Using the Salesloft API to Get Accounts in Javascript
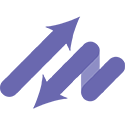
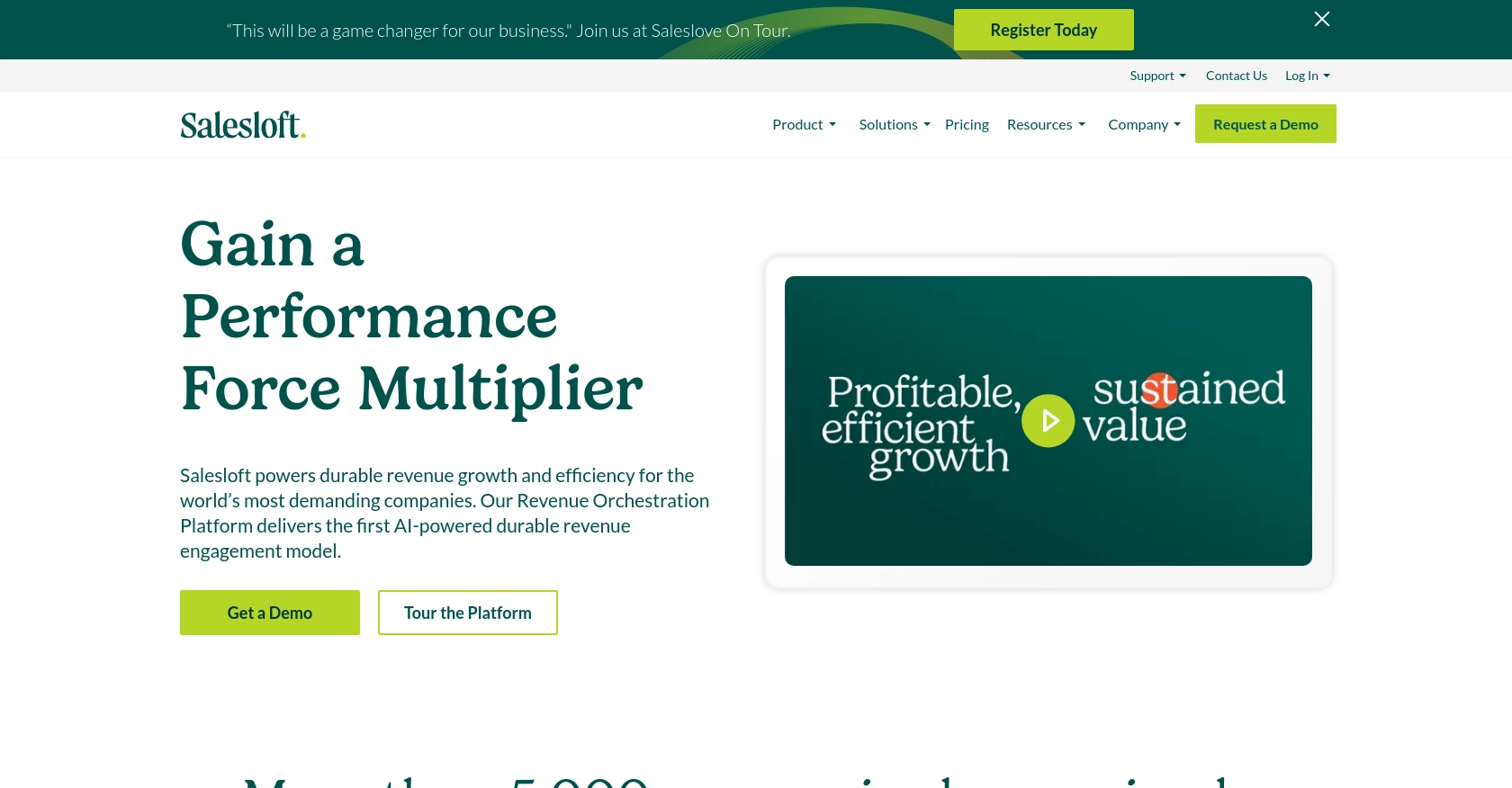
Introduction to Salesloft API Integration
Salesloft is a powerful sales engagement platform that enables sales teams to connect with prospects more effectively. With its comprehensive suite of tools, Salesloft helps businesses streamline their sales processes, improve communication, and drive revenue growth.
Integrating with the Salesloft API allows developers to access and manage sales data programmatically, enhancing the ability to automate workflows and improve efficiency. For example, developers can use the Salesloft API to retrieve account information, enabling seamless integration with CRM systems and other sales tools.
