How to Get Users with the Mode API in PHP
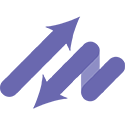
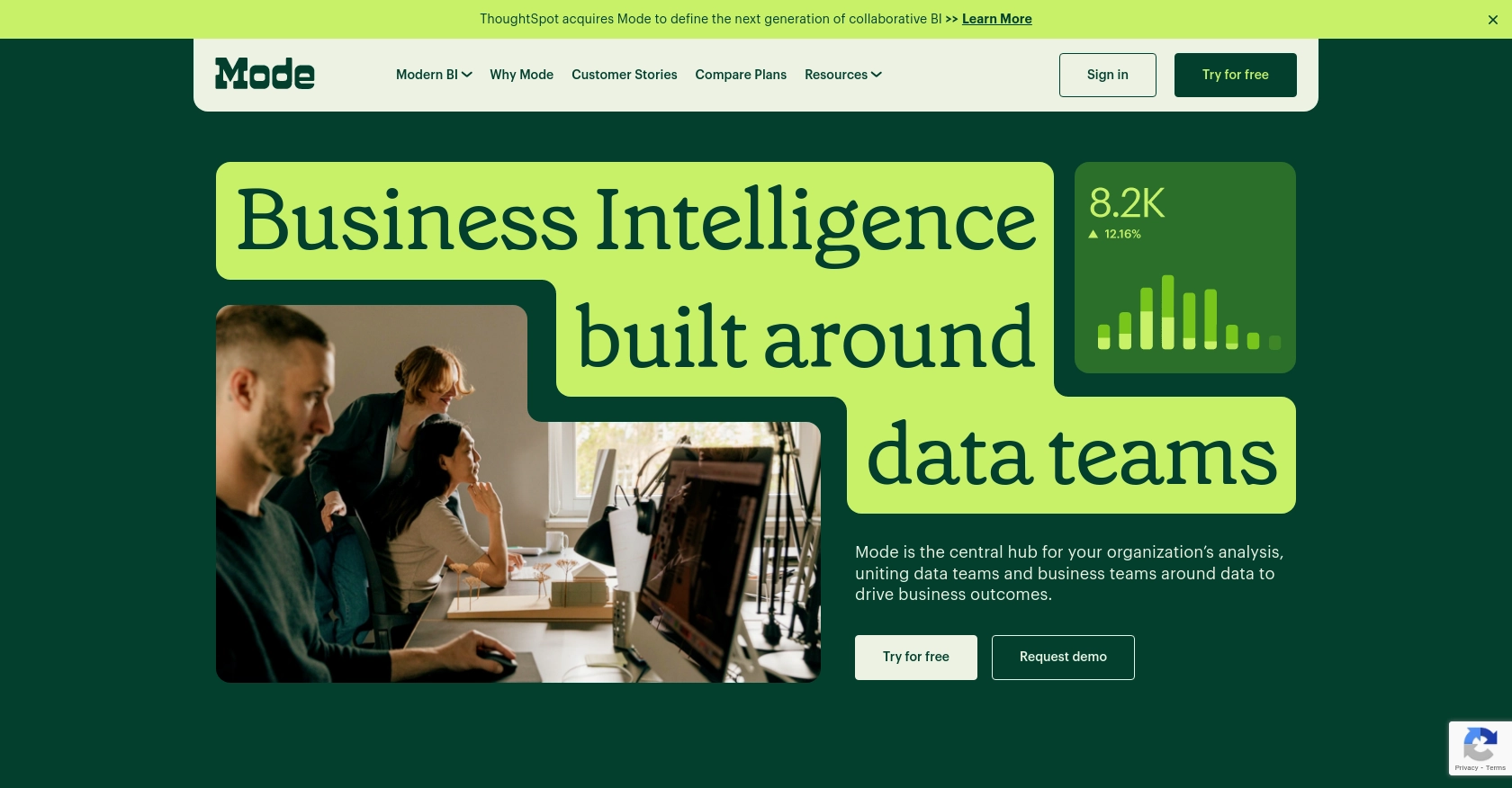
Introduction to Mode API for User Management
Mode is a collaborative analytics platform that empowers teams to work together seamlessly, leveraging data to make informed decisions. With its robust suite of analytics tools, Mode enables users to write queries, build reports, and manage data efficiently.
