How to Get Product Variants with the BigCommerce API in PHP
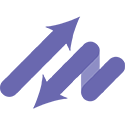
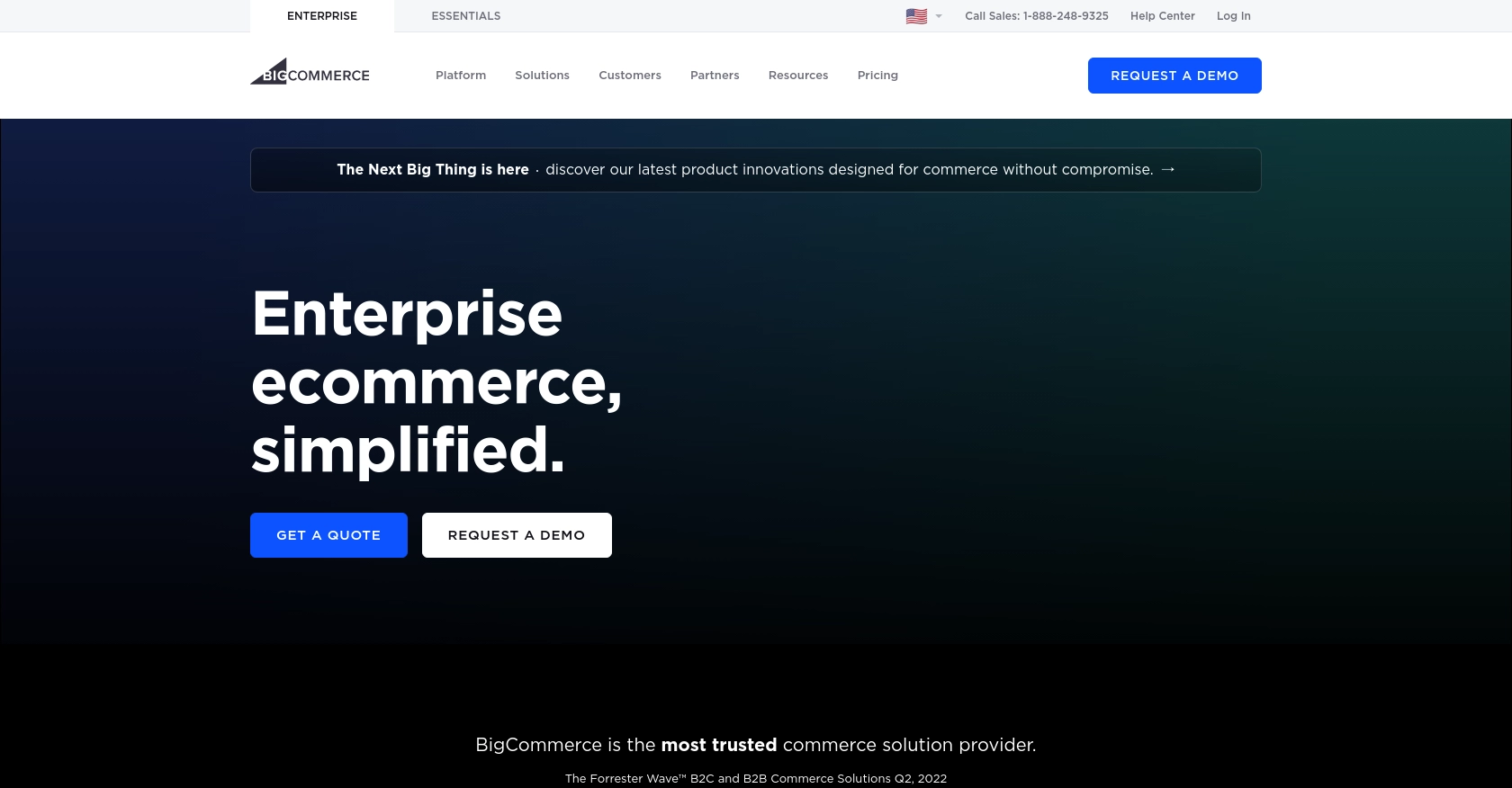
Introduction to BigCommerce API for Product Variants
BigCommerce is a robust e-commerce platform that empowers businesses to build, innovate, and grow their online presence. It offers a comprehensive suite of tools for managing products, orders, and customer relationships, making it a popular choice for businesses of all sizes.
