How to Get Policies with the Applied Epic API in PHP
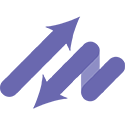
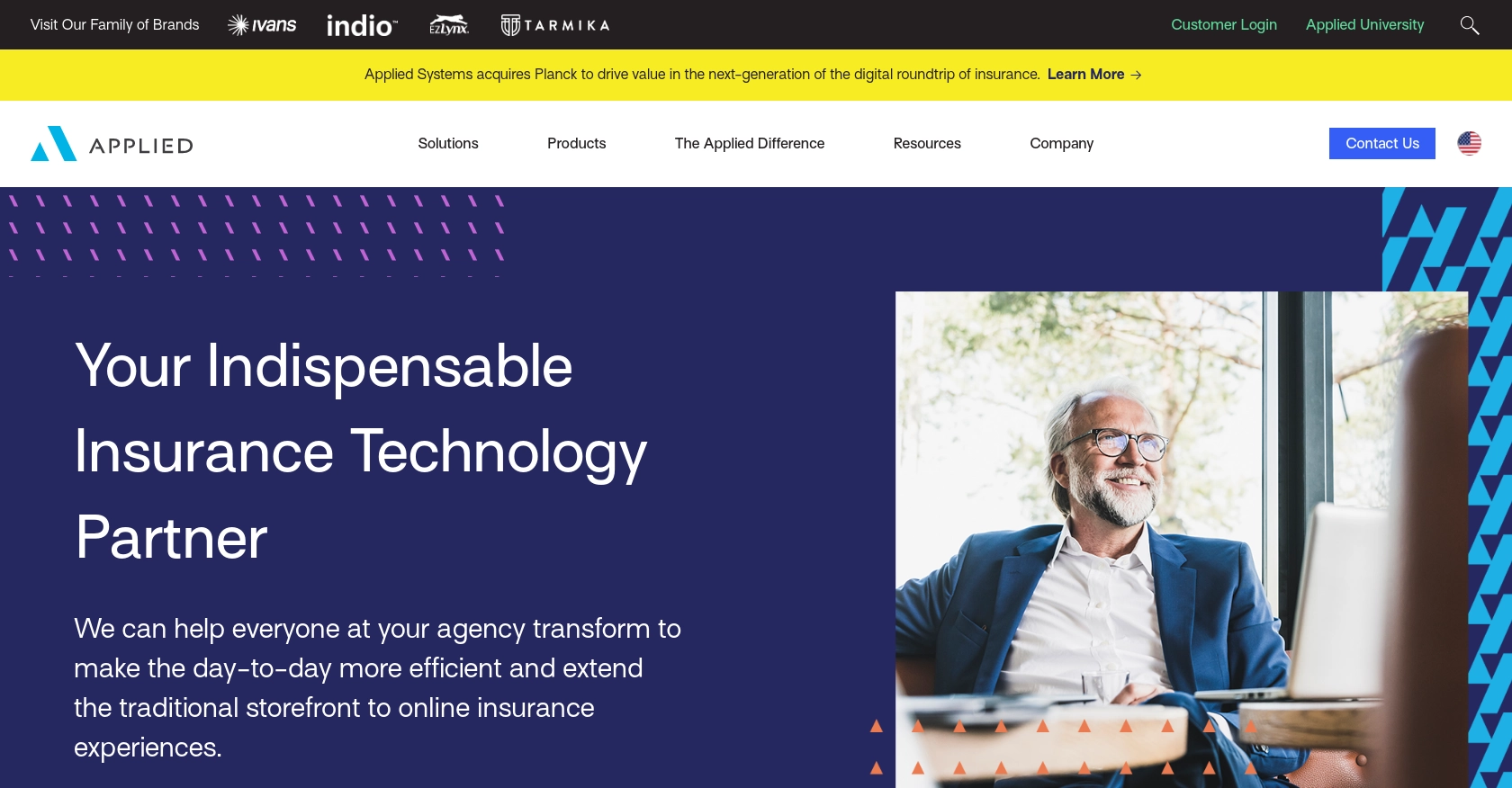
Introduction to Applied Epic API
Applied Epic is a comprehensive insurance management platform widely used by agencies to streamline their operations and enhance client relationships. It offers a robust set of tools for managing policies, claims, and customer interactions, making it a preferred choice for insurance professionals.
