How to Get Messages with the Re:amaze API in PHP
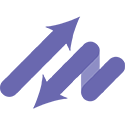
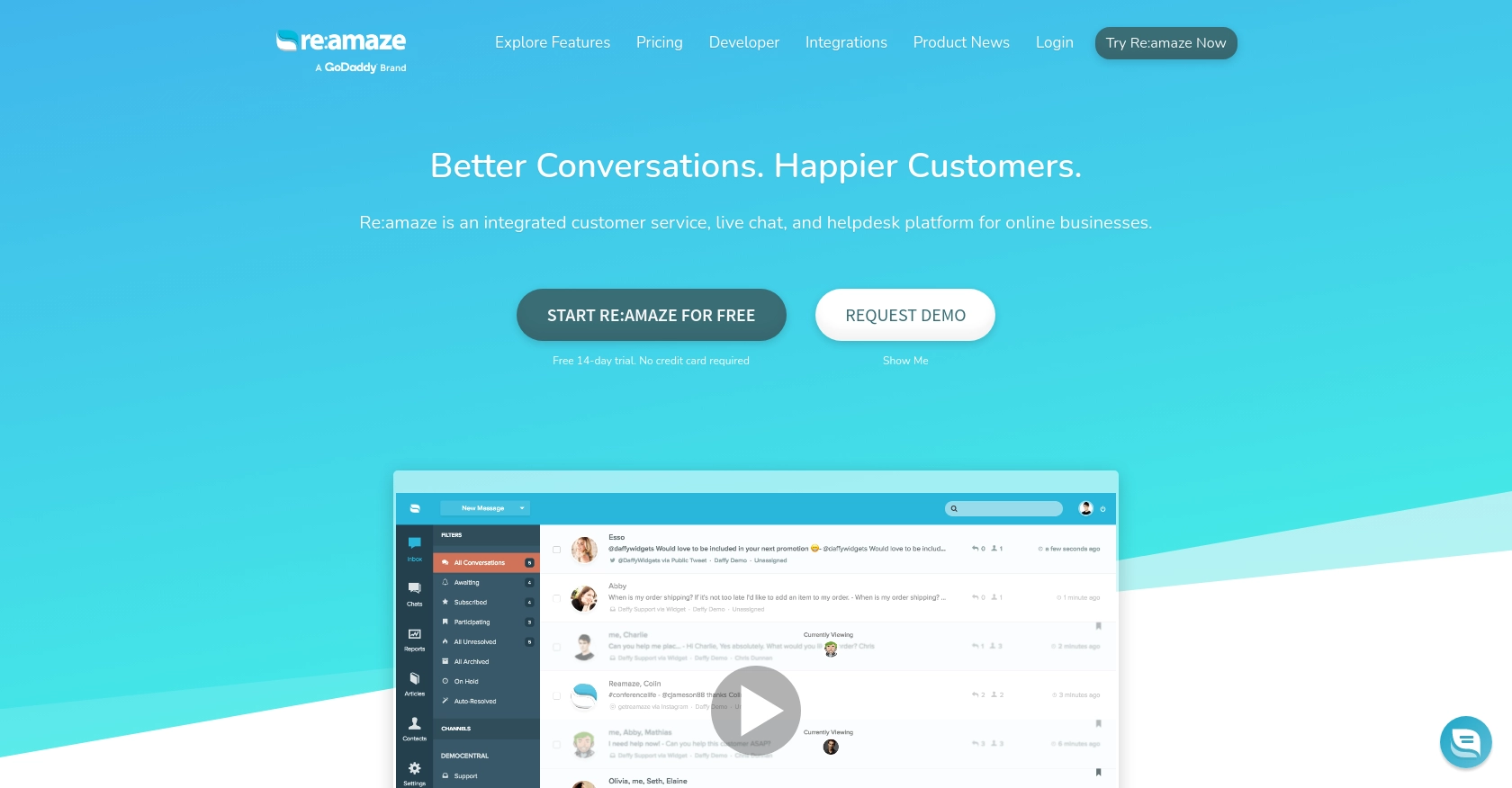
Introduction to Re:amaze API
Re:amaze is a versatile customer support platform that offers a unified inbox for managing various communication channels, including email, chat, and social media. It is designed to help businesses streamline their customer service operations, enhance engagement, and improve response times.
Developers may want to integrate with the Re:amaze API to automate and enhance customer interactions. For example, retrieving messages from Re:amaze using PHP can help developers analyze customer inquiries, automate responses, or integrate with other business systems for a seamless support experience.
