Using the Todoist API to Get Tasks in PHP
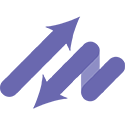
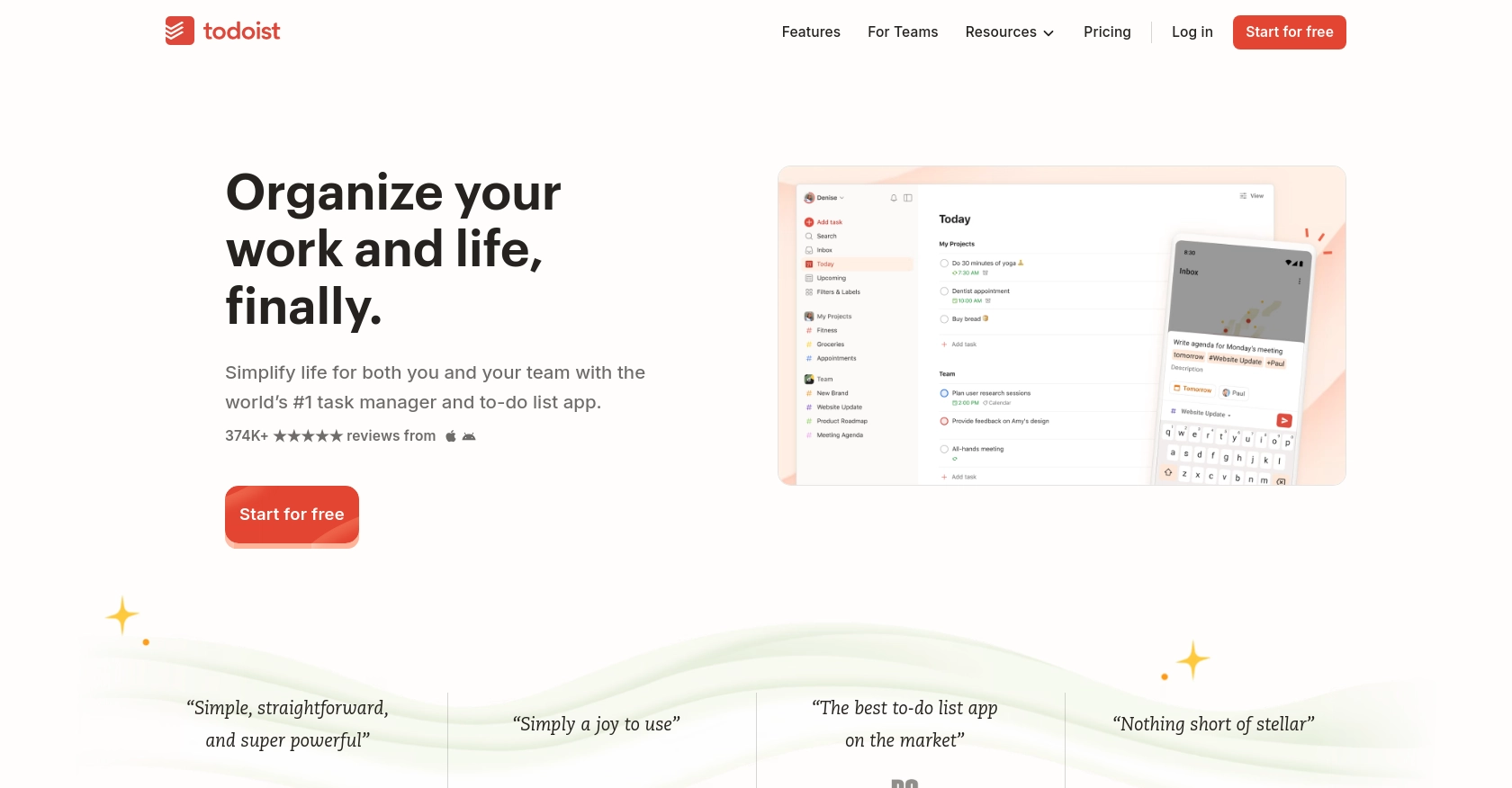
Introduction to Todoist API Integration
Todoist is a popular task management platform that helps individuals and teams organize, plan, and collaborate on projects. With its intuitive interface and robust features, Todoist is a go-to solution for managing tasks efficiently across various devices.
