How to Get Parties with the Capsule API in PHP
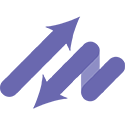
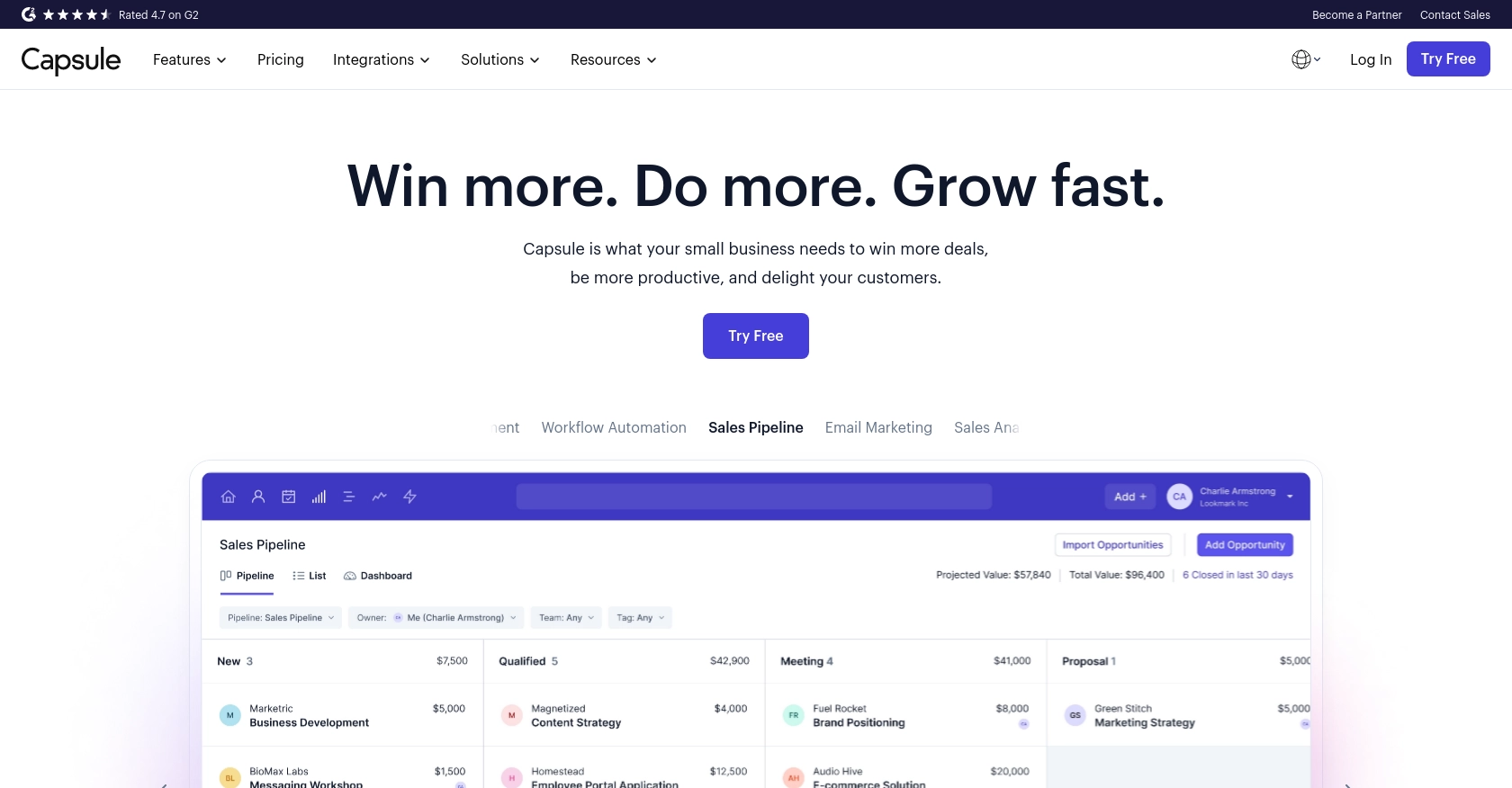
Introduction to Capsule CRM
Capsule CRM is a versatile customer relationship management platform designed to help businesses manage their contacts, sales opportunities, and customer interactions efficiently. Known for its user-friendly interface and robust features, Capsule CRM is a popular choice for businesses looking to streamline their CRM processes.
Developers may want to integrate with Capsule CRM to access and manage customer data, such as parties, which include both people and organizations. For example, a developer might use the Capsule API to retrieve party information to synchronize customer data across multiple platforms, ensuring consistency and accuracy.
