Using the Google Ads API to Get Leads (with PHP examples)
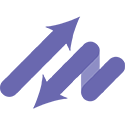
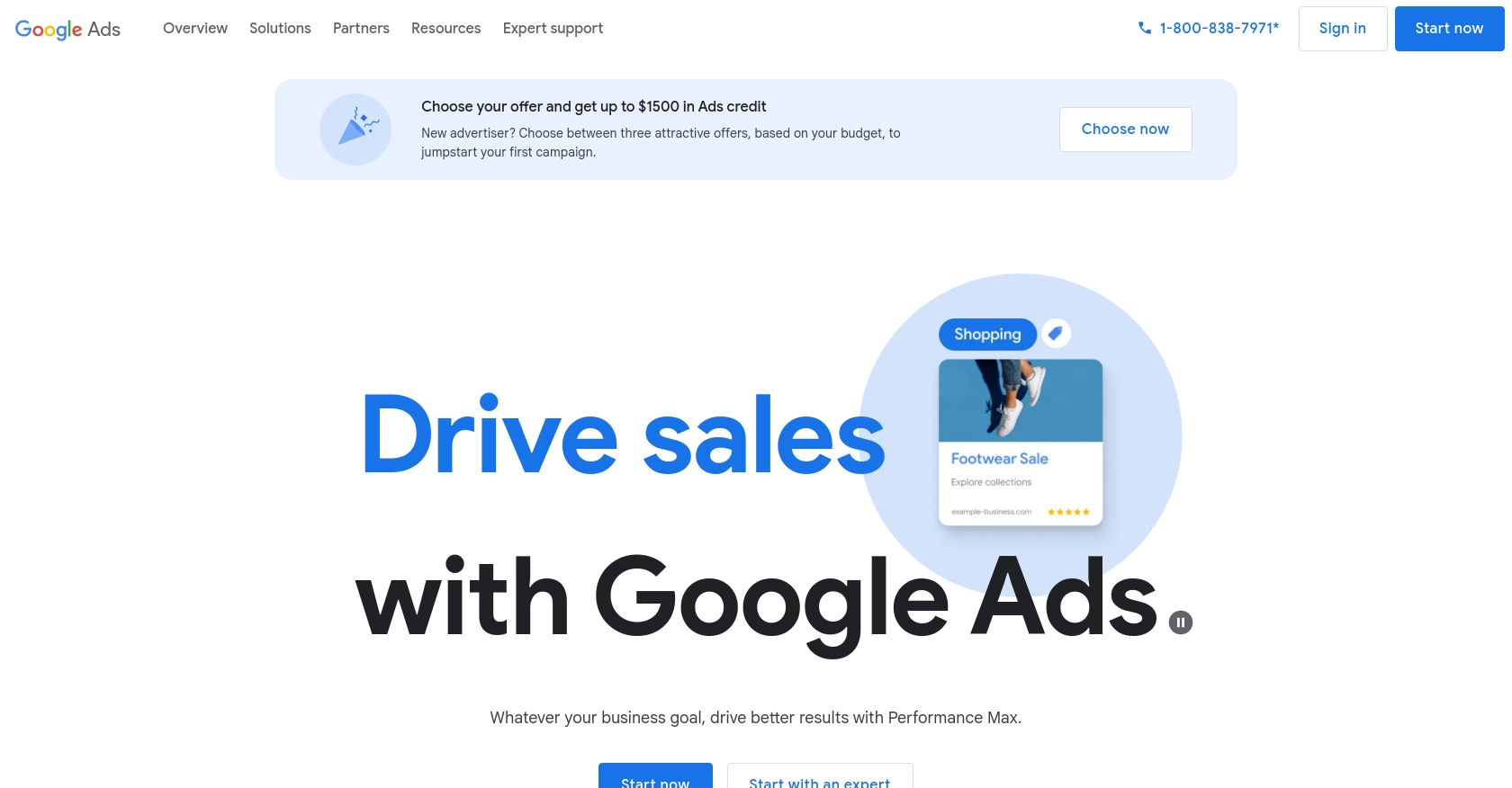
Introduction to Google Ads API
The Google Ads API is a powerful tool for managing and optimizing Google Ads accounts, providing developers with the ability to automate complex tasks and access detailed data. This API is essential for businesses looking to enhance their advertising strategies by integrating Google Ads functionalities directly into their own systems.
Developers might want to connect with the Google Ads API to streamline lead management processes. For example, by retrieving lead data directly from Google Ads, businesses can automatically import this information into their CRM systems, allowing for real-time updates and more efficient lead nurturing.