For developers, integrating with the BigCommerce API can significantly enhance the functionality of an online store. One common use case is retrieving product variants, which are different versions of a product, each with its own SKU. This is particularly useful for managing inventory and displaying detailed product options to customers.
In this article, we will explore how to use PHP to interact with the BigCommerce API to get product variants. This integration can help developers automate inventory management and provide a seamless shopping experience for customers.
Setting Up Your BigCommerce Test/Sandbox Account for API Integration
Before diving into the integration process, it's essential to set up a BigCommerce test or sandbox account. This environment allows developers to experiment with API calls without affecting a live store, ensuring a safe and controlled testing space.
Creating a BigCommerce Sandbox Account
To begin, you'll need to create a sandbox account on BigCommerce. Follow these steps:
- Visit the BigCommerce Sandbox page and sign up for a free sandbox store.
- Fill in the required details and submit the form to create your sandbox account.
- Once your account is set up, log in to access the BigCommerce control panel.
Generating API Credentials for BigCommerce
With your sandbox account ready, the next step is to generate API credentials. These credentials will allow you to authenticate your API requests:
- Navigate to the Advanced Settings in the BigCommerce control panel.
- Select API Accounts and click on Create API Account.
- Choose V2/V3 API Token as the type of API account.
- Provide a name for your API account and set the necessary permissions for accessing product variants.
- Click Save to generate your API credentials, including the
X-Auth-Token
. - Copy and securely store the
Client ID
,Client Secret
, andAccess Token
as they will be used for authentication in your PHP code.
For more detailed information on authentication, refer to the BigCommerce Authentication Documentation.
Configuring OAuth Scopes for API Access
Ensure that your API account has the correct OAuth scopes configured to access product variants:
- Go to the OAuth Scopes section in your API account settings.
- Select the scopes that are relevant to product management, such as Products and Catalog.
- Save your changes to apply the new scopes.
With your sandbox account and API credentials set up, you're now ready to start making API calls to retrieve product variants using PHP.
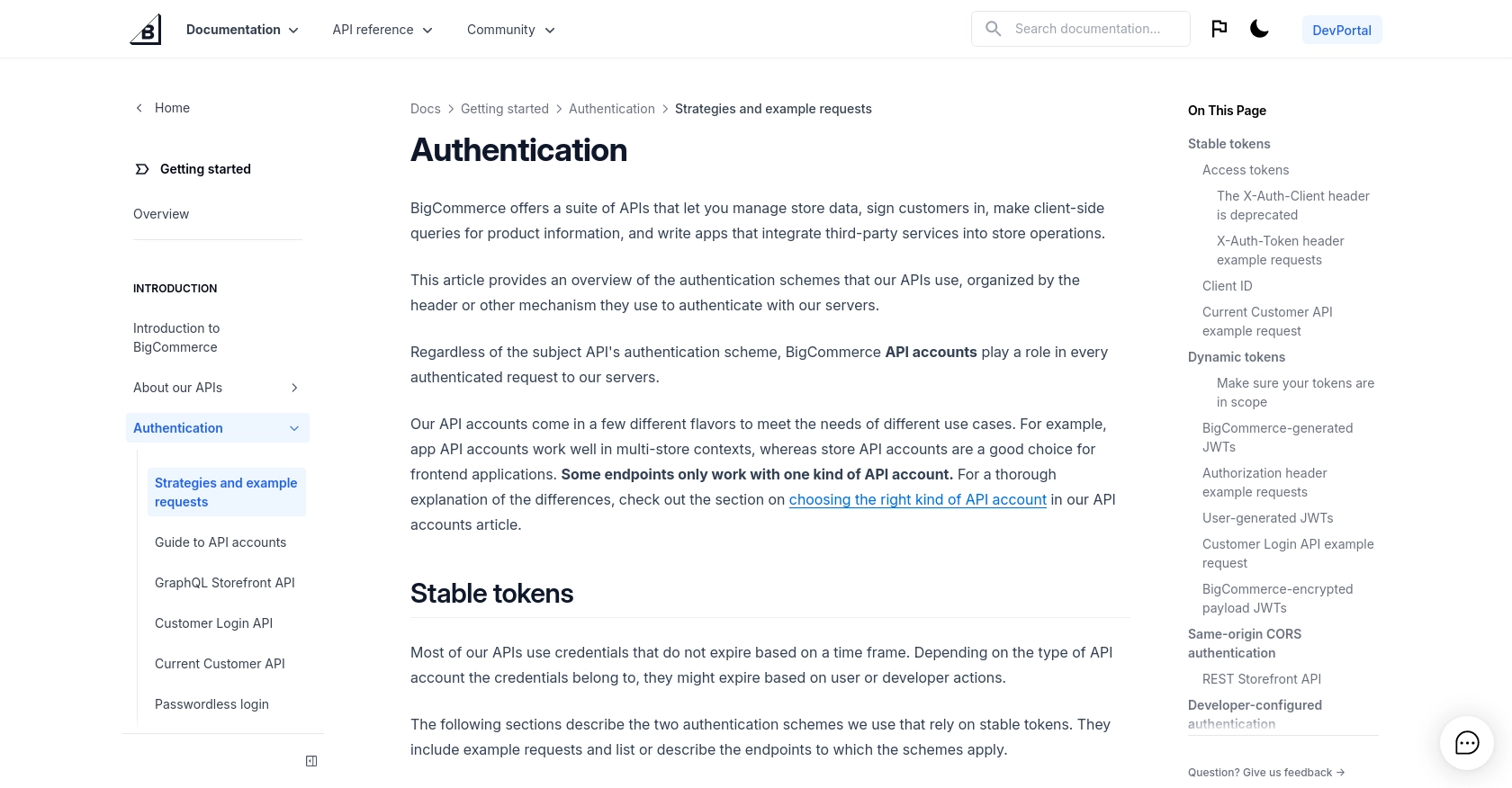
sbb-itb-96038d7
