How to Get Purchase Orders with the Quickbooks API in Python
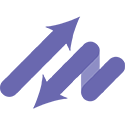
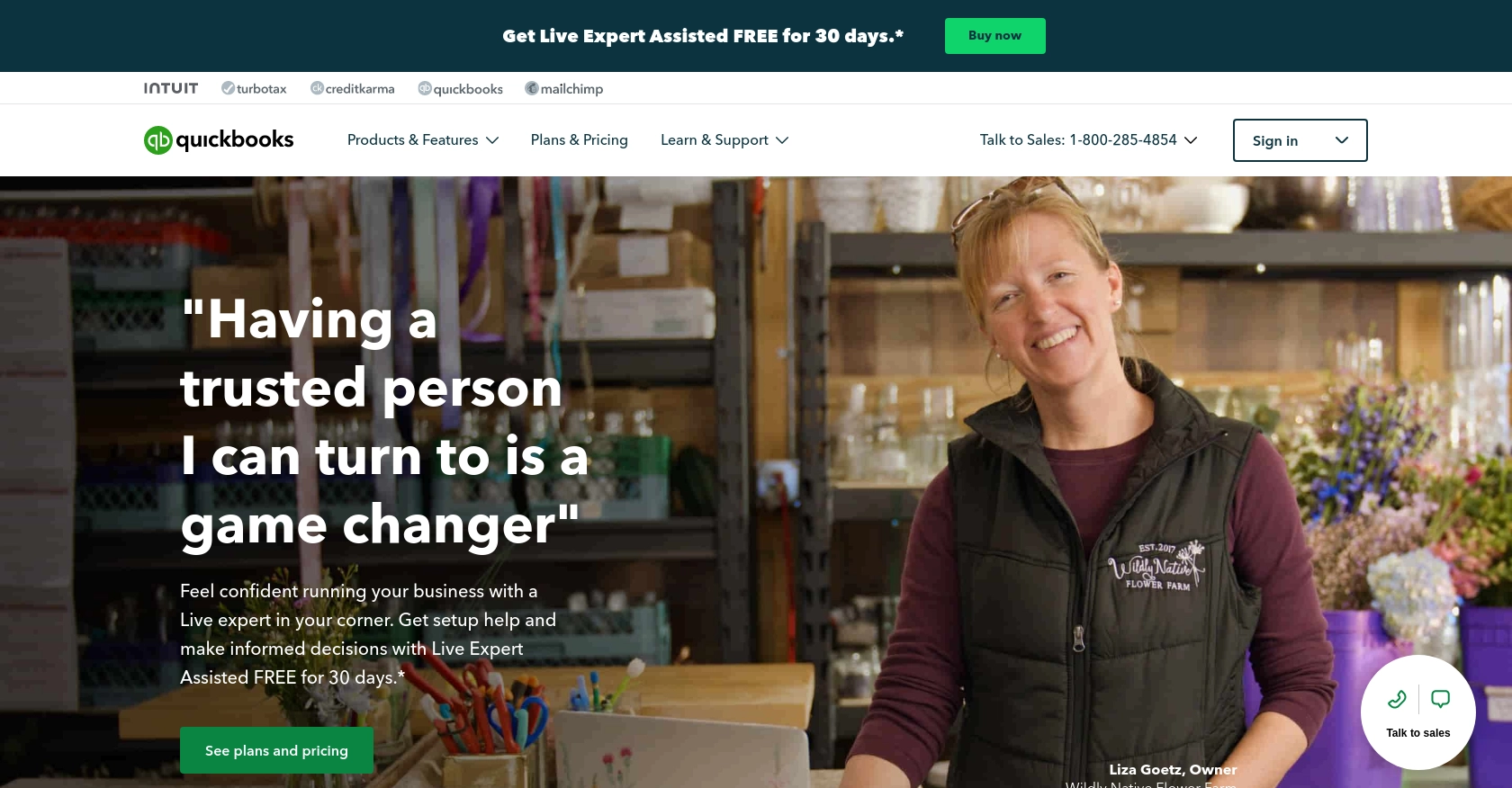
Introduction to QuickBooks API for Purchase Orders
QuickBooks is a widely-used accounting software that offers a robust platform for managing financial operations, including invoicing, payroll, and expense tracking. Its API provides developers with the tools needed to integrate QuickBooks functionalities into their own applications, enabling seamless financial management and data synchronization.
Integrating with the QuickBooks API allows developers to automate and streamline various accounting processes. For example, accessing purchase orders via the QuickBooks API can help businesses efficiently manage their procurement activities by retrieving detailed purchase order information directly into their systems.
This article will guide you through the process of using Python to interact with the QuickBooks API, specifically focusing on retrieving purchase orders. By the end of this tutorial, you'll be equipped to integrate QuickBooks purchase order data into your applications, enhancing your financial management capabilities.