Setting Up Your Re:amaze Test Account
Before you can start interacting with the Re:amaze API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Free Re:amaze Account
If you don't already have a Re:amaze account, you can sign up for a free account on the Re:amaze website. Follow the instructions to complete the registration process. Once your account is created, you will have access to the Re:amaze dashboard.
Generating an API Token for Re:amaze
To authenticate your API requests, you'll need an API token. Follow these steps to generate one:
- Log in to your Re:amaze account.
- Navigate to the Settings section.
- Under the Developer menu, click on API Token.
- Click on Generate New Token to create a unique API token for your account.
Make sure to store this token securely, as it will be used to authenticate your API requests.
Understanding Re:amaze API Authentication
The Re:amaze API uses HTTP Basic Auth for authentication. You will need to include your login email and API token in the request header. Ensure that all requests are made over HTTPS for security purposes.
curl 'https://{brand}.reamaze.io/api/v1/messages' \
-u {login-email}:{api-token} \
-H 'Accept: application/json'
Replace {brand}
, {login-email}
, and {api-token}
with your specific details.
With your test account and API token ready, you can now proceed to make API calls to retrieve messages using PHP.
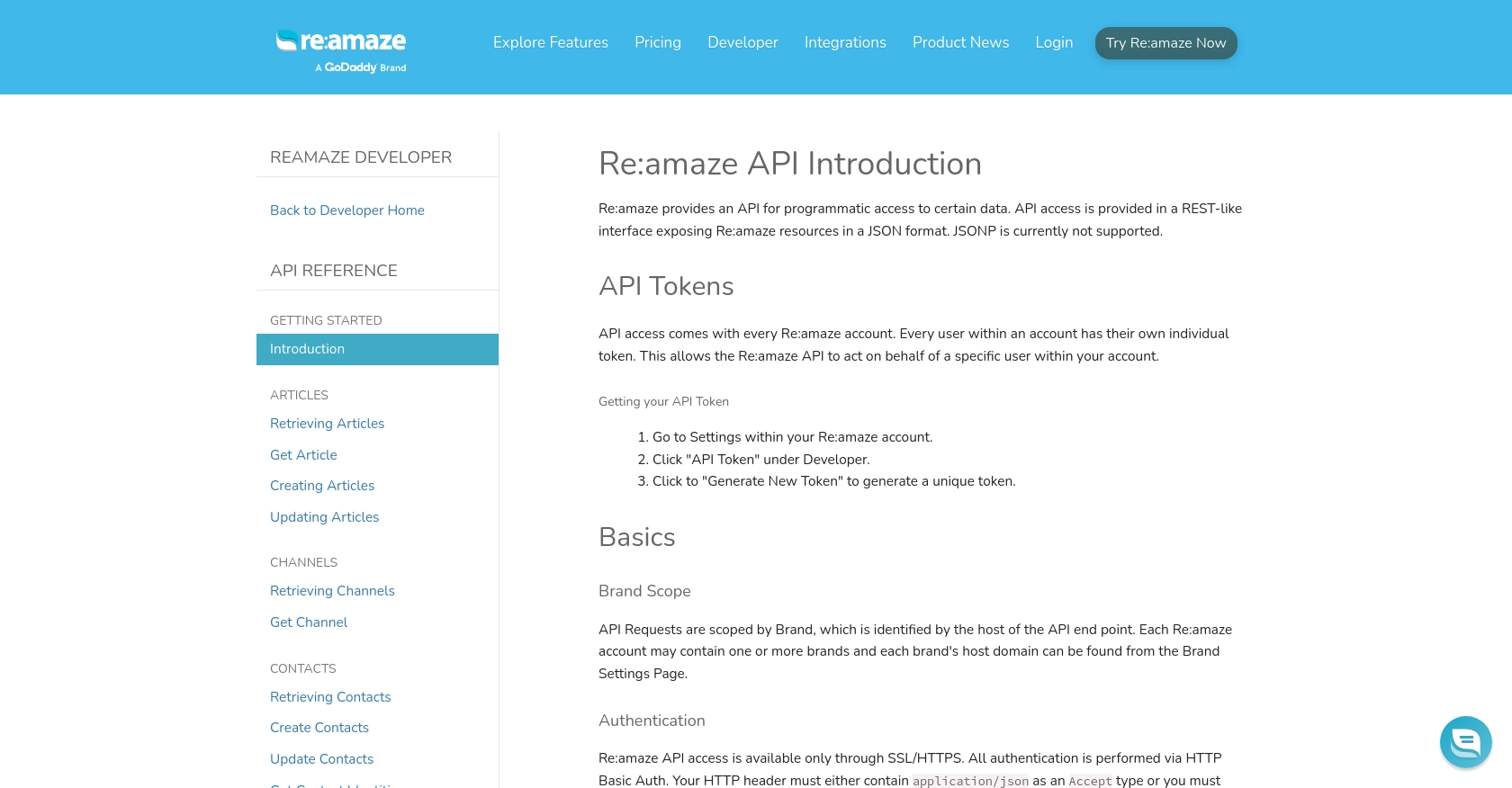
sbb-itb-96038d7
Making API Calls to Retrieve Messages from Re:amaze Using PHP
Setting Up Your PHP Environment for Re:amaze API Integration
To interact with the Re:amaze API using PHP, ensure you have PHP installed on your system. We recommend using PHP 7.4 or later for compatibility and performance improvements. Additionally, you'll need the cURL
extension enabled, which is commonly used for making HTTP requests in PHP.
