Using the Keap API to Get Companies (with PHP examples)
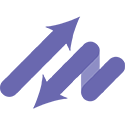
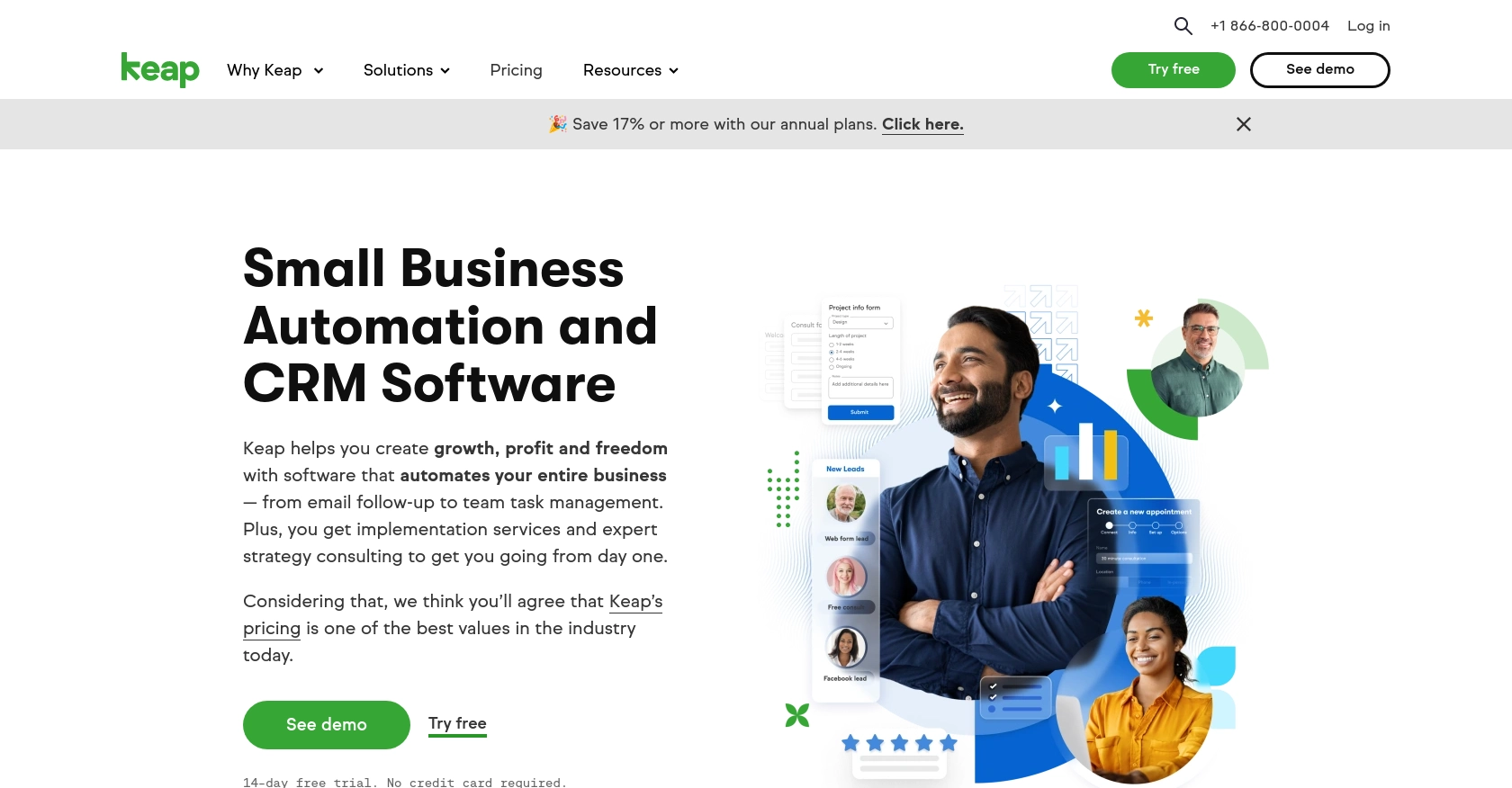
Introduction to Keap API Integration
Keap, formerly known as Infusionsoft, is a powerful CRM and marketing automation platform designed to help small businesses manage customer relationships and streamline their sales processes. With features like contact management, email marketing, and e-commerce, Keap provides a comprehensive solution for businesses looking to enhance their customer engagement strategies.
