Using the Quickbooks API to Get Invoices in PHP
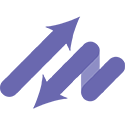
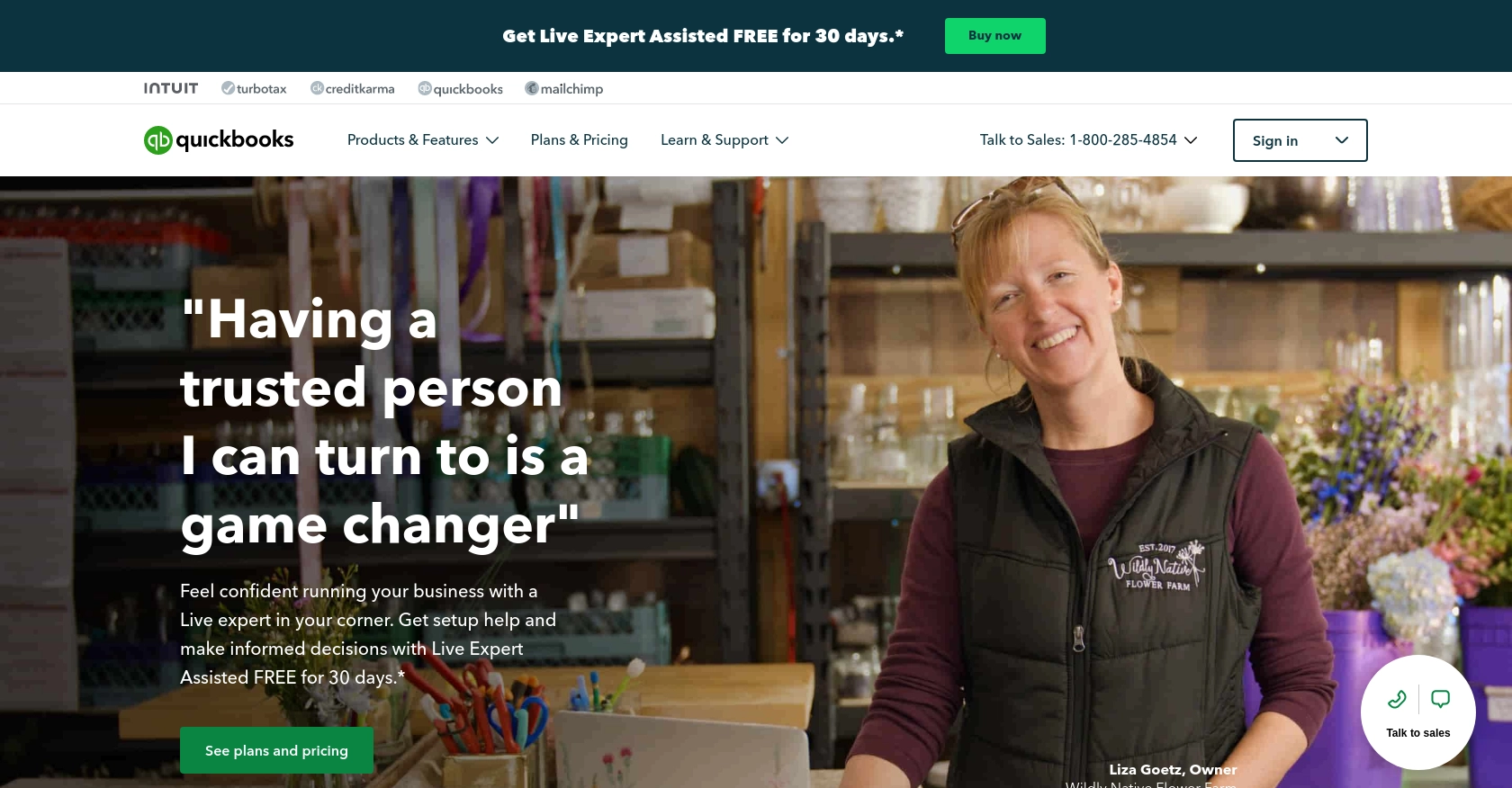
Introduction to QuickBooks API Integration
QuickBooks is a widely-used accounting software that offers a comprehensive suite of tools for managing business finances. It is particularly popular among small to medium-sized businesses due to its user-friendly interface and robust features, including invoicing, expense tracking, and financial reporting.
