How to Get Service Accounts with the Mixpanel API in Python
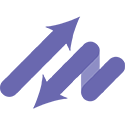
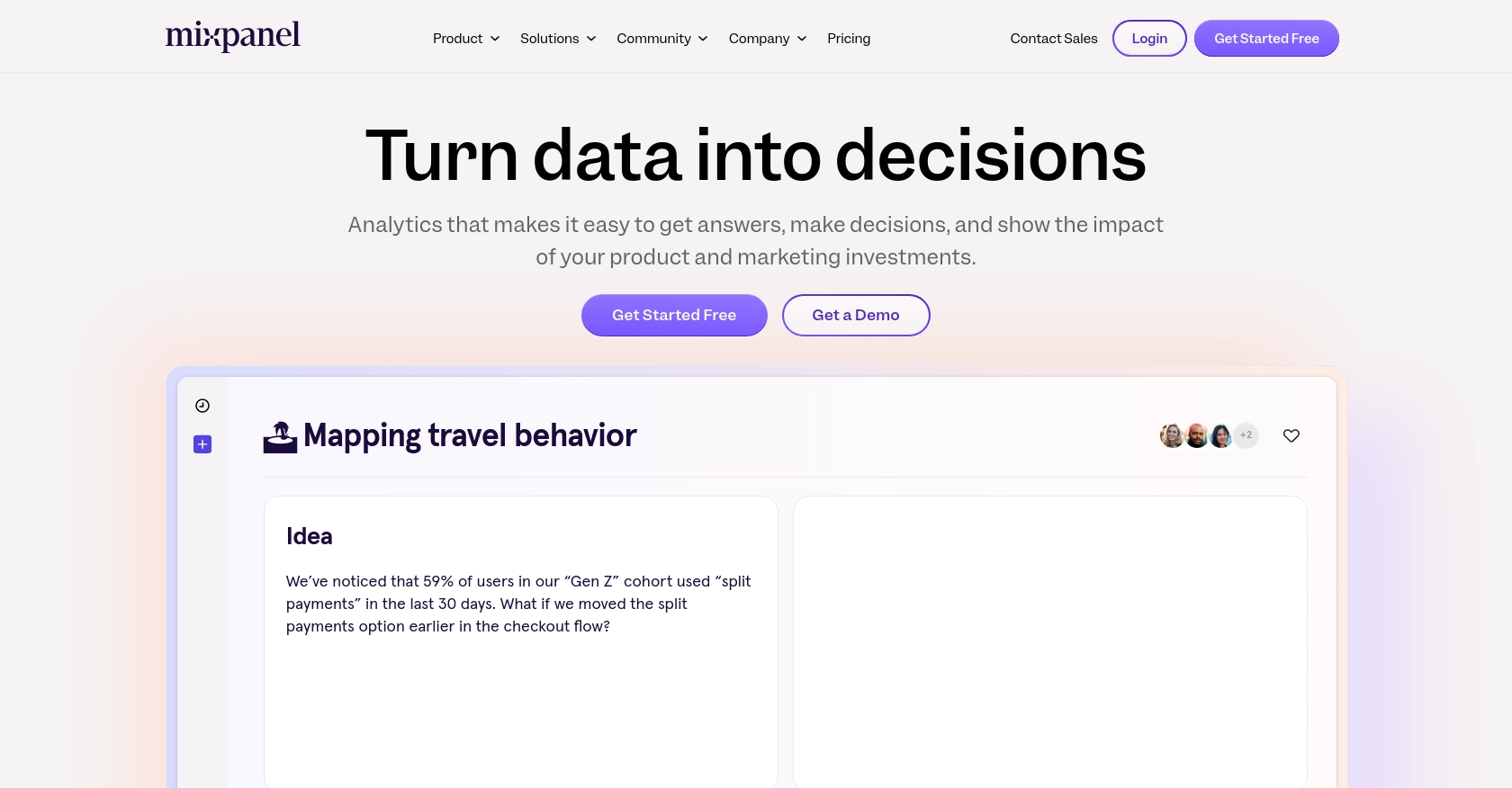
Introduction to Mixpanel API Integration
Mixpanel is a powerful analytics platform that helps businesses track user interactions with web and mobile applications. It provides detailed insights into user behavior, enabling companies to make data-driven decisions to enhance user engagement and optimize product offerings.
Integrating with Mixpanel's API allows developers to access a wealth of data, including service accounts, which are essential for managing automated processes and backend services. For example, a developer might want to retrieve a list of service accounts to audit permissions and ensure compliance with organizational policies.
