How to Get Tax with the Avalara API in Javascript
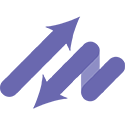
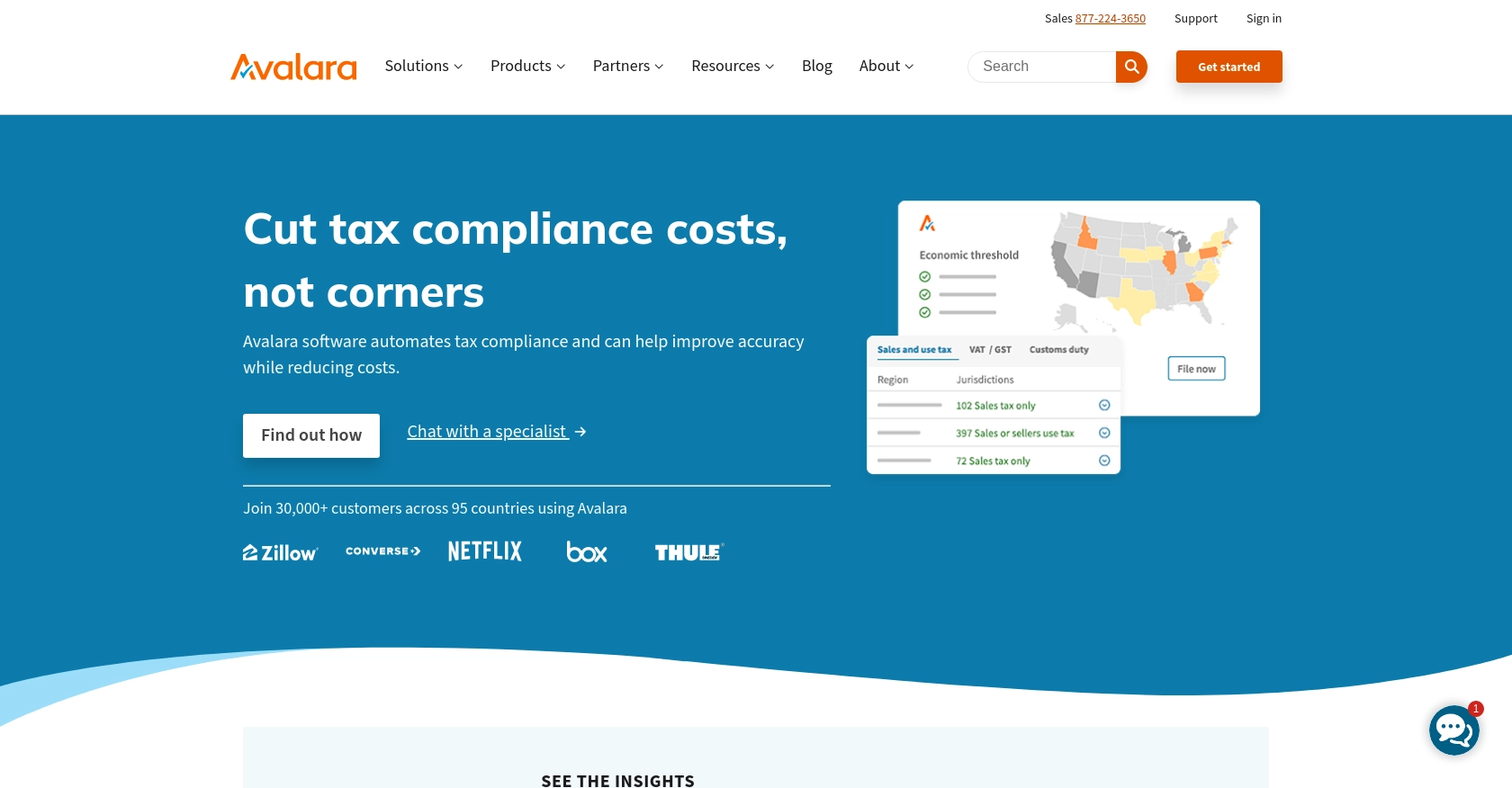
Introduction to Avalara API Integration
Avalara is a prominent provider of cloud-based tax compliance solutions, offering a comprehensive suite of tools to automate tax calculations, returns, and compliance for businesses of all sizes. Its flagship product, AvaTax, is widely used for accurate and efficient tax management across various industries, including retail, ecommerce, and software.
Integrating with Avalara's API allows developers to streamline tax-related processes, such as calculating sales tax for transactions. For example, a developer might use the Avalara API to automatically calculate and apply the correct sales tax for online purchases, ensuring compliance with local tax regulations and improving the customer checkout experience.
