How to Get Sale Orders with the Xero API in Javascript
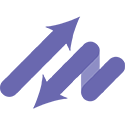
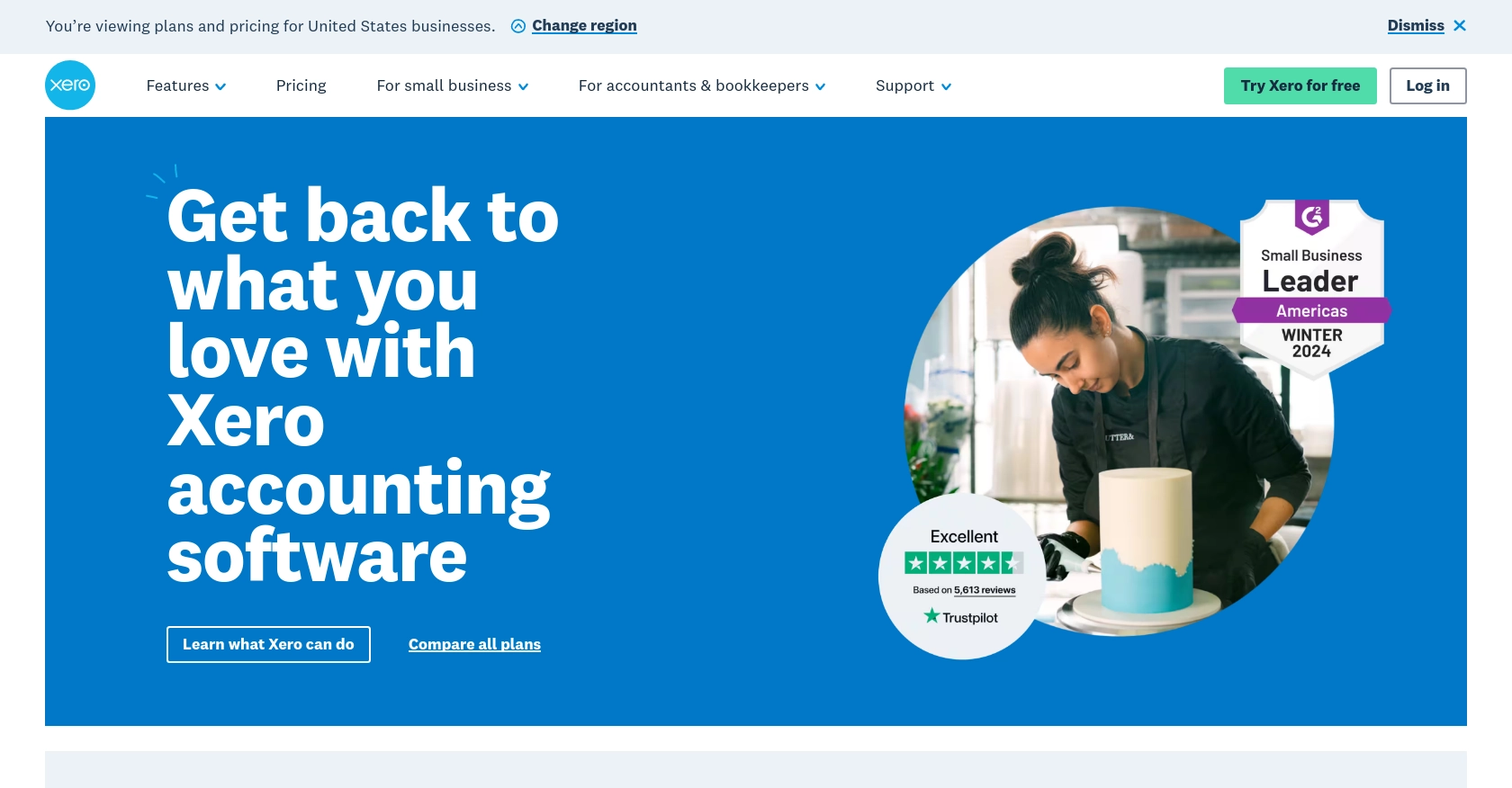
Introduction to Xero API Integration
Xero is a powerful cloud-based accounting software platform designed to help businesses manage their finances efficiently. With features like invoicing, payroll, and expense tracking, Xero is a popular choice for small to medium-sized enterprises looking to streamline their financial operations.
