How to Get Customers with the Quickbooks API in PHP
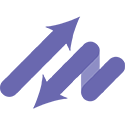
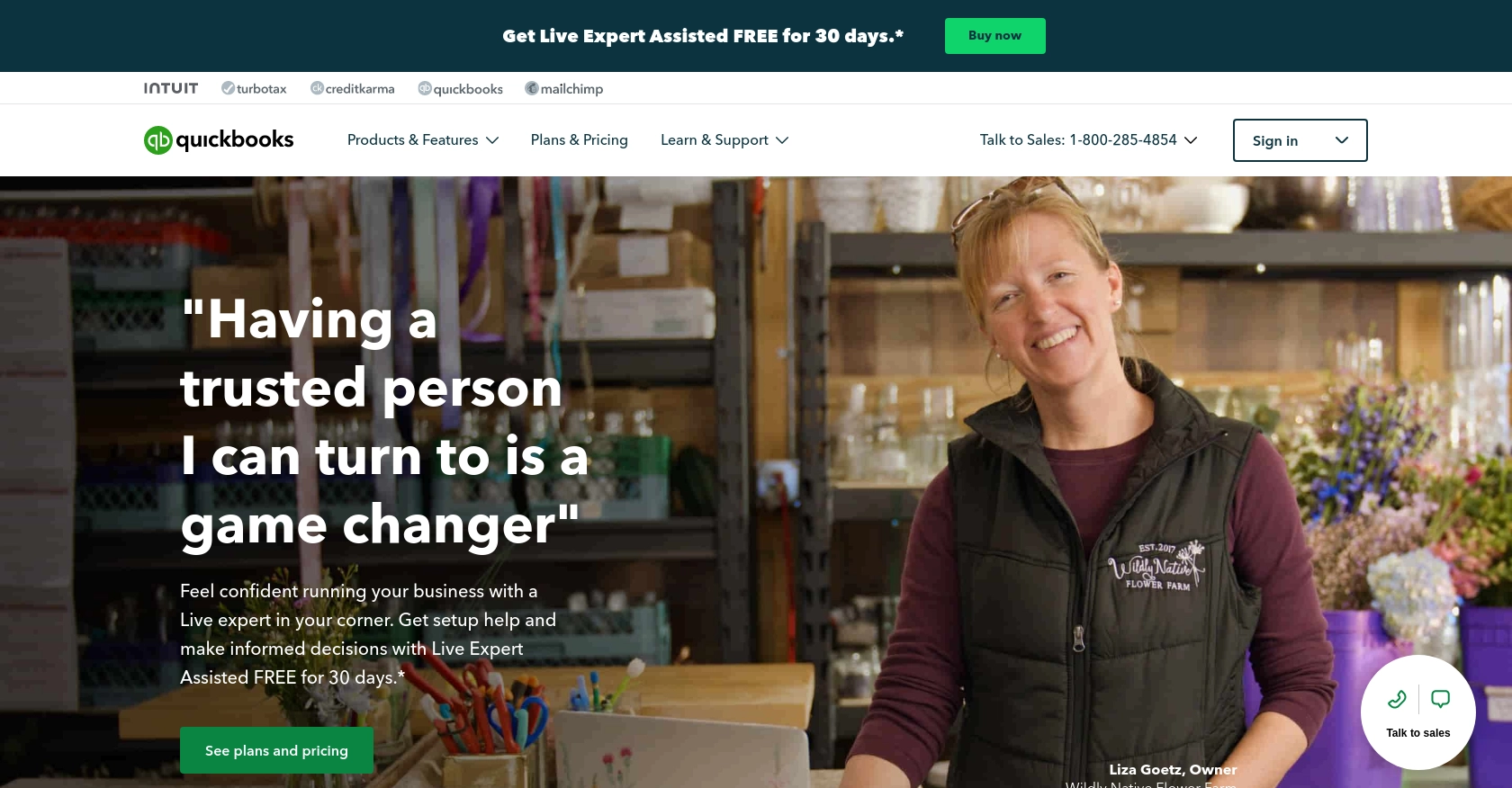
Introduction to QuickBooks API
QuickBooks is a widely used accounting software that offers comprehensive financial management solutions for businesses of all sizes. It provides tools for invoicing, expense tracking, payroll, and more, making it an essential platform for managing business finances efficiently.