Making API Calls to Retrieve Product Variants from BigCommerce Using PHP
With your BigCommerce sandbox account and API credentials ready, you can now proceed to make API calls to retrieve product variants. This section will guide you through the process of setting up your PHP environment and executing the necessary API requests.
Setting Up Your PHP Environment for BigCommerce API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need the following:
- PHP 7.4 or later
- cURL extension enabled
To check your PHP version and installed extensions, run the following command in your terminal:
php -v
Ensure that cURL is enabled by checking your php.ini
file or running:
php -m | grep curl
Installing Necessary PHP Dependencies for BigCommerce API
To handle HTTP requests, you can use the built-in cURL functions in PHP. No additional libraries are required, but you can use Composer to manage dependencies if needed.
Example Code to Retrieve Product Variants from BigCommerce
Below is an example PHP script that demonstrates how to retrieve product variants using the BigCommerce API:
<?php
// Set your API credentials
$store_hash = 'your_store_hash';
$access_token = 'your_access_token';
// Set the API endpoint
$product_id = 123; // Replace with your product ID
$endpoint = "https://api.bigcommerce.com/stores/{$store_hash}/v3/catalog/products/{$product_id}/variants";
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'X-Auth-Token: ' . $access_token,
'Accept: application/json',
]);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Parse the JSON response
$data = json_decode($response, true);
print_r($data);
}
// Close cURL session
curl_close($ch);
?>
Replace your_store_hash
and your_access_token
with your actual store hash and access token. Also, update $product_id
with the ID of the product whose variants you wish to retrieve.
Verifying the Success of Your API Request
After running the script, you should see the product variants data printed on your screen. This confirms that the API call was successful. You can further verify by checking the BigCommerce control panel to ensure the data matches.
Handling Errors and Understanding BigCommerce API Response Codes
It's crucial to handle potential errors when making API requests. The BigCommerce API may return various HTTP status codes:
- 200 OK: The request was successful.
- 404 Not Found: The specified product or variant does not exist.
- 401 Unauthorized: Authentication failed. Check your access token.
For more detailed error handling, refer to the BigCommerce API documentation.
