Using the Quickbooks API to Get Purchase Orders (with Javascript examples)
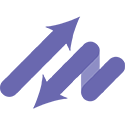
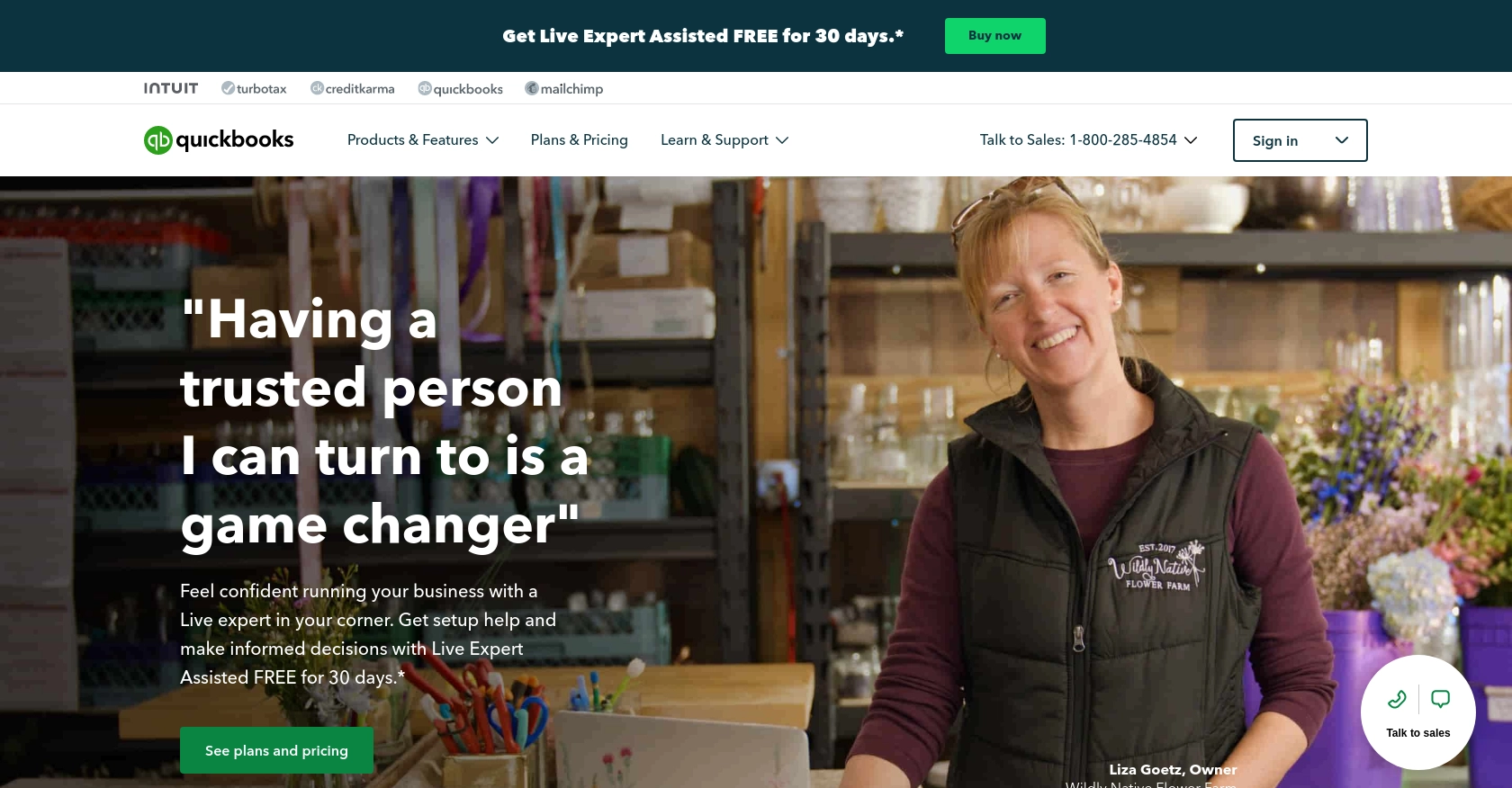
Introduction to QuickBooks API
QuickBooks is a widely-used accounting software that helps businesses manage their finances efficiently. It offers a range of features including invoicing, expense tracking, payroll management, and financial reporting. QuickBooks is especially popular among small to medium-sized businesses for its user-friendly interface and robust functionality.