Setting Up Your Capsule CRM Test Account
Before you can start integrating with the Capsule API, you'll need to set up a test account. This will allow you to safely experiment with the API without affecting any live data.
Creating a Capsule CRM Account
If you don't already have a Capsule CRM account, you can sign up for a free trial on the Capsule CRM website. Follow the instructions to create your account and log in.
Generating OAuth Credentials for Capsule API
Capsule CRM uses OAuth 2.0 for authentication, which means you'll need to create an application to obtain the necessary credentials.
- Navigate to the My Preferences section in your Capsule account.
- Click on API Authentication Tokens to create a new token.
- Register your application by providing a name and description.
- Once registered, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are essential for API access.
Obtaining a Bearer Token
To interact with the Capsule API, you'll need a bearer token. Follow these steps to obtain one:
- Redirect users to the Capsule authorization URL:
https://api.capsulecrm.com/oauth/authorise
with the necessary query parameters such asresponse_type=code
,client_id
, andredirect_uri
. - After user authorization, Capsule will redirect back to your specified
redirect_uri
with an authorization code. - Exchange this code for an access token by making a POST request to
https://api.capsulecrm.com/oauth/token
with the required parameters.
// Example PHP code to exchange authorization code for access token
$client_id = 'your_client_id';
$client_secret = 'your_client_secret';
$code = 'authorization_code_received';
$redirect_uri = 'your_redirect_uri';
$response = file_get_contents('https://api.capsulecrm.com/oauth/token', false, stream_context_create([
'http' => [
'method' => 'POST',
'header' => 'Content-Type: application/x-www-form-urlencoded',
'content' => http_build_query([
'grant_type' => 'authorization_code',
'client_id' => $client_id,
'client_secret' => $client_secret,
'code' => $code,
'redirect_uri' => $redirect_uri
])
]
]));
$token_data = json_decode($response, true);
$access_token = $token_data['access_token'];
Store the access token securely, as it will be used to authenticate your API requests.
Testing Your Capsule API Integration
With your bearer token ready, you can now test your integration by making API calls to the Capsule sandbox environment. This ensures that your integration works as expected before deploying it to production.
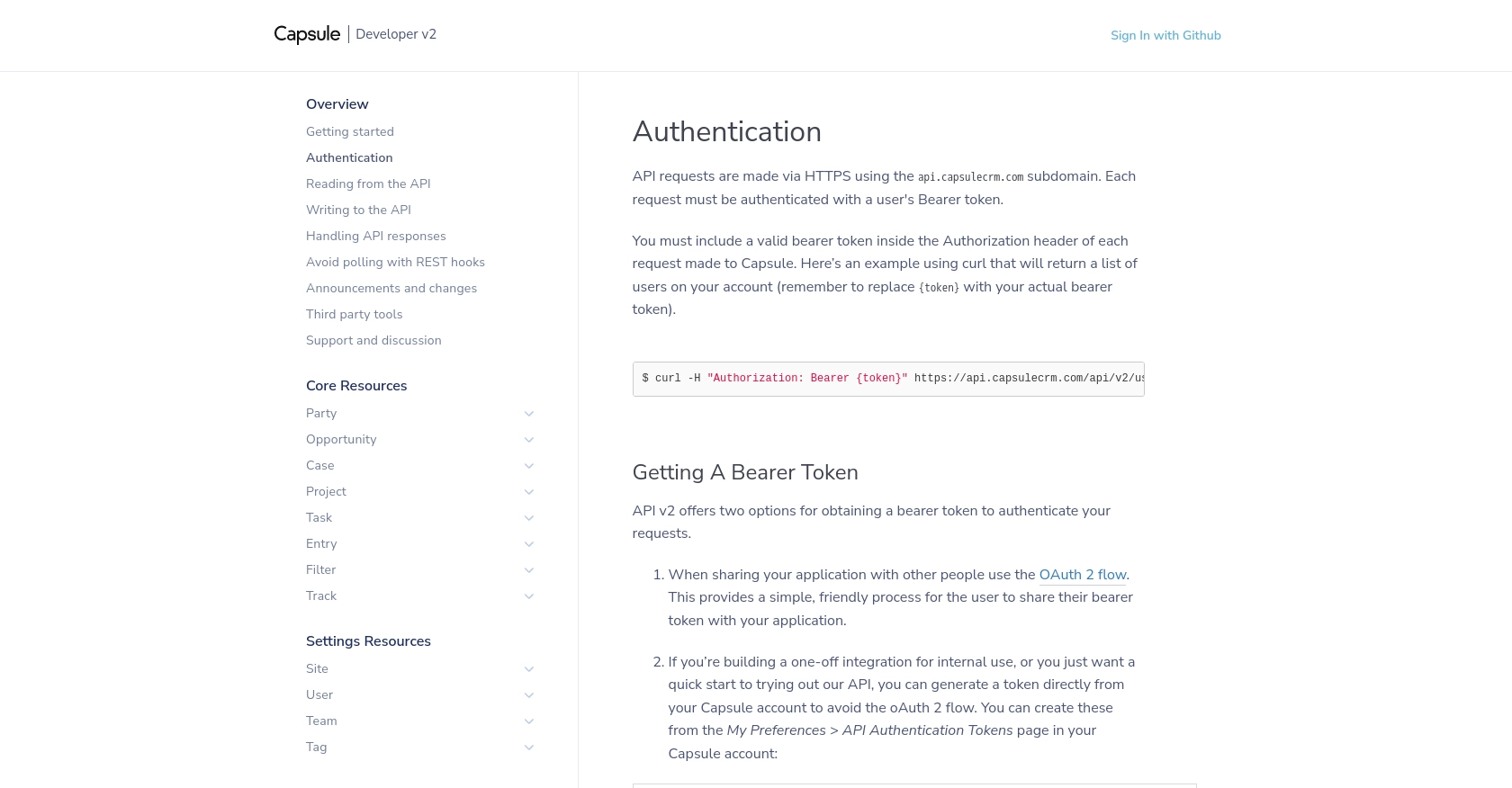
sbb-itb-96038d7
Making API Calls to Retrieve Parties from Capsule CRM Using PHP