Integrating with Xero's API allows developers to automate and enhance financial workflows, such as retrieving sales orders. For example, a developer might use the Xero API to automatically pull sales order data into a custom dashboard, providing real-time insights into sales performance and inventory management.
Setting Up Your Xero Sandbox Account for API Integration
Before you can start interacting with the Xero API using JavaScript, you'll need to set up a sandbox account. This allows you to test your integration without affecting live data. Xero provides a demo company that you can use for this purpose.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. If you don't have one, follow these steps:
- Visit the Xero Developer Portal and sign up for a free account.
- Once registered, log in to your developer account.
Accessing the Xero Demo Company
After logging in, you can access the Xero demo company, which provides a safe environment to test your API calls:
- Navigate to the "My Apps" section in your Xero developer dashboard.
- Select "Demo Company" from the available options.
Creating a Xero App for OAuth 2.0 Authentication
Xero uses OAuth 2.0 for authentication, which requires you to create an app to obtain the necessary credentials:
- In the "My Apps" section, click on "New App."
- Fill in the required details, such as the app name and company URL.
- Set the redirect URI, which is where users will be redirected after authentication. This can be a local URL for testing purposes.
- Save your app to generate the client ID and client secret.
Configuring OAuth 2.0 Scopes
Scopes define the level of access your app has to the Xero API. For retrieving sales orders, ensure you have the necessary scopes:
- Navigate to the "Scopes" section of your app settings.
- Select the appropriate scopes related to sales orders and accounting data.
For more details on OAuth 2.0 and scopes, refer to the Xero OAuth 2.0 Scopes Documentation.
Obtaining Access Tokens
With your app set up, you can now obtain access tokens to authenticate API requests:
- Use the client ID and client secret to initiate the OAuth 2.0 authorization flow.
- Follow the standard authorization code flow to obtain an access token. Detailed steps can be found in the Xero OAuth 2.0 Authorization Flow Guide.
Keep your access tokens secure and refresh them as needed to maintain access.
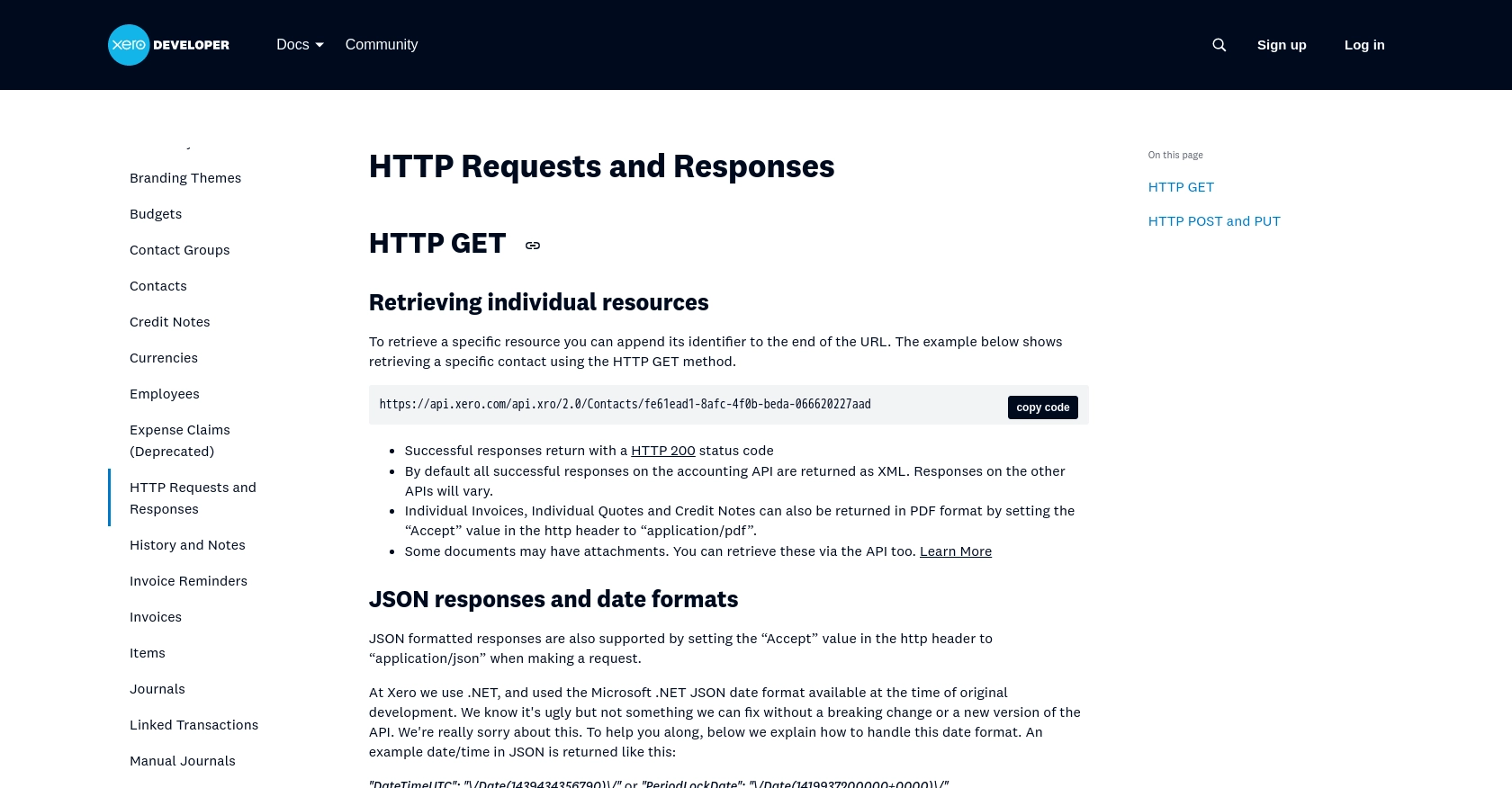