This article will guide you through using JavaScript to interact with the Salesloft API, specifically focusing on retrieving account data. By following this tutorial, you'll learn how to set up authentication, make API calls, and handle responses effectively.
Setting Up Your Salesloft Test Account for API Integration
Before you can start interacting with the Salesloft API using JavaScript, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Follow these steps to create a sandbox environment and obtain the necessary credentials for OAuth authentication.
Creating a Salesloft Sandbox Account
If you don't already have a Salesloft account, you can sign up for a free trial or demo account on the Salesloft website. This will provide you with access to the platform's features and allow you to test API interactions.
- Visit the Salesloft website and navigate to the sign-up page.
- Fill out the required information to create your account.
- Once your account is created, log in to access the Salesloft dashboard.
Setting Up OAuth Authentication for Salesloft API
Salesloft uses OAuth 2.0 for authentication, which requires you to create an application within your Salesloft account. This will provide you with a client ID and client secret needed for API access.
- Log in to your Salesloft account and navigate to Your Applications under the account settings.
- Select OAuth Applications and click on Create New.
- Fill in the necessary fields, such as application name and redirect URI, then click Save.
- After saving, you will see your Application ID (Client ID) and Secret (Client Secret). Make sure to store these securely as they will be used in the authorization process.
Obtaining Authorization Code and Access Tokens
To interact with the Salesloft API, you'll need to obtain an access token. Follow these steps to complete the OAuth flow:
- Generate a request to the authorization endpoint using your Client ID and Redirect URI:
- Authorize the application when prompted. Upon approval, you'll receive an authorization code.
- Exchange the authorization code for an access token by making a POST request:
- Store the access token securely, as it will be used to authenticate your API requests.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token
{
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"code": "AUTHORIZATION_CODE",
"grant_type": "authorization_code",
"redirect_uri": "YOUR_REDIRECT_URI"
}
With your Salesloft test account and OAuth credentials set up, you're ready to start making API calls to retrieve account data using JavaScript.
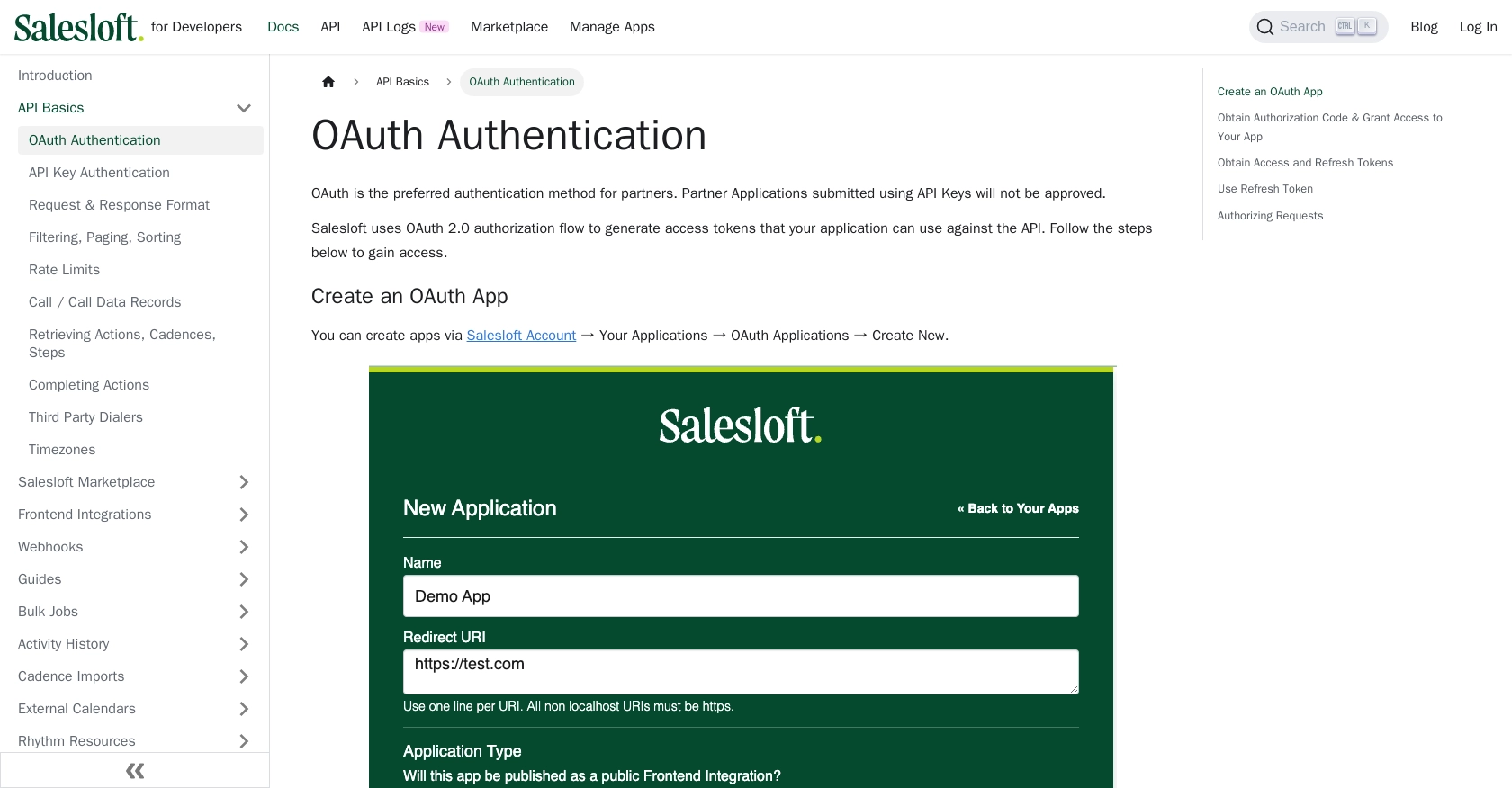
sbb-itb-96038d7
Making API Calls to Retrieve Salesloft Accounts Using JavaScript