In this article, we will explore how to use PHP to interact with the Google Ads API, specifically focusing on retrieving leads. This integration can significantly enhance your marketing efforts by ensuring that your sales team has immediate access to the latest lead information.
Setting Up Your Google Ads Test Account for API Integration
Before you can start retrieving leads using the Google Ads API with PHP, you need to set up a Google Ads test account. This involves creating a Google Ads manager account, obtaining a developer token, and setting up OAuth 2.0 credentials. These steps will ensure you have the necessary permissions and access to interact with the API.
Create a Google Ads Manager Account
If you don't already have a Google Ads manager account, you'll need to create one. A manager account allows you to manage multiple Google Ads accounts and is essential for accessing the Google Ads API.
- Visit the Google Ads Manager Accounts page.
- Follow the instructions to create a new manager account.
- Once your account is set up, note down your 10-digit account number, which will be used in API calls.
Obtain a Developer Token
A developer token is required to make API calls. This token is linked to your manager account and controls the number of API calls you can make.
- Log in to your Google Ads manager account.
- Navigate to the Tools & Settings menu and select Setup > API Center.
- Apply for a developer token by following the on-screen instructions.
- Once approved, your token will be available in the API Center.
Set Up a Google API Console Project
To use OAuth 2.0 for authentication, you need to create a project in the Google API Console. This project will generate the client ID and client secret required for OAuth 2.0 credentials.
- Go to the Google API Console.
- Create a new project or select an existing one.
- Enable the Google Ads API for your project by navigating to APIs & Services > Library and searching for "Google Ads API".
- Click Enable to activate the API for your project.
Generate OAuth 2.0 Credentials
OAuth 2.0 credentials are necessary for secure access to the Google Ads API. Follow these steps to create them:
- In the Google API Console, navigate to APIs & Services > Credentials.
- Click Create credentials and select OAuth client ID.
- Configure the consent screen by providing the necessary information.
- Select Web application as the application type and enter your redirect URI.
- Click Create to generate your client ID and client secret.
- Save these credentials securely as they will be used in your API calls.
With your Google Ads manager account, developer token, and OAuth 2.0 credentials set up, you're now ready to start integrating with the Google Ads API using PHP. For more detailed information, refer to the Google Ads API documentation.
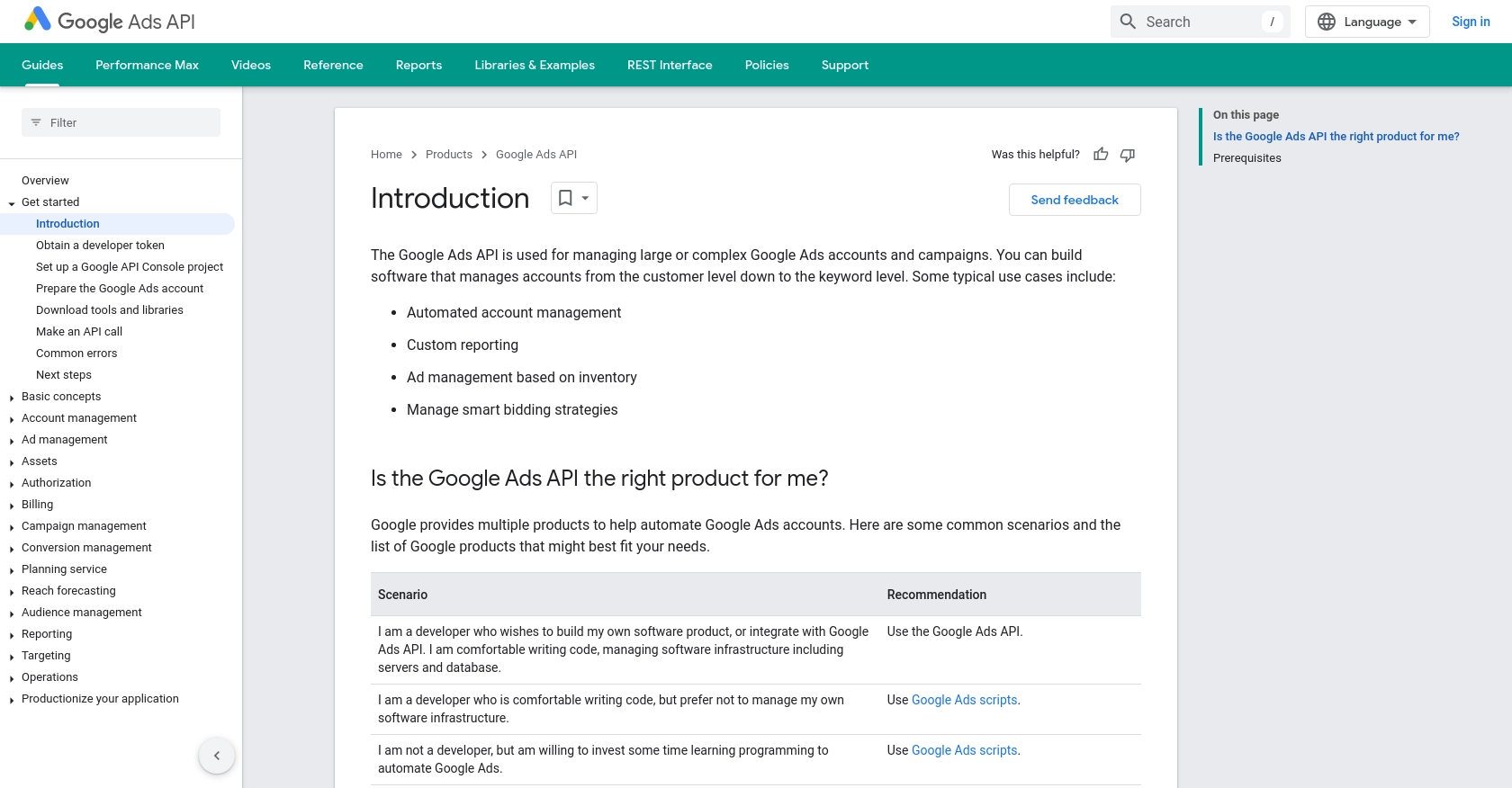
sbb-itb-96038d7
