How to Get Orders with the Sap Business One API in Javascript
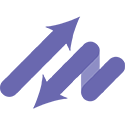
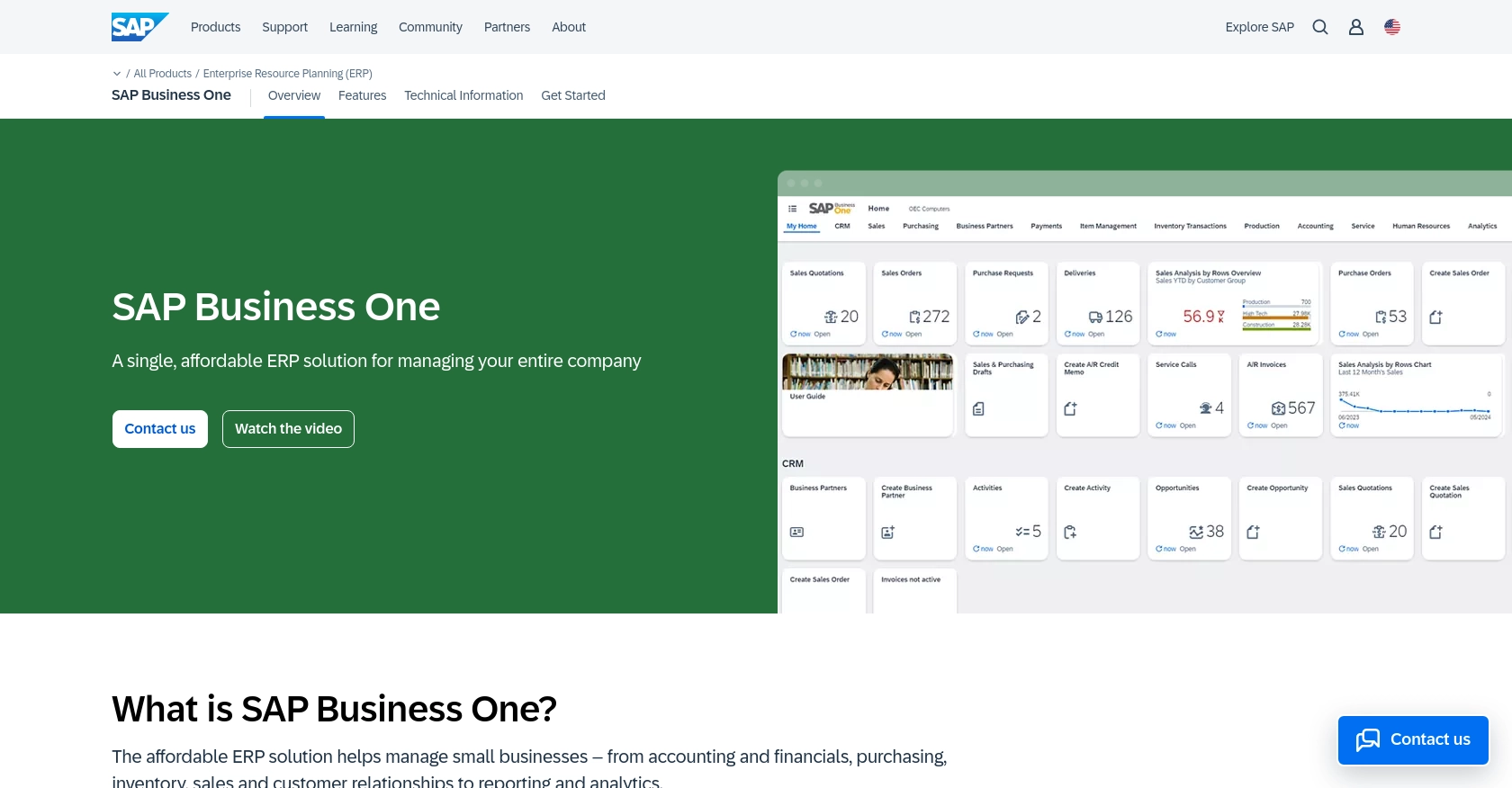
Introduction to SAP Business One
SAP Business One is a comprehensive enterprise resource planning (ERP) solution designed specifically for small to medium-sized businesses. It offers a wide range of functionalities, including financial management, sales, customer relationship management, and inventory control, all within a single integrated platform.