Setting Up Your QuickBooks Sandbox Account for API Integration
Before you can start interacting with the QuickBooks API to retrieve purchase orders, you'll need to set up a sandbox account. This environment allows you to test API calls without affecting live data, providing a safe space to develop and refine your integration.
Creating a QuickBooks Developer Account
To begin, you'll need to create a QuickBooks Developer account. Follow these steps:
- Visit the QuickBooks Developer Portal.
- Sign up for a free developer account if you haven't already.
- Once registered, log in to your developer account.
Setting Up a QuickBooks Sandbox Company
With your developer account ready, the next step is to set up a sandbox company:
- Navigate to the "Sandbox" section in your QuickBooks Developer Dashboard.
- Create a new sandbox company by following the on-screen instructions.
- This sandbox will simulate a real QuickBooks environment, allowing you to test API interactions.
Creating an App for OAuth Authentication
QuickBooks API uses OAuth 2.0 for authentication. You'll need to create an app to obtain the necessary credentials:
- Go to the "My Apps" section in your QuickBooks Developer account.
- Click on "Create an App" and select "QuickBooks Online and Payments."
- Fill in the required details, such as app name and description.
- Once the app is created, navigate to the "Keys & OAuth" section to obtain your Client ID and Client Secret.
Ensure you save these credentials securely, as they will be used to authenticate your API requests.
Configuring OAuth 2.0 Redirect URIs
To complete the OAuth setup, configure your redirect URIs:
- In the "Keys & OAuth" section, add your redirect URI. This is the URL where users will be redirected after authentication.
- Ensure the URI is correctly set up to handle OAuth callbacks in your application.
With your sandbox account and app configured, you're now ready to start making API calls to QuickBooks. For more detailed information, refer to the official documentation on getting your Client ID and Client Secret and app settings.
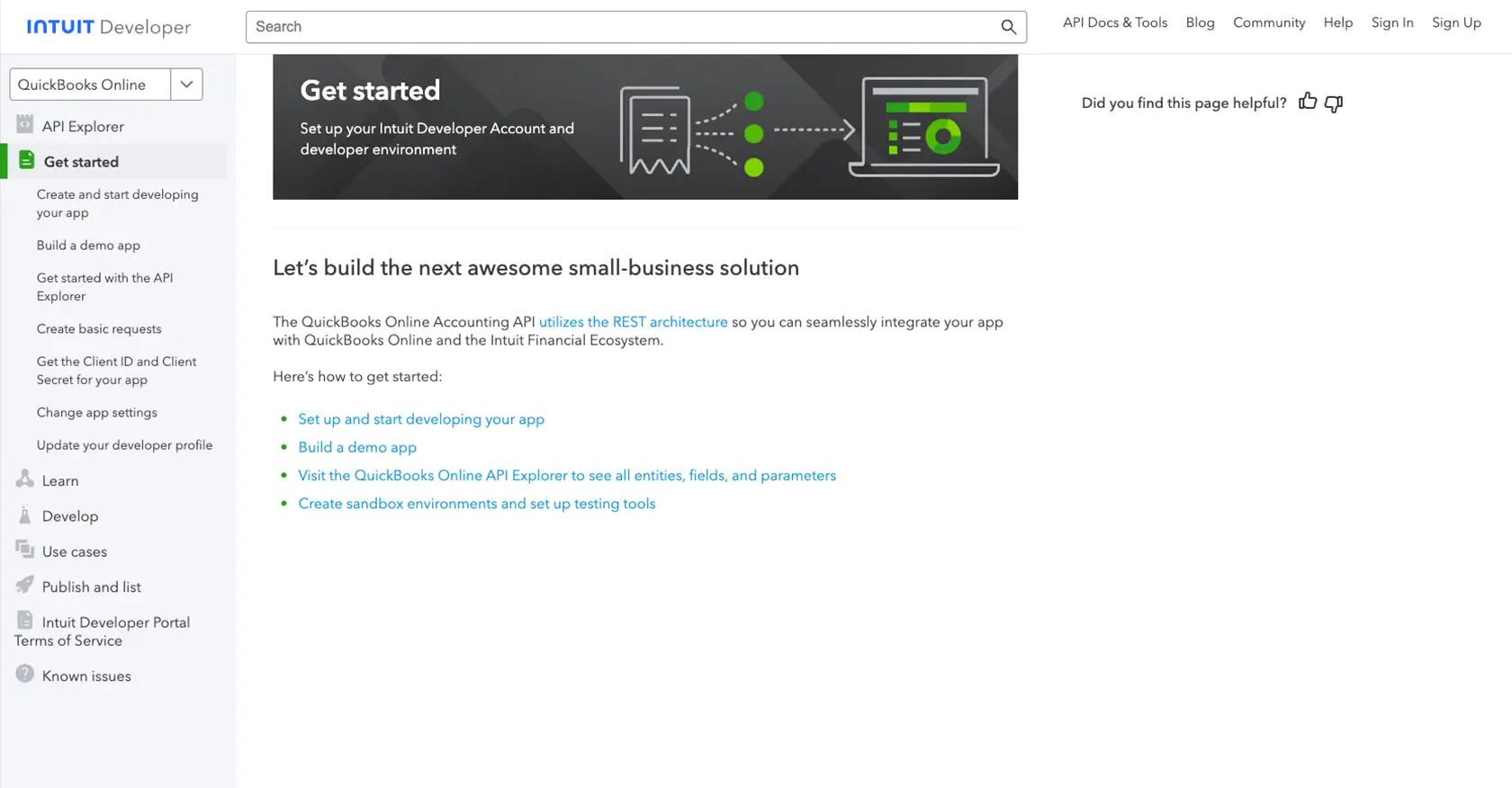
sbb-itb-96038d7
Making API Calls to Retrieve Purchase Orders from QuickBooks Using Python
Now that your QuickBooks sandbox account and OAuth app are set up, you can proceed to make API calls to retrieve purchase orders. This section will guide you through the process of using Python to interact with the QuickBooks API.
