Using the Airtable API to Get Users (with PHP examples)
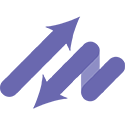
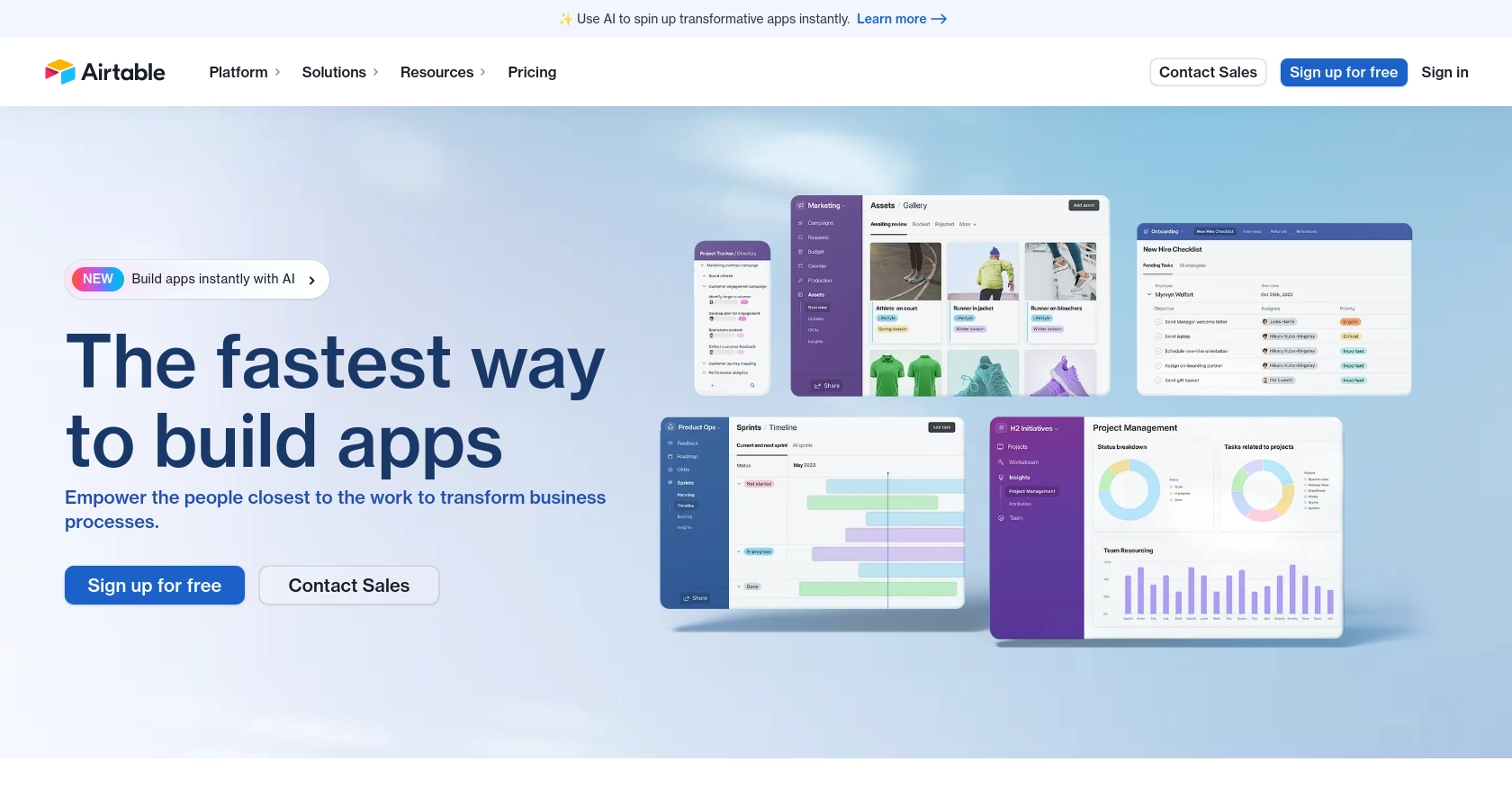
Introduction to Airtable API Integration
Airtable is a versatile cloud collaboration service that combines the simplicity of a spreadsheet with the power of a database. It is widely used by businesses to organize and manage data across various projects and teams, offering a flexible platform for tracking tasks, managing workflows, and collaborating in real-time.
Developers may want to integrate with Airtable's API to access and manage user data efficiently. For example, using the Airtable API, a developer can retrieve user information to synchronize team member details across different applications, ensuring that all systems reflect the most current data.
This article will guide you through using PHP to interact with the Airtable API, specifically focusing on retrieving user information. By following this tutorial, you will learn how to set up authentication, make API calls, and handle responses effectively.
