How to Get Customers with the Microsoft Dynamics 365 Business Central API in PHP
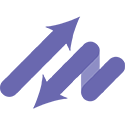
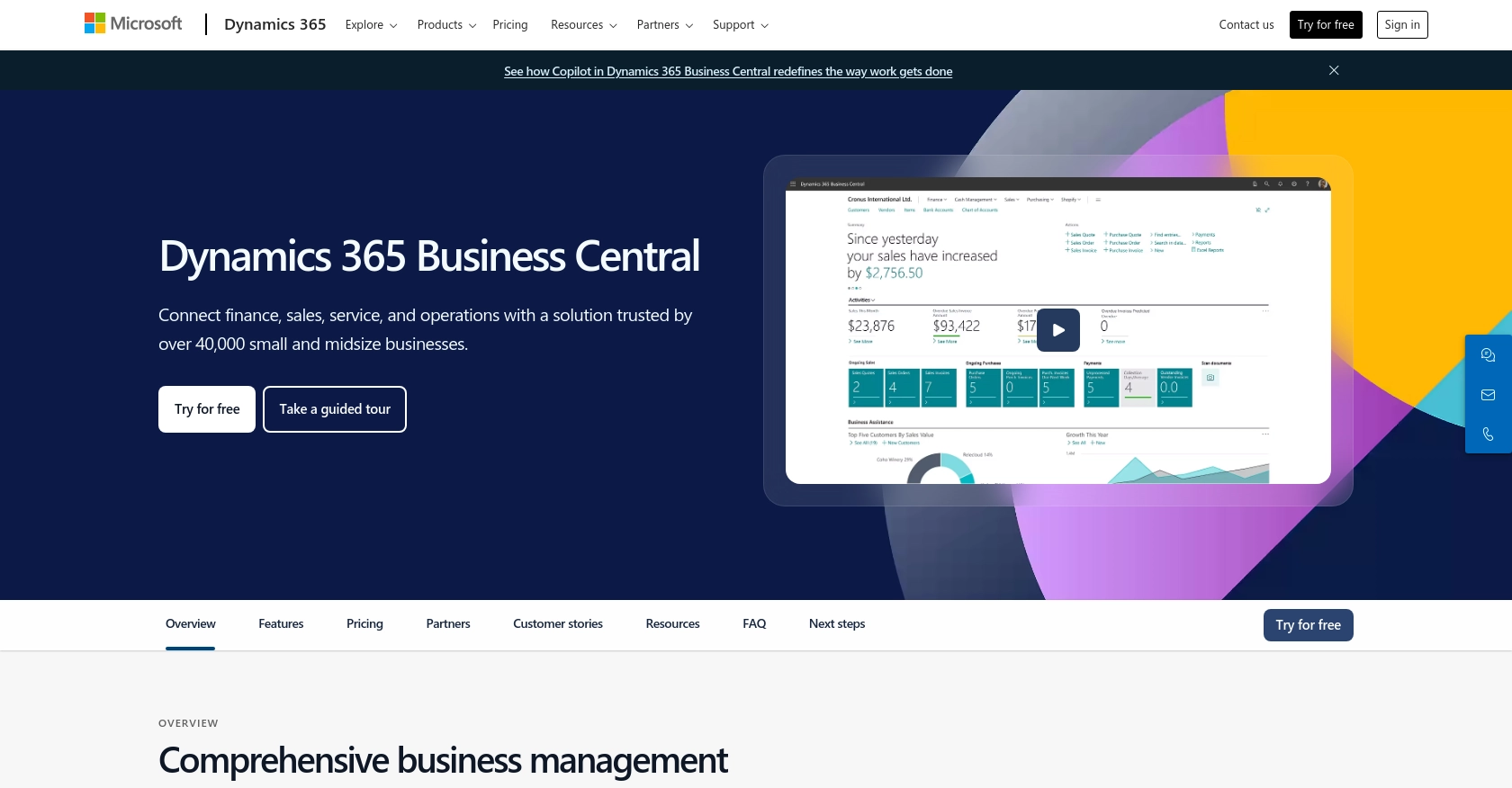
Introduction to Microsoft Dynamics 365 Business Central
Microsoft Dynamics 365 Business Central is a comprehensive business management solution designed for small to medium-sized enterprises. It integrates various business processes, including finance, sales, service, and operations, into a single platform, enabling companies to streamline their operations and make informed decisions.
Developers often seek to integrate with Microsoft Dynamics 365 Business Central to access and manage customer data efficiently. By leveraging the Business Central API, developers can automate tasks such as retrieving customer information, which can be used to enhance customer relationship management and improve service delivery.