For developers, integrating with the QuickBooks API can unlock powerful capabilities to automate and streamline financial processes. By connecting with QuickBooks, developers can access and manage financial data programmatically, enabling seamless synchronization between QuickBooks and other business applications.
A common use case for the QuickBooks API is retrieving invoices. For example, a developer might want to pull invoice data from QuickBooks to generate custom financial reports or to integrate with a third-party billing system. This article will guide you through the process of using PHP to interact with the QuickBooks API to fetch invoice details.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start interacting with the QuickBooks API, you need to set up a sandbox account. This environment allows you to test your application without affecting live data, providing a safe space to experiment with API calls.
Creating a QuickBooks Developer Account
To begin, you'll need a QuickBooks Developer account. Follow these steps to create one:
- Visit the Intuit Developer Portal.
- Click on "Sign Up" and fill in the required information to create your account.
- Once registered, log in to your developer account.
Setting Up a QuickBooks Sandbox Company
After creating your developer account, set up a sandbox company to test your API interactions:
- Navigate to the "Sandbox" section in your developer dashboard.
- Click on "Add Sandbox" to create a new sandbox company.
- Choose the QuickBooks Online product and complete the setup process.
Creating an App for OAuth Authentication
QuickBooks API uses OAuth 2.0 for authentication. You'll need to create an app to obtain the necessary credentials:
- Go to the "My Apps" section in your developer dashboard.
- Click on "Create an App" and select "QuickBooks Online and Payments."
- Fill in the app details and save your app.
Obtaining Client ID and Client Secret
Once your app is created, you'll need the client ID and client secret for authentication:
- In your app settings, navigate to the "Keys & OAuth" section.
- Copy the client ID and client secret provided.
- Store these credentials securely, as you'll need them to authenticate API requests.
For more detailed instructions, refer to the Intuit Developer Documentation.
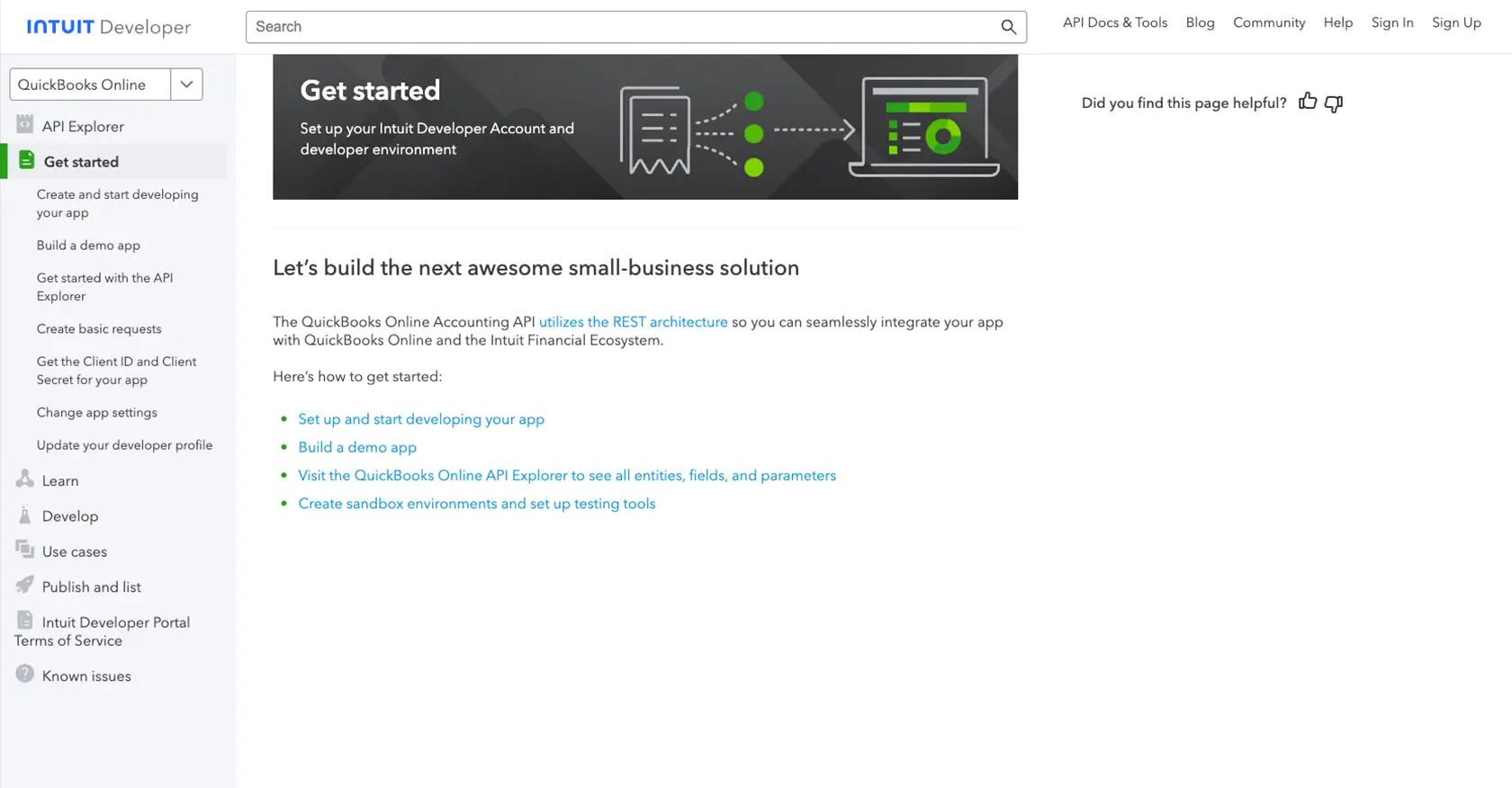
sbb-itb-96038d7
Making API Calls to Retrieve QuickBooks Invoices Using PHP