This article will guide you through the process of using Python to interact with the Mixpanel API, specifically focusing on retrieving service accounts. By following this tutorial, you'll learn how to efficiently access and manage service accounts within your organization using Python.
Setting Up Your Mixpanel Test Account
Before you can start interacting with the Mixpanel API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data. Mixpanel provides a straightforward way to create and manage service accounts, which are crucial for accessing various features of the platform.
Creating a Mixpanel Account
If you don't already have a Mixpanel account, you can sign up for a free trial on the Mixpanel website. This will give you access to all the necessary tools and features to begin your integration journey.
- Visit the Mixpanel website and click on the "Sign Up" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Mixpanel dashboard.
Generating Service Account Credentials
To authenticate your API requests, you'll need to create a service account in Mixpanel. Service accounts are designed for automated processes and backend services, providing a secure way to access your data.
- Navigate to the "Organization Settings" in your Mixpanel dashboard.
- Select the "Service Accounts" tab.
- Click on "Create Service Account" and fill in the required details, such as the role and project access.
- Once created, you'll receive a username and secret. Store these credentials securely, as they will be used for API authentication.
Authenticating with Mixpanel API
Mixpanel uses HTTP Basic Auth for service account authentication. You can provide the service account's username and secret directly in your API requests.
import requests
response = requests.get(
'https://mixpanel.com/api/app/organizations/{organizationId}/service-accounts',
auth=('your_serviceaccount_username', 'your_serviceaccount_secret')
)
Replace your_serviceaccount_username
and your_serviceaccount_secret
with the credentials you obtained earlier. This will allow you to authenticate and interact with the Mixpanel API securely.
For more detailed information on authentication, you can refer to the Mixpanel Service Accounts documentation.
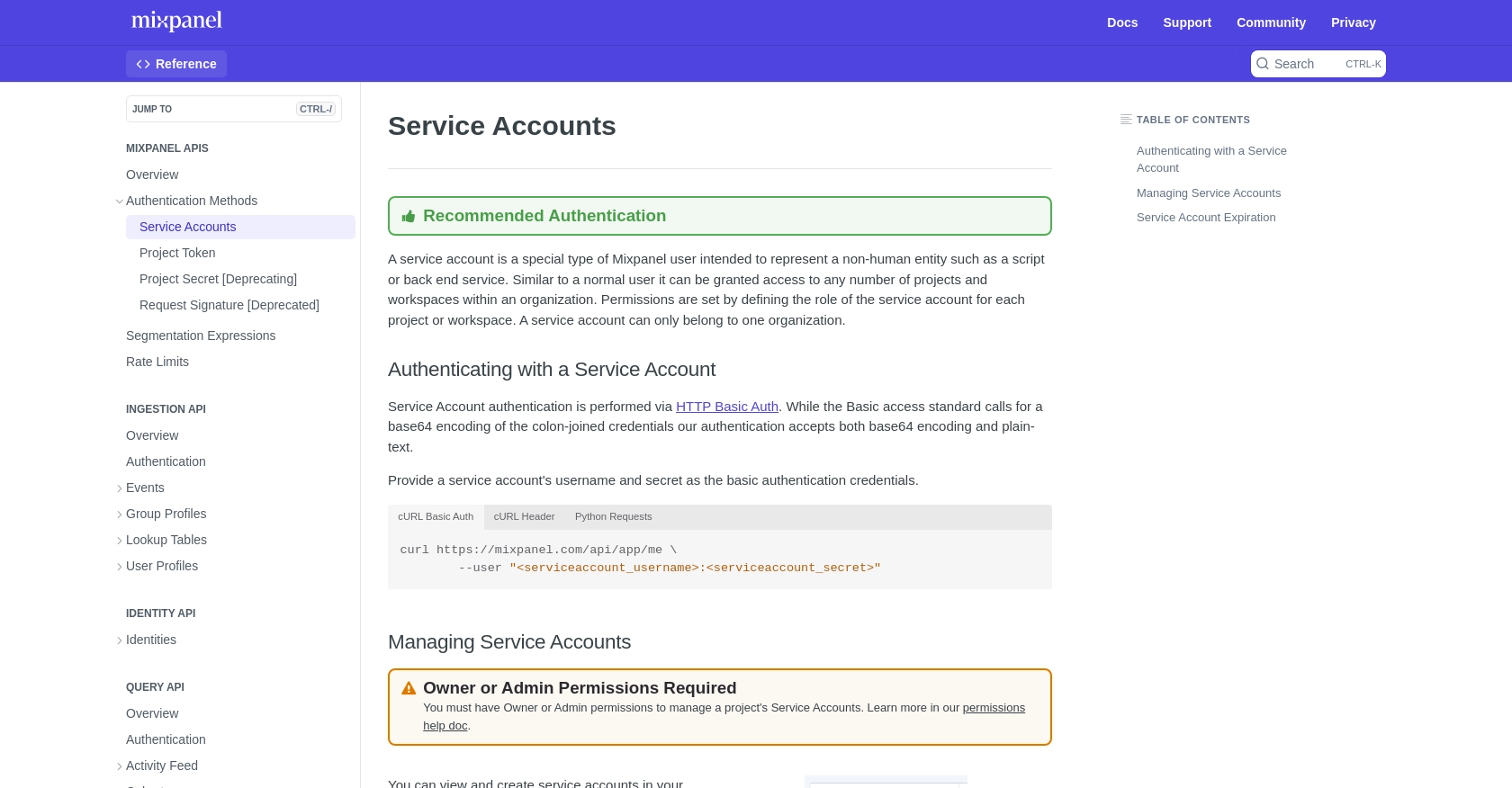
sbb-itb-96038d7
Making API Calls to Retrieve Mixpanel Service Accounts Using Python
To interact with the Mixpanel API and retrieve service accounts, you'll need to use Python's requests
library. This section will guide you through the necessary steps to make an API call and handle the response effectively.