Setting Up Your Avalara Test or Sandbox Account
Before you can start integrating with the Avalara API, you need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Avalara provides a sandbox environment that mimics the production environment, enabling developers to test their integrations thoroughly.
Creating an Avalara Sandbox Account
To begin, you need to create a sandbox account on the Avalara website. Follow these steps:
- Visit the Avalara Developer Portal.
- Sign up for a free developer account if you don't already have one.
- Once registered, log in to your account and navigate to the sandbox environment setup section.
- Follow the prompts to create your sandbox account, which will provide you with the necessary credentials to access the Avalara API.
Generating Avalara API Credentials
After setting up your sandbox account, you need to generate API credentials to authenticate your requests. Avalara supports Basic HTTP Authentication, which requires a username and password. Here's how to obtain these credentials:
- Log in to your Avalara sandbox account.
- Navigate to the "Settings" section and select "API Credentials."
- Generate a new set of credentials, which will include your username and password.
- Make sure to store these credentials securely, as you will need them to authenticate your API calls.
Understanding Avalara Authentication Methods
Avalara offers two primary authentication methods: Basic Authentication and License Key Authentication. For most integrations, Basic Authentication is recommended. Here's a brief overview:
- Basic Authentication: Uses your username and password to authenticate API requests. This method is straightforward and suitable for individual users.
- License Key Authentication: Utilizes an account ID and a license key. This method is ideal for connectors that customers set up on their premises.
For more detailed information on Avalara's authentication methods, refer to the Avalara Authentication Documentation.
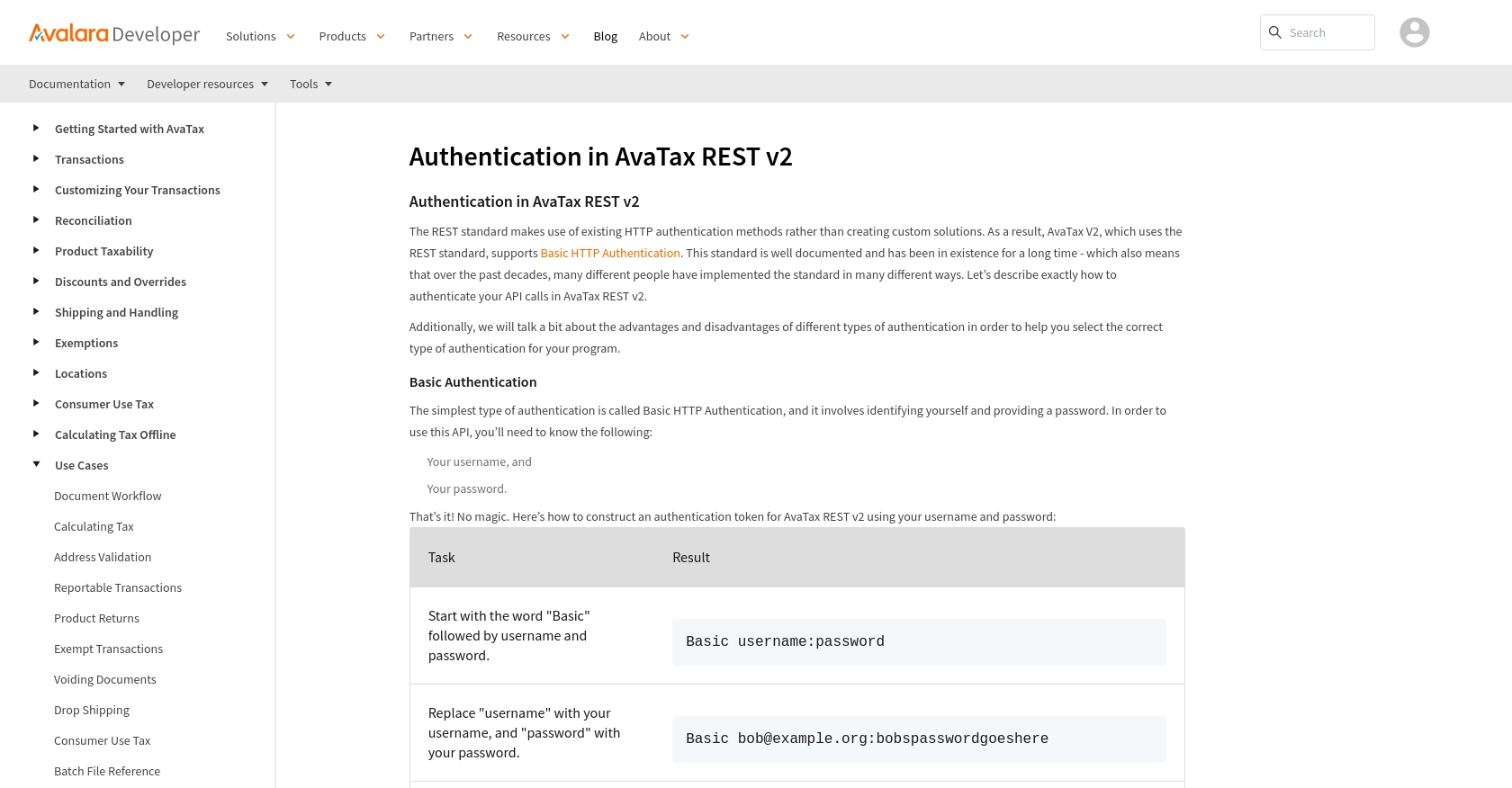
sbb-itb-96038d7
Making API Calls to Retrieve Tax Information with Avalara in JavaScript
To interact with the Avalara API using JavaScript, you'll need to set up your environment and write code to make HTTP requests. This section will guide you through the process of retrieving tax information using Avalara's API.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, making it ideal for server-side applications.
