How to Get Customers with the Shopify API in PHP
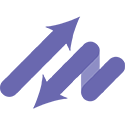
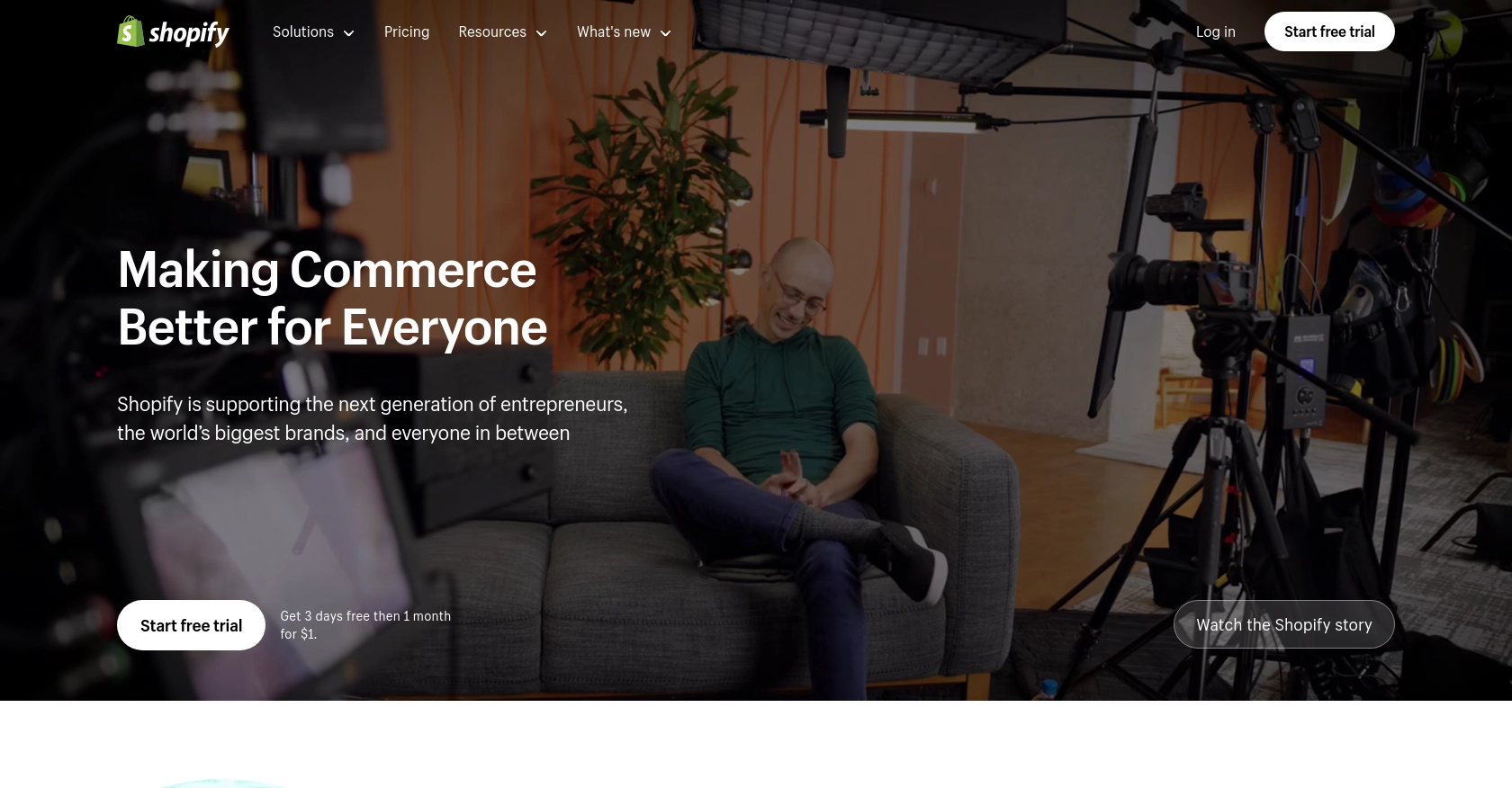
Introduction to Shopify API for Customer Management
Shopify is a leading e-commerce platform that empowers businesses to create and manage their online stores with ease. With a comprehensive suite of tools, Shopify enables merchants to handle everything from product listings to payment processing and customer management.