To interact with the Capsule API and retrieve party information, you'll need to make HTTP requests using PHP. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your PHP Environment for Capsule API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or later
- cURL extension enabled
These prerequisites will allow you to make HTTP requests and handle JSON responses efficiently.
Installing Required PHP Dependencies for Capsule API
To interact with the Capsule API, you'll use the cURL library, which is typically included with PHP. Ensure it's enabled in your php.ini
file:
; Enable cURL extension module
extension=curl
Writing PHP Code to Retrieve Parties from Capsule CRM
With your environment set up, you can now write the PHP code to make a GET request to the Capsule API and retrieve party data.
// Set the API endpoint and access token
$endpoint = 'https://api.capsulecrm.com/api/v2/parties';
$access_token = 'your_access_token';
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $access_token,
'Content-Type: application/json'
]);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
exit;
}
// Close cURL session
curl_close($ch);
// Decode the JSON response
$data = json_decode($response, true);
// Display the parties
foreach ($data['parties'] as $party) {
echo 'ID: ' . $party['id'] . ', Name: ' . $party['firstName'] . ' ' . $party['lastName'] . "\n";
}
Replace your_access_token
with the bearer token obtained during the authentication process. This code initializes a cURL session, sets the necessary headers, and makes a GET request to the Capsule API to retrieve parties.
Handling API Responses and Errors from Capsule CRM
After executing the request, it's crucial to handle the response and any potential errors. The Capsule API returns a JSON object containing party data if the request is successful. If an error occurs, such as an invalid token, you'll receive an HTTP status code indicating the issue.
Common error codes include:
- 401 Unauthorized: Invalid or expired token.
- 403 Forbidden: Insufficient permissions.
- 429 Too Many Requests: Rate limit exceeded. Capsule allows up to 4,000 requests per hour. Check the
X-RateLimit-Remaining
header to monitor your usage.
For more details on handling errors, refer to the Capsule API documentation.
Verifying Successful API Requests in Capsule CRM
To confirm that your API requests are successful, log into your Capsule CRM account and navigate to the parties section. You should see the data retrieved by your PHP script reflected in the CRM interface.
By following these steps, you can efficiently integrate with Capsule CRM and manage party data using PHP. For more advanced use cases, consider exploring additional endpoints and features provided by the Capsule API.