Integrating with Mode's API allows developers to programmatically access and manage user data within a Mode Workspace. This can be particularly useful for automating user management tasks, such as retrieving user information for analytics or reporting purposes. For example, a developer might want to fetch a list of all users in a workspace to analyze team collaboration metrics or to synchronize user data with another system.
Setting Up Your Mode API Test Account for User Management
Before you can start interacting with the Mode API to manage users, you'll need to set up a Mode account and obtain the necessary API credentials. This process involves creating a Mode Workspace and generating an API access token, which will allow you to authenticate your requests.
Step-by-Step Guide to Creating a Mode Workspace
- Create a Mode Account: Visit the Mode website and sign up for a new account if you don't already have one. Follow the on-screen instructions to complete the registration process.
- Set Up a Workspace: Once your account is created, log in and navigate to the Workspace section. Here, you can create a new Workspace where your team can collaborate on data analysis.
- Connect a Database: Optionally, connect a database to your Workspace or use public data available within Mode to start exploring the platform's capabilities.
Generating an API Access Token for Mode API Authentication
Mode requires basic authentication using API tokens to access its API. Follow these steps to create a Workspace API token:
- Navigate to API Settings: In your Mode Workspace, go to Settings > Privacy & Security > API.
- Create a New API Token: Click the gear icon and select "Create new API token." Provide a display name for the token and save it securely. Remember, the token will only be shown once.
- Store Your Credentials Securely: The API token consists of a public token (username) and a secret (password). Ensure these credentials are stored securely and not exposed in public code repositories.
With your Mode Workspace set up and API token generated, you're ready to start making API calls to manage users programmatically. In the next section, we'll explore how to use PHP to interact with the Mode API and retrieve user data.
For more detailed information on authentication, refer to the Mode API Authentication Documentation.
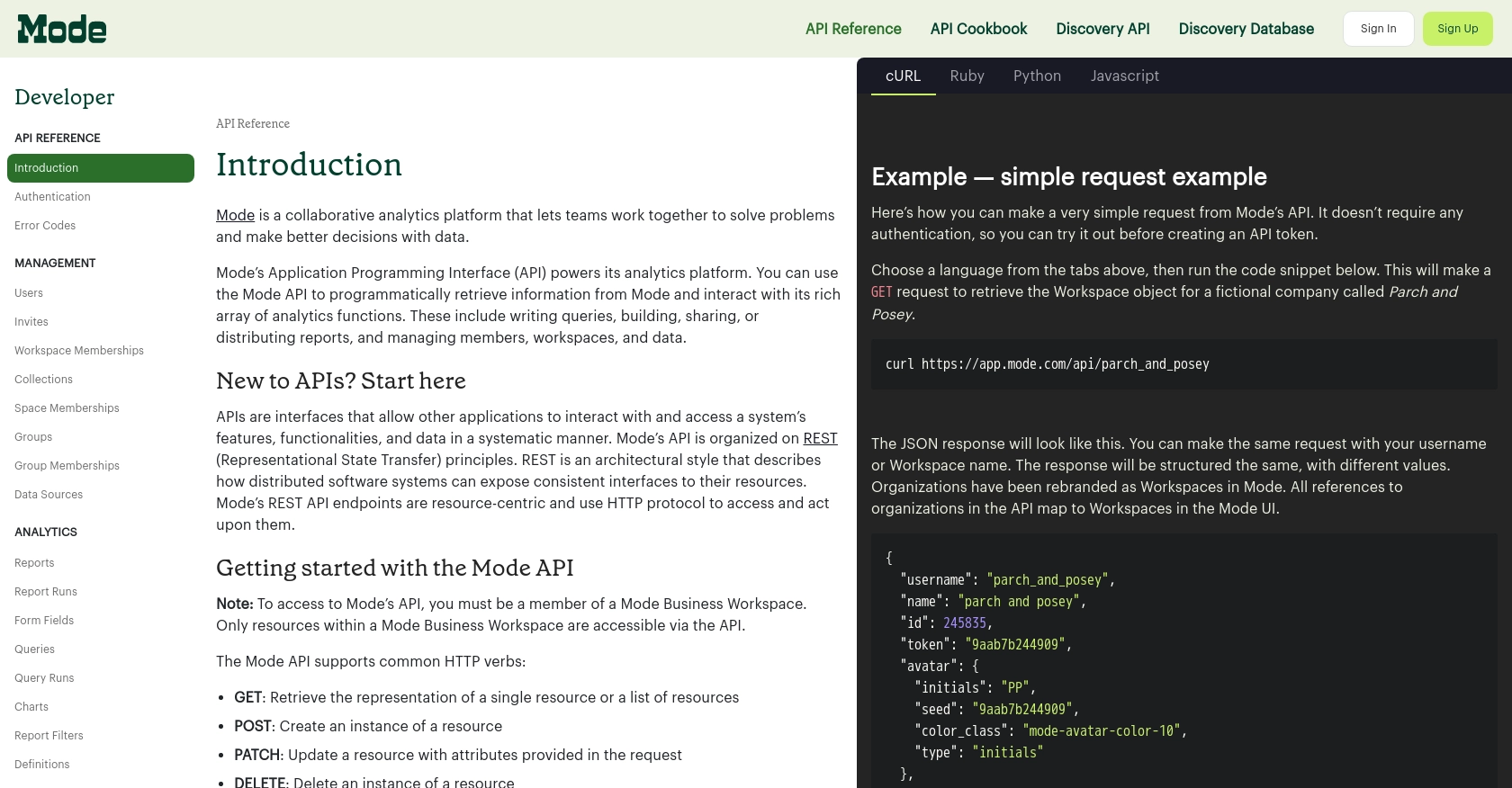
sbb-itb-96038d7
Making API Calls to Retrieve Users with Mode API in PHP