Developers may want to integrate with QuickBooks to streamline financial operations and automate accounting tasks. For example, using the QuickBooks API, a developer can retrieve purchase orders to analyze spending patterns or synchronize data with other business systems. This integration can enhance productivity by reducing manual data entry and providing real-time financial insights.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before diving into the QuickBooks API to retrieve purchase orders, you need to set up a sandbox account. This environment allows you to test your integration without affecting live data, providing a safe space to experiment and develop your application.
Creating a QuickBooks Developer Account
To begin, you'll need a QuickBooks Developer account. Follow these steps to create one:
- Visit the QuickBooks Developer Portal.
- Click on "Sign Up" and fill in the required details to create your account.
- Once registered, log in to your QuickBooks Developer account.
Setting Up a QuickBooks Sandbox Company
With your developer account ready, the next step is to set up a sandbox company:
- Navigate to the "Dashboard" in your QuickBooks Developer account.
- Select "Sandbox" from the menu and click on "Add Sandbox Company."
- Follow the prompts to create a new sandbox company. This will provide you with a test environment to work with.
Creating a QuickBooks App for OAuth Authentication
The QuickBooks API uses OAuth 2.0 for authentication. To interact with the API, you'll need to create an app to obtain your client ID and client secret:
- Go to the App Management section of your QuickBooks Developer account.
- Click on "Create an App" and choose "QuickBooks Online and Payments."
- Fill in the necessary details such as app name and description.
- Once the app is created, navigate to the "Keys & OAuth" section to find your client ID and client secret.
Configuring OAuth Scopes and Redirect URIs
To ensure your app has the correct permissions, configure the OAuth scopes:
- In the app settings, go to the "Scopes" section.
- Select the necessary scopes for accessing purchase orders, such as "com.intuit.quickbooks.accounting."
- Set up your redirect URIs to handle OAuth callbacks.
With your sandbox account and app set up, you're ready to start making API calls to QuickBooks. This setup ensures you have a secure and isolated environment to test your integration.
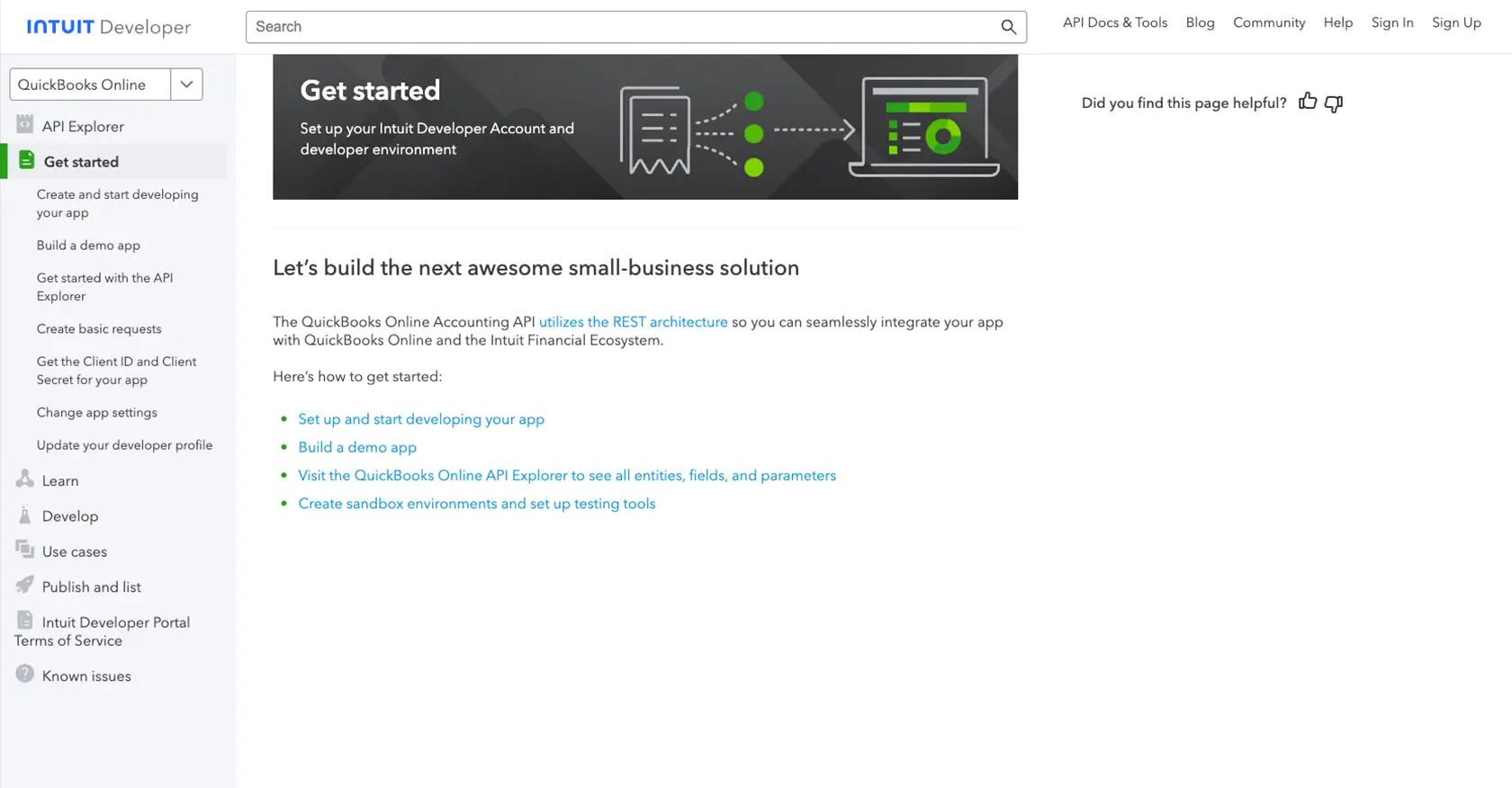
sbb-itb-96038d7
Making API Calls to Retrieve QuickBooks Purchase Orders Using JavaScript
With your QuickBooks sandbox and app set up, you can now proceed to make API calls to retrieve purchase orders. This section will guide you through the process using JavaScript, ensuring you have the necessary tools and knowledge to interact with the QuickBooks API effectively.