Integrating with the QuickBooks API allows developers to access and manage financial data programmatically. For example, a developer might want to retrieve customer information from QuickBooks to synchronize with a CRM system, ensuring that customer records are up-to-date across platforms.
Setting Up a QuickBooks Test/Sandbox Account for API Integration
Before you can start interacting with the QuickBooks API, you need to set up a test or sandbox account. This environment allows you to safely develop and test your integration without affecting live data.
Creating a QuickBooks Developer Account
To begin, you'll need a QuickBooks Developer account. Follow these steps to create one:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" and fill in the required information to create your account.
- Once registered, log in to your developer account.
Setting Up a QuickBooks Sandbox Company
After creating your developer account, you can set up a sandbox company to test your API calls:
- Navigate to the "Dashboard" in your QuickBooks Developer account.
- Select "Sandbox" from the menu and click on "Add Sandbox Company."
- Follow the prompts to create a new sandbox company, which will mimic a real QuickBooks environment.
Creating a QuickBooks App for OAuth Authentication
QuickBooks API uses OAuth for authentication. You'll need to create an app to obtain the necessary credentials:
- In your QuickBooks Developer account, go to the App Management section.
- Click on "Create an App" and select "QuickBooks Online and Payments."
- Fill in the required details, such as app name and description.
- Once the app is created, navigate to the "Keys & OAuth" section to find your Client ID and Client Secret.
Configuring OAuth Settings for QuickBooks API
To ensure your app can authenticate with the QuickBooks API, configure the OAuth settings:
- In the app settings, set the Redirect URI to a valid endpoint where you can handle OAuth callbacks.
- Ensure that the scopes required for accessing customer data are selected.
- Save your settings to finalize the configuration.
With your sandbox account and app set up, you are now ready to start making API calls to QuickBooks. This setup ensures that you can safely develop and test your integration without impacting live data.