For developers, integrating with the Shopify API offers a powerful way to automate and enhance store operations. By connecting to the Shopify API, developers can access and manage customer data, streamline order processing, and improve customer engagement. For example, you might use the Shopify API to retrieve customer information and personalize marketing campaigns, enhancing customer experience and boosting sales.
This article will guide you through the process of using PHP to interact with the Shopify API, specifically focusing on retrieving customer data. By following this tutorial, you'll learn how to efficiently access and manage customer information on the Shopify platform using PHP.
Setting Up Your Shopify Test Account for API Integration
Before you can start interacting with the Shopify API using PHP, you need to set up a Shopify test account. This will allow you to safely experiment with API calls without affecting a live store. Shopify provides a development environment where you can create a test store and use it to test your integrations.
Create a Shopify Partner Account
To begin, you'll need a Shopify Partner account. This account allows you to create development stores and access Shopify's API for testing purposes.
- Visit the Shopify Partners page and sign up for a free account.
- Once registered, log in to your Shopify Partner dashboard.
Create a Shopify Development Store
With your Partner account set up, you can now create a development store:
- In the Shopify Partner dashboard, navigate to Stores and click on Add store.
- Select Development store as the store type.
- Fill in the required details, such as store name and password, and click Create store.
Set Up OAuth Authentication for Shopify API
Shopify uses OAuth 2.0 for authentication. You'll need to create a private app to obtain the necessary credentials:
- In your development store, go to Apps and click on Develop apps.
- Click Create an app and provide a name for your app.
- Under Configuration, select the necessary API scopes to access customer data.
- Click Install app to generate your API key and API secret key.
For more detailed information on setting up OAuth, refer to the Shopify Admin API documentation.
Generate Access Tokens
Once your app is created, you need to generate an access token to authenticate your API requests:
- Use the API key and secret key to request an access token from Shopify.
- Include the access token in the
X-Shopify-Access-Token
header of your API requests.
For further guidance on authentication, see the Shopify API authentication documentation.
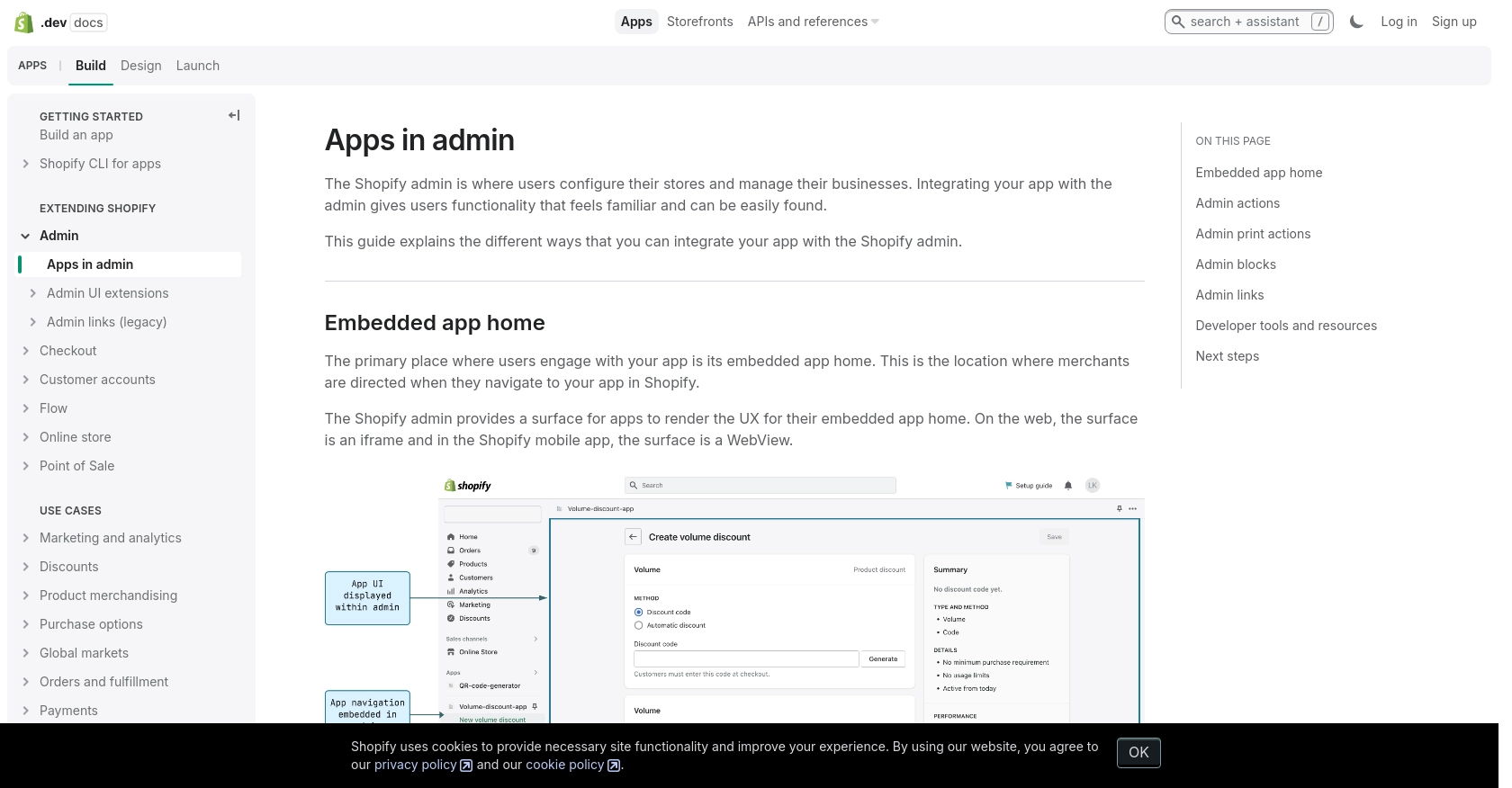
sbb-itb-96038d7
Making API Calls to Retrieve Shopify Customer Data Using PHP
