How to Get Recipients with the Mailshake API in Python
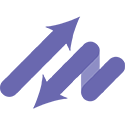
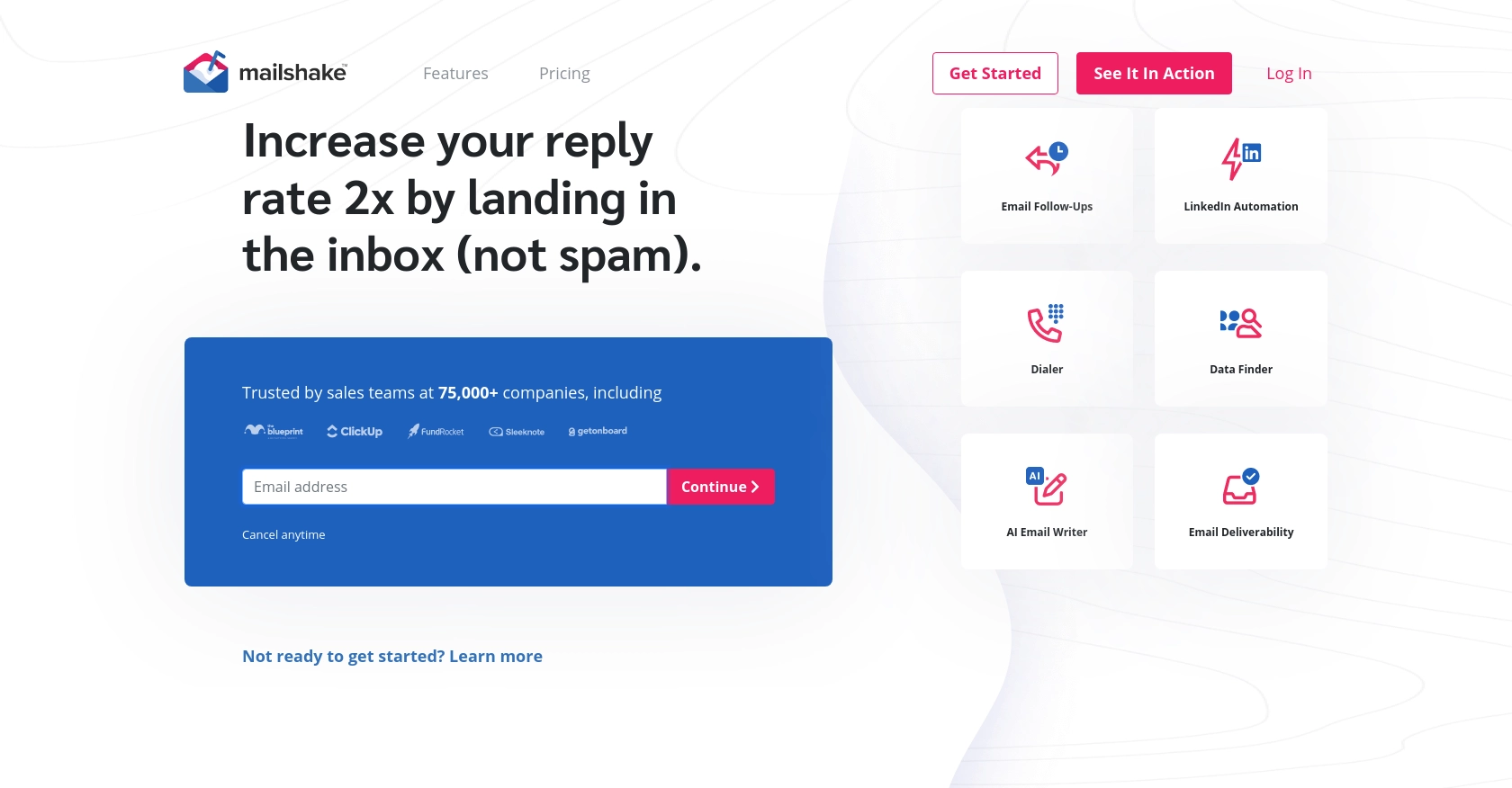
Introduction to Mailshake API Integration
Mailshake is a powerful email outreach platform designed to help businesses streamline their email campaigns and engage with prospects effectively. It offers a suite of tools for managing campaigns, tracking engagement, and optimizing outreach strategies.
