How to Get Tax Rates with the Zoho Books API in PHP
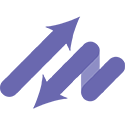
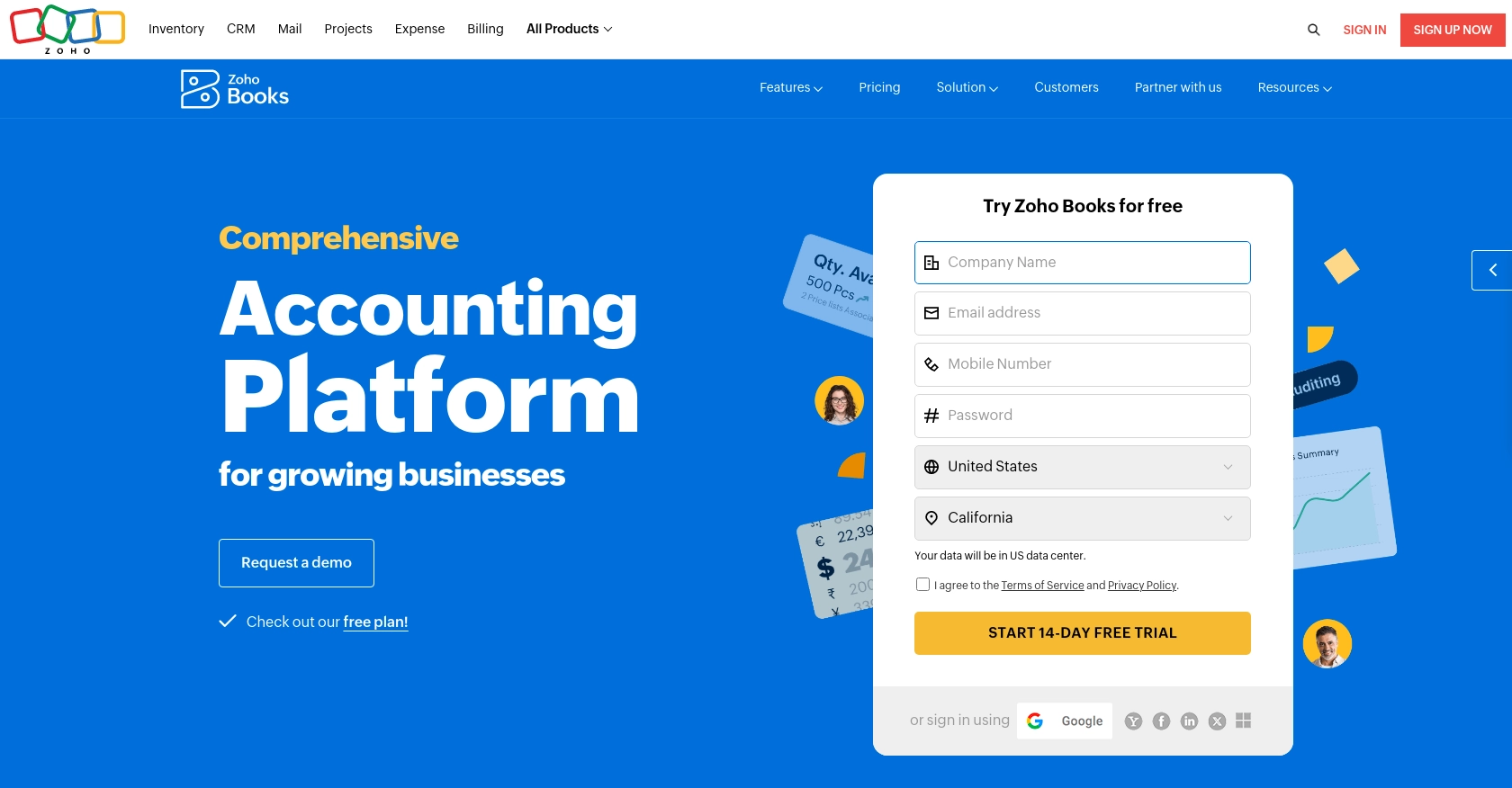
Introduction to Zoho Books API for Tax Rates
Zoho Books is a comprehensive online accounting software that helps businesses manage their finances efficiently. It offers a wide range of features, including invoicing, expense tracking, and financial reporting, making it a popular choice for businesses of all sizes.