Developers may want to integrate with the SAP Business One API to streamline business processes and enhance operational efficiency. For example, accessing order data through the SAP Business One API can enable developers to automate order management, track sales performance, and improve customer service by providing real-time order status updates.
Setting Up a Test/Sandbox Account for SAP Business One API
Before you can start interacting with the SAP Business One API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a SAP Business One Sandbox Account
To get started, you need access to a SAP Business One sandbox environment. If your organization already uses SAP Business One, you can request access to a test environment from your system administrator. If not, you may need to contact SAP directly or a certified SAP partner to set up a trial or demo account.
Configuring OAuth Authentication for SAP Business One API
SAP Business One uses a custom authentication method. To authenticate your API requests, you'll need to create an application within your sandbox account. Follow these steps:
- Log in to your SAP Business One sandbox environment.
- Navigate to the Service Layer Management section.
- Create a new application and note down the Client ID and Client Secret.
- Set the necessary permissions for accessing order data.
These credentials will be used to authenticate your API requests.
Generating API Keys for SAP Business One
In addition to OAuth credentials, you may need an API key for certain operations. Follow these steps to generate an API key:
- Within your SAP Business One sandbox account, go to the API Management section.
- Select Create API Key and follow the prompts.
- Store the generated API key securely, as it will be required for making API calls.
For more detailed information on authentication, refer to the SAP Business One Service Layer documentation.
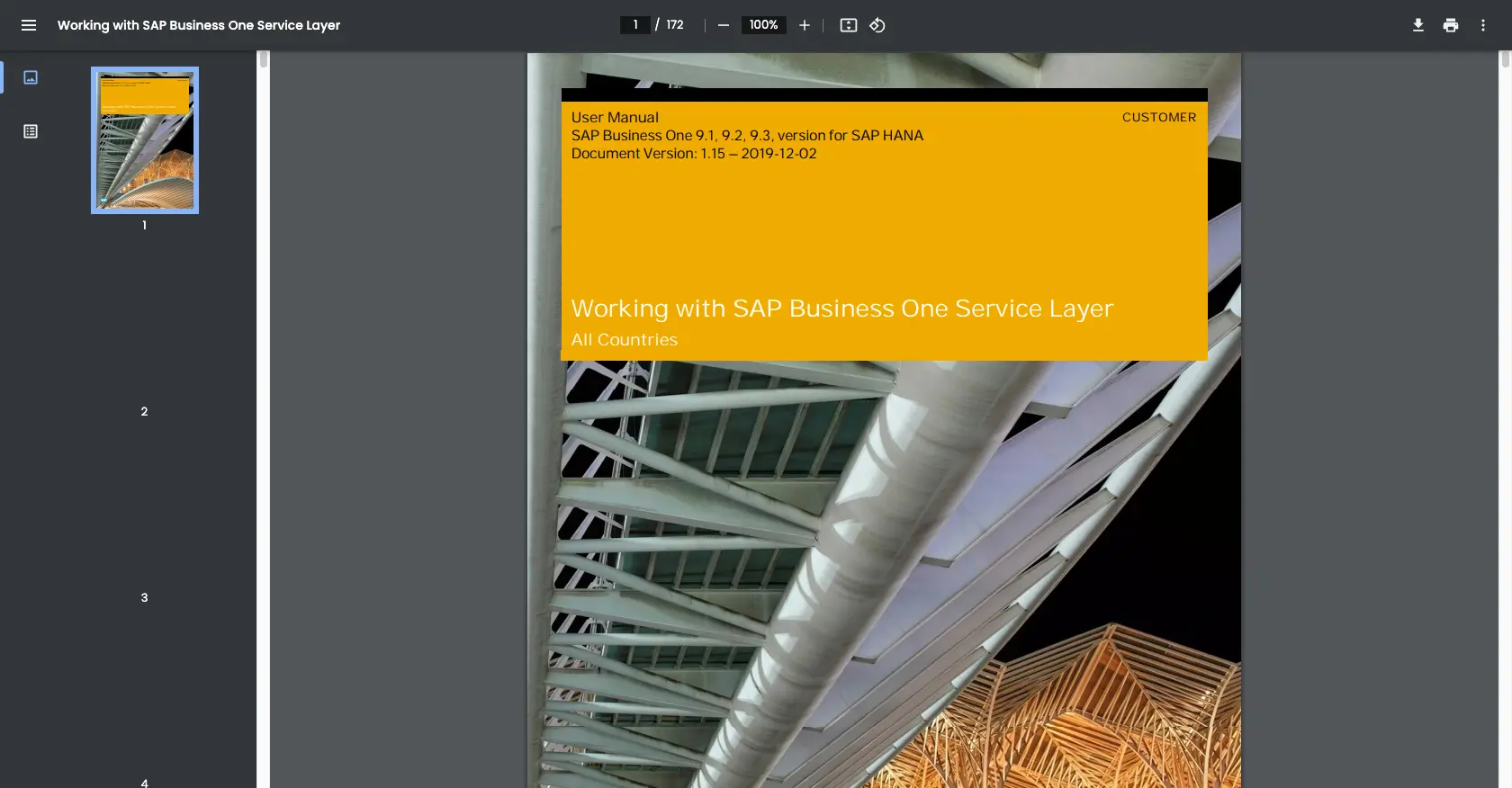
sbb-itb-96038d7
Making API Calls to Retrieve Orders from SAP Business One Using JavaScript
To interact with the SAP Business One API and retrieve order data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for SAP Business One API
Before you begin coding, ensure you have the following prerequisites installed on your machine:
- Node.js (version 14.x or later)
- npm (Node Package Manager)
Once you have Node.js and npm installed, you can set up your project by creating a new directory and initializing it:
mkdir sap-b1-api
cd sap-b1-api
npm init -y
Next, install the axios
library, which will be used to make HTTP requests:
npm install axios
Writing JavaScript Code to Fetch Orders from SAP Business One
Create a new file named getOrders.js
and add the following code to it:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://your-sap-business-one-url.com/b1s/v1/Orders';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer Your_Token'