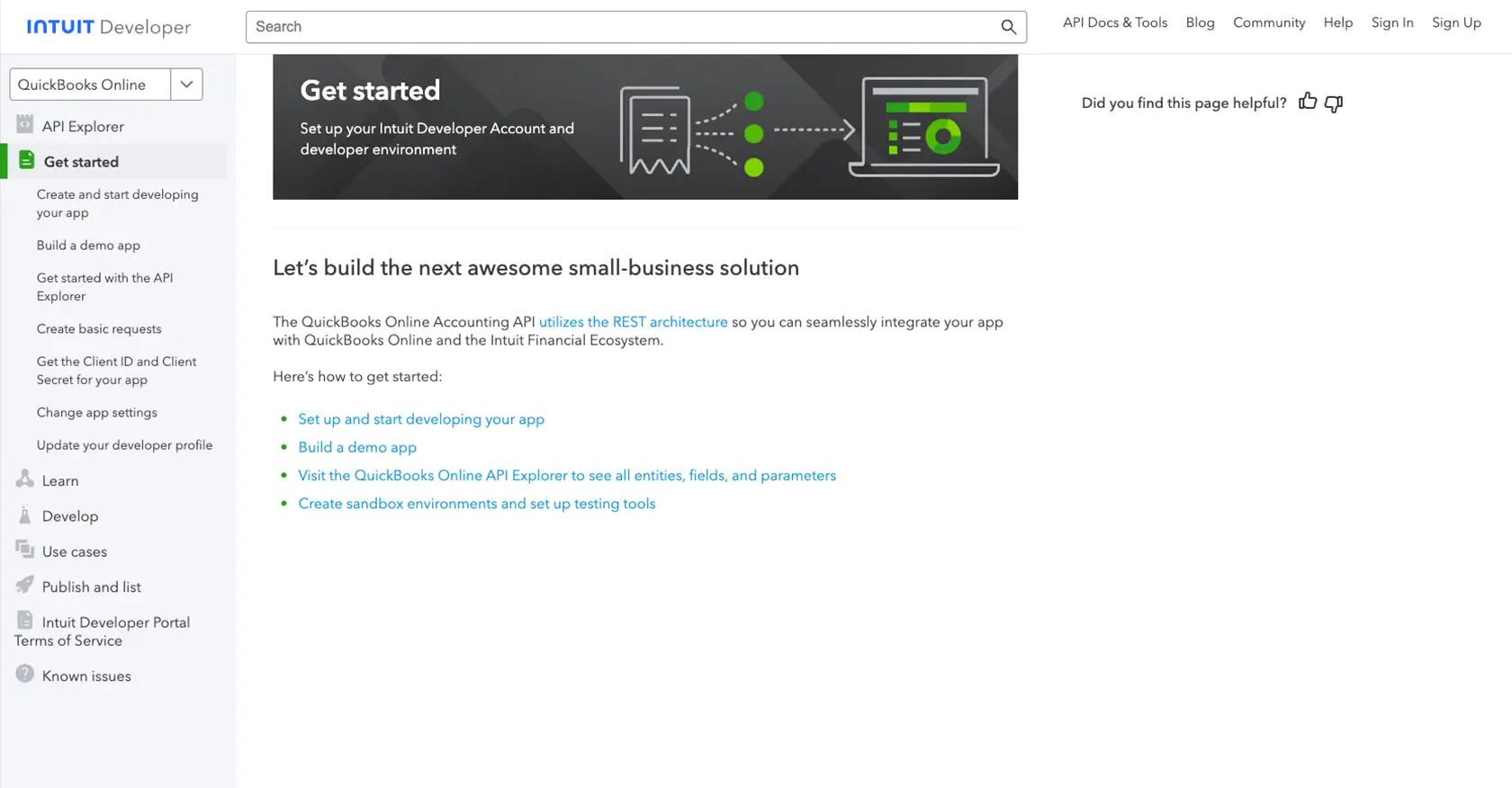
sbb-itb-96038d7
Making API Calls to Retrieve Customers from QuickBooks Using PHP
To interact with the QuickBooks API and retrieve customer data, you'll need to use PHP, a popular server-side scripting language. This section will guide you through the process of setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for QuickBooks API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
Once you have these installed, use Composer to install the guzzlehttp/guzzle
library, which simplifies HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Customers from QuickBooks
Create a new PHP file named get_quickbooks_customers.php
and add the following code:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token';
$realmId = 'Your_Realm_ID';
$response = $client->request('GET', 'https://quickbooks.api.intuit.com/v3/company/' . $realmId . '/query', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
'Content-Type' => 'application/text'
],
'query' => [
'query' => "SELECT * FROM Customer"
]
]);
if ($response->getStatusCode() == 200) {
$customers = json_decode($response->getBody()->getContents(), true);
foreach ($customers['QueryResponse']['Customer'] as $customer) {
echo 'Customer Name: ' . $customer['DisplayName'] . "\n";
}
} else {
echo 'Failed to retrieve customers. Status code: ' . $response->getStatusCode();
}
Replace Your_Access_Token
and Your_Realm_ID
with the actual values obtained from your QuickBooks app settings.
Running the PHP Script and Verifying the Output
Execute the script from the command line using the following command:
php get_quickbooks_customers.php
If successful, you should see a list of customer names printed in the console. This confirms that your API call to QuickBooks was successful.
Handling Errors and Debugging QuickBooks API Calls
When making API calls, it's essential to handle potential errors. QuickBooks API may return various error codes, such as:
- 401 Unauthorized: Check your access token and ensure it's valid.
- 403 Forbidden: Verify that your app has the necessary permissions.
- 500 Internal Server Error: Retry the request or contact support if the issue persists.
Refer to the QuickBooks API documentation for more details on error handling.
