Using the Active Campaign API to Get Users in PHP
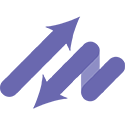
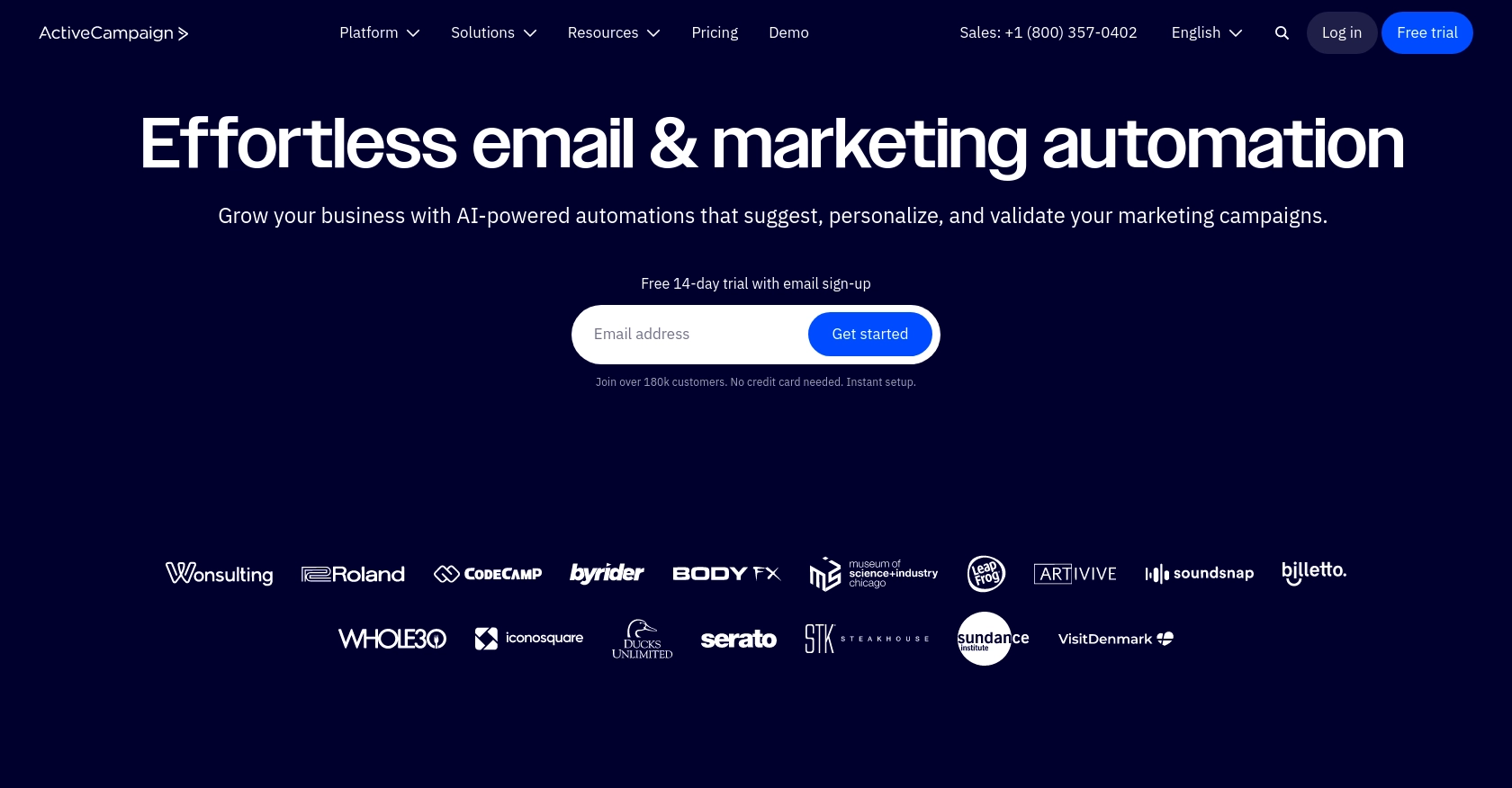
Introduction to Active Campaign API
Active Campaign is a powerful marketing automation platform that offers a suite of tools to help businesses engage with their audience through email marketing, CRM, and sales automation. Its robust features make it a popular choice for businesses looking to enhance their marketing efforts and streamline customer interactions.
Developers may want to integrate with Active Campaign's API to access and manage user data, enabling personalized marketing strategies and improving customer relationship management. For example, a developer might use the Active Campaign API to retrieve user information and tailor marketing campaigns based on user preferences and behaviors.