Integrating with the Mailshake API allows developers to automate and enhance their email marketing efforts. For instance, you can use the API to retrieve recipient data from a specific campaign, enabling you to analyze engagement metrics or synchronize data with other systems. This integration can significantly improve the efficiency of your email marketing workflows.
Setting Up Your Mailshake API Test Account
Before you can start interacting with the Mailshake API, you'll need to set up a test account. This involves creating an API key that will allow you to authenticate your requests and access the necessary data.
Creating a Mailshake Account
If you don't already have a Mailshake account, you can sign up for a free trial on the Mailshake website. This will give you access to the platform's features and allow you to explore its capabilities.
- Visit the Mailshake website and click on the "Sign Up" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Mailshake dashboard.
Generating Your Mailshake API Key
To interact with the Mailshake API, you'll need an API key. This key acts as a password for your application, allowing it to communicate securely with Mailshake's servers.
- Navigate to the "Extensions" section in the Mailshake dashboard.
- Select "API" from the menu options.
- Click on "Create API Key" to generate a new key.
- Copy the generated API key and store it securely, as you'll need it to authenticate your API requests.
Understanding Mailshake API Authentication
Mailshake uses simple API key-based authentication. You can include your API key in your requests in one of the following ways:
- As a query string parameter:
?apiKey=your_api_key
- In the request body as JSON:
{"apiKey": "your_api_key"}
- Via an HTTP Authorization header:
Authorization: Basic [base-64 encoded version of your api key]
For more details on authentication, refer to the Mailshake API documentation.
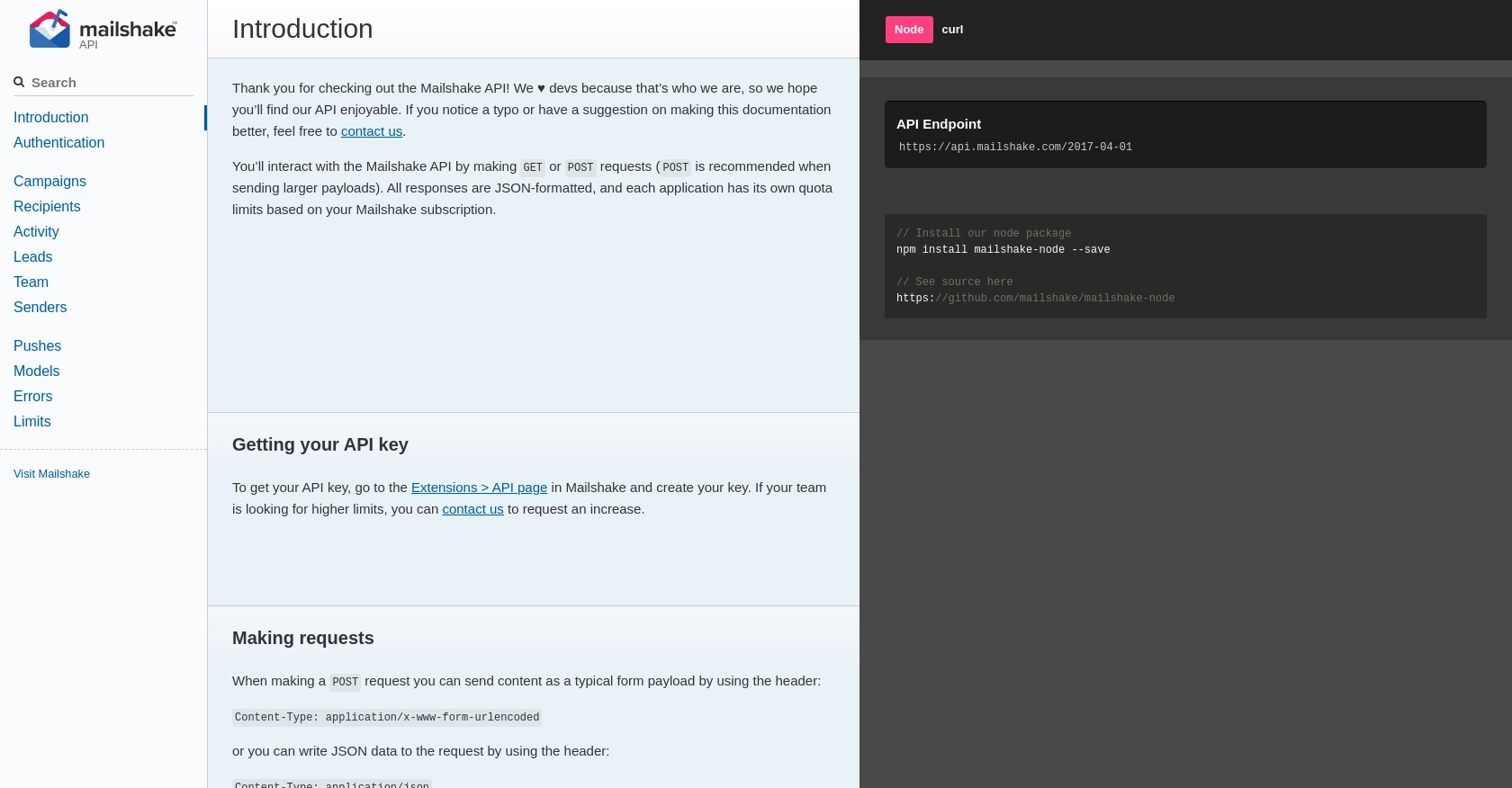
sbb-itb-96038d7
How to Make API Calls to Retrieve Recipients with Mailshake in Python
To interact with the Mailshake API and retrieve recipient data, you'll need to use Python. This section will guide you through the process of setting up your environment, writing the necessary code, and executing API calls to fetch recipient information from your Mailshake campaigns.
Setting Up Your Python Environment for Mailshake API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Writing Python Code to Fetch Recipients from Mailshake API
Once your environment is set up, you can write a Python script to retrieve recipients from a specific Mailshake campaign. The following code demonstrates how to make a GET request to the Mailshake API to list recipients.
import requests