Making API Calls to Retrieve Leads from Google Ads Using PHP
To interact with the Google Ads API and retrieve leads using PHP, you'll need to set up your development environment with the necessary tools and libraries. This section will guide you through the process of making API calls, handling responses, and managing errors effectively.
Setting Up Your PHP Environment for Google Ads API
Before you begin, ensure you have PHP installed on your machine. You will also need Composer, a dependency manager for PHP, to install the required libraries.
- Ensure PHP 7.4 or higher is installed. You can download it from the official PHP website.
- Install Composer by following the instructions on the Composer website.
Installing Google Ads API Client Library for PHP
The Google Ads API client library for PHP simplifies the process of interacting with the API. Install it using Composer:
composer require googleads/google-ads-php
Writing PHP Code to Retrieve Leads from Google Ads
With the client library installed, you can now write PHP code to make API calls and retrieve leads. Create a new PHP file and add the following code:
require 'vendor/autoload.php';
use Google\Ads\GoogleAds\Lib\V17\GoogleAdsClientBuilder;
use Google\Ads\GoogleAds\V17\Services\GoogleAdsServiceClient;
use Google\Ads\GoogleAds\Util\V17\ResourceNames;
use Google\Auth\CredentialsLoader;
// Set your Google Ads API credentials
$clientId = 'YOUR_CLIENT_ID';
$clientSecret = 'YOUR_CLIENT_SECRET';
$refreshToken = 'YOUR_REFRESH_TOKEN';
$developerToken = 'YOUR_DEVELOPER_TOKEN';
$loginCustomerId = 'YOUR_LOGIN_CUSTOMER_ID';
// Create a Google Ads client
$googleAdsClient = (new GoogleAdsClientBuilder())
->withOAuth2Credential(CredentialsLoader::makeOAuth2Credential(
$clientId,
$clientSecret,
$refreshToken
))
->withDeveloperToken($developerToken)
->withLoginCustomerId($loginCustomerId)
->build();
// Define the customer ID and query to retrieve leads
$customerId = 'YOUR_CUSTOMER_ID';
$query = 'SELECT lead_form_submission_data.resource_name, lead_form_submission_data.id FROM lead_form_submission_data';
// Make the API call
$response = $googleAdsClient->getGoogleAdsServiceClient()->search($customerId, $query);
// Process the response
foreach ($response->iterateAllElements() as $lead) {
printf("Lead ID: %s\n", $lead->getLeadFormSubmissionData()->getId());
}
Replace placeholders like YOUR_CLIENT_ID
and YOUR_CUSTOMER_ID
with your actual credentials and customer ID.
Verifying API Call Success and Handling Errors
After running your PHP script, verify the success of your API call by checking the output for lead IDs. If the call fails, handle errors by catching exceptions and reviewing error messages:
try {
// Your API call code here
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Refer to the Google Ads API documentation for more details on error codes and troubleshooting.