Setting Up Your Active Campaign Test Account
Before you can start using the Active Campaign API to retrieve user data, you'll need to set up a test account. This will allow you to safely experiment with the API without affecting your live data.
Create an Active Campaign Account
If you don't already have an Active Campaign account, you can sign up for a free trial on their website. This trial will give you access to all the features you need to test the API.
- Visit the Active Campaign website.
- Click on the "Start Your Free Trial" button.
- Follow the on-screen instructions to create your account.
Accessing the Developer Settings
Once your account is set up, you'll need to access the developer settings to obtain your API key and base URL, which are essential for authenticating your API requests.
- Log in to your Active Campaign account.
- Navigate to the "Settings" section in the bottom left corner of the dashboard.
- Click on the "Developer" tab to find your API key and base URL.
Generating Your API Key
Active Campaign uses API key-based authentication. Each user in your account has a unique API key that you will use to authenticate API requests.
- In the "Developer" tab, locate your API key.
- Copy the API key and store it securely. Ensure it is not exposed in client-side code.
For more details on authentication, refer to the Active Campaign Authentication Documentation.
Understanding the Base URL
Your API requests will be made to a base URL specific to your account. This URL is found in the "Developer" tab and typically follows the format: https://
.
Ensure you use the correct base URL for your account to avoid any connectivity issues. More information can be found in the Base URL Documentation.
Testing Your API Setup
With your API key and base URL ready, you can now test your setup by making a simple API call to list all users. This will confirm that your authentication is working correctly.
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://youraccountname.api-us1.com/api/3/users',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
CURLOPT_HTTPHEADER => array(
'accept: application/json',
'Api-Token: your_api_key'
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo 'cURL Error #:' . $err;
} else {
echo $response;
}
Replace your_api_key
with your actual API key. This script should return a list of users in JSON format if everything is set up correctly.
For more information on listing users, visit the List All Users Documentation.
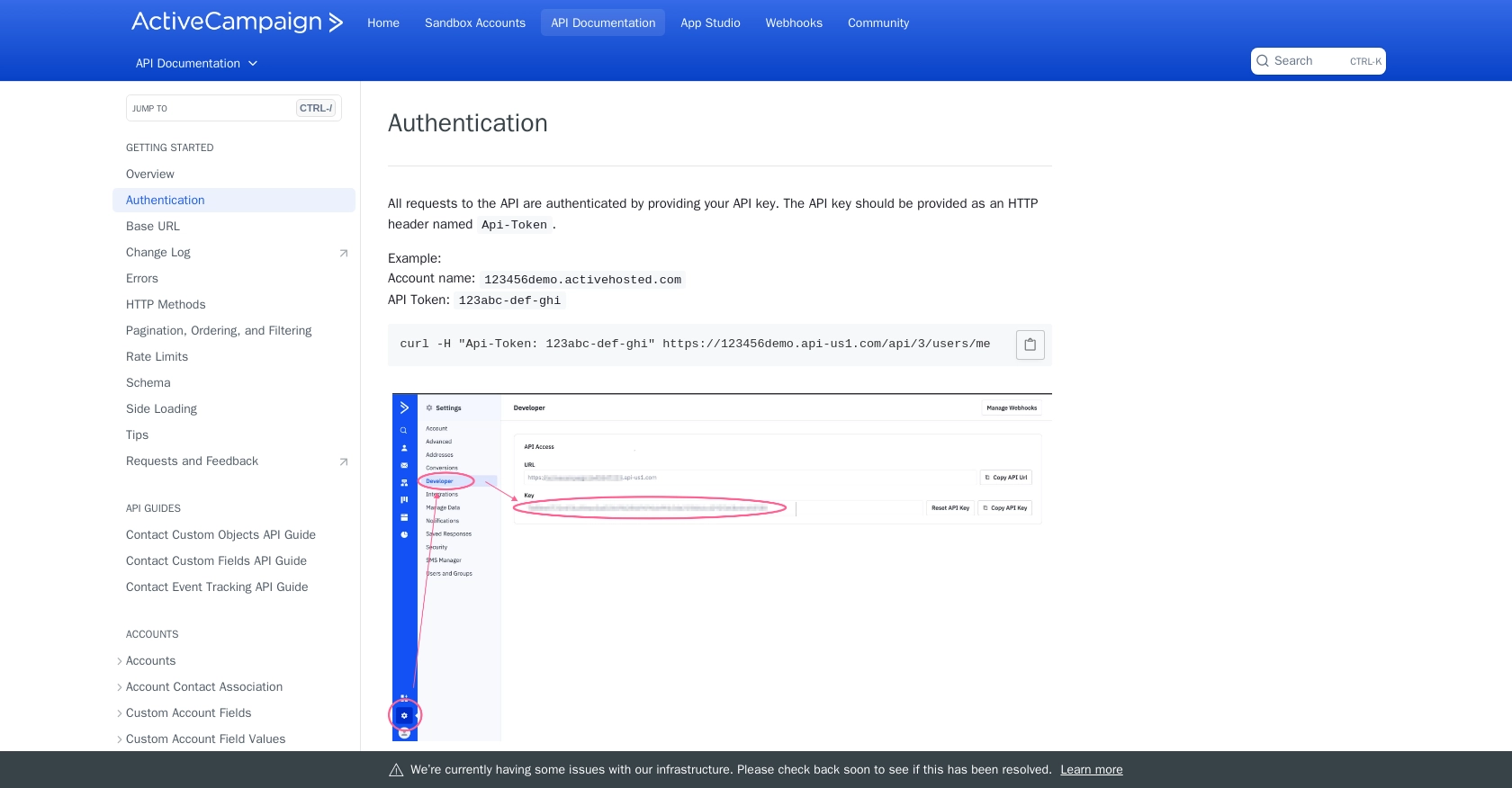
sbb-itb-96038d7