To interact with the QuickBooks API and retrieve invoice data using PHP, you'll need to set up your development environment and write the necessary code to authenticate and make API requests. This section will guide you through these steps, ensuring you can efficiently access invoice information from QuickBooks.
Setting Up Your PHP Environment for QuickBooks API Integration
Before making API calls, ensure your PHP environment is correctly configured. You'll need the following:
- PHP 7.4 or later installed on your machine.
- Composer, the PHP package manager, to manage dependencies.
To install Composer, follow the instructions on the Composer website.
Installing Required PHP Libraries for QuickBooks API
You'll need the guzzlehttp/guzzle
library to handle HTTP requests. Install it using Composer:
composer require guzzlehttp/guzzle
Writing PHP Code to Authenticate and Fetch Invoices from QuickBooks
With your environment set up, you can now write the PHP code to authenticate and retrieve invoices. Here's a step-by-step guide:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Set up the client with the base URI
$client = new Client(['base_uri' => 'https://quickbooks.api.intuit.com/']);
// Define your OAuth 2.0 credentials
$clientId = 'Your_Client_ID';
$clientSecret = 'Your_Client_Secret';
$accessToken = 'Your_Access_Token';
// Set the headers for the request
$headers = [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
'Content-Type' => 'application/json'
];
// Make a GET request to the QuickBooks API to fetch invoices
$response = $client->request('GET', 'v3/company/Your_Company_ID/query', [
'headers' => $headers,
'query' => [
'query' => 'SELECT * FROM Invoice'
]
]);
// Parse the JSON response
$data = json_decode($response->getBody(), true);
// Output the invoice data
foreach ($data['QueryResponse']['Invoice'] as $invoice) {
echo 'Invoice ID: ' . $invoice['Id'] . "\n";
echo 'Total Amount: ' . $invoice['TotalAmt'] . "\n";
echo 'Customer: ' . $invoice['CustomerRef']['name'] . "\n\n";
}
Replace Your_Client_ID
, Your_Client_Secret
, Your_Access_Token
, and Your_Company_ID
with your actual credentials and company ID from QuickBooks.
Verifying API Request Success and Handling Errors
After running the script, you should see a list of invoices printed in your terminal. To verify the request's success, check the response status code:
if ($response->getStatusCode() == 200) {
echo "Request was successful.\n";
} else {
echo "Failed to retrieve invoices. Status code: " . $response->getStatusCode() . "\n";
}
Handle potential errors by catching exceptions and checking error codes. Refer to the Intuit Developer Documentation for detailed error code information.