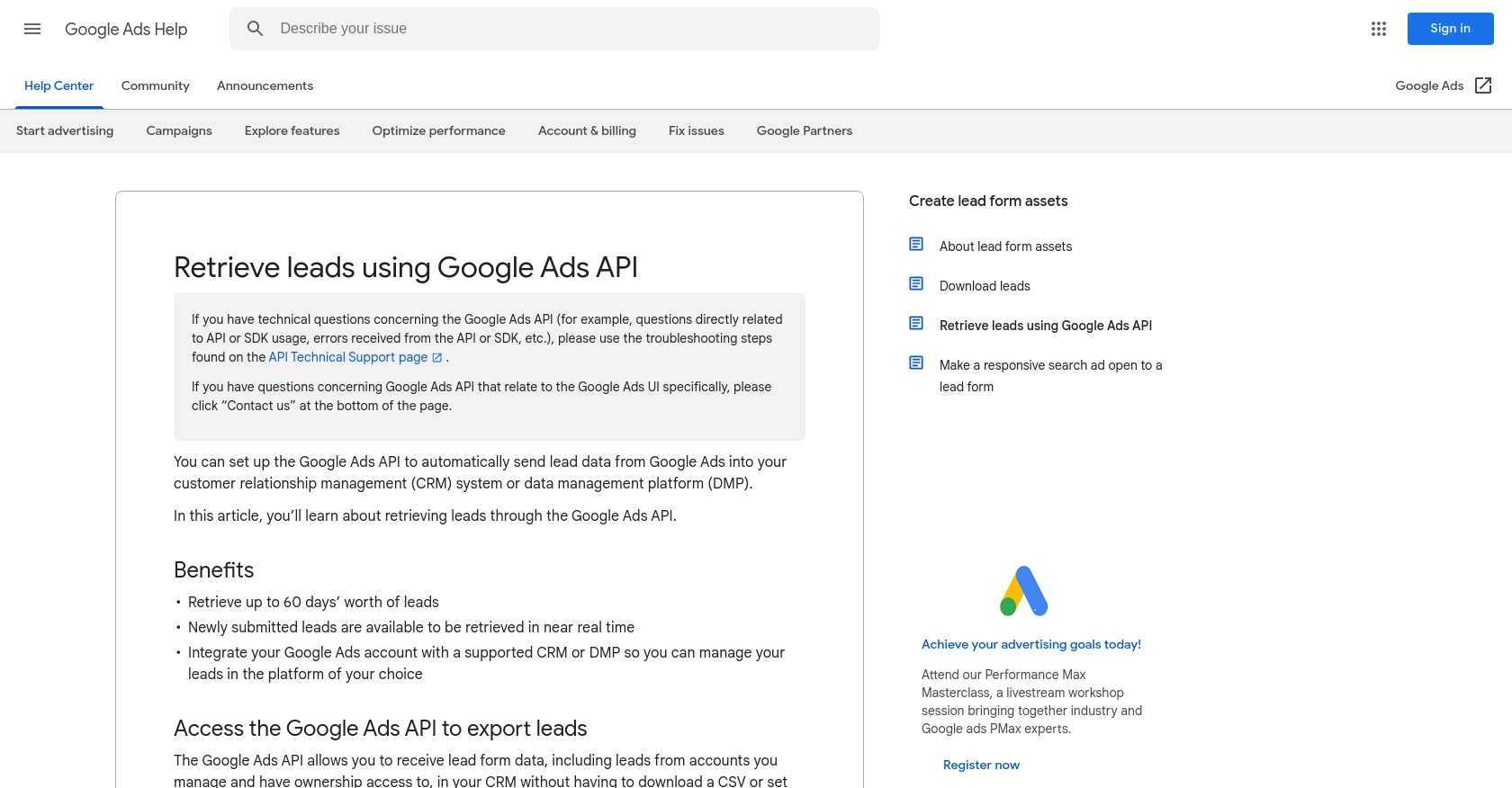
Conclusion and Best Practices for Using Google Ads API with PHP
Integrating the Google Ads API into your PHP applications can significantly enhance your ability to manage and optimize advertising campaigns. By automating lead retrieval and integrating this data into your CRM, you can streamline your marketing efforts and improve lead nurturing processes.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth 2.0 credentials, developer token, and other sensitive information securely. Consider using environment variables or secure vaults to manage these credentials.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid exceeding the allowed number of requests. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that the data retrieved from the API is standardized before integrating it into your CRM. This will help maintain data consistency and improve data analysis.
- Monitor API Usage: Regularly monitor your API usage to ensure compliance with Google's policies and to optimize your application's performance.
Enhance Your Integration Strategy with Endgrate
For developers looking to streamline multiple integrations, Endgrate offers a unified API endpoint that simplifies the process of connecting with various platforms, including Google Ads. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development while providing an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a seamless integration solution.
Read More
Ready to get started?