How to Get Page Parameters with the Pendo API in Javascript
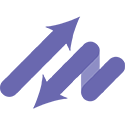
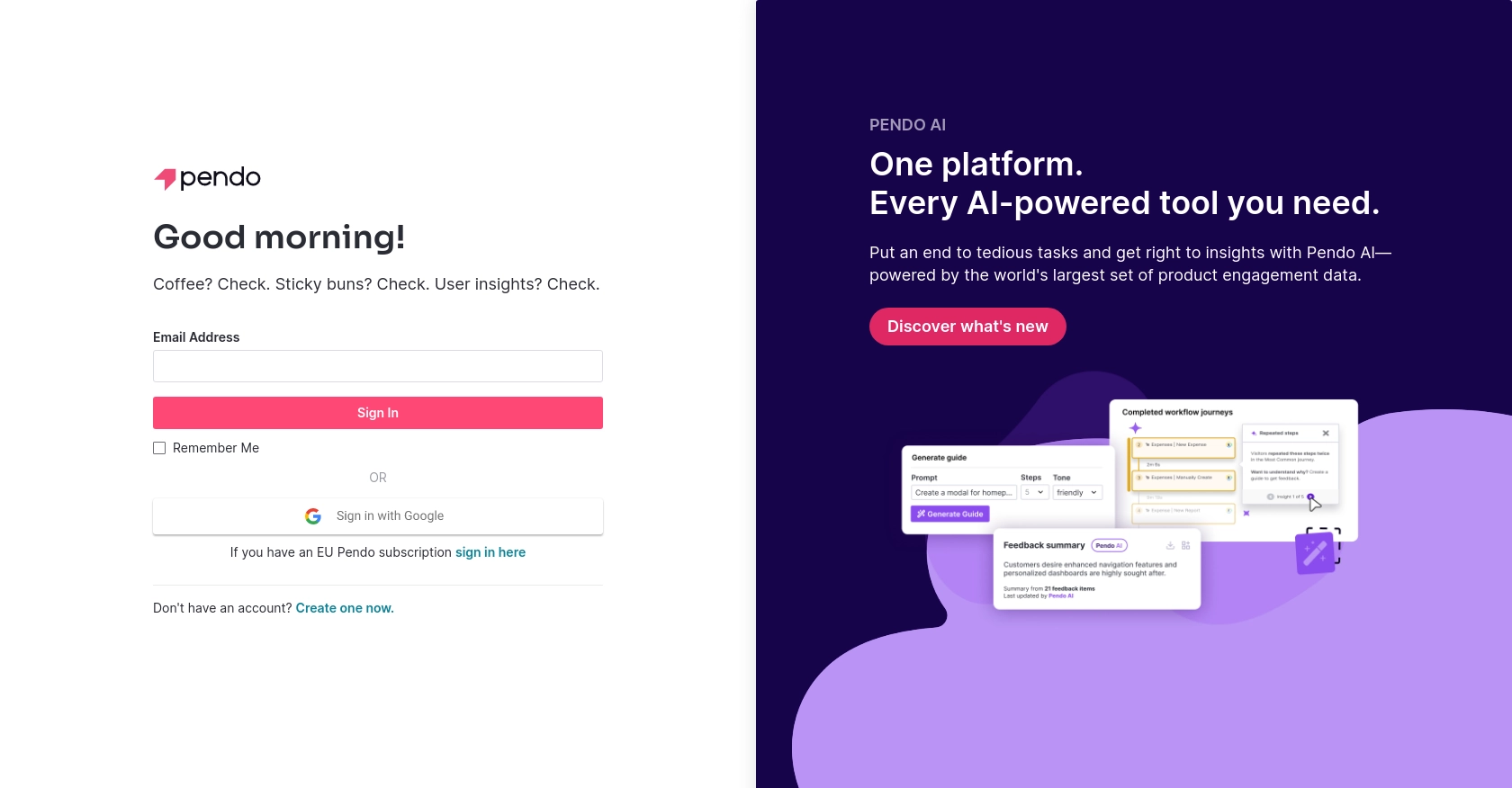
Introduction to Pendo API for Page Parameter Retrieval
Pendo is a powerful platform that provides product teams with insights into user behavior, helping them to improve user experiences and drive product adoption. With its robust analytics and feedback tools, Pendo enables businesses to understand how users interact with their products and make data-driven decisions.