Setting Up Your Airtable Test Account for API Integration
Before diving into the Airtable API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Airtable offers a free version that is perfect for development and testing purposes.
Creating an Airtable Account
If you don't already have an Airtable account, follow these steps to create one:
- Visit the Airtable signup page and register for a free account.
- Follow the on-screen instructions to complete your registration.
- Once registered, log in to your Airtable dashboard.
Setting Up OAuth for Airtable API Access
Airtable uses OAuth for authentication, which is essential for securely accessing user data. Follow these steps to set up OAuth for your application:
- Navigate to the Airtable OAuth creation page.
- Create a new OAuth integration by providing the necessary details such as the name, description, and redirect URI.
- Set the required scopes for your integration. For retrieving user data, ensure you include the
enterprise.user:read
scope. - Save your integration settings to generate a client ID and client secret.
Generating Access Tokens
With your OAuth integration set up, you can now generate access tokens to authenticate API requests:
- Direct users to the authorization URL provided by Airtable, including your client ID and requested scopes.
- Upon user approval, Airtable will redirect to your specified URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to Airtable's token endpoint.
// Example PHP code to exchange authorization code for access token
$client_id = 'YOUR_CLIENT_ID';
$client_secret = 'YOUR_CLIENT_SECRET';
$redirect_uri = 'YOUR_REDIRECT_URI';
$authorization_code = 'AUTHORIZATION_CODE';
$token_url = 'https://airtable.com/oauth2/v1/token';
$data = [
'grant_type' => 'authorization_code',
'code' => $authorization_code,
'redirect_uri' => $redirect_uri,
'client_id' => $client_id,
'client_secret' => $client_secret
];
$options = [
'http' => [
'header' => "Content-Type: application/x-www-form-urlencoded\r\n",
'method' => 'POST',
'content' => http_build_query($data)
]
];
$context = stream_context_create($options);
$response = file_get_contents($token_url, false, $context);
$token_info = json_decode($response, true);
$access_token = $token_info['access_token'];
Store the access token securely, as it will be used to authenticate your API requests.
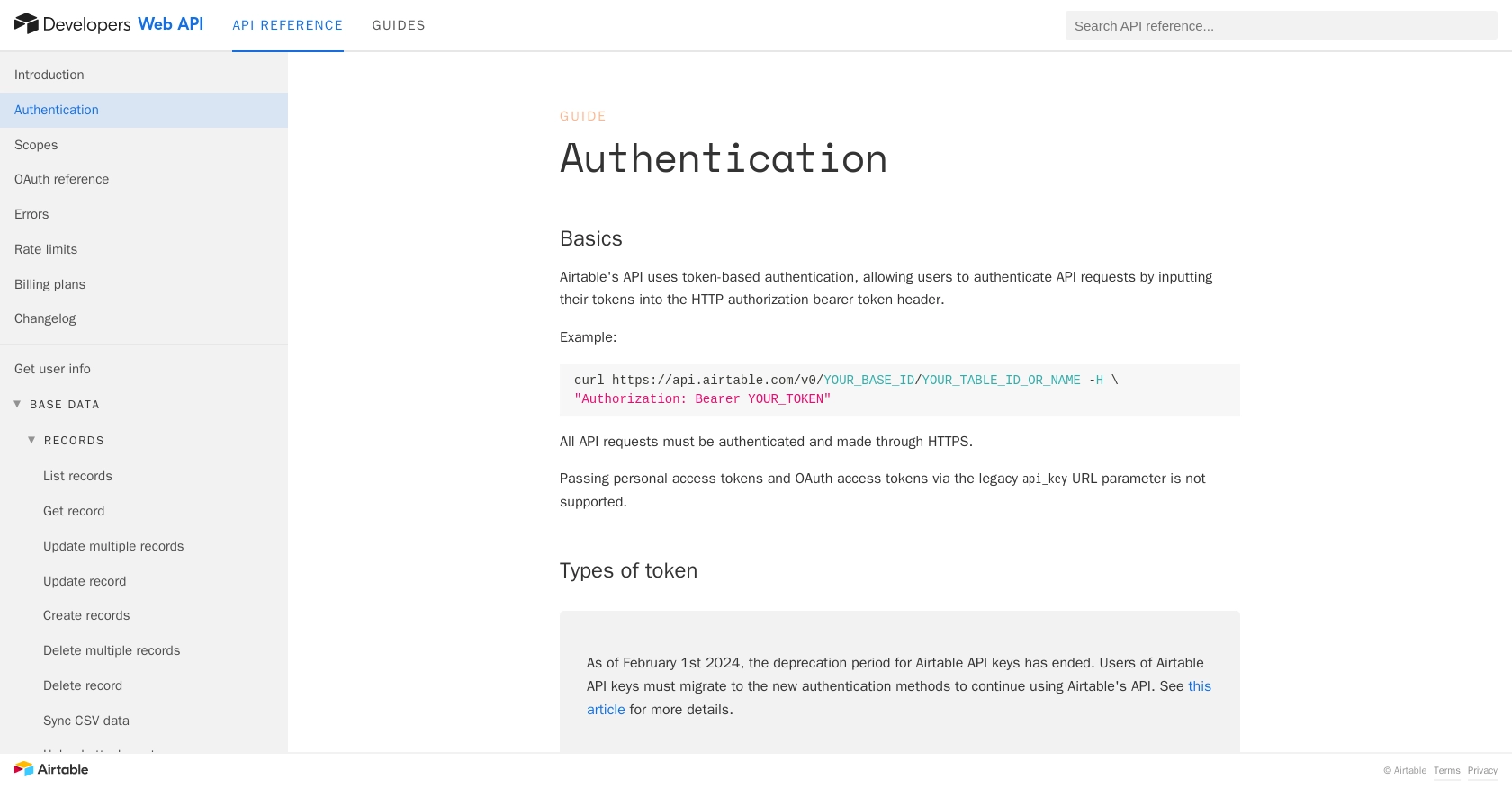
sbb-itb-96038d7
Making API Calls to Retrieve Users from Airtable Using PHP
To interact with the Airtable API and retrieve user information, you'll need to make HTTP requests using PHP. This section will guide you through the process of setting up your environment, writing the necessary code, and handling the API response.