Integrating with the Applied Epic API allows developers to access and manage policy data programmatically, enabling automation and improved efficiency in handling insurance operations. For example, a developer might use the Applied Epic API to retrieve policy information for a specific client, facilitating seamless integration with other systems or applications.
Setting Up Your Applied Epic Test Account
Before you can start interacting with the Applied Epic API, you need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating an Applied Epic Developer Account
To begin, you need to sign up for a developer account on the Applied Developer Portal. This account will provide access to the necessary tools and resources for API integration.
- Visit the Applied Developer Portal.
- Follow the instructions to create a new account. You may need to verify your email address to complete the registration process.
Generating API Credentials for Applied Epic
Once your developer account is set up, you need to generate API credentials. These credentials are essential for authenticating your API requests.
- Log in to your Applied Developer account.
- Navigate to the API credentials section.
- Create a new application to obtain your client ID and client secret.
- Ensure you have the necessary permissions for accessing policy data.
Understanding Applied Epic Custom Authentication
The Applied Epic API uses a custom authentication method. Ensure you have your client ID and client secret ready, as these will be used to authenticate your requests.
For more detailed information on authentication, refer to the Applied Epic Documentation.
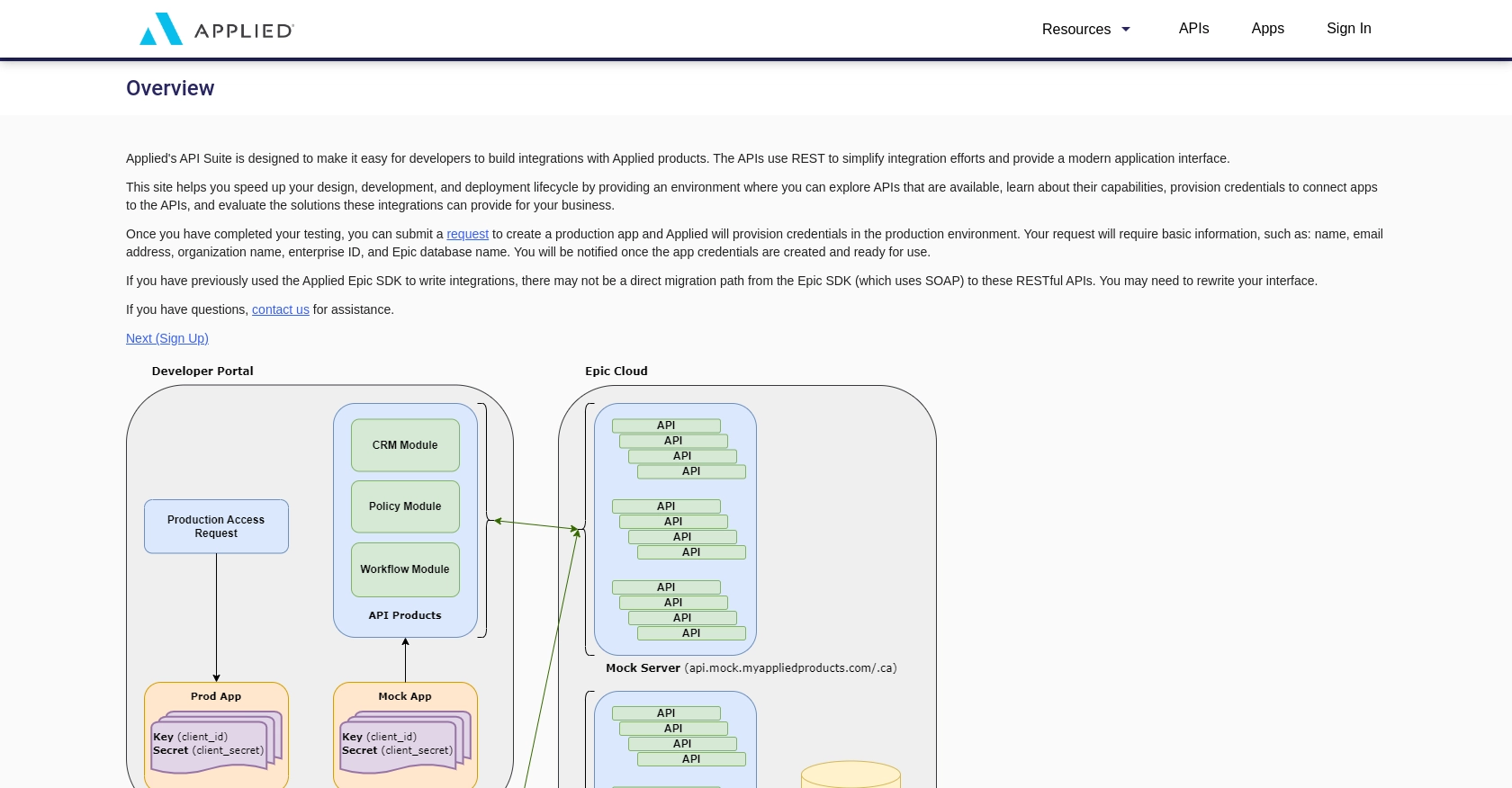
sbb-itb-96038d7
Making API Calls to Retrieve Policies with Applied Epic in PHP
To interact with the Applied Epic API and retrieve policy information, you'll need to set up your PHP environment and make HTTP requests to the API endpoints. This section will guide you through the process of making these API calls using PHP.