For example, a developer might use the Business Central API to fetch customer details and integrate them into a custom CRM system, allowing sales teams to access up-to-date customer information and tailor their interactions accordingly.
Setting Up a Microsoft Dynamics 365 Business Central Sandbox Account
Before you can start integrating with the Microsoft Dynamics 365 Business Central API, you need to set up a sandbox account. This allows you to test your API interactions without affecting live data. Microsoft provides a free trial and sandbox environment for developers to experiment with their APIs.
Creating a Microsoft Dynamics 365 Business Central Sandbox Account
Follow these steps to create your sandbox account:
- Visit the Microsoft Dynamics 365 Business Central website and sign up for a free trial.
- Once registered, log in to your Microsoft account and navigate to the Business Central dashboard.
- In the dashboard, select Sandbox Environments to create a new sandbox instance.
- Follow the prompts to configure your sandbox environment, including selecting the region and language.
- Once the sandbox is set up, you will have access to a separate environment where you can safely test API calls.
Registering an App for OAuth Authentication
To interact with the Business Central API, you need to register an application in Microsoft Entra ID for OAuth authentication. This process involves obtaining a client ID and client secret, which are necessary for API authorization.
- Navigate to the Azure Portal and sign in with your Microsoft account.
- Go to Azure Active Directory and select App registrations.
- Click on New registration and fill in the required details, such as the application name and redirect URI.
- Once registered, note down the Application (client) ID and Directory (tenant) ID.
- Under the app's settings, navigate to Certificates & secrets and create a new client secret. Save this secret securely as it will not be shown again.
Configuring API Permissions
To ensure your application can access the necessary data, configure the API permissions:
- In the Azure Portal, go to your registered app and select API permissions.
- Click on Add a permission and choose Dynamics 365 Business Central.
- Select the permissions required for your application, such as user_impersonation for delegated access.
- Grant admin consent for the permissions to ensure they are active.
With your sandbox account and app registration set up, you are now ready to start making API calls to Microsoft Dynamics 365 Business Central using PHP.
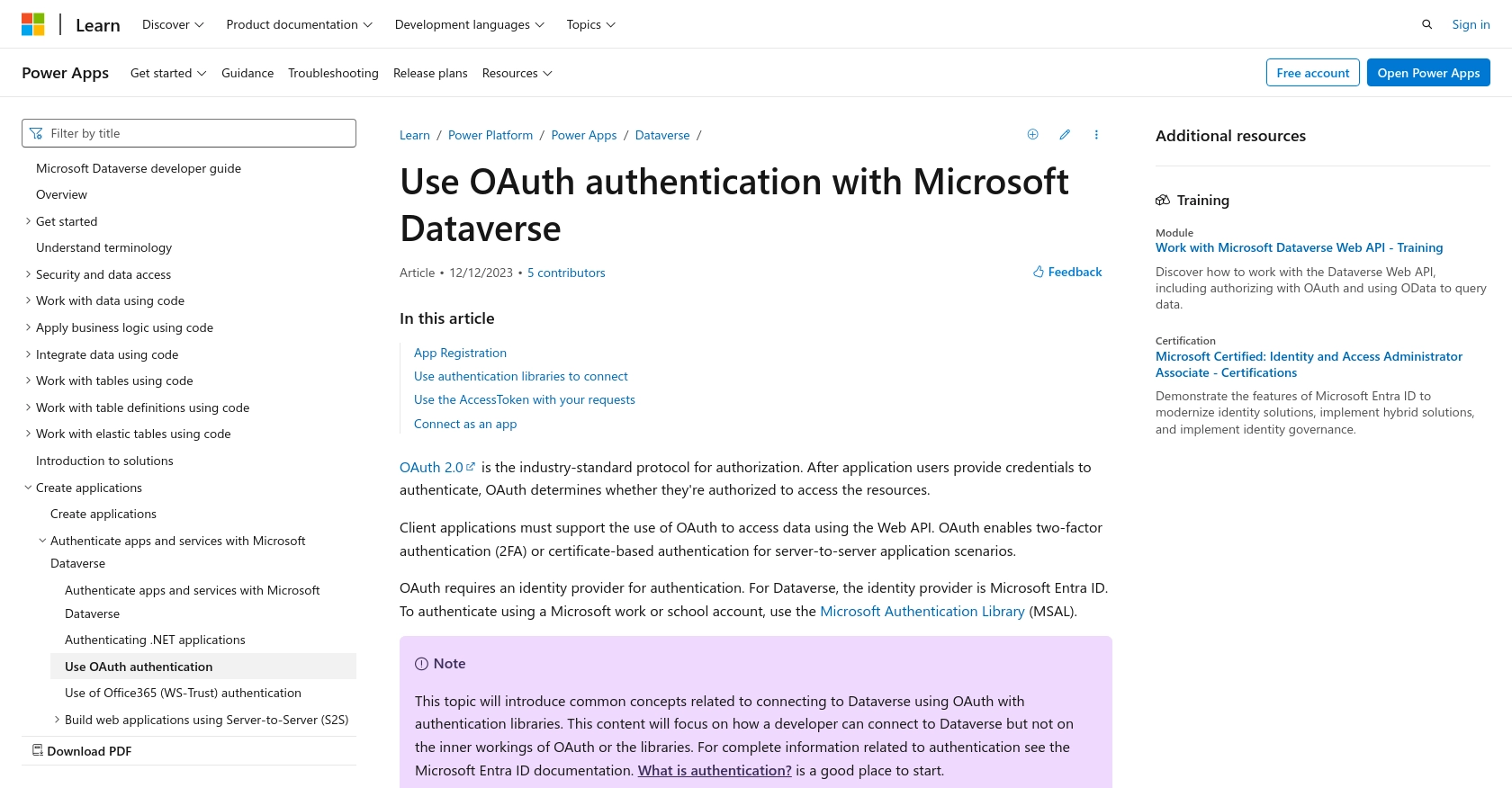
sbb-itb-96038d7
Making API Calls to Retrieve Customers from Microsoft Dynamics 365 Business Central Using PHP