Setting Up Your PHP Environment for Airtable API Integration
Before making API calls, ensure your PHP environment is ready. You'll need PHP 7.4 or later and the cURL
extension enabled. You can verify this by running the following command:
php -m | grep curl
If cURL
is not listed, enable it in your php.ini
file and restart your server.
Writing PHP Code to Retrieve Users from Airtable
With your environment set up, you can now write the PHP code to make a GET request to the Airtable API. This example demonstrates how to retrieve users by email or ID.
// Define the API endpoint and access token
$api_url = 'https://api.airtable.com/v0/meta/enterpriseAccounts/{enterpriseAccountId}/users';
$access_token = 'YOUR_ACCESS_TOKEN';
// Set the query parameters
$query_params = http_build_query([
'email[]' => 'example@domain.com',
'id[]' => 'usr12345',
'include[]' => 'collaborations'
]);
// Initialize cURL session
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $api_url . '?' . $query_params);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $access_token
]);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request and handle the response
$response = curl_exec($ch);
curl_close($ch);
// Decode the JSON response
$user_data = json_decode($response, true);
// Output user information
foreach ($user_data['users'] as $user) {
echo 'Name: ' . $user['name'] . "\n";
echo 'Email: ' . $user['email'] . "\n";
echo 'State: ' . $user['state'] . "\n";
}
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth setup. Adjust the query parameters to match the user IDs or emails you wish to retrieve.
Handling API Responses and Errors
After executing the API call, it's crucial to handle the response correctly. Check for errors and ensure the request was successful:
- Verify the HTTP status code. A 200 status indicates success.
- Handle common error codes such as 401 (Unauthorized) and 429 (Too Many Requests). Refer to the Airtable error documentation for detailed information.
If you encounter a 429 error, note that Airtable's API is limited to 5 requests per second per base. Implement a retry mechanism with exponential backoff to handle rate limits effectively. More details can be found in the Airtable rate limits documentation.
Verifying API Call Success in Airtable
To confirm the API call's success, check the returned user data against your Airtable account. Ensure the retrieved information matches the expected user details. This verification step helps ensure your integration is functioning correctly.