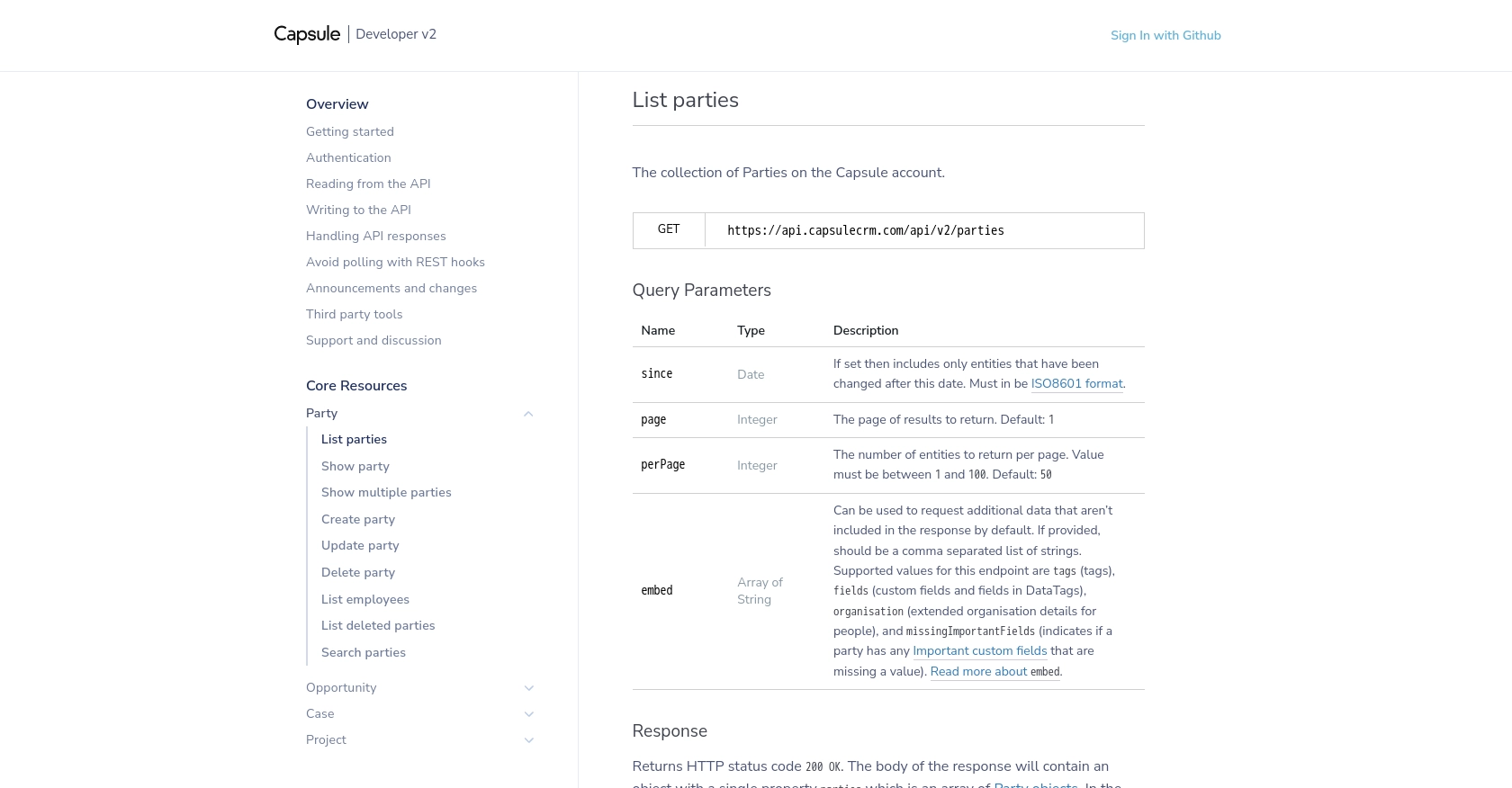
Conclusion and Best Practices for Capsule CRM API Integration Using PHP
Integrating with Capsule CRM's API using PHP provides a powerful way to manage customer data efficiently. By following the steps outlined in this guide, you can retrieve and handle party information seamlessly, ensuring your applications remain synchronized with Capsule CRM.
Best Practices for Secure and Efficient Capsule API Integration
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code.
- Handle Rate Limits: Capsule CRM allows up to 4,000 requests per hour. Monitor your usage with the
X-RateLimit-Remaining
header and implement throttling to avoid exceeding limits. - Implement Error Handling: Ensure robust error handling by checking HTTP status codes and handling common errors like 401 Unauthorized and 429 Too Many Requests.
- Optimize Data Handling: Use caching and the
since
query parameter to minimize API calls and keep your data up-to-date efficiently.
Enhance Your Integration Capabilities with Endgrate
For developers looking to streamline their integration processes further, consider leveraging Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore the possibilities with Endgrate and take your Capsule CRM integrations to the next level. Visit Endgrate to learn more about how you can simplify and enhance your integration workflows.
Read More
- https://endgrate.com/provider/capsulecrm
- https://developer.capsulecrm.com/v2/overview/authentication
- https://developer.capsulecrm.com/v2/overview/handling-api-responses
- https://developer.capsulecrm.com/v2/overview/avoid-polling-with-rest-hooks
- https://developer.capsulecrm.com/v2/operations/Party#listParties
Ready to get started?