Setting Up Your Python Environment for Mixpanel API Integration
Before making any API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need to install the requests
library if it's not already available. You can do this by running the following command:
pip install requests
Writing Python Code to Retrieve Service Accounts from Mixpanel
Once your environment is set up, you can proceed to write the Python script to interact with the Mixpanel API. Create a new file named get_mixpanel_service_accounts.py
and add the following code:
import requests
# Define the API endpoint
url = 'https://mixpanel.com/api/app/organizations/{organizationId}/service-accounts'
# Provide your service account credentials
auth_credentials = ('your_serviceaccount_username', 'your_serviceaccount_secret')
# Make a GET request to the API
response = requests.get(url, auth=auth_credentials)
# Check if the request was successful
if response.status_code == 200:
# Parse and print the JSON data from the response
service_accounts = response.json()
print(service_accounts)
else:
print(f"Failed to retrieve service accounts. Status code: {response.status_code}")
Replace your_serviceaccount_username
and your_serviceaccount_secret
with the credentials you obtained from Mixpanel. This script sends a GET request to the Mixpanel API to retrieve a list of service accounts.
Understanding and Handling API Responses from Mixpanel
After running the script, you should see a JSON response containing the details of the service accounts associated with your organization. If the request fails, the script will output an error message with the status code.
To verify the request's success, ensure that the returned data matches the service accounts listed in your Mixpanel dashboard. If you encounter any errors, refer to the Mixpanel API documentation for troubleshooting tips.
Handling Errors and Rate Limits in Mixpanel API Calls
When making API calls, it's essential to handle potential errors gracefully. The Mixpanel API may return various HTTP status codes indicating different issues. For instance, a 401 status code indicates authentication failure, while a 429 status code means you've hit the rate limit.
Mixpanel enforces rate limits to ensure fair usage. According to the Mixpanel Rate Limits documentation, the Query API allows a maximum of 60 queries per hour. To avoid rate limiting, consider spreading your requests over time or consolidating them when possible.