Developers may want to integrate with Keap's API to access and manage company data, enabling them to automate workflows and improve data synchronization between platforms. For example, retrieving company information using the Keap API can help developers create custom dashboards that provide insights into customer interactions and sales performance.
Setting Up Your Keap Developer Account and Sandbox Environment
Before you can start integrating with the Keap API, you'll need to set up a developer account and create a sandbox environment. This will allow you to test your API interactions without affecting live data. Follow these steps to get started:
Register for a Keap Developer Account
- Visit the Keap Developer Portal and register for a developer account.
- Fill out the necessary information and submit your application to become a developer partner.
- Once your account is approved, log in to the developer portal to access your dashboard.
Create a Keap Sandbox App
- In your developer dashboard, navigate to the section for creating a new sandbox app.
- Follow the prompts to set up your sandbox environment. This will provide you with a safe space to test API calls.
- Note down your sandbox app credentials, including the client ID and client secret, as you'll need these for OAuth authentication.
Set Up OAuth Authentication for Keap API
Keap uses OAuth 2.0 for authentication, which requires you to create an app and obtain authorization credentials. Here's how to set it up:
- In your sandbox app settings, navigate to the OAuth section.
- Create a new OAuth application by providing the necessary details, such as the application name and redirect URI.
- Once created, you'll receive a client ID and client secret. Keep these credentials secure, as they are essential for authenticating API requests.
With your developer account, sandbox app, and OAuth credentials set up, you're ready to start making API calls to Keap. In the next section, we'll explore how to use PHP to interact with the Keap API and retrieve company data.
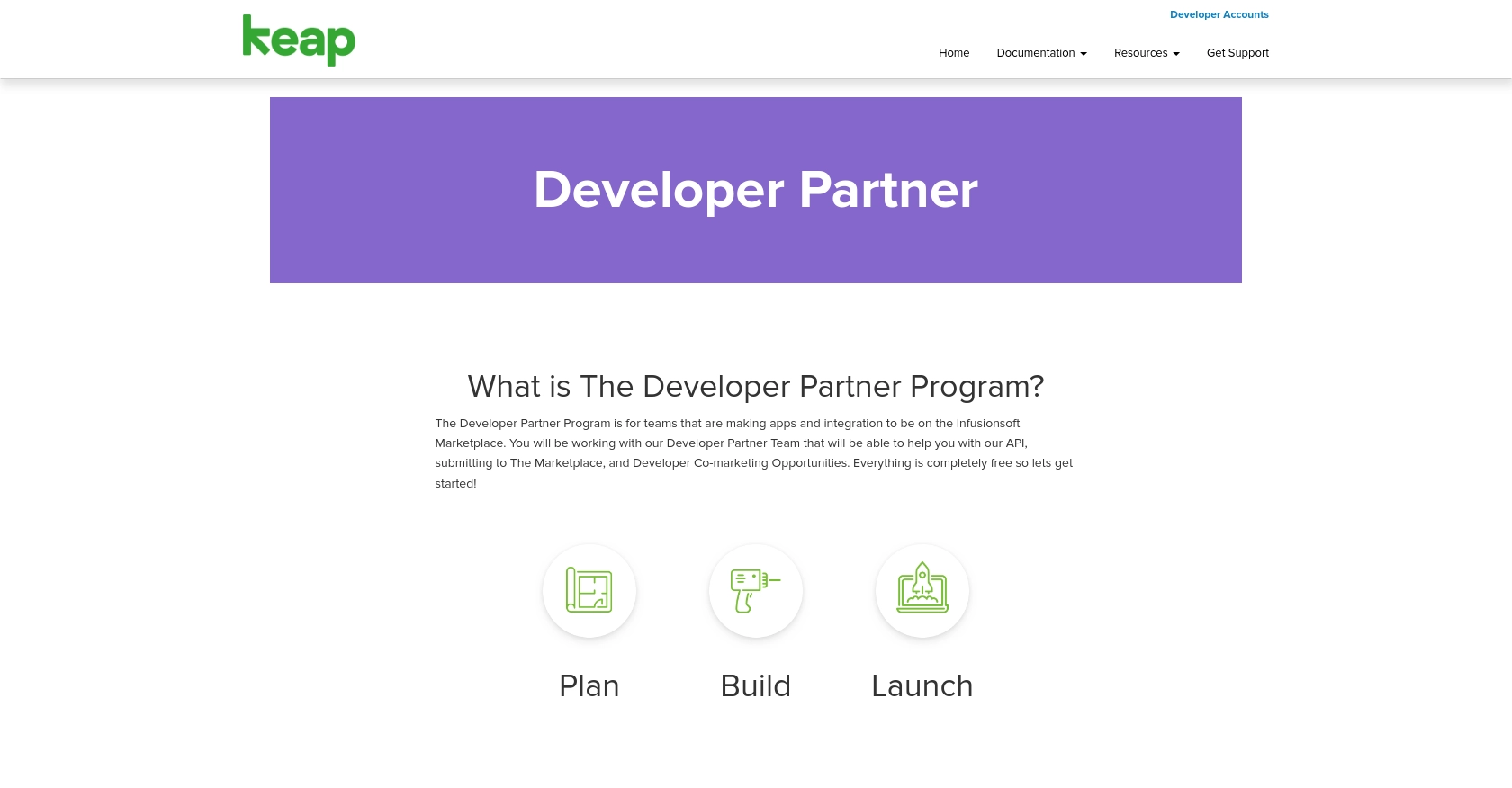
sbb-itb-96038d7
Making API Calls to Retrieve Company Data from Keap Using PHP
To interact with the Keap API and retrieve company data, you'll need to use PHP to make HTTP requests. This section will guide you through the process, including setting up your environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Keap API Integration
Before making API calls, ensure you have the following prerequisites:
- PHP 7.4 or later installed on your machine.
- Composer, the PHP package manager, to manage dependencies.
Install the Guzzle HTTP client, which simplifies making HTTP requests in PHP, by running the following command:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Companies from Keap API