};
// Function to get orders
async function getOrders() {
try {
const response = await axios.get(endpoint, { headers });
const orders = response.data.value;
// Display the orders
orders.forEach(order => {
console.log(`Order ID: ${order.DocEntry}, Customer: ${order.CardName}`);
});
} catch (error) {
console.error('Error fetching orders:', error.response ? error.response.data : error.message);
}
}
// Call the function
getOrders();
Replace Your_Token
with the token obtained from your SAP Business One sandbox account. This script uses the axios
library to send a GET request to the SAP Business One API endpoint for orders. It then logs the order ID and customer name for each order retrieved.
Running the JavaScript Code and Verifying Results
To execute the script, run the following command in your terminal:
node getOrders.js
You should see a list of orders printed in the terminal, each displaying the order ID and customer name. Verify the data by checking your SAP Business One sandbox account to ensure the orders match the output.
Handling Errors and Troubleshooting API Requests
When making API calls, it's essential to handle potential errors gracefully. The code above includes a try-catch
block to catch and log errors. Common issues may include:
- Invalid authentication token: Ensure your token is correct and not expired.
- Network issues: Check your internet connection and API endpoint URL.
- Permission errors: Verify that your application has the necessary permissions to access order data.
For more detailed error information, refer to the SAP Business One Service Layer documentation.
Conclusion and Best Practices for Using SAP Business One API in JavaScript
Integrating with the SAP Business One API using JavaScript can significantly enhance your business operations by automating order management and providing real-time insights into sales performance. By following the steps outlined in this guide, you can efficiently retrieve order data and integrate it into your existing systems.
Best Practices for Secure and Efficient SAP Business One API Integration
- Securely Store Credentials: Always store your API keys and tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage potential issues such as network failures or invalid responses, ensuring your application remains resilient.
Streamlining Integration with Endgrate
While integrating with SAP Business One API can be highly beneficial, it can also be complex and time-consuming. Endgrate offers a solution by providing a unified API endpoint that simplifies the integration process across multiple platforms, including SAP Business One. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration experience by visiting Endgrate and discover how you can streamline your integration processes efficiently.
Read More
Ready to get started?