How to Get People with the Affinity API in PHP
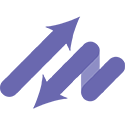
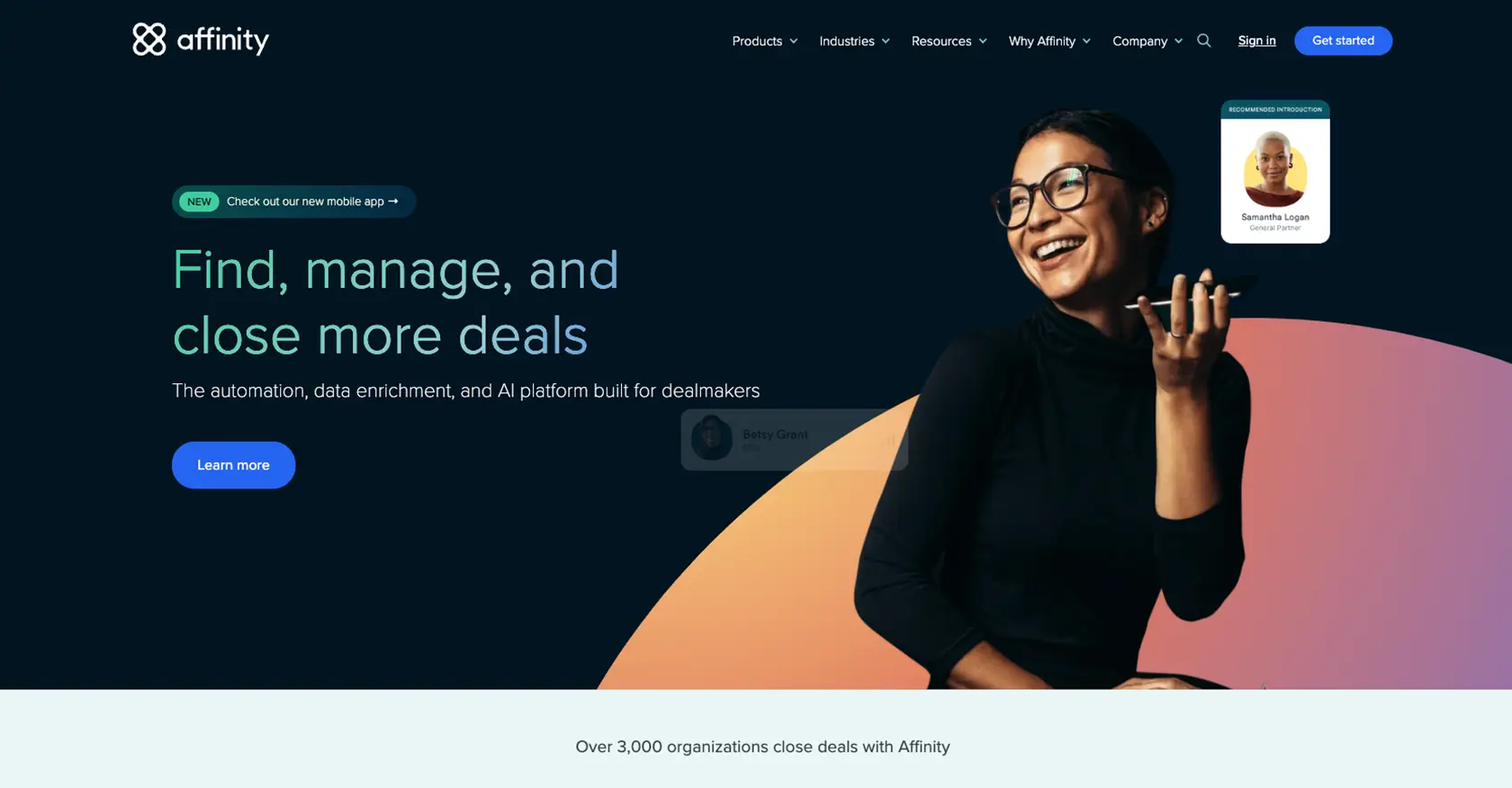
Introduction to Affinity API
Affinity is a powerful relationship intelligence platform designed to help businesses manage and leverage their professional networks. It offers a comprehensive suite of tools to track interactions, manage contacts, and analyze relationship data, making it an essential tool for businesses looking to enhance their networking capabilities.