With your environment set up, you can now write the PHP code to make a GET request to the Keap API and retrieve company data. Create a file named get_keap_companies.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your OAuth access token
try {
$response = $client->request('GET', 'https://api.infusionsoft.com/crm/rest/v1/companies', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
],
]);
$data = json_decode($response->getBody(), true);
foreach ($data['companies'] as $company) {
echo 'Company Name: ' . $company['company_name'] . '<br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
Replace Your_Access_Token
with the OAuth access token you obtained during the setup process. This token is crucial for authenticating your API requests.
Running the PHP Script and Verifying API Response
To execute the script, run the following command in your terminal:
php get_keap_companies.php
If successful, the script will output the names of the companies retrieved from the Keap API. Verify the response by checking the data against your sandbox environment.
Handling Errors and Understanding Keap API Error Codes
When making API calls, it's essential to handle potential errors gracefully. The Keap API may return various error codes, such as:
- 401 Unauthorized: Indicates an issue with your access token. Ensure it's valid and hasn't expired.
- 404 Not Found: The requested resource doesn't exist. Check the endpoint URL.
- 500 Internal Server Error: A server-side issue. Retry the request later.
Implement error handling in your code to manage these scenarios and provide meaningful feedback to users.
Best Practices for Keap API Integration and Data Management
When integrating with the Keap API, it's crucial to follow best practices to ensure secure and efficient data management. Here are some recommendations:
- Securely Store OAuth Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Instead, use environment variables or secure vaults.
- Handle Rate Limiting: Be aware of Keap's API rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that data retrieved from Keap is transformed and standardized to match your application's data schema. This will facilitate seamless data integration and analysis.
Enhancing Integration Efficiency with Endgrate
Building and maintaining multiple integrations can be time-consuming and resource-intensive. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Keap. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product development while outsourcing integration complexities to Endgrate.
- Build Once, Use Everywhere: Develop a single integration for each use case and leverage it across multiple platforms.
- Improve Customer Experience: Offer an intuitive and seamless integration experience to your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate's website and discover the benefits of a unified integration solution.
Read More
Ready to get started?