How to Get Leads with the Mailshake API in Python
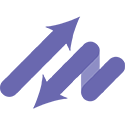
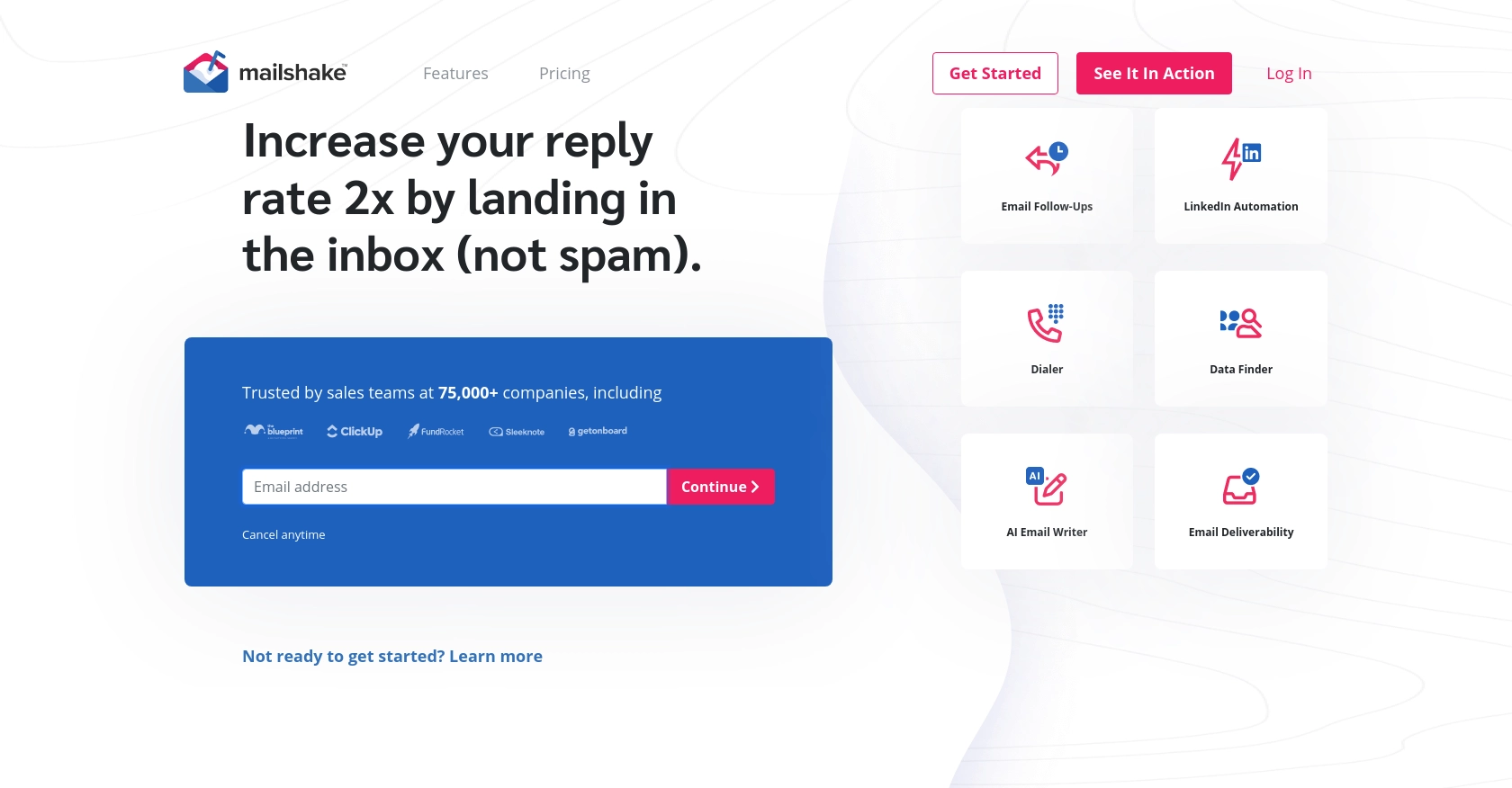
Introduction to Mailshake API for Lead Generation
Mailshake is a powerful sales engagement platform designed to streamline outreach efforts through email campaigns and social media. It offers a suite of tools that help sales teams automate and personalize their communication, making it easier to connect with prospects and convert them into leads.