In this section, we'll guide you through the process of making API calls to retrieve customer data from Microsoft Dynamics 365 Business Central using PHP. This involves setting up your PHP environment, writing the code to interact with the API, and handling potential errors.
Setting Up Your PHP Environment
Before making API calls, ensure your PHP environment is correctly configured. You will need:
- PHP 7.4 or later
- Composer for dependency management
- The
guzzlehttp/guzzle
library for making HTTP requests
Install the Guzzle library using Composer with the following command:
composer require guzzlehttp/guzzle
Writing the PHP Code to Call the Microsoft Dynamics 365 Business Central API
With your environment set up, you can now write the PHP code to retrieve customer data. Create a file named get_customers.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
use GuzzleHttp\Exception\RequestException;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your actual access token
$companyId = 'Your_Company_ID'; // Replace with your actual company ID
try {
$response = $client->request('GET', "https://api.businesscentral.dynamics.com/v2.0/companies/{$companyId}/customers", [
'headers' => [
'Authorization' => "Bearer {$accessToken}",
'Accept' => 'application/json',
]
]);
$customers = json_decode($response->getBody(), true);
foreach ($customers['value'] as $customer) {
echo "Customer Name: " . $customer['displayName'] . "\n";
echo "Customer Email: " . $customer['email'] . "\n\n";
}
} catch (RequestException $e) {
if ($e->hasResponse()) {
echo "Error: " . $e->getResponse()->getBody();
} else {
echo "Request failed: " . $e->getMessage();
}
}
Replace Your_Access_Token
and Your_Company_ID
with your actual access token and company ID obtained from the Microsoft Entra ID registration process.
Running the PHP Script and Verifying the Output
Run the script from the command line using:
php get_customers.php
If successful, the script will output the names and emails of customers retrieved from your Microsoft Dynamics 365 Business Central sandbox environment.
Handling Errors and Common Issues
When making API calls, you may encounter errors such as authentication failures or rate limits. Here are some tips for handling these issues:
- Ensure your access token is valid and not expired. Tokens typically expire after a set period and need refreshing.
- Check the API response for error codes and messages to diagnose issues. Common HTTP status codes include 401 (Unauthorized) and 429 (Too Many Requests).
- Implement error handling in your code to gracefully manage exceptions and retry requests if necessary.
For more detailed information on error codes, refer to the Microsoft Dynamics 365 Business Central API documentation.