Making API Calls to Retrieve Users with Active Campaign in PHP
To effectively interact with the Active Campaign API using PHP, you'll need to ensure your environment is properly set up. This section will guide you through the necessary steps to make API calls and retrieve user data.
Setting Up Your PHP Environment for Active Campaign API
Before making API calls, ensure you have PHP installed on your machine. You can verify this by running php -v
in your terminal. Additionally, ensure that the cURL extension is enabled, as it is essential for making HTTP requests.
Installing Required PHP Dependencies
For this tutorial, we'll use PHP's built-in cURL functions to interact with the Active Campaign API. Ensure that your PHP installation includes the cURL extension. If not, you may need to enable it in your php.ini
file.
Example Code to Retrieve Users from Active Campaign
Below is a sample PHP script that demonstrates how to retrieve a list of users from Active Campaign using the API. This script uses cURL to send a GET request to the Active Campaign API endpoint.
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://youraccountname.api-us1.com/api/3/users',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
CURLOPT_HTTPHEADER => array(
'accept: application/json',
'Api-Token: your_api_key'
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo 'cURL Error #:' . $err;
} else {
echo $response;
}
Replace your_api_key
with your actual API key obtained from the Active Campaign Developer settings. This script will return a JSON response containing user data if the request is successful.
Understanding the API Response
The API response will include a list of users in JSON format. Each user object contains details such as username
, firstName
, lastName
, and email
. You can parse this JSON data in PHP using json_decode()
to access individual user details.
Verifying Successful API Requests
To ensure your API request was successful, check the HTTP status code returned by the API. A status code of 200 indicates success. You can also verify the retrieved data by comparing it with the user information available in your Active Campaign account.
Handling API Errors and Rate Limits
If the API request fails, the cURL error will be displayed. Common issues include incorrect API keys or base URLs. Additionally, be mindful of the rate limit of 5 requests per second per account as specified in the Active Campaign Rate Limits Documentation. Implementing retry logic or exponential backoff can help manage rate limiting effectively.