Developers may want to integrate with the Pendo API to access detailed analytics and user behavior data. For example, retrieving page parameters using the Pendo API in JavaScript can help developers track user interactions on specific pages, allowing for more targeted improvements and personalized user experiences.
Setting Up Your Pendo Account for API Access
Before you can start retrieving page parameters using the Pendo API in JavaScript, you'll need to set up your Pendo account and obtain the necessary API key for authentication. Pendo provides a straightforward process to get started, allowing developers to access its powerful analytics tools.
Creating a Pendo Account
If you don't already have a Pendo account, you can sign up for a free trial on the Pendo website. This will give you access to the platform's features and allow you to explore its capabilities.
- Visit the Pendo website and click on the sign-up button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Pendo dashboard.
Generating an API Key for Pendo
To interact with the Pendo API, you'll need an API key. This key will authenticate your requests and allow you to access the data you need.
- Navigate to the settings section of your Pendo dashboard.
- Look for the API keys section and click on it.
- Generate a new API key and make sure to copy it somewhere safe, as you'll need it for your API calls.
Configuring Your Development Environment
With your API key in hand, you can now set up your development environment to start making API calls. Ensure you have a JavaScript environment ready, such as Node.js, and any necessary libraries installed.
- Install Node.js if you haven't already.
- Use npm to install any required packages for making HTTP requests, such as Axios or Fetch.
With your Pendo account set up and your API key ready, you're now prepared to start retrieving page parameters using the Pendo API in JavaScript.
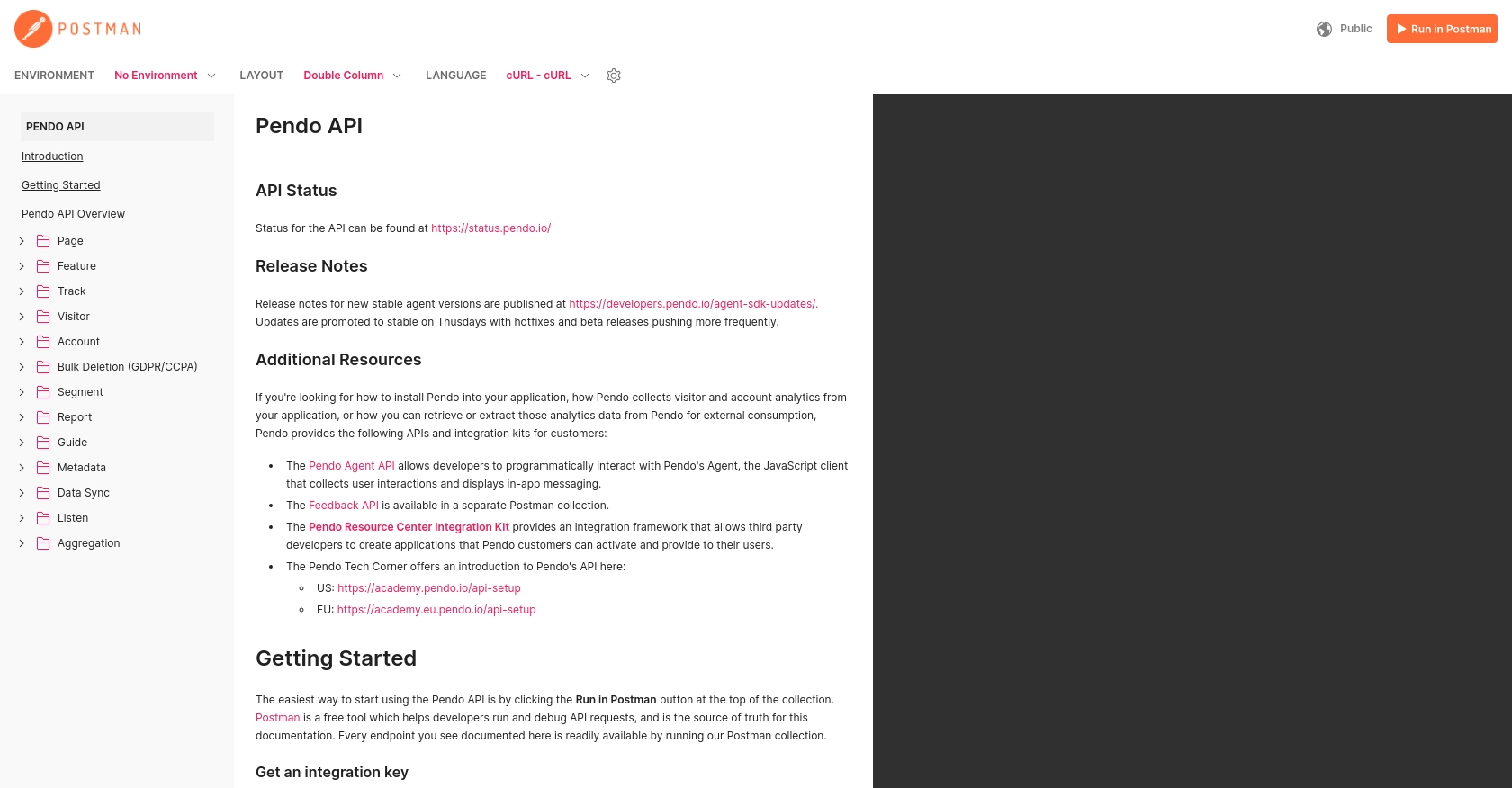
sbb-itb-96038d7
Making API Calls to Retrieve Page Parameters with Pendo API in JavaScript
To effectively interact with the Pendo API and retrieve page parameters, you'll need to set up your JavaScript environment and make HTTP requests using the API key you generated earlier. This section will guide you through the process of making these API calls using JavaScript.
Setting Up Your JavaScript Environment for Pendo API
Before making API calls, ensure your development environment is ready. You'll need Node.js and a library for making HTTP requests, such as Axios or Fetch.
- Ensure Node.js is installed on your machine. You can download it from the official Node.js website.
- Use npm to install Axios by running the following command:
npm install axios
Writing JavaScript Code to Retrieve Page Parameters from Pendo API
With your environment set up, you can now write the JavaScript code to retrieve page parameters from the Pendo API. Below is a sample code snippet to guide you through the process:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://engageapi.pendo.io/api/v1/pageParameters';
const headers = {