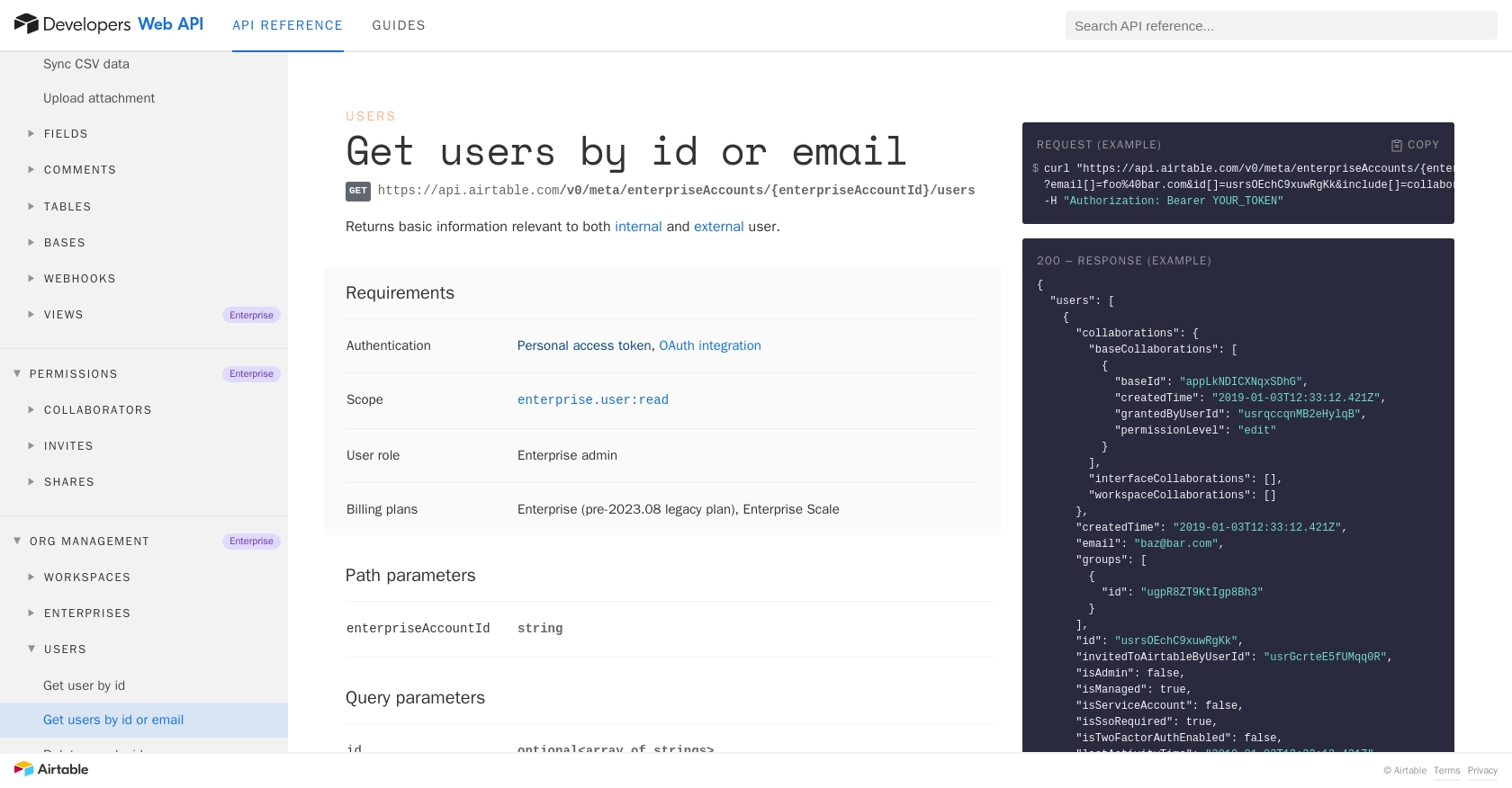
Best Practices for Airtable API Integration Using PHP
When integrating with the Airtable API using PHP, it's essential to follow best practices to ensure security, efficiency, and maintainability. Here are some key recommendations:
- Securely Store Access Tokens: Always store access tokens securely, such as in environment variables or a secure vault, to prevent unauthorized access.
- Implement Rate Limiting: Be mindful of Airtable's rate limits, which are 5 requests per second per base. Implement a retry mechanism with exponential backoff to handle rate limits gracefully. For more details, refer to the Airtable rate limits documentation.
- Handle Errors Gracefully: Ensure your application can handle common API errors, such as 401 Unauthorized and 429 Too Many Requests. Detailed error handling can be found in the Airtable error documentation.
- Data Transformation and Standardization: Consider transforming and standardizing data fields to maintain consistency across different systems and applications.
Streamlining Your Integration Process with Endgrate
Building and maintaining integrations can be time-consuming and complex, especially when dealing with multiple platforms. Endgrate offers a solution by providing a unified API endpoint that connects to various platforms, including Airtable.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product by outsourcing integrations to Endgrate, allowing you to build once for each use case instead of multiple times for different integrations.
- Enhance User Experience: Provide an easy and intuitive integration experience for your customers, simplifying their interaction with multiple platforms.
- Expand Integration Capabilities: Take advantage of Endgrate's constantly expanding support for new platforms and services, ensuring your integrations remain up-to-date and comprehensive.
To learn more about how Endgrate can streamline your integration process, visit Endgrate's website.
Read More
- https://endgrate.com/provider/airtable
- https://airtable.com/developers/web/api/authentication
- https://airtable.com/developers/web/api/scopes
- https://airtable.com/developers/web/api/oauth-reference
- https://airtable.com/developers/web/api/errors
- https://airtable.com/developers/web/api/rate-limits
- https://airtable.com/developers/web/api/get-users-by-id-or-email
Ready to get started?