To check if Node.js is installed, run the following command in your terminal:
node -v
If Node.js is not installed, download and install it from the official Node.js website.
Next, you'll need to install the axios
library, which simplifies making HTTP requests. Run the following command to install it:
npm install axios
Writing JavaScript Code to Call Avalara API
Now that your environment is set up, you can write the JavaScript code to retrieve tax information from Avalara. Create a new file named getTaxInfo.js
and add the following code:
const axios = require('axios');
// Set your Avalara API credentials
const username = 'your_username';
const password = 'your_password';
// Encode credentials in Base64
const auth = Buffer.from(`${username}:${password}`).toString('base64');
// Define the API endpoint
const endpoint = 'https://sandbox-rest.avatax.com/api/v2/transactions/create';
// Set up the request headers
const headers = {
'Authorization': `Basic ${auth}`,
'Content-Type': 'application/json'
};
// Define the transaction data
const transactionData = {
type: 'SalesInvoice',
companyCode: 'YOUR_COMPANY_CODE',
date: '2023-10-01',
customerCode: 'CUSTOMER_CODE',
lines: [
{
number: '1',
quantity: 1,
amount: 100,
taxCode: 'P0000000'
}
],
addresses: {
singleLocation: {
line1: '123 Main Street',
city: 'Seattle',
region: 'WA',
postalCode: '98101',
country: 'US'
}
}
};
// Make the API call
axios.post(endpoint, transactionData, { headers })
.then(response => {
console.log('Tax Information:', response.data);
})
.catch(error => {
console.error('Error fetching tax information:', error.response ? error.response.data : error.message);
});
Replace your_username
, your_password
, YOUR_COMPANY_CODE
, and CUSTOMER_CODE
with your actual Avalara credentials and relevant data.
Running the JavaScript Code
To execute the code, run the following command in your terminal:
node getTaxInfo.js
If successful, the console will display the tax information retrieved from Avalara. If there are errors, ensure your credentials and data are correct and check the error message for more details.
Handling Errors and Verifying API Requests
When making API calls, it's crucial to handle potential errors gracefully. The example code includes error handling using catch
to log any issues that arise during the request.
To verify the success of your API requests, you can cross-check the returned data with the Avalara sandbox environment. Ensure that the transaction details match the expected output.
For more information on error codes and troubleshooting, refer to the Avalara Authentication Documentation.
Conclusion and Best Practices for Avalara API Integration
Integrating with the Avalara API using JavaScript can significantly enhance your tax compliance processes by automating tax calculations and ensuring accuracy. By following the steps outlined in this guide, you can efficiently retrieve tax information and handle transactions with ease.
Best Practices for Secure and Efficient Avalara API Usage
- Secure Storage of Credentials: Always store your Avalara API credentials securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of Avalara's rate limits to avoid service disruptions. Implement retry logic with exponential backoff to manage API call limits effectively.
- Data Standardization: Ensure that the data you send to Avalara is standardized and validated to prevent errors and ensure compliance with tax regulations.
- Error Handling: Implement comprehensive error handling to manage API call failures gracefully. Log errors for troubleshooting and use Avalara's documentation to understand error codes.
Streamlining Integrations with Endgrate
For developers looking to simplify their integration processes, Endgrate offers a unified API solution that connects to multiple platforms, including Avalara. By using Endgrate, you can save time and resources, allowing you to focus on your core product development. Explore how Endgrate can enhance your integration experience by visiting Endgrate's website.
By leveraging Avalara's robust API and following best practices, you can ensure seamless tax compliance and improve your business operations. Whether you're managing a small business or an enterprise, integrating with Avalara can provide the accuracy and efficiency needed for effective tax management.
Read More
Ready to get started?