Integrating with the Zoho Books API allows developers to automate financial processes and access critical data, such as tax rates, directly within their applications. For example, a developer might want to retrieve tax rates to ensure accurate tax calculations in a custom invoicing system.
This article will guide you through the process of using PHP to interact with the Zoho Books API to fetch tax rates, providing a seamless integration experience for your financial applications.
Setting Up Your Zoho Books Test Account for API Integration
Before you can start interacting with the Zoho Books API to retrieve tax rates, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Zoho Books Account
If you don't already have a Zoho Books account, you can sign up for a free trial on the Zoho Books website. Follow the instructions to create your account, and you'll be ready to proceed with the integration setup.
Registering Your Application for OAuth Authentication
Zoho Books uses OAuth 2.0 for secure API authentication. Follow these steps to register your application and obtain the necessary credentials:
- Visit the Zoho Developer Console and sign in with your Zoho account.
- Click on Add Client ID to register your application.
- Provide the required details, such as the application name and redirect URI, and submit the form.
- Upon successful registration, you'll receive a Client ID and Client Secret. Keep these credentials secure as they are essential for API access.
Generating an OAuth Access Token
With your Client ID and Client Secret, you can now generate an OAuth access token to authenticate API requests:
- Redirect users to the following authorization URL, replacing the placeholders with your details:
- After user consent, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request to:
- Store the received access_token and refresh_token securely for future API calls.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.settings.READ&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
For more details on OAuth setup, refer to the Zoho Books OAuth Documentation.
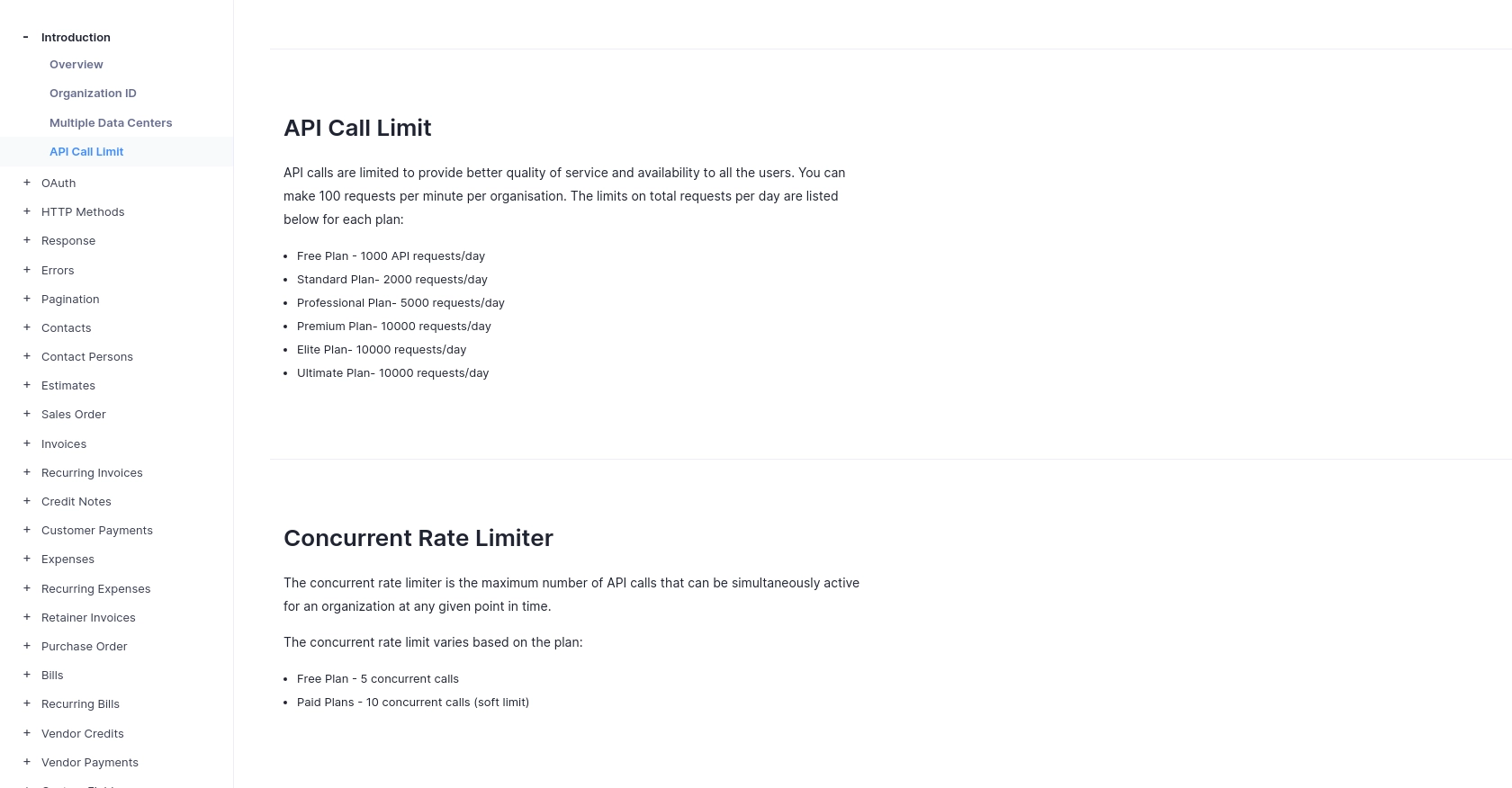