To interact with the Shopify API and retrieve customer data using PHP, you'll need to ensure your development environment is properly set up. This section will guide you through the necessary steps, including setting up PHP, installing dependencies, and writing the code to make API calls.
Setting Up Your PHP Environment for Shopify API Integration
Before you begin, make sure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer, the PHP package manager
Once you have these installed, you can proceed to install the required libraries for making HTTP requests.
Installing Required PHP Libraries for Shopify API
To make HTTP requests to the Shopify API, you'll need to install the Guzzle HTTP client. You can do this using Composer:
composer require guzzlehttp/guzzle
This command will add Guzzle to your project, allowing you to easily handle HTTP requests and responses.
Writing PHP Code to Retrieve Shopify Customers
With your environment set up, you can now write the PHP code to retrieve customer data from Shopify. Create a new PHP file named get_shopify_customers.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle client
$client = new Client();
// Set the API endpoint and headers
$storeName = 'your-development-store';
$accessToken = 'your_access_token';
$endpoint = "https://{$storeName}.myshopify.com/admin/api/2024-07/customers.json";
$headers = [
'Content-Type' => 'application/json',
'X-Shopify-Access-Token' => $accessToken
];
// Make a GET request to the Shopify API
$response = $client->request('GET', $endpoint, ['headers' => $headers]);
// Parse the JSON response
$data = json_decode($response->getBody(), true);
// Display customer information
foreach ($data['customers'] as $customer) {
echo "Customer ID: " . $customer['id'] . "\n";
echo "Email: " . $customer['email'] . "\n";
echo "First Name: " . $customer['first_name'] . "\n";
echo "Last Name: " . $customer['last_name'] . "\n\n";
}
?>
Replace your-development-store
and your_access_token
with your actual store name and access token.
Running the PHP Script to Fetch Shopify Customers
To execute the script and retrieve customer data, run the following command in your terminal:
php get_shopify_customers.php
You should see a list of customers printed in the terminal, including their IDs, emails, and names.
Handling Shopify API Errors and Responses
When making API calls, it's important to handle potential errors. The Shopify API may return various HTTP status codes indicating the result of your request:
- 200 OK: The request was successful.
- 401 Unauthorized: Authentication failed. Check your access token.
- 429 Too Many Requests: You've exceeded the rate limit. Wait before retrying.
For more information on handling errors, refer to the Shopify API documentation.