Developers may want to integrate with the Affinity API to automate the management of contacts and relationships. For example, using the Affinity API, a developer can retrieve a list of people associated with an organization, enabling seamless integration of contact data into custom applications or CRM systems.
Setting Up Your Affinity API Test Account
Before you can start using the Affinity API to manage contacts and relationships, you'll need to set up a test account. Affinity provides a straightforward process to create an account and generate the necessary API key for authentication.
Step-by-Step Guide to Creating an Affinity Test Account
-
Sign Up for an Affinity Account:
Visit the Affinity website and sign up for a free trial or demo account. This will give you access to the Affinity platform and its features.
-
Access the Settings Panel:
Once logged in, navigate to the Settings Panel. This is accessible through the left sidebar on the Affinity web app.
-
Generate Your API Key:
In the Settings Panel, look for the option to generate an API key. This key will be used to authenticate your API requests. Make sure to store it securely, as it will be required for all API interactions.
Understanding Affinity API Authentication
The Affinity API uses HTTP Basic Auth for authentication. Here's how you can authenticate your requests:
$apiKey = 'Your_Affinity_API_Key';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https://api.affinity.co/api_endpoint");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, ":$apiKey");
$response = curl_exec($ch);
curl_close($ch);
Replace Your_Affinity_API_Key
with the API key you generated. This code snippet demonstrates how to set up a cURL request in PHP to authenticate with the Affinity API.
Handling Authentication Errors
If your API key is invalid or missing, you will receive a 401 Unauthorized error. Ensure that your API key is correctly included in the request headers. For more details on error codes, refer to the Affinity API documentation.
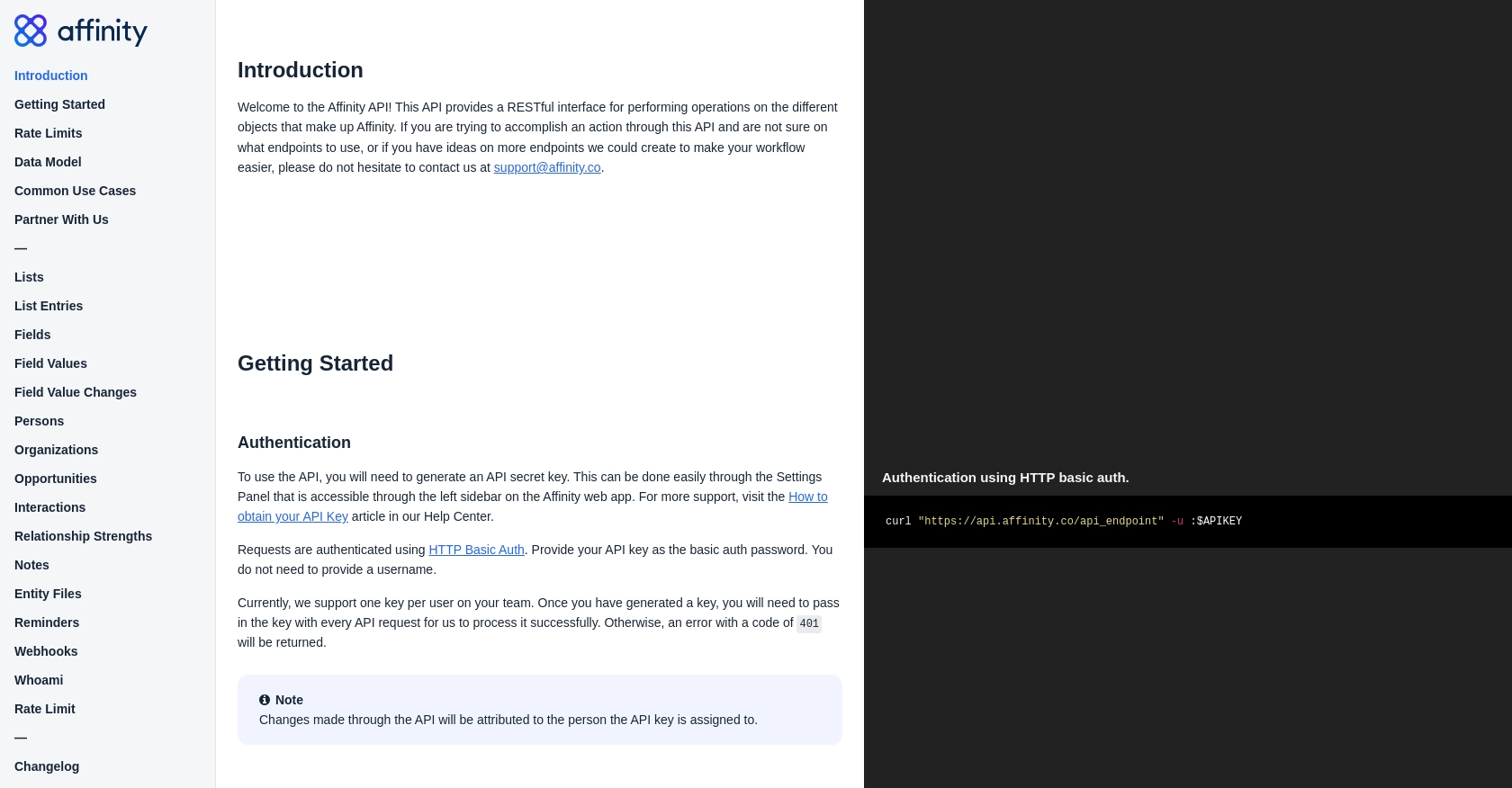
sbb-itb-96038d7
Making API Calls to Retrieve People Data with Affinity API in PHP
To interact with the Affinity API and retrieve people data, you need to set up your PHP environment correctly. This involves ensuring you have the necessary PHP version and dependencies installed, and then writing the code to make the API call.
PHP Environment Setup for Affinity API Integration
Before making API calls, ensure your PHP environment is ready:
- PHP Version: Ensure you are using PHP 7.4 or higher.
- cURL Extension: The cURL extension must be enabled in your PHP installation to make HTTP requests.
Installing Required PHP Dependencies
For this tutorial, you need the cURL extension, which is typically included with PHP. Verify it's enabled by checking your php.ini