Integrating with the Mailshake API allows developers to automate lead generation processes, such as retrieving and managing leads efficiently. For example, a developer can use the Mailshake API to extract leads from email campaigns, enabling seamless integration with CRM systems for enhanced sales tracking and follow-up.
Setting Up Your Mailshake API Test Account
Before you can start using the Mailshake API for lead generation, you need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data.
Create a Mailshake Account
If you don't already have a Mailshake account, you can sign up for a free trial on the Mailshake website. This will give you access to the platform's features and allow you to generate an API key for testing purposes.
- Visit the Mailshake website and click on "Sign Up".
- Follow the instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Generate Your Mailshake API Key
Mailshake uses API key-based authentication to secure API requests. Follow these steps to generate your API key:
- Navigate to the "Extensions" section in the Mailshake dashboard.
- Select "API" from the menu.
- Click on "Create API Key" to generate a new key.
- Copy the API key and store it securely, as you will need it to authenticate your API requests.
Test Your Mailshake API Connection
To ensure that your API key is working correctly, you can test the connection using a simple API call. Here's how you can do it using Python:
import requests
# Set the API endpoint and headers
endpoint = "https://api.mailshake.com/2017-04-01/me"
headers = {"Authorization": "Basic YOUR_BASE64_ENCODED_API_KEY"}
# Make a GET request to test the connection
response = requests.get(endpoint, headers=headers)
# Check the response
if response.status_code == 200:
print("Connection successful!")
else:
print("Failed to connect:", response.json())
Replace YOUR_BASE64_ENCODED_API_KEY
with your actual API key encoded in base64 format. If the connection is successful, you should see a confirmation message.
For more details on authentication, refer to the Mailshake API documentation.
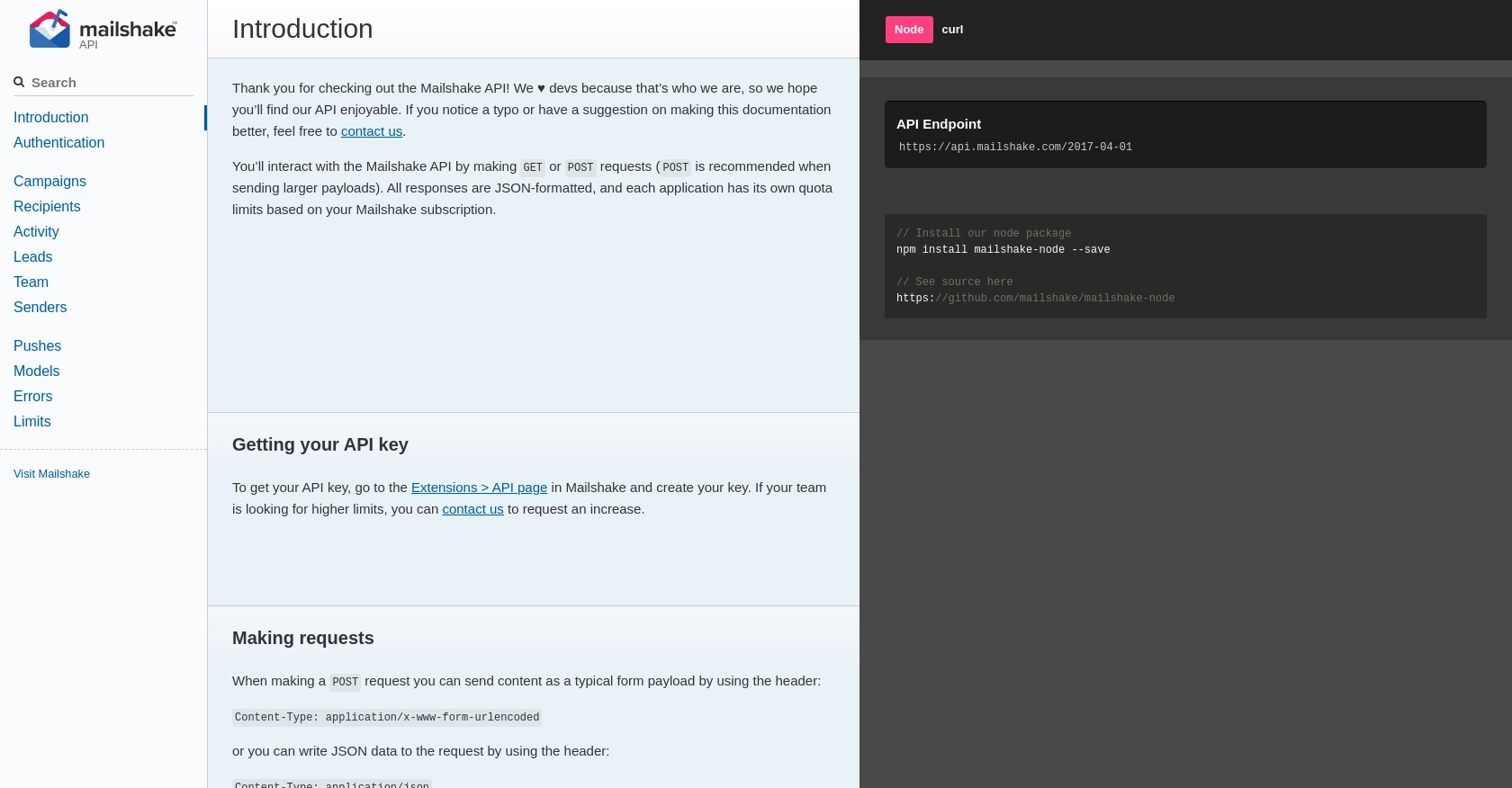
sbb-itb-96038d7
How to Make API Calls to Retrieve Leads with Mailshake in Python
To effectively interact with the Mailshake API for lead generation, you'll need to make API calls using Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Mailshake API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python from the official website if you haven't already.
- Use pip to install the
requests
library by running the following command in your terminal:
pip install requests
Writing Python Code to Retrieve Leads from Mailshake
Now that your environment is ready, you can write a Python script to retrieve leads from Mailshake. Here's a step-by-step guide:
import requests
# Define the API endpoint and headers
endpoint = "https://api.mailshake.com/2017-04-01/leads/list"
headers = {
"Authorization": "Basic YOUR_BASE64_ENCODED_API_KEY",
"Content-Type": "application/json"
}
# Set the parameters for the API call
params = {
"campaignID": 1, # Replace with your campaign ID
"perPage": 100
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers, params=params)
# Check the response status
if response.status_code == 200:
leads = response.json()
print("Leads retrieved successfully:", leads)
else:
print("Failed to retrieve leads:", response.json())
Replace YOUR_BASE64_ENCODED_API_KEY
with your actual API key encoded in base64 format. The campaignID
should be replaced with the ID of the campaign you wish to retrieve leads from.
Handling API Response and Errors
After making the API call, it's crucial to handle the response correctly. If the request is successful, the leads will be printed. If not, the error message will be displayed. Common error codes include:
invalid_api_key
: The API key is missing or incorrect.not_found
: The specified campaign or resource could not be found.limit_reached
: You've exceeded your quota and must wait before making more requests.
For more detailed error handling, refer to the Mailshake API documentation.
Verifying API Call Success in Mailshake Dashboard
To ensure that the API call was successful, you can verify the retrieved leads in your Mailshake dashboard. Navigate to the leads section and confirm that the data matches the output from your script.
Conclusion and Best Practices for Using Mailshake API in Python
Integrating with the Mailshake API can significantly enhance your lead generation efforts by automating the retrieval and management of leads. By following the steps outlined in this guide, you can efficiently connect to the Mailshake API using Python and streamline your sales processes.
Best Practices for Secure and Efficient Mailshake API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Mailshake's rate limits. Implement logic to handle the
limit_reached
error and retry requests after the specified wait time. For more details, refer to the Mailshake API documentation. - Data Standardization: Ensure that the data retrieved from Mailshake is standardized before integrating it with other systems, such as CRMs, to maintain consistency.
Enhance Your Integration Strategy with Endgrate
While integrating with the Mailshake API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Mailshake. This allows you to focus on your core product while outsourcing integration management.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can streamline your integration strategy and save valuable time and resources.
Read More
Ready to get started?