sbb-itb-96038d7
Making API Calls to Retrieve Sales Orders with Xero API in JavaScript
To interact with the Xero API and retrieve sales orders using JavaScript, you'll need to ensure your development environment is properly set up. This section will guide you through the necessary steps, including setting up JavaScript, installing dependencies, and making the API call.
Setting Up Your JavaScript Environment for Xero API
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment needed to execute JavaScript code outside a web browser.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
andnpm -v
in your terminal to check the versions.
Installing Required Dependencies
You'll need the axios
library to handle HTTP requests. Install it using npm:
npm install axios
Writing JavaScript Code to Fetch Sales Orders from Xero
With your environment set up, you can now write the JavaScript code to fetch sales orders from Xero. Create a file named getSalesOrders.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.xero.com/api.xro/2.0/Quotes';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Accept': 'application/json'
};
// Function to get sales orders
async function getSalesOrders() {
try {
const response = await axios.get(endpoint, { headers });
const salesOrders = response.data.Quotes;
console.log(salesOrders);
} catch (error) {
console.error('Error fetching sales orders:', error.response.data);
}
}
// Execute the function
getSalesOrders();
Replace YOUR_ACCESS_TOKEN
with the access token obtained from the OAuth 2.0 authorization process.
Running the JavaScript Code
Execute the script using Node.js:
node getSalesOrders.js
If successful, the console will display the sales orders retrieved from the Xero API.
Handling Errors and Validating API Responses
It's crucial to handle potential errors when making API calls. The code above includes a try-catch
block to catch and log errors. Common error codes include:
- 400 Bad Request: The request was invalid. Check the request parameters.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 403 Forbidden: The request is not allowed. Check your app's scopes and permissions.
For more information on error codes, refer to the Xero API Requests and Responses Documentation.
By following these steps, you can efficiently retrieve sales orders from Xero using JavaScript, allowing you to integrate this data into your applications and workflows seamlessly.
