How to Get Users with the Zendesk Sell API in Javascript
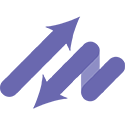
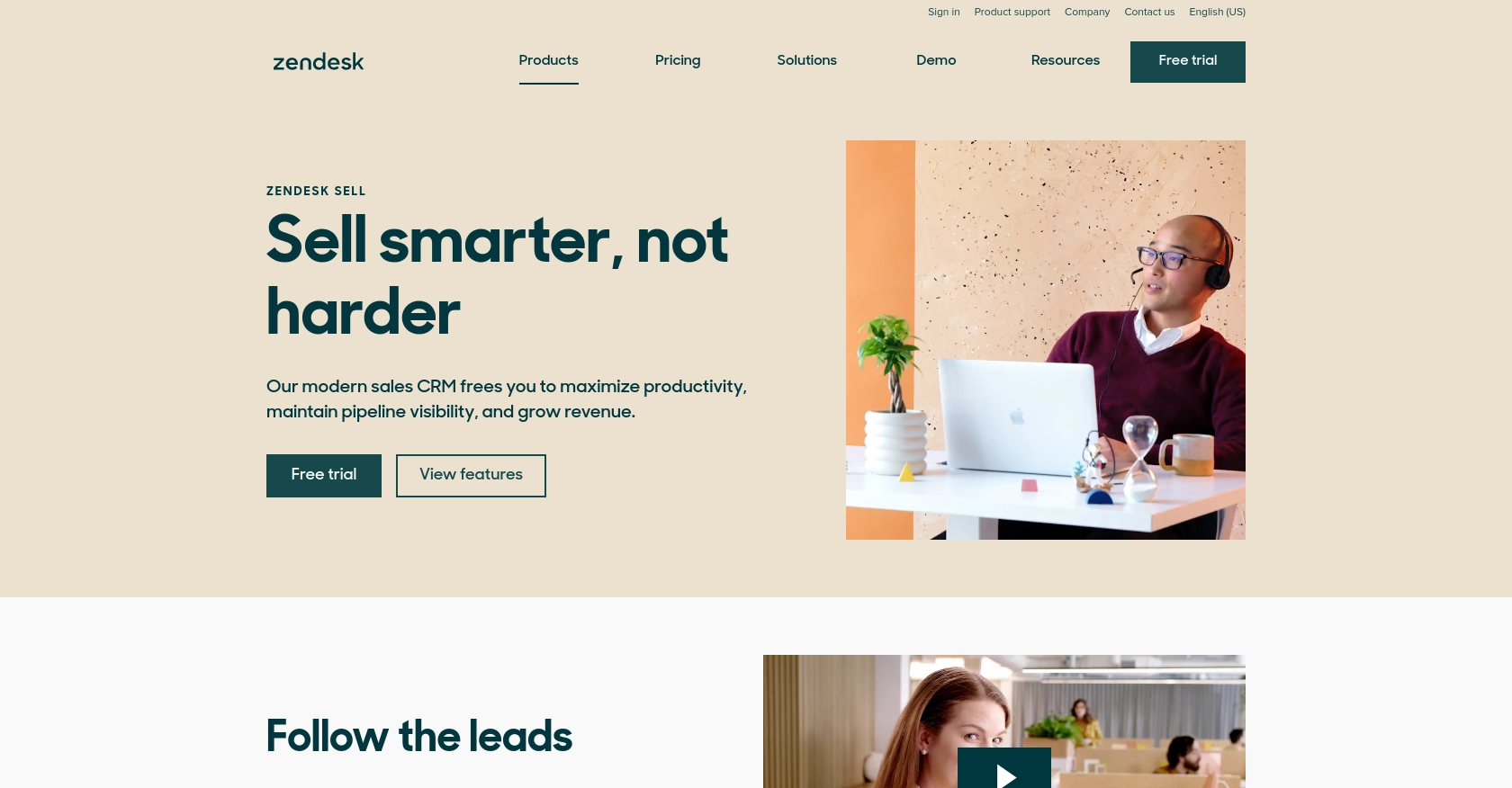
Introduction to Zendesk Sell API
Zendesk Sell is a powerful sales CRM platform designed to enhance productivity and streamline sales processes for businesses. It offers a comprehensive suite of tools that help sales teams manage leads, contacts, and deals efficiently. With its robust API, developers can build custom integrations to automate and optimize sales workflows.
Integrating with the Zendesk Sell API allows developers to access and manage user data, which can be crucial for creating personalized sales strategies or syncing user information across different platforms. For example, a developer might use the Zendesk Sell API to retrieve user details and integrate them into a custom dashboard, providing sales teams with real-time insights into user interactions and performance metrics.