JavaScript Environment Setup for QuickBooks API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A package manager like npm (comes with Node.js) to manage dependencies.
Once you have Node.js and npm installed, create a new project directory and initialize it:
mkdir quickbooks-api-integration
cd quickbooks-api-integration
npm init -y
Installing Required Dependencies for QuickBooks API Calls
To interact with the QuickBooks API, you'll need the axios
library for making HTTP requests and dotenv
to manage environment variables:
npm install axios dotenv
Writing JavaScript Code to Fetch QuickBooks Purchase Orders
Create a file named getPurchaseOrders.js
and add the following code:
require('dotenv').config();
const axios = require('axios');
// QuickBooks API endpoint for purchase orders
const endpoint = 'https://sandbox-quickbooks.api.intuit.com/v3/company/YOUR_COMPANY_ID/purchaseorder';
// Set up headers with OAuth token
const headers = {
'Authorization': `Bearer ${process.env.ACCESS_TOKEN}`,
'Accept': 'application/json',
'Content-Type': 'application/json'
};
// Function to get purchase orders
async function getPurchaseOrders() {
try {
const response = await axios.get(endpoint, { headers });
console.log('Purchase Orders:', response.data);
} catch (error) {
console.error('Error fetching purchase orders:', error.response ? error.response.data : error.message);
}
}
getPurchaseOrders();
Replace YOUR_COMPANY_ID
with your actual QuickBooks company ID and ensure your .env
file contains the ACCESS_TOKEN
obtained during OAuth authentication.
Running the JavaScript Code to Retrieve Purchase Orders
To execute the code, run the following command in your terminal:
node getPurchaseOrders.js
If successful, you should see the purchase orders from your QuickBooks sandbox environment printed in the console. Verify the data by checking your sandbox account to ensure it matches the retrieved information.
Handling Errors and QuickBooks API Response Codes
When making API calls, it's crucial to handle potential errors. The QuickBooks API may return various HTTP status codes indicating the success or failure of your request:
- 200 OK: The request was successful, and the purchase orders are returned.
- 401 Unauthorized: Authentication failed. Check your OAuth token.
- 403 Forbidden: You don't have permission to access the resource.
- 404 Not Found: The requested resource doesn't exist.
- 429 Too Many Requests: You've hit the rate limit. Try again later.
For more detailed error handling, refer to the QuickBooks API documentation.