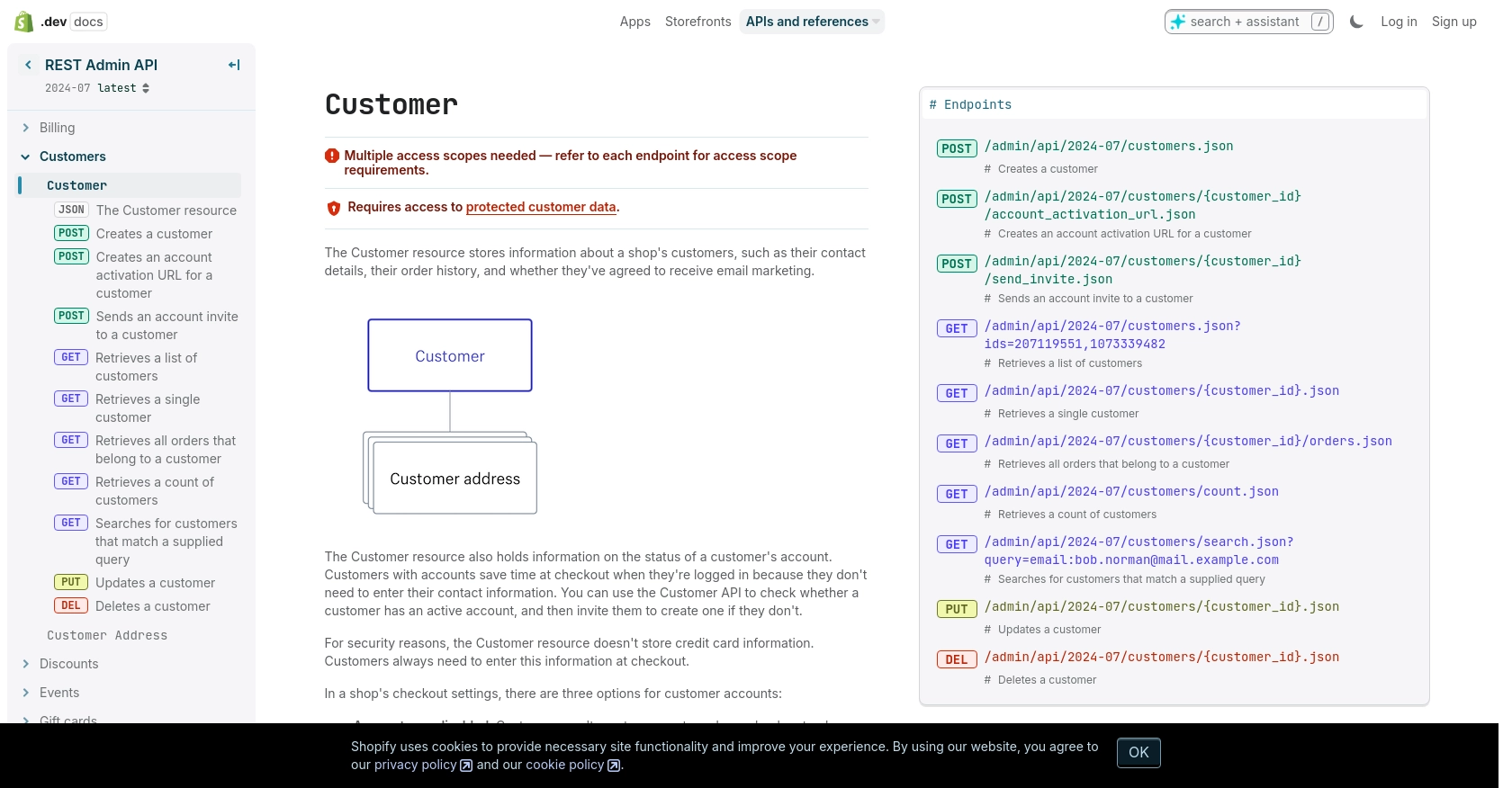
Conclusion and Best Practices for Shopify API Integration Using PHP
Integrating with the Shopify API using PHP provides a robust solution for managing customer data and enhancing e-commerce operations. By following the steps outlined in this guide, you can efficiently retrieve and manage customer information, enabling personalized marketing and improved customer engagement.
Best Practices for Secure and Efficient Shopify API Usage
- Securely Store Credentials: Always store your API credentials securely. Avoid hardcoding them in your scripts. Instead, use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Shopify imposes a rate limit of 40 requests per app per store per minute. Implement logic to handle the
429 Too Many Requests
error by pausing requests and retrying after the specified time. For more details, refer to the Shopify API documentation. - Data Standardization: Ensure that customer data retrieved from Shopify is standardized and transformed as needed for your application. This will help maintain consistency across different systems.
Leverage Endgrate for Streamlined Integration Management
While building integrations with the Shopify API can be rewarding, it can also be time-consuming and complex. Endgrate offers a powerful solution to simplify this process. By using Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, ensuring a consistent experience.
- Provide an intuitive integration experience for your customers, enhancing their satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?