Installing Necessary PHP Dependencies for Re:amaze API
Before proceeding, make sure you have the necessary PHP dependencies installed. You can use Composer, a dependency manager for PHP, to install any required packages. If you don't have Composer installed, you can download it from the official website.
Example Code to Retrieve Messages from Re:amaze API
Below is a sample PHP script to retrieve messages from the Re:amaze API. This script uses cURL to make a GET request to the API endpoint.
<?php
// Set your Re:amaze brand, login email, and API token
$brand = 'your_brand';
$loginEmail = 'your_email@example.com';
$apiToken = 'your_api_token';
// Initialize cURL session
$ch = curl_init();
// Set the API endpoint URL
$url = "https://{$brand}.reamaze.io/api/v1/messages";
// Set cURL options
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPAUTH, CURLAUTH_BASIC);
curl_setopt($ch, CURLOPT_USERPWD, "{$loginEmail}:{$apiToken}");
curl_setopt($ch, CURLOPT_HTTPHEADER, array('Accept: application/json'));
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode the JSON response
$data = json_decode($response, true);
// Display the messages
foreach ($data['messages'] as $message) {
echo "Message: " . $message['body'] . "\n";
echo "From: " . $message['user']['name'] . " (" . $message['user']['email'] . ")\n";
echo "Date: " . $message['created_at'] . "\n\n";
}
}
// Close the cURL session
curl_close($ch);
?>
Understanding the PHP Code for Re:amaze API Calls
In this script, we start by setting the necessary variables, including your Re:amaze brand, login email, and API token. We then initialize a cURL session and set the API endpoint URL. The script configures cURL options to handle HTTP Basic Auth and specify the request headers.
Upon executing the request, the script checks for any errors. If successful, it decodes the JSON response and iterates through the messages, displaying key information such as the message body, sender, and creation date.
Verifying Successful API Requests in Re:amaze
After running the script, you should see the retrieved messages printed in your terminal. To verify the request's success, you can log in to your Re:amaze dashboard and check the messages in your inbox. They should match the output from your script.
Handling Errors and Rate Limiting in Re:amaze API
If the API request fails, the script will output an error message. Common issues include incorrect credentials or network problems. Additionally, be aware of Re:amaze's rate limiting, which may restrict the number of requests per minute. If you exceed the limit, you'll receive a HTTP 429 Too Many Requests
response. For more details, refer to the Re:amaze API documentation.