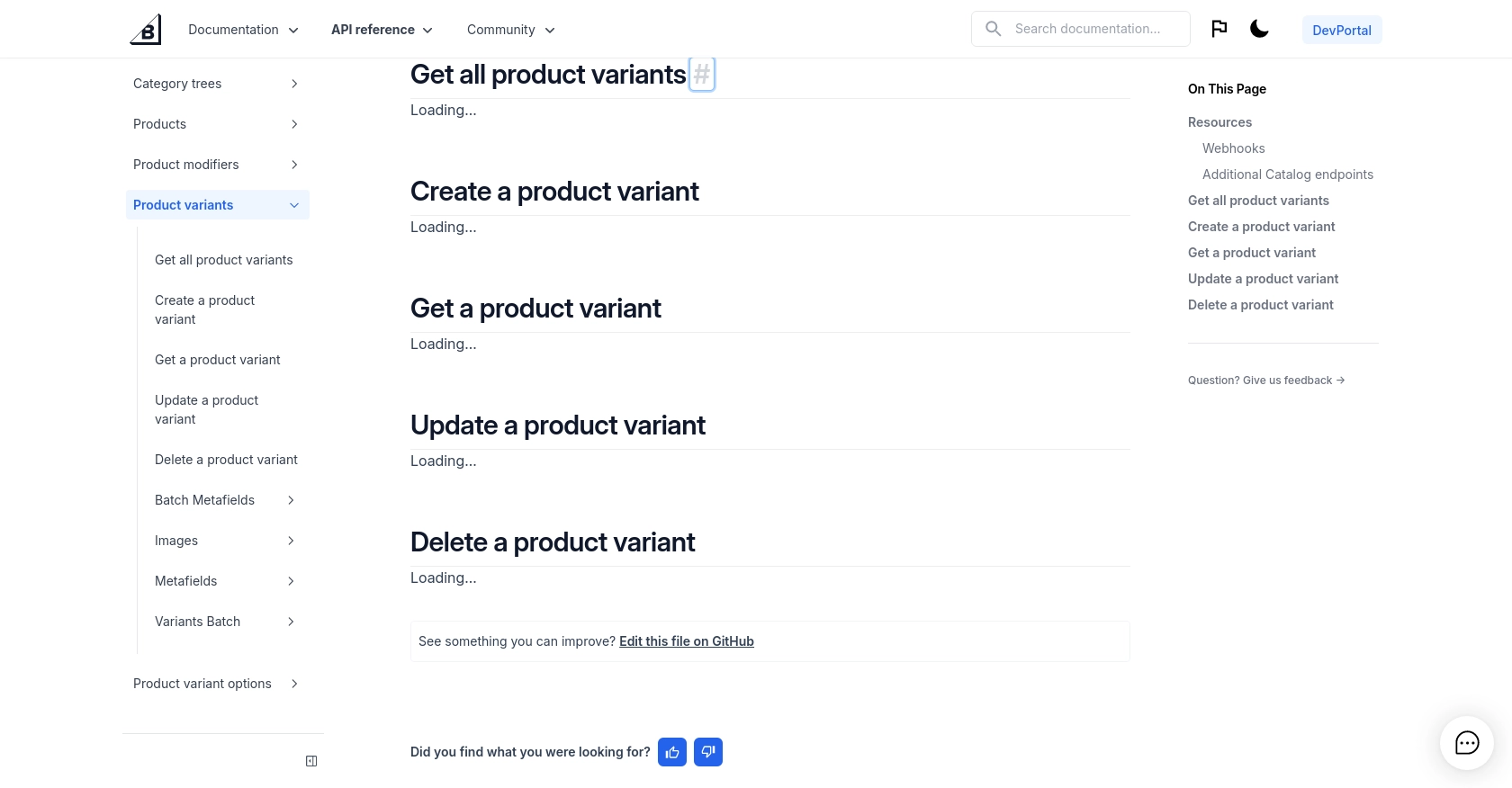
Conclusion and Best Practices for BigCommerce API Integration in PHP
Integrating with the BigCommerce API to retrieve product variants using PHP can significantly enhance your e-commerce platform's capabilities. By automating inventory management and providing detailed product options, you can offer a seamless shopping experience to your customers.
Best Practices for Secure and Efficient BigCommerce API Usage
- Secure Storage of Credentials: Always store your API credentials securely. Consider using environment variables or a secure vault to keep your
Client ID
,Client Secret
, andAccess Token
safe. - Handle Rate Limiting: Be mindful of BigCommerce's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from the API is transformed and standardized according to your application's requirements for consistency and accuracy.
Enhance Your Integration Strategy with Endgrate
While integrating with the BigCommerce API can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including BigCommerce.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration strategy by visiting Endgrate.
Read More
Ready to get started?