file or using phpinfo()
.
Example Code to Retrieve People Data from Affinity API
Below is a PHP code snippet to retrieve a list of people using the Affinity API:
$apiKey = 'Your_Affinity_API_Key';
$endpoint = 'https://api.affinity.co/persons';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, $endpoint);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, ":$apiKey");
$response = curl_exec($ch);
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
$data = json_decode($response, true);
foreach ($data['persons'] as $person) {
echo 'Name: ' . $person['first_name'] . ' ' . $person['last_name'] . "";
echo 'Email: ' . $person['primary_email'] . "";
}
}
curl_close($ch);
Replace Your_Affinity_API_Key
with your actual API key. This code initializes a cURL session, sets the necessary options for the API call, and processes the response to display the names and emails of people retrieved from Affinity.
Verifying Successful API Requests
After running the code, you should see a list of people with their names and emails. If the request is successful, the data will match the entries in your Affinity test account. If you encounter errors, check the error message returned by cURL and ensure your API key and endpoint URL are correct.
Handling API Errors and Response Codes
It's crucial to handle potential errors when making API calls. The Affinity API may return various error codes, such as:
- 401 Unauthorized: Invalid API key. Ensure your API key is correct.
- 404 Not Found: Incorrect endpoint URL. Verify the endpoint.
- 429 Too Many Requests: Rate limit exceeded. Implement rate limiting strategies.
For a complete list of error codes, refer to the Affinity API documentation.
Conclusion and Best Practices for Using Affinity API in PHP
Integrating with the Affinity API using PHP can significantly enhance your application's ability to manage and leverage professional relationships. By automating the retrieval and management of contact data, you can streamline workflows and improve data accuracy across your systems.
Best Practices for Affinity API Integration
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Implement Rate Limiting: Be mindful of the Affinity API's rate limits. Implement strategies to handle the
429 Too Many Requests
error, such as exponential backoff or request queuing. - Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your application's data model.
Enhance Your Integration with Endgrate
While integrating with the Affinity API can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Affinity. This allows you to focus on your core product while outsourcing the complexities of integration management.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate and discover how companies like yours have scaled their integrations efficiently.
Read More
Ready to get started?