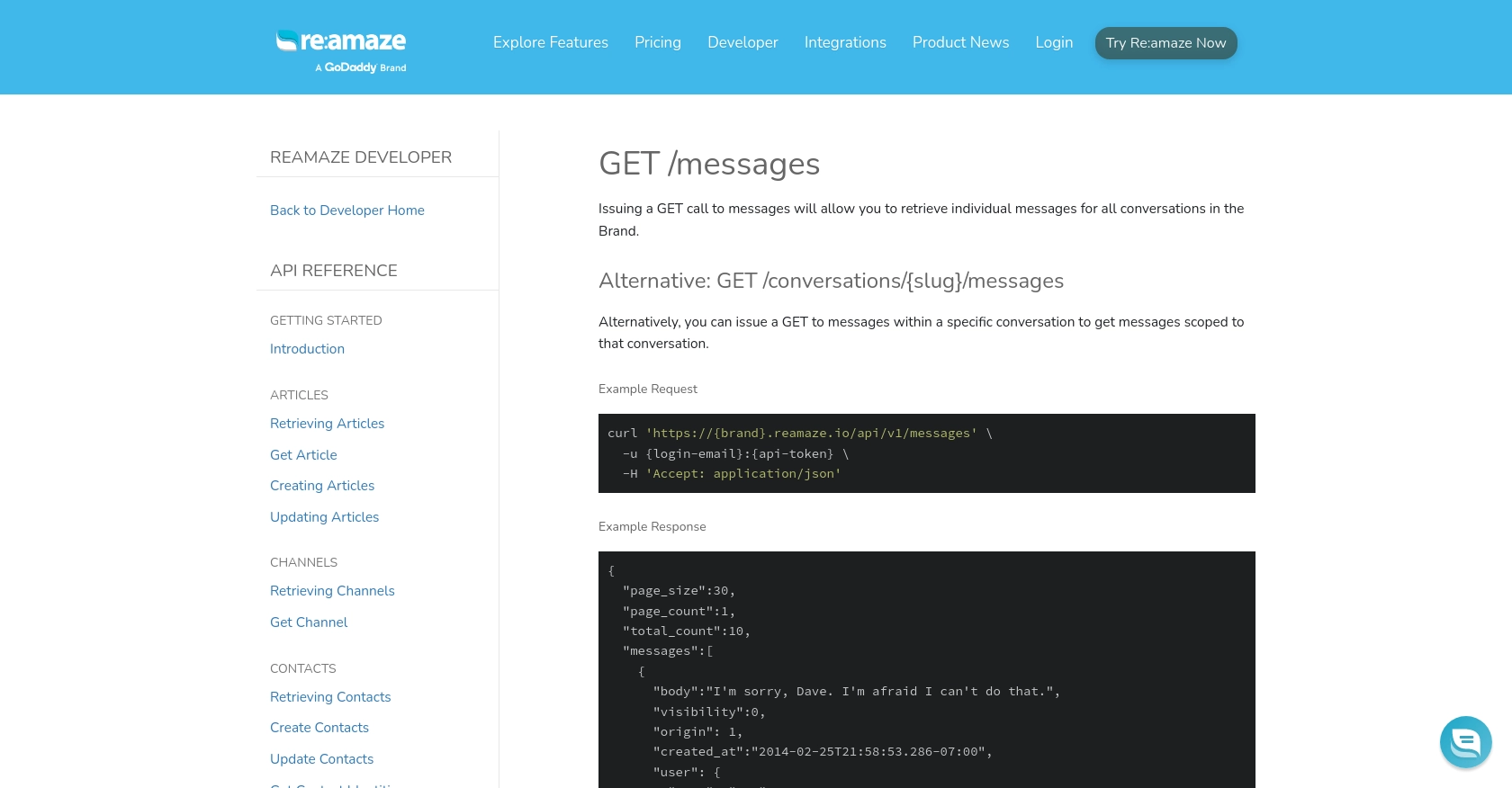
Conclusion and Best Practices for Using Re:amaze API with PHP
Integrating with the Re:amaze API using PHP can significantly enhance your customer support capabilities by automating message retrieval and processing. This integration allows you to streamline communication workflows, improve response times, and provide a seamless support experience for your customers.
Best Practices for Storing Re:amaze API Credentials
- Always store your API credentials securely. Use environment variables or secure vaults to keep your API token and login details safe.
- Avoid hardcoding sensitive information directly in your codebase to prevent unauthorized access.
Handling Re:amaze API Rate Limiting
- Be mindful of Re:amaze's rate limits to avoid disruptions. Implement logic to handle
HTTP 429 Too Many Requests
responses by retrying requests after a delay. - Consider batching requests or optimizing your API calls to stay within the allowed limits.
Data Transformation and Standardization
- Ensure that the data retrieved from Re:amaze is transformed and standardized to match your application's requirements.
- Use consistent data formats and structures to facilitate integration with other systems.
Enhancing Your Integration with Endgrate
While integrating with Re:amaze directly is powerful, using a tool like Endgrate can further simplify the process. Endgrate provides a unified API endpoint that connects to multiple platforms, allowing you to build once and deploy across various integrations seamlessly.
By leveraging Endgrate, you can save time and resources, focus on your core product, and offer an intuitive integration experience for your customers. Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
Ready to get started?