Now that you have set up your Salesloft test account and obtained the necessary OAuth credentials, it's time to make API calls to retrieve account data using JavaScript. This section will guide you through the process of setting up your development environment, writing the code to interact with the Salesloft API, and handling the responses effectively.
Setting Up Your JavaScript Environment
To interact with the Salesloft API, you'll need a JavaScript environment that supports HTTP requests. You can use Node.js for server-side scripting or any modern browser for client-side scripting. Ensure you have Node.js installed if you choose to work server-side.
Additionally, you'll need to install the Axios library, which simplifies making HTTP requests. You can install it using npm:
npm install axios
Writing JavaScript Code to Fetch Salesloft Accounts
With your environment set up, you can now write the JavaScript code to fetch account data from Salesloft. Create a new JavaScript file, for example, getSalesloftAccounts.js
, and add the following code:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://api.salesloft.com/v2/accounts';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Accept': 'application/json'
};
// Function to fetch accounts
async function fetchAccounts() {
try {
const response = await axios.get(endpoint, { headers });
const accounts = response.data.data;
// Log account information
accounts.forEach(account => {
console.log(`Name: ${account.name}, Domain: ${account.domain}`);
});
} catch (error) {
console.error('Error fetching accounts:', error.response ? error.response.data : error.message);
}
}
// Execute the function
fetchAccounts();
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth setup.
Understanding the Response and Handling Errors
When you run the script, it will make a GET request to the Salesloft API to retrieve account data. The response will include a list of accounts, which you can iterate over to access individual account details such as name and domain.
To verify that the request succeeded, check the console output for the account information. If there are any errors, the catch block will log the error details. Common error codes include:
- 403 Forbidden: Indicates that the access token is invalid or expired.
- 404 Not Found: The requested resource does not exist.
- 422 Unprocessable Entity: Indicates issues with the request parameters.
For more detailed error handling, refer to the Salesloft API documentation.
Optimizing API Requests and Managing Rate Limits
Salesloft imposes a rate limit of 600 requests per minute. To avoid exceeding this limit, consider implementing strategies such as caching responses or batching requests. Monitor the x-ratelimit-remaining-minute
header in the response to track your usage.
For more information on rate limits, visit the Salesloft API rate limits documentation.