Now that you have set up your Mode Workspace and obtained the necessary API credentials, it's time to make API calls to retrieve user data using PHP. This section will guide you through the process of setting up your PHP environment, writing the code to interact with the Mode API, and handling potential errors.
Setting Up Your PHP Environment for Mode API Integration
Before you begin coding, ensure that your PHP environment is properly configured. You'll need PHP version 7.4 or higher and the cURL extension enabled to make HTTP requests.
- Check PHP Version: Run
php -v
in your terminal to verify your PHP version. - Enable cURL Extension: Ensure that the cURL extension is enabled in your
php.ini
file. You can check this by runningphp -m
and looking for "curl" in the list of modules.
Writing PHP Code to Retrieve Users from Mode API
With your environment set up, you can now write PHP code to interact with the Mode API and retrieve user data. Below is a sample script to get a list of all users in a Mode Workspace:
<?php
// Set your API credentials
$apiToken = 'your_api_token';
$apiSecret = 'your_api_secret';
$workspace = 'your_workspace_name';
// Set the API endpoint
$url = "https://app.mode.com/api/$workspace/memberships";
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
'Accept: application/hal+json'
]);
curl_setopt($ch, CURLOPT_USERPWD, "$apiToken:$apiSecret");
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Parse and display the response
$data = json_decode($response, true);
foreach ($data['_embedded']['memberships'] as $membership) {
echo "User: " . $membership['member_username'] . "<br>";
}
}
// Close cURL session
curl_close($ch);
?>
Replace your_api_token
, your_api_secret
, and your_workspace_name
with your actual Mode API credentials and workspace name.
Verifying API Call Success and Handling Errors
After executing the script, you should see a list of users in your Mode Workspace. If the request fails, ensure that your API credentials are correct and that your workspace name is accurate.
Mode API uses standard HTTP response codes to indicate success or failure. Here are some common error codes you might encounter:
- 401 Unauthorized: Authentication failed. Check your API token and secret.
- 404 Not Found: The specified workspace or resource could not be found. Verify the workspace name.
For more information on error handling, refer to the Mode API Error Codes Documentation.