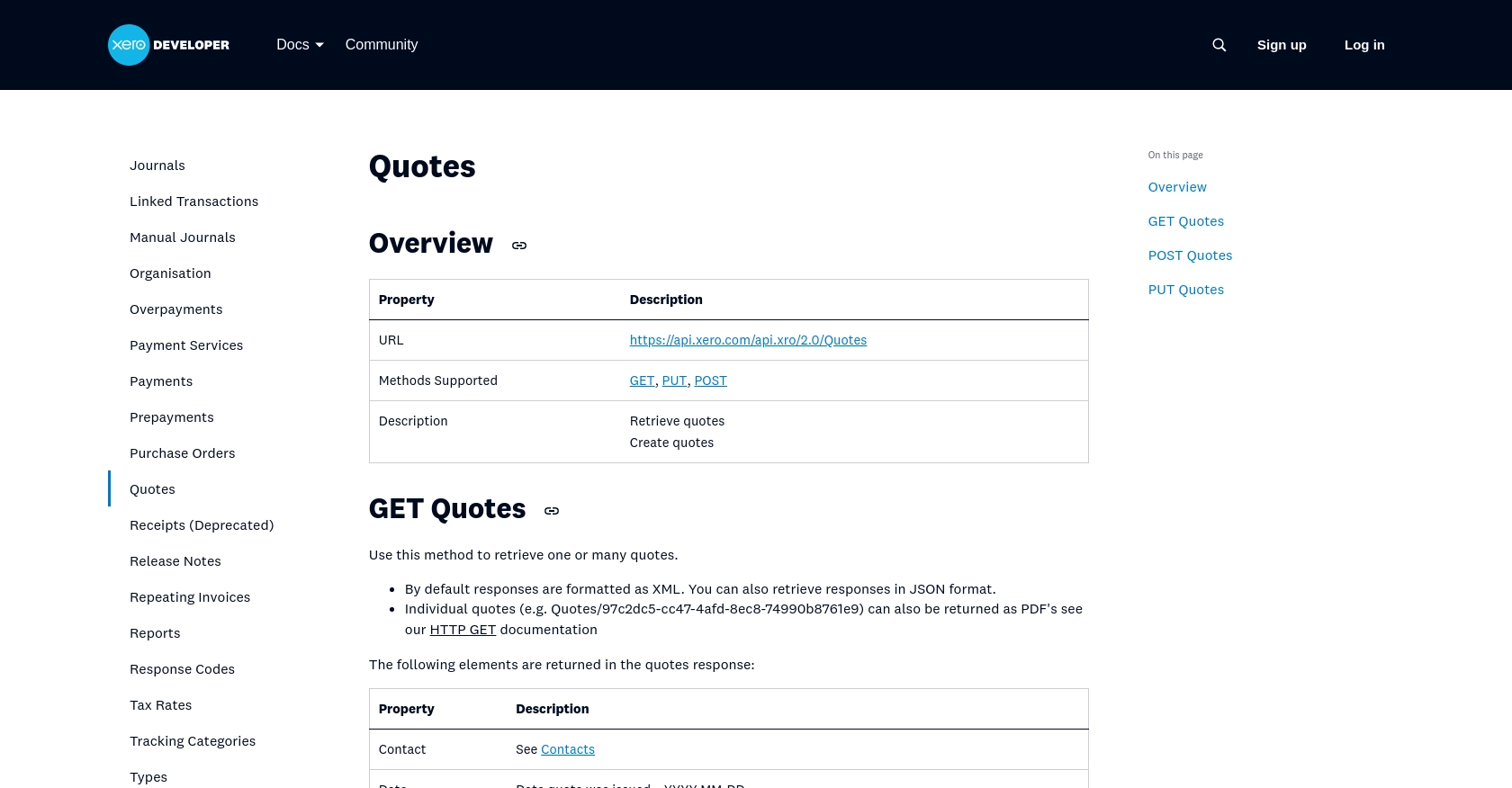
Conclusion and Best Practices for Xero API Integration
Integrating with the Xero API using JavaScript provides a powerful way to automate and enhance financial workflows within your applications. By following the steps outlined in this guide, you can efficiently retrieve sales orders and incorporate them into your business processes.
Best Practices for Secure and Efficient Xero API Usage
- Secure Credential Storage: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Xero's API imposes rate limits to ensure fair usage. Be mindful of these limits and implement retry logic or exponential backoff strategies to handle rate limit errors gracefully. For more details, refer to the Xero API Rate Limits Documentation.
- Data Transformation and Standardization: When integrating sales order data, ensure that you transform and standardize fields to match your application's data model, enhancing data consistency and usability.
Streamlining Integrations with Endgrate
While building integrations with Xero is a valuable skill, managing multiple integrations can be time-consuming. Endgrate offers a unified API endpoint that simplifies the integration process across various platforms, including Xero. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover how you can build once for each use case instead of multiple times for different integrations.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/quotes
Ready to get started?