Setting Up Your Python Environment for QuickBooks API Integration
To begin, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need to install the requests
library to handle HTTP requests.
pip install requests
Authenticating with QuickBooks API Using OAuth 2.0
Before making API calls, you must authenticate using OAuth 2.0. This involves obtaining an access token using your Client ID and Client Secret.
Here's a basic example of how to authenticate:
import requests
# Set your credentials
client_id = 'Your_Client_ID'
client_secret = 'Your_Client_Secret'
redirect_uri = 'Your_Redirect_URI'
auth_url = 'https://appcenter.intuit.com/connect/oauth2'
token_url = 'https://oauth.platform.intuit.com/oauth2/v1/tokens/bearer'
# Obtain the authorization code
auth_code = 'Your_Authorization_Code'
# Exchange the authorization code for an access token
response = requests.post(token_url, data={
'grant_type': 'authorization_code',
'code': auth_code,
'redirect_uri': redirect_uri,
'client_id': client_id,
'client_secret': client_secret
})
access_token = response.json().get('access_token')
Replace Your_Client_ID
, Your_Client_Secret
, Your_Redirect_URI
, and Your_Authorization_Code
with your actual credentials and authorization code.
Retrieving Purchase Orders from QuickBooks API
With the access token in hand, you can now make a request to the QuickBooks API to retrieve purchase orders. Here's how you can do it:
import requests
# Set the API endpoint
endpoint = 'https://quickbooks.api.intuit.com/v3/company/Your_Company_ID/query'
# Set the headers
headers = {
'Authorization': f'Bearer {access_token}',
'Accept': 'application/json'
}
# Define the query to retrieve purchase orders
query = "SELECT * FROM PurchaseOrder"
# Make the API call
response = requests.post(endpoint, headers=headers, json={'query': query})
# Check if the request was successful
if response.status_code == 200:
purchase_orders = response.json().get('QueryResponse', {}).get('PurchaseOrder', [])
for po in purchase_orders:
print(po)
else:
print(f"Failed to retrieve purchase orders: {response.status_code}")
Replace Your_Company_ID
with your actual QuickBooks company ID. This script will print out the purchase orders retrieved from your QuickBooks sandbox environment.
Handling Errors and Verifying API Call Success
It's crucial to handle potential errors when making API calls. Check the response status code to verify success. A status code of 200 indicates a successful request. If the request fails, the response will contain an error code and message.
Refer to the QuickBooks API documentation for detailed information on error codes and handling.