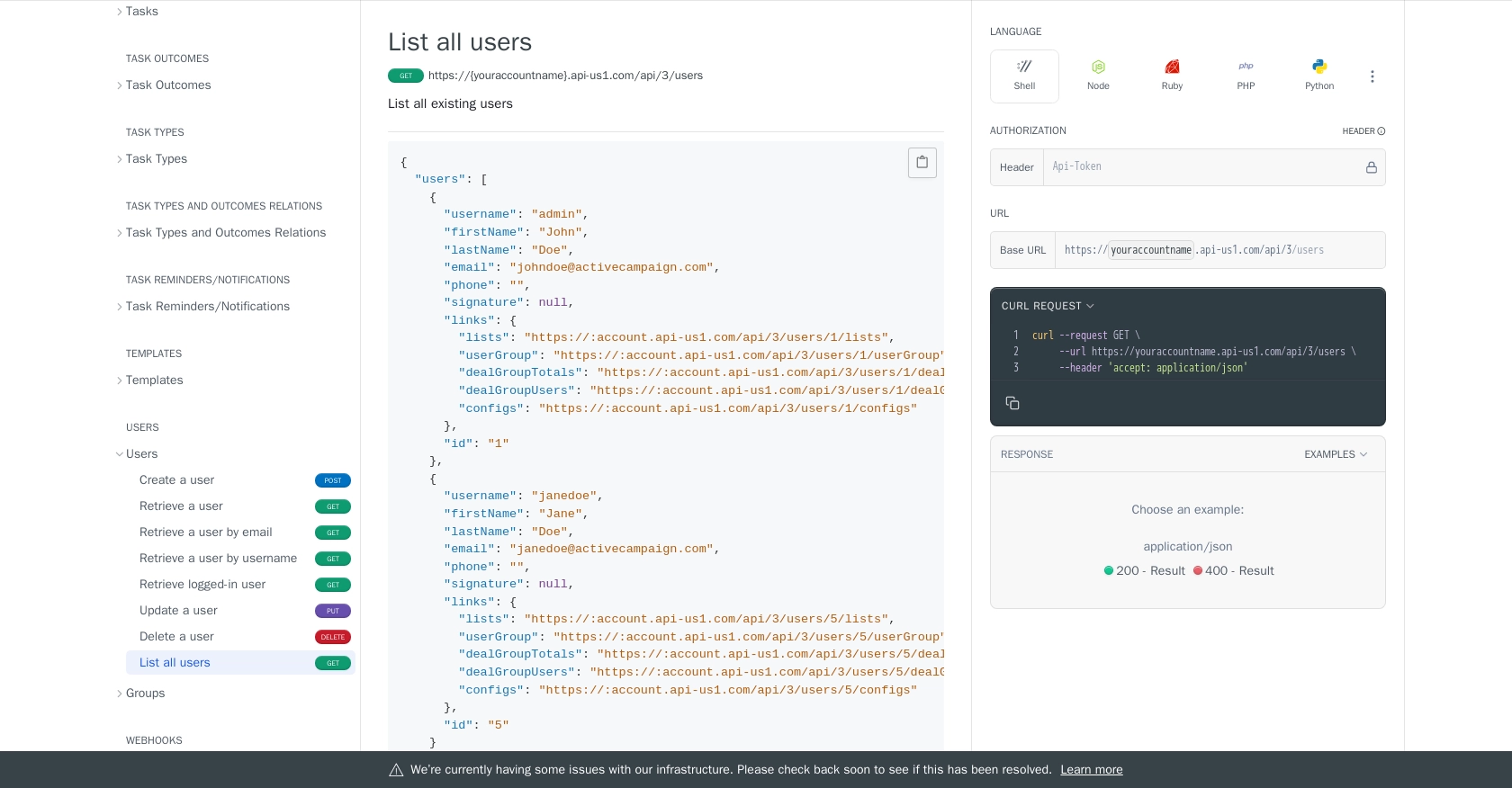
Best Practices for Using the Active Campaign API in PHP
When working with the Active Campaign API, it's crucial to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Securely Store API Credentials: Always keep your API key confidential. Avoid exposing it in client-side code and consider using environment variables or secure vaults to store sensitive information.
- Implement Rate Limiting Strategies: Be aware of the rate limit of 5 requests per second per account. Implementing retry logic or exponential backoff can help manage rate limiting effectively. For more details, refer to the Active Campaign Rate Limits Documentation.
- Handle API Errors Gracefully: Ensure your application can handle errors by checking HTTP status codes and implementing error handling logic. This will improve the reliability of your integration.
- Optimize Data Handling: Use PHP's
json_decode()
to parse JSON responses efficiently. Consider caching frequently accessed data to reduce API calls and improve performance.
Conclusion and Call to Action for Using Endgrate
Integrating with the Active Campaign API using PHP can significantly enhance your marketing automation and CRM capabilities. By following the steps outlined in this guide, you can efficiently retrieve and manage user data, enabling personalized marketing strategies and improved customer interactions.
If you're looking to streamline your integration processes further, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that simplifies the integration experience, making it easier to connect with multiple platforms, including Active Campaign.
Visit Endgrate to learn more about how you can enhance your integration capabilities and provide a seamless experience for your customers.
Read More
- https://endgrate.com/provider/activecampaign
- https://developers.activecampaign.com/reference/authentication
- https://developers.activecampaign.com/reference/url
- https://developers.activecampaign.com/reference/pagination
- https://developers.activecampaign.com/reference/rate-limits
- https://developers.activecampaign.com/reference/list-all-users
Ready to get started?