Integrating with the Todoist API allows developers to automate and enhance task management processes. For example, you can use the Todoist API to retrieve tasks and display them in a custom dashboard, enabling users to track their progress seamlessly.
This article will guide you through using PHP to interact with the Todoist API, specifically focusing on retrieving tasks. By the end of this tutorial, you'll be equipped to integrate Todoist's task management capabilities into your applications, streamlining workflows and boosting productivity.
Setting Up Your Todoist Developer Account for API Access
Before you can start interacting with the Todoist API using PHP, you'll need to set up a developer account and obtain the necessary credentials. This process involves creating a Todoist app and generating an OAuth token for authentication.
Creating a Todoist Developer Account
If you don't already have a Todoist account, you'll need to create one. Visit the Todoist website and sign up for a free account. Once your account is set up, you can proceed to the developer portal.
Accessing the Todoist Developer Portal
Navigate to the Todoist Developer Portal and log in using your Todoist account credentials. This portal provides access to the tools and resources needed to create and manage your Todoist app.
Creating a Todoist App for OAuth Authentication
To interact with the Todoist API, you'll need to create an app within the developer portal. Follow these steps:
- Click on "Create a new app" in the developer portal.
- Fill in the required details such as the app name, description, and redirect URI.
- Submit the form to create your app.
Once your app is created, you'll receive a client ID and client secret. These credentials are essential for OAuth authentication.
Generating an OAuth Token
To authenticate API requests, you'll need an OAuth token. Follow these steps to generate one:
- Direct users to the Todoist authorization URL, including your client ID and redirect URI.
- Upon user consent, Todoist will redirect to your specified URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to the Todoist token endpoint, including your client ID, client secret, and authorization code.
Store the access token securely, as it will be used to authenticate API requests.
For more detailed information on OAuth authentication with Todoist, refer to the Todoist API documentation.
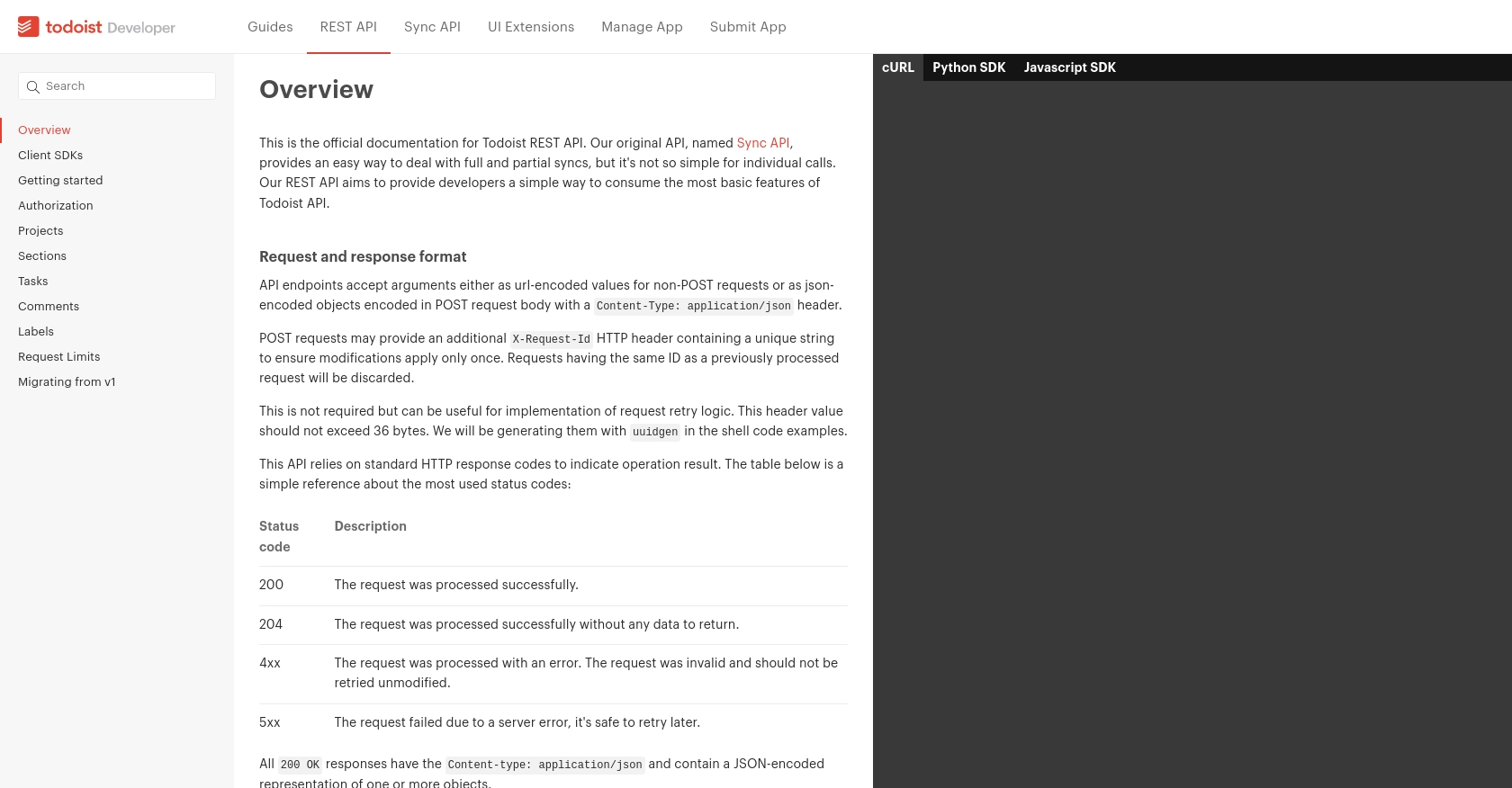
sbb-itb-96038d7
Making API Calls to Retrieve Tasks from Todoist Using PHP
To interact with the Todoist API using PHP, you'll need to set up your environment and write code to make API requests. This section will guide you through the process of retrieving tasks from Todoist, ensuring you have the necessary tools and understanding to integrate Todoist's task management features into your PHP applications.
Setting Up Your PHP Environment for Todoist API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need PHP version 7.4 or higher and the cURL extension enabled. You can check your PHP version and installed extensions by running the following command:
php -v
php -m | grep curl