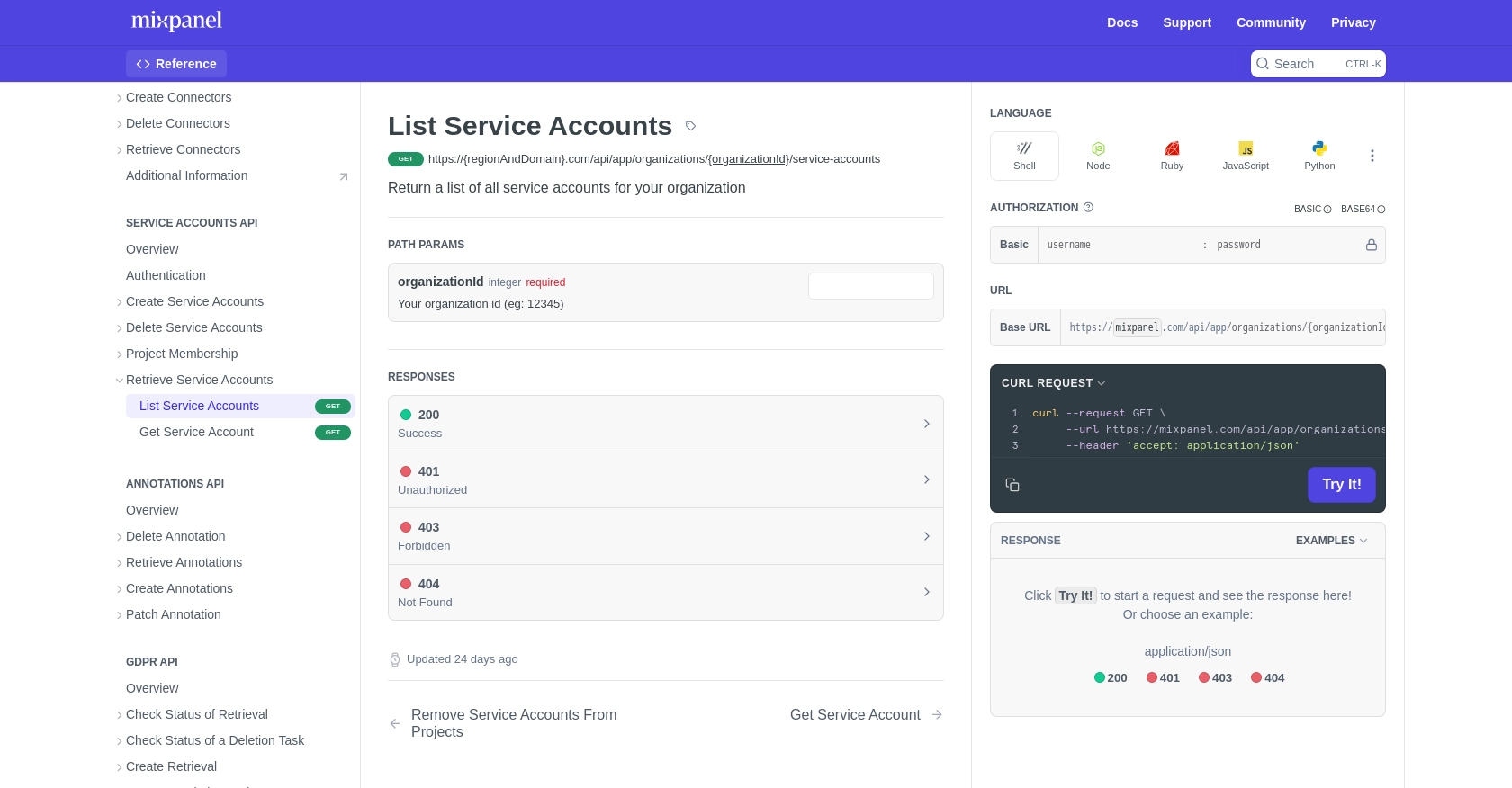
Conclusion and Best Practices for Using Mixpanel API with Python
Integrating with the Mixpanel API using Python provides developers with powerful capabilities to manage and audit service accounts effectively. By following the steps outlined in this guide, you can seamlessly retrieve and handle service accounts, ensuring your organization maintains compliance and security standards.
Best Practices for Securely Managing Mixpanel API Credentials
- Secure Storage: Always store your service account credentials in a secure location, such as environment variables or a secure vault, to prevent unauthorized access.
- Regular Rotation: Implement a policy for regularly rotating your service account credentials to enhance security.
- Access Control: Limit the permissions of service accounts to only what is necessary for their intended use.
Handling Mixpanel API Rate Limits and Optimizing Requests
- Monitor Usage: Keep track of your API usage to avoid hitting rate limits. Use logging to monitor the frequency of your requests.
- Batch Requests: Where possible, consolidate multiple queries into a single request to optimize your API usage.
- Spread Requests: Distribute your API calls over time to prevent exceeding the rate limits, especially during peak usage periods.
Transforming and Standardizing Data from Mixpanel API
When retrieving data from Mixpanel, consider transforming and standardizing the data fields to align with your organization's data models. This ensures consistency and facilitates easier integration with other systems.
Streamlining Integrations with Endgrate
For developers looking to simplify the integration process across multiple platforms, Endgrate offers a unified API solution. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Endgrate provides an intuitive integration experience, enabling you to build once and deploy across various platforms effortlessly.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
Ready to get started?