sbb-itb-96038d7
Making API Calls to Retrieve Tax Rates from Zoho Books Using PHP
To interact with the Zoho Books API and retrieve tax rates, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make API calls, and handling responses.
Setting Up Your PHP Environment for Zoho Books API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer for dependency management
Install the Guzzle HTTP client using Composer to simplify making HTTP requests:
composer require guzzlehttp/guzzle
Writing PHP Code to Fetch Tax Rates from Zoho Books API
With your environment set up, you can now write PHP code to interact with the Zoho Books API. Create a file named get_tax_rates.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
// Initialize the Guzzle client
$client = new Client();
// Set the API endpoint and headers
$endpoint = 'https://www.zohoapis.com/books/v3/settings/taxes';
$headers = [
'Authorization' => 'Zoho-oauthtoken YOUR_ACCESS_TOKEN',
'Content-Type' => 'application/json'
];
// Make a GET request to the API
$response = $client->request('GET', $endpoint, [
'headers' => $headers,
'query' => ['organization_id' => 'YOUR_ORGANIZATION_ID']
]);
// Parse the JSON data from the response
$data = json_decode($response->getBody(), true);
// Display the tax rates
foreach ($data['taxes'] as $tax) {
echo 'Tax Name: ' . $tax['tax_name'] . '<br>';
echo 'Tax Percentage: ' . $tax['tax_percentage'] . '%<br><br>';
}
Replace YOUR_ACCESS_TOKEN
and YOUR_ORGANIZATION_ID
with your actual access token and organization ID.
Verifying API Call Success and Handling Errors
After running the script, you should see the tax rates displayed. Verify the request's success by checking the response status code:
if ($response->getStatusCode() == 200) {
echo 'API call successful!';
} else {
echo 'Failed to retrieve tax rates.';
}
Handle potential errors by checking for common HTTP status codes, such as 400 for bad requests or 401 for unauthorized access. Refer to the Zoho Books Error Documentation for more details.
For more information on API call limits, refer to the Zoho Books API Call Limit Documentation.