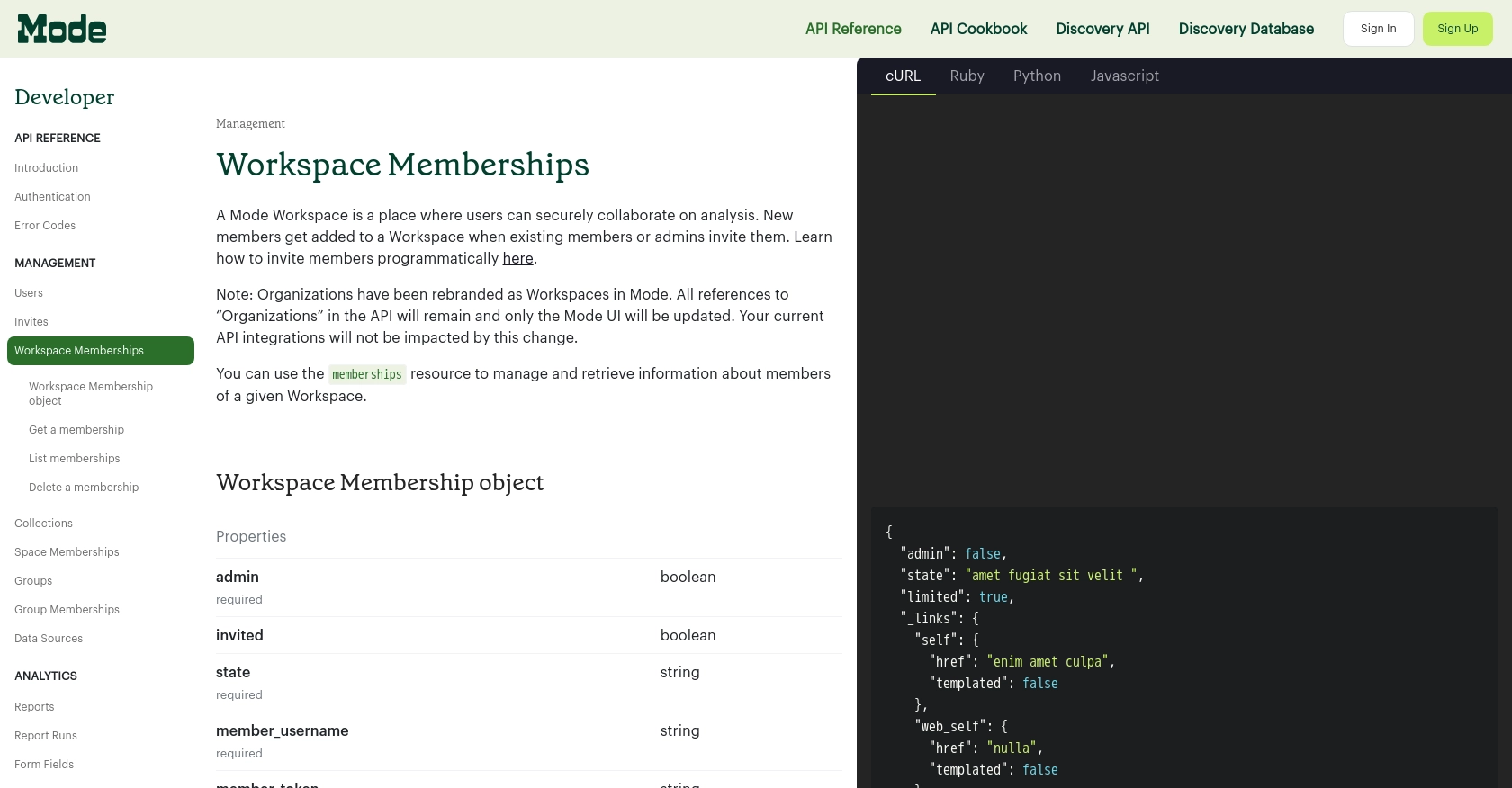
Best Practices for Using Mode API in PHP
When integrating with the Mode API, it's essential to follow best practices to ensure security, efficiency, and maintainability. Here are some recommendations:
- Securely Store API Credentials: Always store your API token and secret securely. Avoid hardcoding them in your scripts. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of Mode's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: When retrieving user data, ensure that fields are standardized to match your application's data model. This will facilitate easier integration with other systems.
Streamlining Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. With Endgrate, you can simplify this process by leveraging a unified API endpoint that connects to various platforms, including Mode.
Endgrate allows you to focus on your core product by outsourcing integrations, saving both time and resources. By building once for each use case, you can provide an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate today.
Read More
Ready to get started?