'Content-Type': 'application/json',
'x-pendo-integration-key': 'Your_API_Key'
};
// Function to get page parameters
async function getPageParameters() {
try {
const response = await axios.get(endpoint, { headers });
const data = response.data;
console.log('Page Parameters:', data);
} catch (error) {
console.error('Error fetching page parameters:', error.response ? error.response.data : error.message);
}
}
// Call the function
getPageParameters();
Replace Your_API_Key
with the API key you generated from your Pendo account. This code uses Axios to make a GET request to the Pendo API endpoint for page parameters. If successful, it logs the retrieved data to the console.
Verifying API Call Success and Handling Errors
After running the code, you should see the page parameters printed in your console. To verify the success of your API call, check the response data against your Pendo dashboard to ensure it matches the expected parameters.
In case of errors, the code includes error handling to log the error message or response data. Common error codes include:
- 401 Unauthorized: Check if your API key is correct and has the necessary permissions.
- 404 Not Found: Ensure the API endpoint URL is correct.
By following these steps, you can efficiently retrieve page parameters using the Pendo API in JavaScript, enabling you to gain valuable insights into user interactions on your platform.
Conclusion: Best Practices for Using Pendo API in JavaScript
Integrating with the Pendo API to retrieve page parameters in JavaScript can significantly enhance your ability to analyze user interactions and improve product experiences. By following the steps outlined in this guide, you can efficiently set up your environment, make API calls, and handle potential errors.
Best Practices for Storing Pendo API Credentials Securely
- Store your API key in environment variables or secure vaults rather than hardcoding it in your codebase.
- Regularly rotate your API keys to minimize security risks.
Handling Pendo API Rate Limiting and Optimizing Requests
While interacting with the Pendo API, be mindful of any rate limits that may apply. Although specific rate limit details are not provided, it's a good practice to implement retry logic with exponential backoff to handle rate limit errors gracefully.
Transforming and Standardizing Data from Pendo API
When retrieving data from the Pendo API, consider transforming and standardizing the data fields to match your application's data structures. This will ensure consistency and ease of integration with other systems.
By adhering to these best practices, you can maximize the benefits of using the Pendo API and enhance your product's user experience. If you're looking to streamline your integration processes further, consider leveraging Endgrate. Endgrate allows you to save time and resources by providing a unified API endpoint for multiple platforms, enabling you to focus on your core product development.
Explore how Endgrate can simplify your integration needs by visiting Endgrate today.
Read More
Ready to get started?