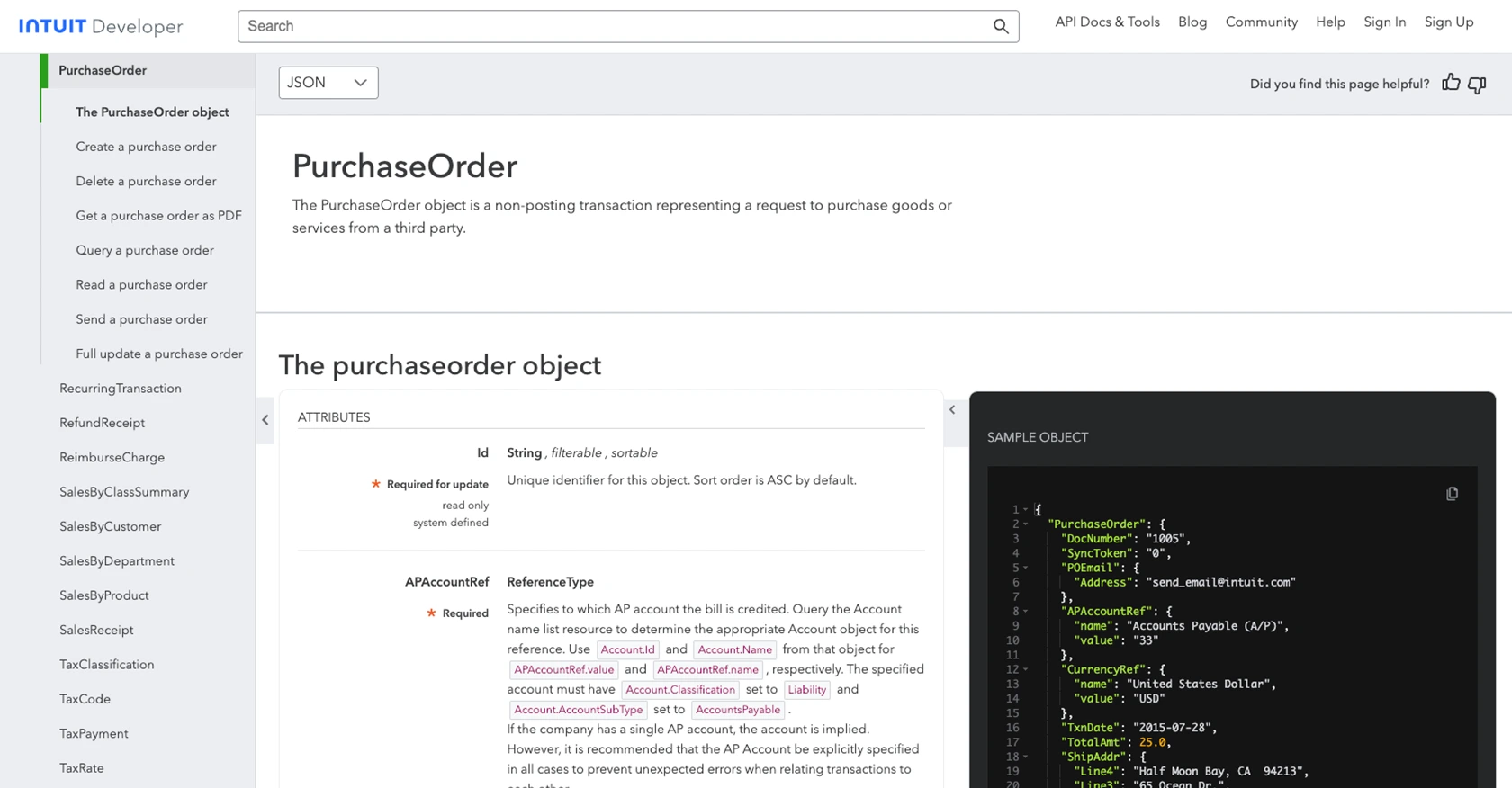
Conclusion and Best Practices for QuickBooks API Integration
Integrating with the QuickBooks API to retrieve purchase orders using JavaScript can significantly enhance your business's financial operations. By automating data retrieval and synchronization, you can reduce manual entry, minimize errors, and gain real-time insights into your financial data.
Best Practices for Secure and Efficient QuickBooks API Usage
- Securely Store Credentials: Always store your OAuth tokens and client secrets securely, using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of QuickBooks API rate limits, such as 429 Too Many Requests. Implement retry logic with exponential backoff to handle these limits gracefully.
- Standardize Data Fields: Ensure that data retrieved from QuickBooks is transformed and standardized to fit your application's data model, enhancing data consistency across systems.
Streamlining Integrations with Endgrate
While integrating with QuickBooks can be highly beneficial, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies this process by allowing you to connect with multiple platforms through a single endpoint.
By leveraging Endgrate, you can save time and resources, focusing on your core product development while providing an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration efforts and enhance your business operations by visiting Endgrate today.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/purchaseorder
Ready to get started?