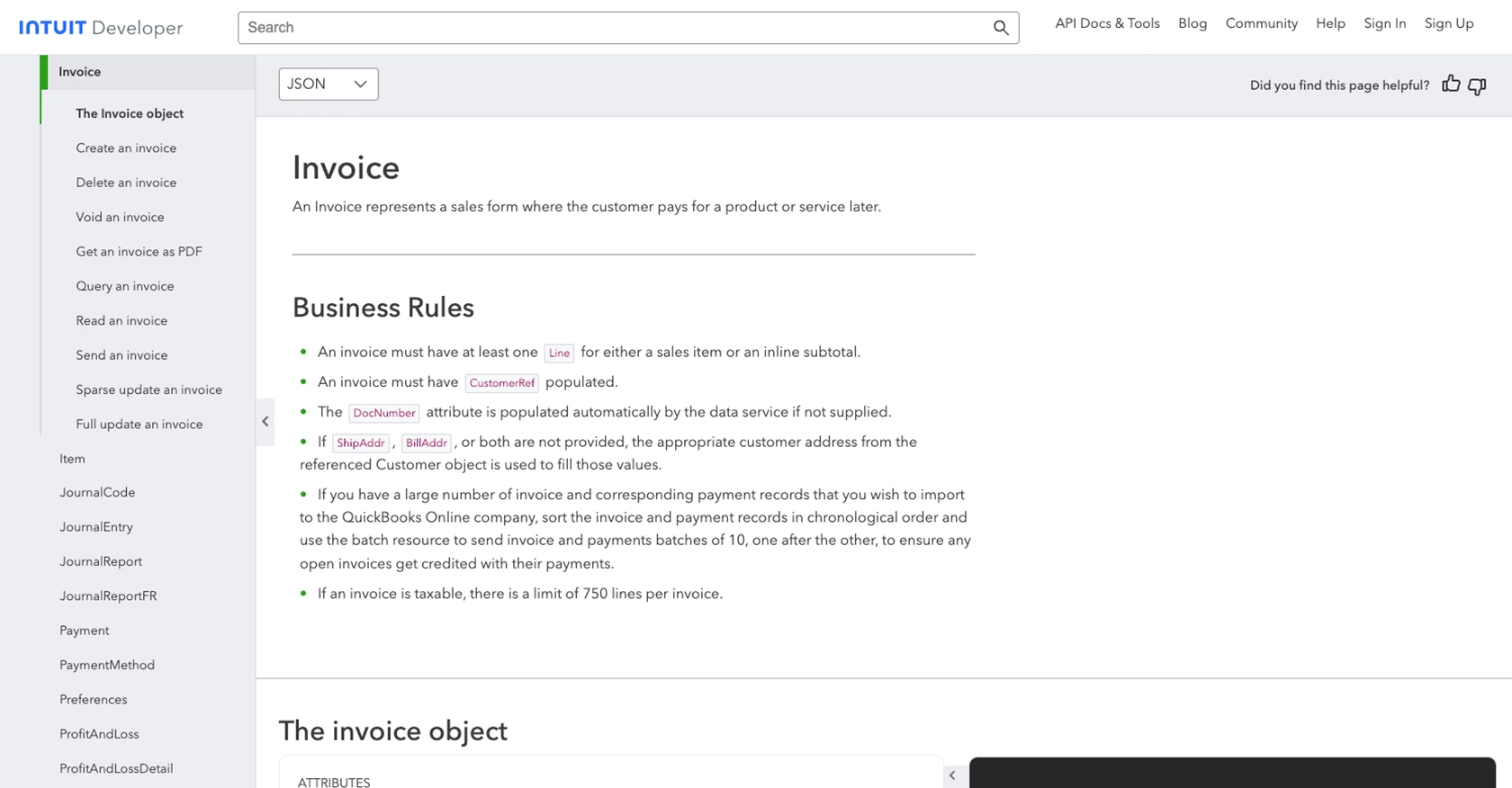
Conclusion and Best Practices for QuickBooks API Integration with PHP
Integrating with the QuickBooks API using PHP allows developers to automate financial processes and seamlessly access invoice data. By following the steps outlined in this guide, you can efficiently retrieve invoices and integrate them into your applications.
Best Practices for Secure and Efficient QuickBooks API Usage
- Secure Storage of Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: QuickBooks API has rate limits. Ensure your application handles these limits gracefully by implementing retry logic and monitoring API usage. Refer to the Intuit Developer Documentation for specific rate limit details.
- Data Transformation and Standardization: When integrating QuickBooks data with other systems, ensure that data fields are transformed and standardized to maintain consistency across platforms.
Streamline Your Integration Process with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including QuickBooks. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Offer an easy, intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/invoice
Ready to get started?