Setting Up Your Zendesk Sell Test Account
Before you begin integrating with the Zendesk Sell API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Zendesk Sell offers a sandbox environment that mimics the production environment, providing a risk-free space for development and testing.
Creating a Zendesk Sell Sandbox Account
To get started, follow these steps to create a sandbox account:
- Visit the Zendesk Sell registration page and sign up for a free trial account.
- Once registered, log in to your Zendesk Sell dashboard.
- Navigate to the Admin section and select Sandbox from the menu.
- Follow the prompts to create a new sandbox environment. This may take a few minutes to set up.
Setting Up OAuth Authentication for Zendesk Sell API
The Zendesk Sell API uses OAuth 2.0 for authentication. Here's how to set up your application to use OAuth:
- In your Zendesk Sell dashboard, go to the Admin section and select API & Apps.
- Click on Create API Client to register a new application.
- Fill in the required details, including the Client Name, Redirect URL, and Scopes. Ensure you select the appropriate scopes for accessing user data.
- Once created, note down the Client ID and Client Secret. These will be used to authenticate your API requests.
Generating Access Tokens
With your OAuth application set up, you can now generate access tokens:
- Direct users to the authorization endpoint to obtain an authorization code:
- Exchange the authorization code for an access token by making a POST request to the token endpoint:
- Store the access token securely, as it will be used to authenticate API requests.
https://api.getbase.com/oauth2/authorize?client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI
curl -X POST https://api.getbase.com/oauth2/token \
-u "YOUR_CLIENT_ID:YOUR_CLIENT_SECRET" \
-d "grant_type=authorization_code" \
-d "code=AUTHORIZATION_CODE" \
-d "redirect_uri=YOUR_REDIRECT_URI"
With your sandbox account and OAuth authentication set up, you're ready to start making API calls to Zendesk Sell. This setup ensures that you can test and develop integrations efficiently and securely.
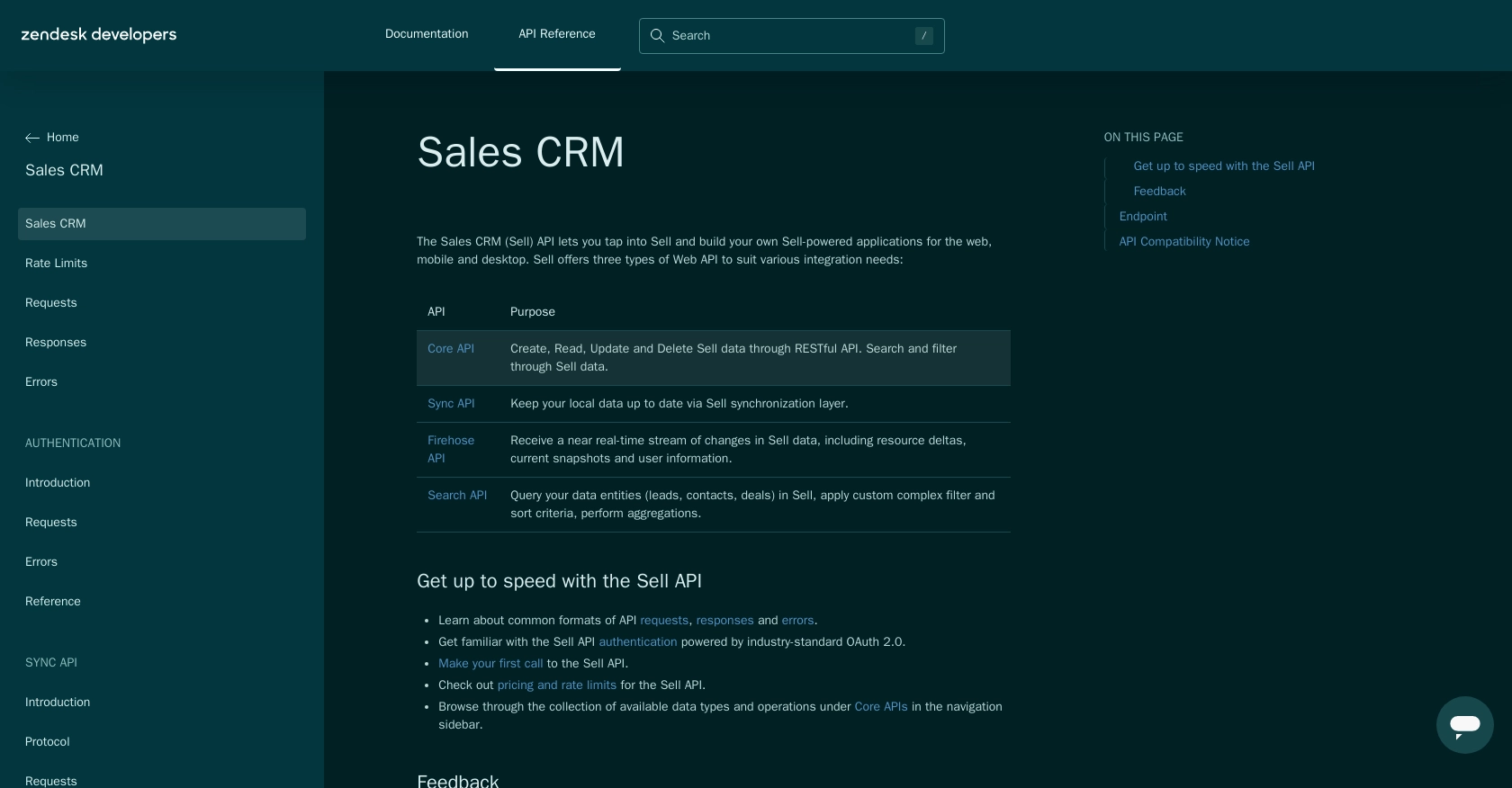
sbb-itb-96038d7