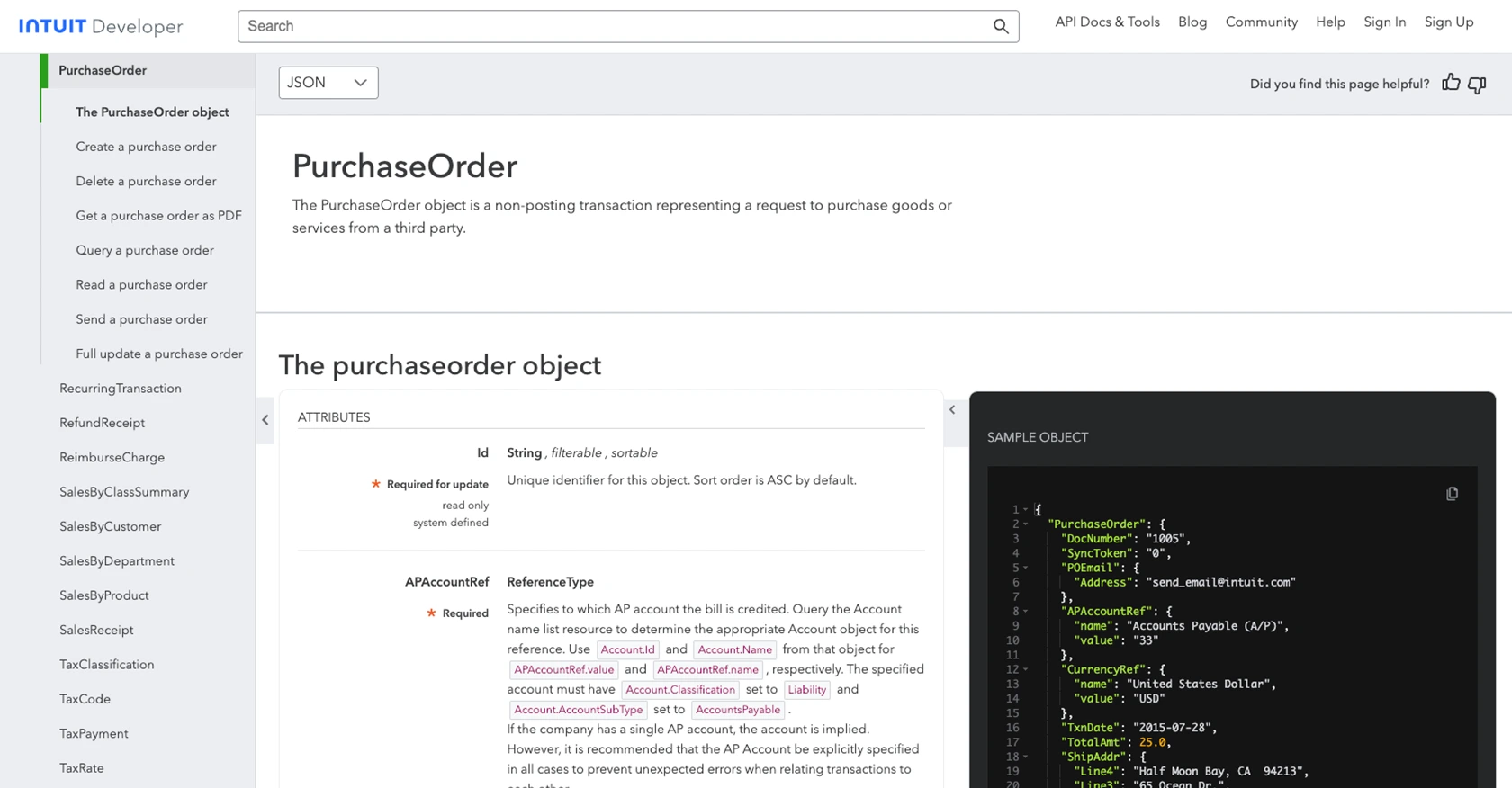
Best Practices for QuickBooks API Integration and Error Handling
Successfully integrating with the QuickBooks API requires attention to best practices, especially when handling sensitive financial data. Here are some recommendations to ensure a smooth and secure integration:
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and access tokens securely. Use environment variables or secure vaults to prevent unauthorized access.
- Implement Rate Limiting: QuickBooks API has rate limits to prevent abuse. Ensure your application handles these limits gracefully by implementing retry logic and backoff strategies. Refer to the QuickBooks API documentation for specific rate limit details.
- Data Transformation and Standardization: When retrieving purchase orders, ensure that the data is transformed and standardized to fit your application's data model. This will facilitate seamless integration and data consistency.
- Error Handling: Implement robust error handling to manage API call failures. Log errors and provide meaningful messages to users. Use the error codes provided by QuickBooks to diagnose issues effectively.
Streamlining QuickBooks API Integrations with Endgrate
Building and maintaining integrations with multiple platforms can be time-consuming and complex. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including QuickBooks.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Develop a single integration that works across multiple platforms, reducing redundant efforts.
- Enhance Customer Experience: Provide your customers with an intuitive and seamless integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of outsourcing your integration needs.
Read More
- https://endgrate.com/provider/quickbooks
- https://developer.intuit.com/app/developer/qbo/docs/get-started/start-developing-your-app
- https://developer.intuit.com/app/developer/qbo/docs/get-started/get-client-id-and-client-secret
- https://developer.intuit.com/app/developer/qbo/docs/get-started/app-settings
- https://developer.intuit.com/app/developer/qbo/docs/api/accounting/all-entities/purchaseorder
Ready to get started?