If cURL is not enabled, you can enable it by modifying your php.ini
file and restarting your web server.
Installing Dependencies for Todoist API Requests
To make HTTP requests in PHP, you can use the cURL library. Ensure it's installed and enabled in your PHP environment. Alternatively, you can use a library like Guzzle for more advanced HTTP client features. Install Guzzle using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Tasks from Todoist
With your environment set up, you can now write PHP code to retrieve tasks from Todoist. The following example demonstrates how to make a GET request to the Todoist API to fetch active tasks:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'your_access_token_here'; // Replace with your OAuth token
try {
$response = $client->request('GET', 'https://api.todoist.com/rest/v2/tasks', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json',
],
]);
$tasks = json_decode($response->getBody(), true);
foreach ($tasks as $task) {
echo 'Task: ' . $task['content'] . '<br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace your_access_token_here
with your actual OAuth token. This code initializes a Guzzle HTTP client, sets the necessary headers for authentication, and makes a GET request to the Todoist API to retrieve tasks. The response is parsed and displayed.
Handling API Response and Errors
After making the API call, it's crucial to handle the response and potential errors. The Todoist API returns a JSON-encoded array of tasks with a 200 OK status on success. If an error occurs, the API will return a 4xx or 5xx status code. Ensure your code handles these scenarios gracefully, logging errors and providing user feedback as needed.
For more information on handling errors and response codes, refer to the Todoist API documentation.
Conclusion and Best Practices for Integrating Todoist API with PHP
Integrating the Todoist API with PHP allows developers to enhance their applications by leveraging Todoist's powerful task management capabilities. By following the steps outlined in this guide, you can efficiently retrieve tasks and incorporate them into your custom solutions, improving productivity and workflow management.
Best Practices for Secure and Efficient Todoist API Integration
- Securely Store OAuth Tokens: Ensure that OAuth tokens are stored securely, using environment variables or secure storage solutions, to prevent unauthorized access.
- Handle Rate Limiting: The Todoist API allows a maximum of 1000 requests per 15-minute period. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay.
- Implement Error Handling: Always check for error codes and handle them appropriately. Log errors for debugging and provide user-friendly messages to enhance the user experience.
- Optimize Data Processing: When retrieving large datasets, consider implementing pagination or filtering to reduce the amount of data processed at once.
Streamline Your Integrations with Endgrate
While integrating with the Todoist API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Todoist. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration experience.
Read More
Ready to get started?