Setting Up Your PHP Environment for Applied Epic API Integration
Before you start coding, ensure your PHP environment is ready. You'll need PHP version 7.4 or later and the Composer package manager to handle dependencies.
- Ensure PHP is installed on your machine. You can download it from the official PHP website.
- Install Composer by following the instructions on the Composer website.
Installing Required PHP Packages for HTTP Requests
To make HTTP requests, you'll use the Guzzle HTTP client, a popular PHP library for sending HTTP requests.
composer require guzzlehttp/guzzle
Writing PHP Code to Fetch Policies from Applied Epic API
Now that your environment is set up, you can write the PHP code to interact with the Applied Epic API and retrieve policy data.
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$clientId = 'your_client_id';
$clientSecret = 'your_client_secret';
$baseUri = 'https://api.myappliedproducts.com/policy/v1/clients/';
$clientIdPath = 'your_client_id_here/policies';
try {
$response = $client->request('GET', $baseUri . $clientIdPath, [
'headers' => [
'Authorization' => 'Bearer ' . base64_encode("$clientId:$clientSecret"),
'Accept' => 'application/hal+json'
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['_embedded']['policies'] as $policy) {
echo "Policy Number: " . $policy['policyNumber'] . "\n";
echo "Description: " . $policy['description'] . "\n";
echo "Status: " . $policy['status']['description'] . "\n";
echo "Effective Date: " . $policy['effectiveOn'] . "\n";
echo "Expiration Date: " . $policy['expirationOn'] . "\n\n";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace your_client_id
and your_client_secret
with your actual credentials, and your_client_id_here
with the specific client ID you wish to query.
Verifying Successful API Requests and Handling Errors
After running the script, you should see the policy details printed in your terminal. If the request is successful, the data will match the policies available in your Applied Epic sandbox account.
In case of errors, the script will catch exceptions and display error messages. Common HTTP status codes to handle include:
- 400 Bad Request: The request was invalid. Check your parameters.
- 401 Unauthorized: Authentication failed. Verify your credentials.
- 403 Forbidden: You do not have permission to access the resource.
- 404 Not Found: The requested resource does not exist.
For more details on error handling, refer to the Applied Epic Documentation.
Best Practices for Applied Epic API Integration in PHP
Successfully integrating with the Applied Epic API requires attention to best practices to ensure security, efficiency, and maintainability. Here are some key considerations:
Securely Storing Credentials
Always store your API credentials securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults to manage sensitive information like client IDs and secrets.
Handling Rate Limits and Efficient API Usage
Be mindful of any rate limits imposed by the API. Although specific rate limit details are not provided, it's a good practice to implement exponential backoff strategies for retrying requests and to monitor your API usage to avoid hitting limits.
Standardizing and Transforming Data
When retrieving policy data, consider transforming and standardizing the data fields to match your application's requirements. This can help in maintaining consistency across different systems and integrations.
Utilizing Endgrate for Streamlined Integrations
For developers looking to simplify their integration processes, consider using Endgrate. By leveraging Endgrate's unified API, you can connect to multiple platforms, including Applied Epic, with ease. This allows you to focus on your core product while outsourcing the complexities of integration management.
Visit Endgrate to learn more about how you can enhance your integration capabilities and streamline your development workflow.
Read More
Ready to get started?