Making API Calls to Retrieve Users with Zendesk Sell API in JavaScript
To interact with the Zendesk Sell API and retrieve user data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Zendesk Sell API
Before making API calls, ensure you have Node.js installed on your machine. This will allow you to run JavaScript code outside of a browser. Additionally, you'll need the axios
library to simplify HTTP requests.
- Install Node.js from the official website.
- Open your terminal and run the following command to install
axios
:
npm install axios
Writing JavaScript Code to Retrieve Users from Zendesk Sell API
With your environment set up, you can now write the JavaScript code to make a GET request to the Zendesk Sell API and retrieve user data.
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.getbase.com/v2/users';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN',
'Accept': 'application/json'
};
// Function to get users
async function getUsers() {
try {
const response = await axios.get(endpoint, { headers });
const users = response.data.items;
console.log('Users:', users);
} catch (error) {
console.error('Error fetching users:', error.response ? error.response.data : error.message);
}
}
// Call the function
getUsers();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth setup. This code uses axios
to send a GET request to the Zendesk Sell API, retrieves the user data, and logs it to the console.
Verifying API Call Success and Handling Errors
After running the code, you should see a list of users printed in the console. To verify the request's success, check the response status code. A status code of 200 indicates success.
If an error occurs, the code will catch it and print an error message. Common error codes include:
- 401 Unauthorized: The access token is missing or invalid. Ensure your token is correct and not expired.
- 429 Too Many Requests: You've exceeded the rate limit of 36,000 requests per hour. Implement rate limiting strategies to avoid this.
For more details on error handling, refer to the Zendesk Sell API error documentation.
By following these steps, you can efficiently retrieve user data from Zendesk Sell using JavaScript, enabling seamless integration into your applications.
