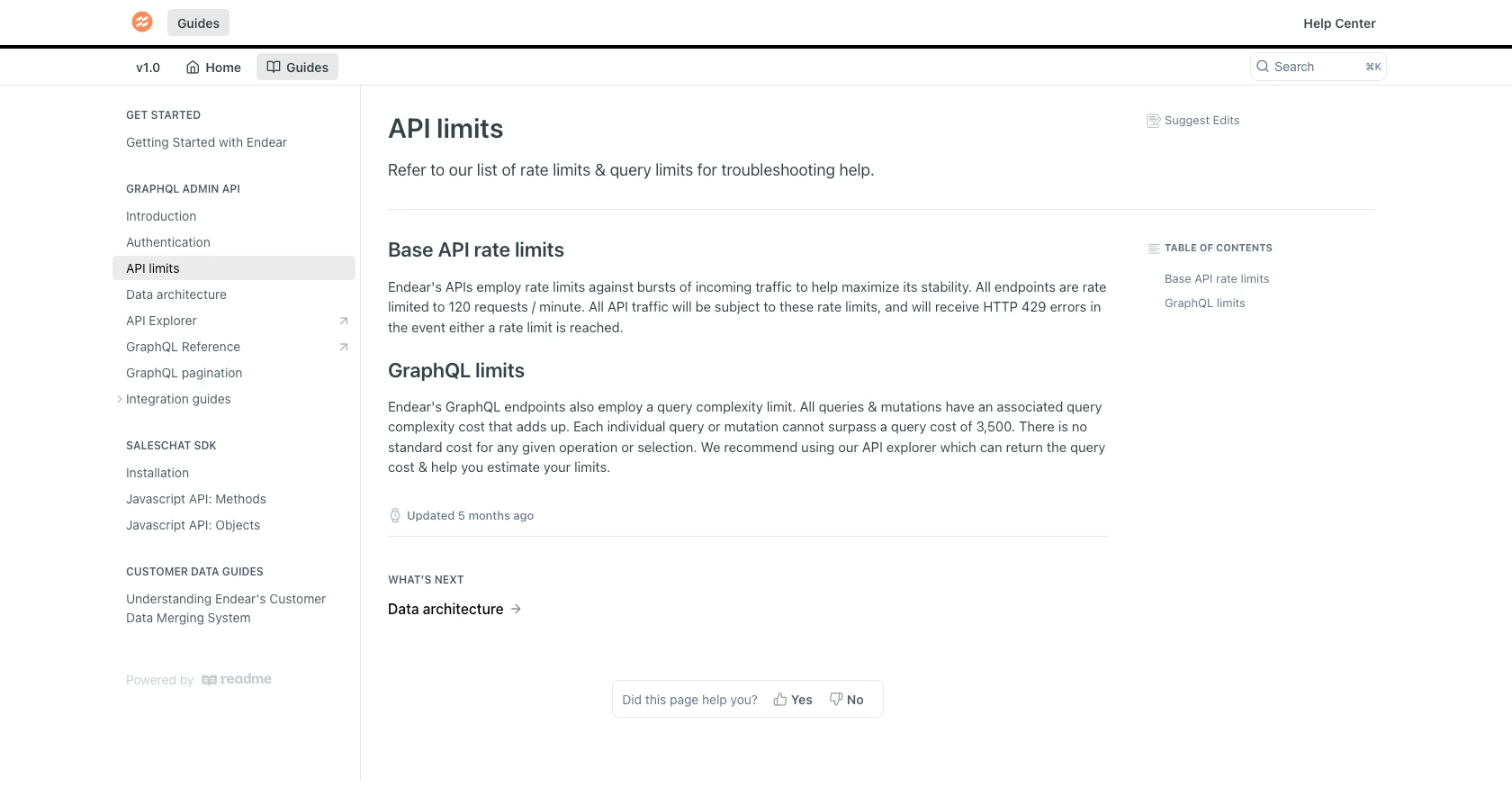
Conclusion and Best Practices for Using Endear API with Python
Integrating with the Endear API using Python provides a powerful way to manage and analyze customer data, enhancing your application's functionality and user experience. By following the steps outlined in this article, you can efficiently retrieve customer information and leverage it to improve marketing strategies and customer interactions.
Best Practices for Secure and Efficient Endear API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Endear's rate limit of 120 requests per minute. Implement retry mechanisms or exponential backoff strategies to manage rate limits effectively.
- Data Standardization: Ensure that customer data retrieved from Endear is standardized and transformed as needed for consistency across your application.
- Error Handling: Implement robust error handling to manage different HTTP status codes and ensure smooth operation of your integration.
Enhance Your Integration Strategy with Endgrate
While integrating with Endear's API can significantly enhance your application's capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Endear.
With Endgrate, you can save time and resources by building once for each use case, rather than multiple times for different integrations. This allows you to focus on your core product while providing an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration strategy by visiting Endgrate and discover the benefits of a unified API solution.
Read More
- https://endgrate.com/provider/endear
- https://docs.endearhq.com/docs/authentication
- https://docs.endearhq.com/docs/rate-limits-status-codes-and-errors
- https://docs.endearhq.com/docs/disclaimers
- https://developers.endearhq.com/docs/graphql/objects/Customer
- https://docs.endearhq.com/docs/send-your-first-record
Ready to get started?