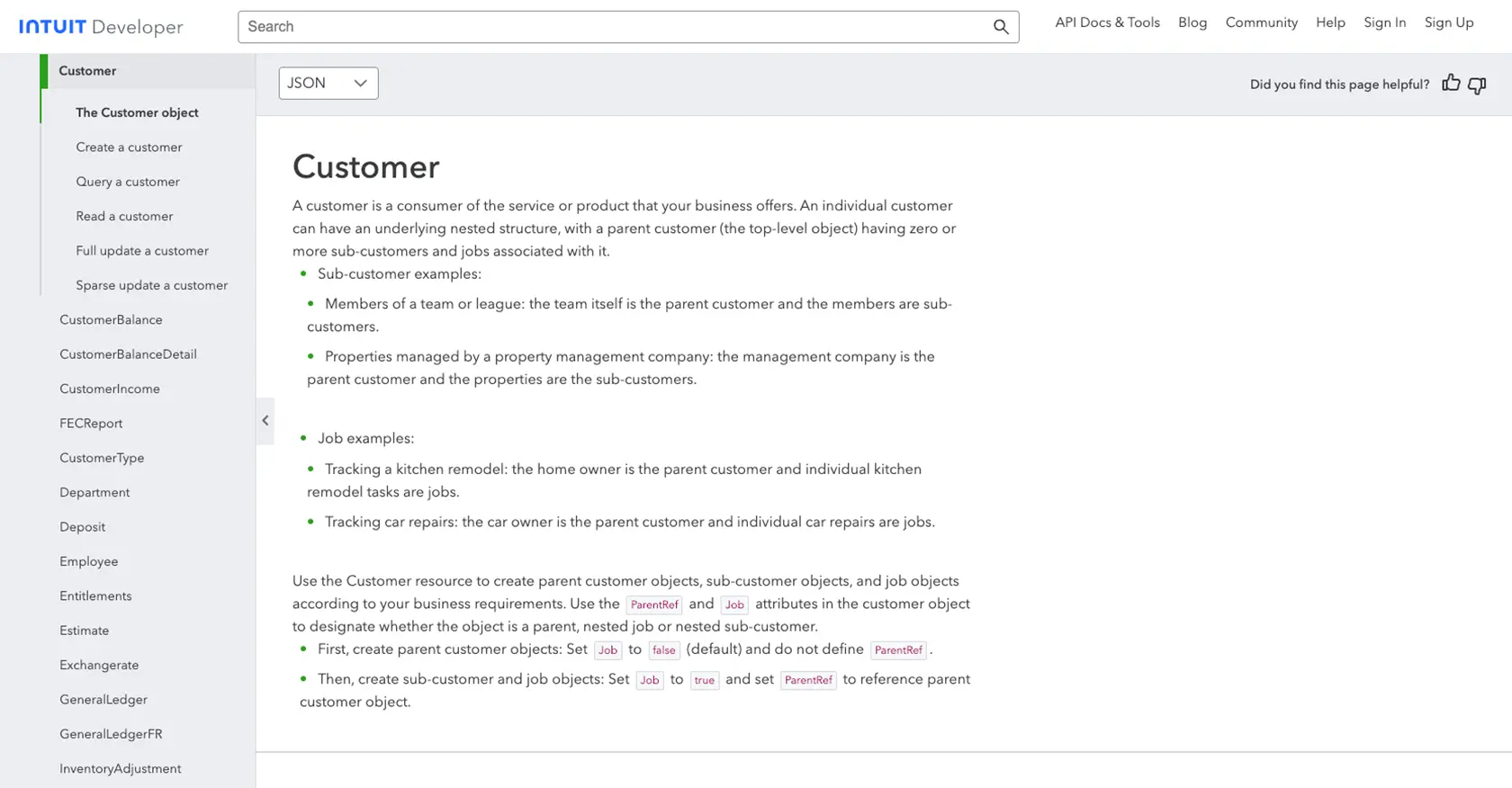
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API using PHP allows developers to efficiently manage and synchronize financial data across platforms. By following the steps outlined in this guide, you can successfully retrieve customer information and ensure your application interacts seamlessly with QuickBooks.
Best Practices for Secure and Efficient QuickBooks API Usage
- Securely Store Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handle Rate Limiting: QuickBooks API has rate limits to prevent abuse. Monitor your API usage and implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from QuickBooks is transformed and standardized to match your application's data schema, facilitating smooth data integration.
Streamlining Integrations with Endgrate
While integrating with QuickBooks API can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by providing a single endpoint to connect with various platforms, including QuickBooks.
By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can streamline your integration efforts.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/customer
Ready to get started?