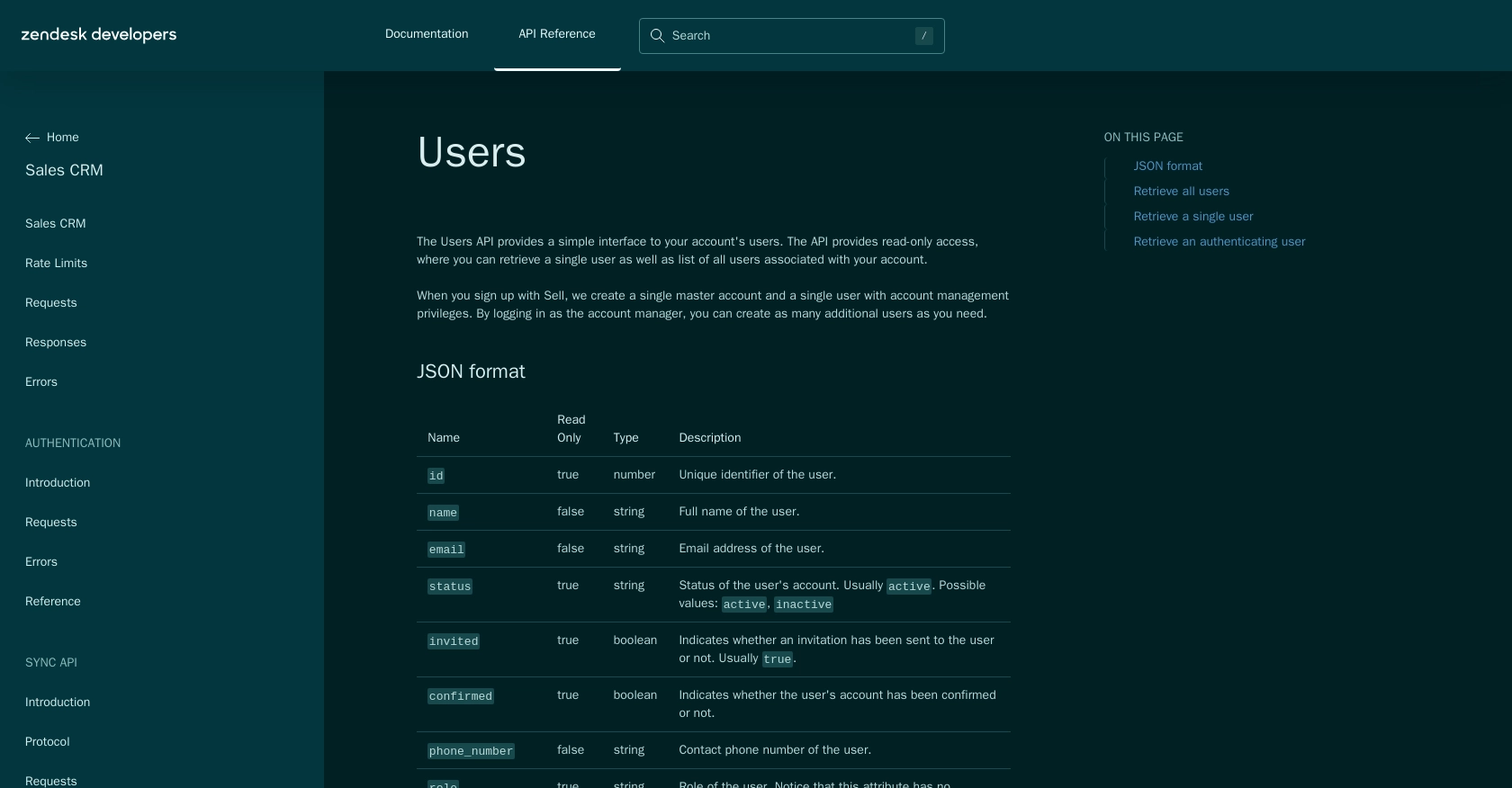
Conclusion and Best Practices for Using Zendesk Sell API in JavaScript
Integrating with the Zendesk Sell API using JavaScript provides a powerful way to access and manage user data, enhancing your sales processes and enabling seamless data synchronization across platforms. By following the steps outlined in this guide, you can efficiently retrieve user information and integrate it into your applications.
Best Practices for Secure and Efficient API Integration
- Secure Storage of Credentials: Always store your OAuth credentials, such as the Client ID, Client Secret, and access tokens, securely. Consider using environment variables or secure vaults to protect sensitive information.
- Implement Rate Limiting: Be mindful of the API rate limits, which allow up to 36,000 requests per hour. Implement strategies like caching and request batching to minimize unnecessary API calls and avoid hitting rate limits.
- Handle Errors Gracefully: Ensure your application can handle common error responses, such as 401 Unauthorized and 429 Too Many Requests, by implementing retry logic and user notifications.
- Data Transformation and Standardization: Consider transforming and standardizing data fields to match your application's requirements, ensuring consistency across different data sources.
Streamlining Integration with Endgrate
While integrating with the Zendesk Sell API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to connect with multiple platforms through a single endpoint.
By leveraging Endgrate, you can save time and resources, focusing on your core product development while providing an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
With these best practices and tools, you can enhance your sales operations and deliver a seamless experience for your users.
Read More
- https://endgrate.com/provider/zendesksell
- https://developer.zendesk.com/api-reference/sales-crm/introduction/
- https://developer.zendesk.com/api-reference/sales-crm/rate-limits/
- https://developer.zendesk.com/api-reference/sales-crm/requests/
- https://developer.zendesk.com/api-reference/sales-crm/errors/
- https://developer.zendesk.com/api-reference/sales-crm/authentication/introduction/
- https://developer.zendesk.com/api-reference/sales-crm/authentication/requests/#client-authentication
- https://developer.zendesk.com/api-reference/sales-crm/resources/users/
Ready to get started?