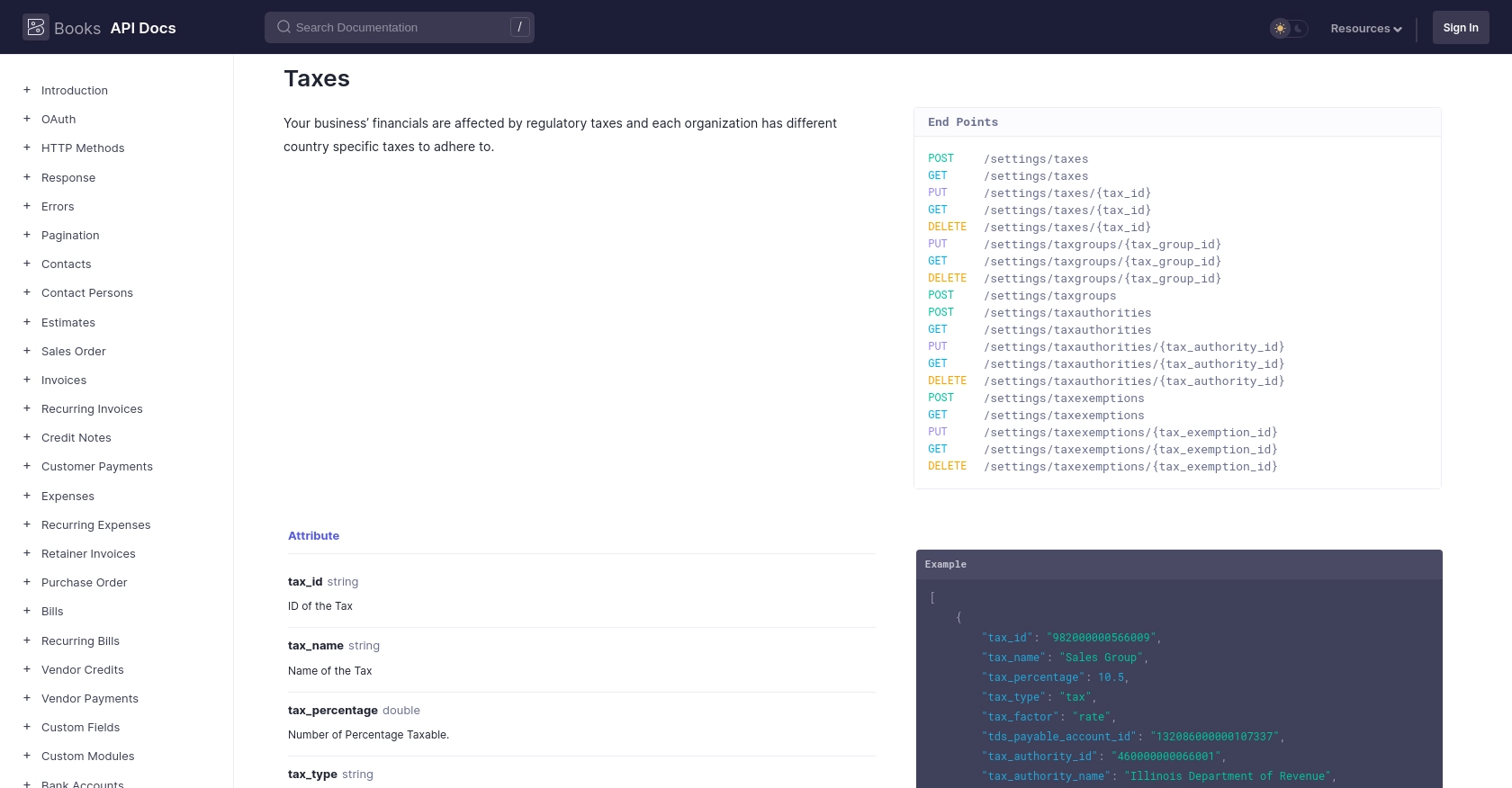
Conclusion and Best Practices for Using Zoho Books API in PHP
Integrating with the Zoho Books API to retrieve tax rates using PHP can significantly enhance your financial applications by automating tax calculations and ensuring accuracy. By following the steps outlined in this guide, you can seamlessly connect your application to Zoho Books and leverage its powerful features.
Best Practices for Secure and Efficient Zoho Books API Integration
- Securely Store Credentials: Always store your OAuth credentials, such as the access token and refresh token, securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Zoho Books API has rate limits of 100 requests per minute per organization. Plan your API calls accordingly and implement retry logic to handle rate limit errors gracefully. For more details, refer to the API Call Limit Documentation.
- Implement Error Handling: Use appropriate error handling to manage different HTTP status codes. This ensures your application can respond to issues such as unauthorized access or bad requests. Consult the Error Documentation for guidance.
- Optimize Data Handling: When processing API responses, ensure that data is transformed and standardized to fit your application's requirements. This will help maintain consistency and accuracy across your systems.
Enhance Your Integration Experience with Endgrate
While integrating with Zoho Books API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Zoho Books. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover the benefits of a single, intuitive API endpoint for all your integration needs.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/taxes/#overview
Ready to get started?