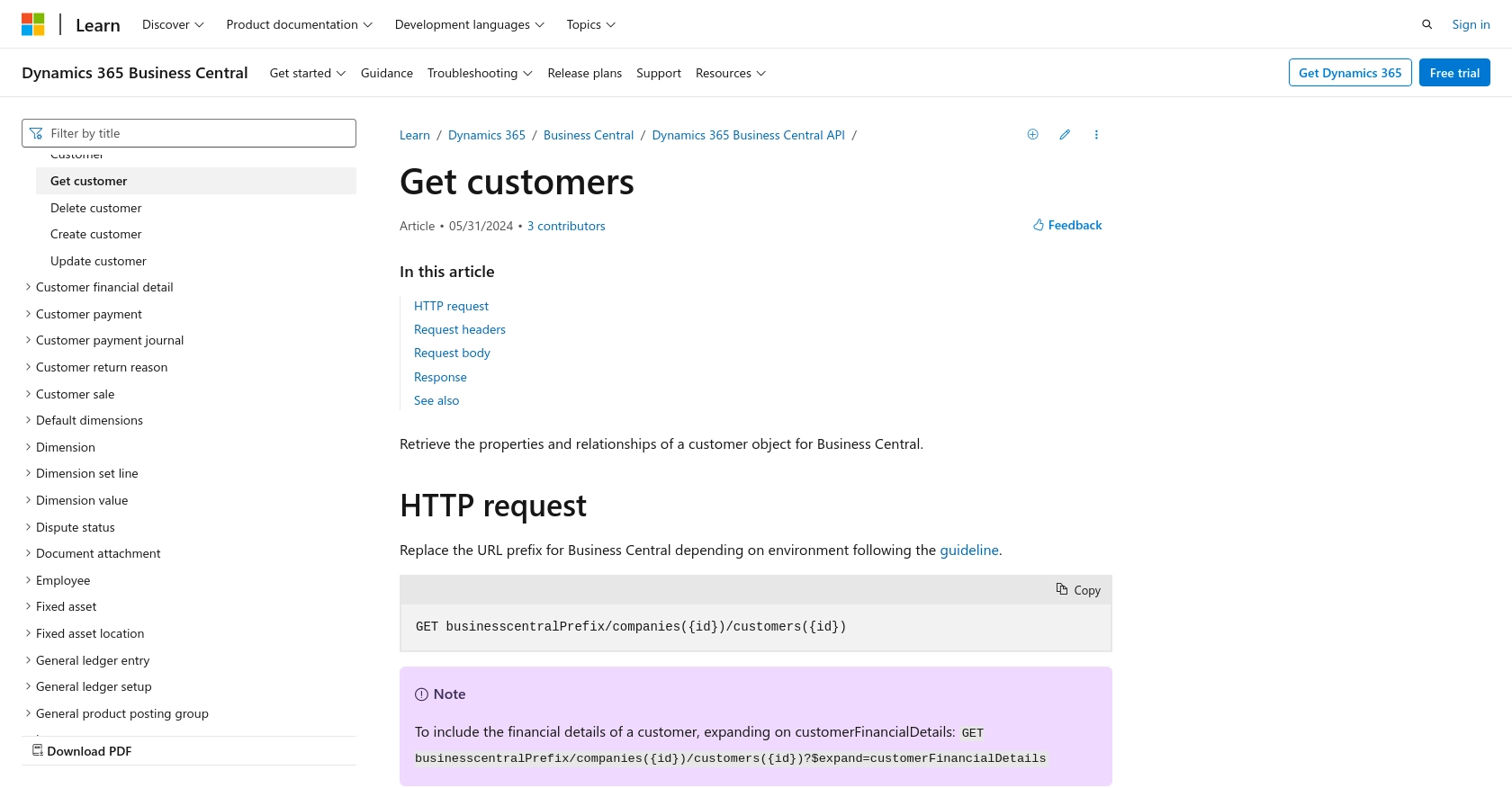
Conclusion and Best Practices for Using Microsoft Dynamics 365 Business Central API with PHP
Integrating with the Microsoft Dynamics 365 Business Central API using PHP can significantly enhance your business operations by automating data retrieval and management tasks. By following the steps outlined in this guide, you can efficiently access customer data and integrate it into your systems, providing your team with up-to-date information to improve customer interactions.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of API rate limits to avoid throttling. Implement exponential backoff strategies to handle retries gracefully when you encounter HTTP 429 (Too Many Requests) errors.
- Refresh Tokens Regularly: OAuth tokens have expiration times. Implement token refresh logic to ensure uninterrupted access to the API.
- Standardize Data: Transform and standardize data fields to ensure consistency across different systems and applications.
Enhance Your Integration Strategy with Endgrate
While integrating with Microsoft Dynamics 365 Business Central can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Microsoft Dynamics 365 Business Central.
With Endgrate, you can save time and resources by building once for each use case instead of multiple times for different integrations. This allows you to focus on your core product while delivering an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/microsoftdynamics365businesscentral
- https://learn.microsoft.com/en-us/power-apps/developer/data-platform/authenticate-oauth
- https://learn.microsoft.com/en-us/graph/use-the-api
- https://learn.microsoft.com/en-us/dynamics365/business-central/dev-itpro/api-reference/v2.0/api/dynamics_customer_get
Ready to get started?