# Define the API endpoint and headers
endpoint = "https://api.mailshake.com/2017-04-01/recipients/list"
headers = {
"Authorization": "Basic [base-64 encoded version of your api key]",
"Content-Type": "application/json"
}
# Set the parameters for the request
params = {
"campaignID": 1, # Replace with your campaign ID
"perPage": 100 # Number of results per page
}
# Make the GET request to the API
response = requests.get(endpoint, headers=headers, params=params)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
for recipient in data["results"]:
print(recipient)
else:
print(f"Failed to retrieve recipients: {response.status_code} - {response.text}")
Replace [base-64 encoded version of your api key]
with your actual API key encoded in base-64 format. Also, update the campaignID
with the ID of the campaign you wish to query.
Verifying Successful API Requests and Handling Errors
After running the script, you should see a list of recipients from the specified campaign. If the request fails, the script will output an error message with the status code and response text. Common error codes include:
invalid_api_key
: The API key is missing or invalid.not_found
: The specified campaign or recipient could not be found.limit_reached
: The request exceeds the allowed quota units.
For more details on error codes, refer to the Mailshake API documentation.
Conclusion and Best Practices for Mailshake API Integration in Python
Integrating with the Mailshake API using Python can significantly enhance your email marketing workflows by automating recipient management and data synchronization. By following the steps outlined in this guide, you can efficiently retrieve recipient data from your Mailshake campaigns and handle any errors that may arise during the process.
Best Practices for Secure and Efficient Mailshake API Usage
- Securely Store API Keys: Ensure that your API keys are stored securely and not hard-coded in your scripts. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Be mindful of Mailshake's rate limits to avoid hitting quota restrictions. Implement logic to handle the
limit_reached
error and retry requests after the specified wait time. - Data Standardization: Standardize and transform data fields as needed to ensure consistency across different systems and integrations.
Enhance Your Integration Experience with Endgrate
While integrating with Mailshake's API can be straightforward, managing multiple integrations can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Mailshake. This allows you to build once for each use case and streamline your integration efforts.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product while providing an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can help you scale your integrations efficiently.
Read More
Ready to get started?