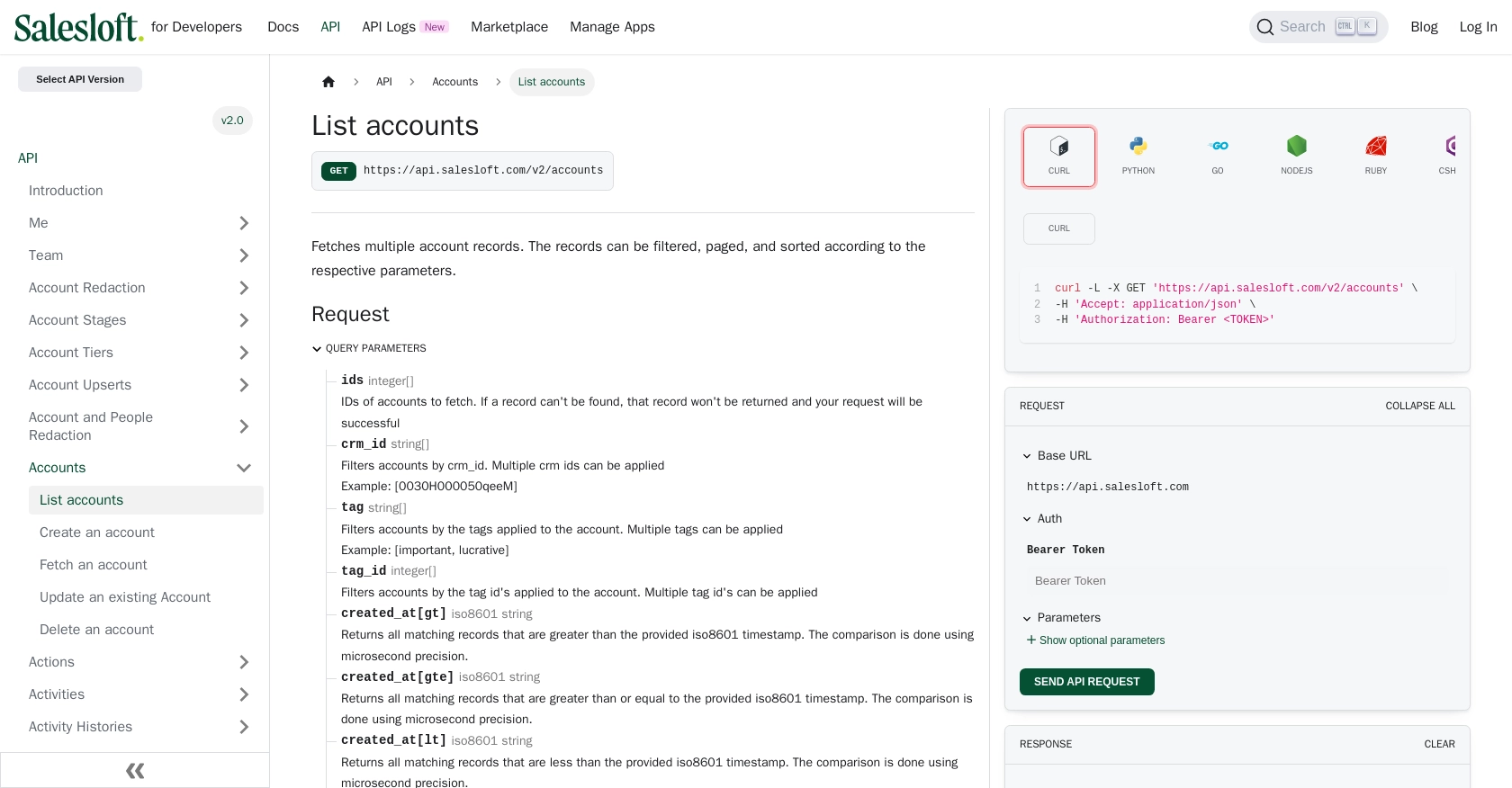
Conclusion and Best Practices for Salesloft API Integration
Integrating with the Salesloft API using JavaScript provides a powerful way to automate and enhance your sales processes. By following the steps outlined in this guide, you can efficiently retrieve account data and integrate it with your existing systems.
Best Practices for Secure and Efficient Salesloft API Usage
- Securely Store Credentials: Always store your OAuth credentials, such as client ID, client secret, and access tokens, securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limits: Be mindful of the Salesloft API rate limit of 600 requests per minute. Implement strategies like caching and batching to optimize your API usage and avoid hitting the limit. Monitor the
x-ratelimit-remaining-minute
header to track your request count. - Error Handling: Implement robust error handling to manage common issues such as expired tokens or invalid requests. Use the error codes provided by the API to diagnose and resolve issues quickly.
- Data Standardization: Ensure that the data retrieved from Salesloft is standardized and transformed as needed to fit your application's requirements. This will help maintain consistency across different systems.
Streamlining Integrations with Endgrate
While building integrations with Salesloft and other platforms can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Salesloft. This allows you to focus on your core product while outsourcing the integration work.
With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can save you time and resources by visiting Endgrate.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/accounts-index/
Ready to get started?