How to Get Users with the Zendesk Support API in Javascript
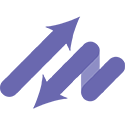
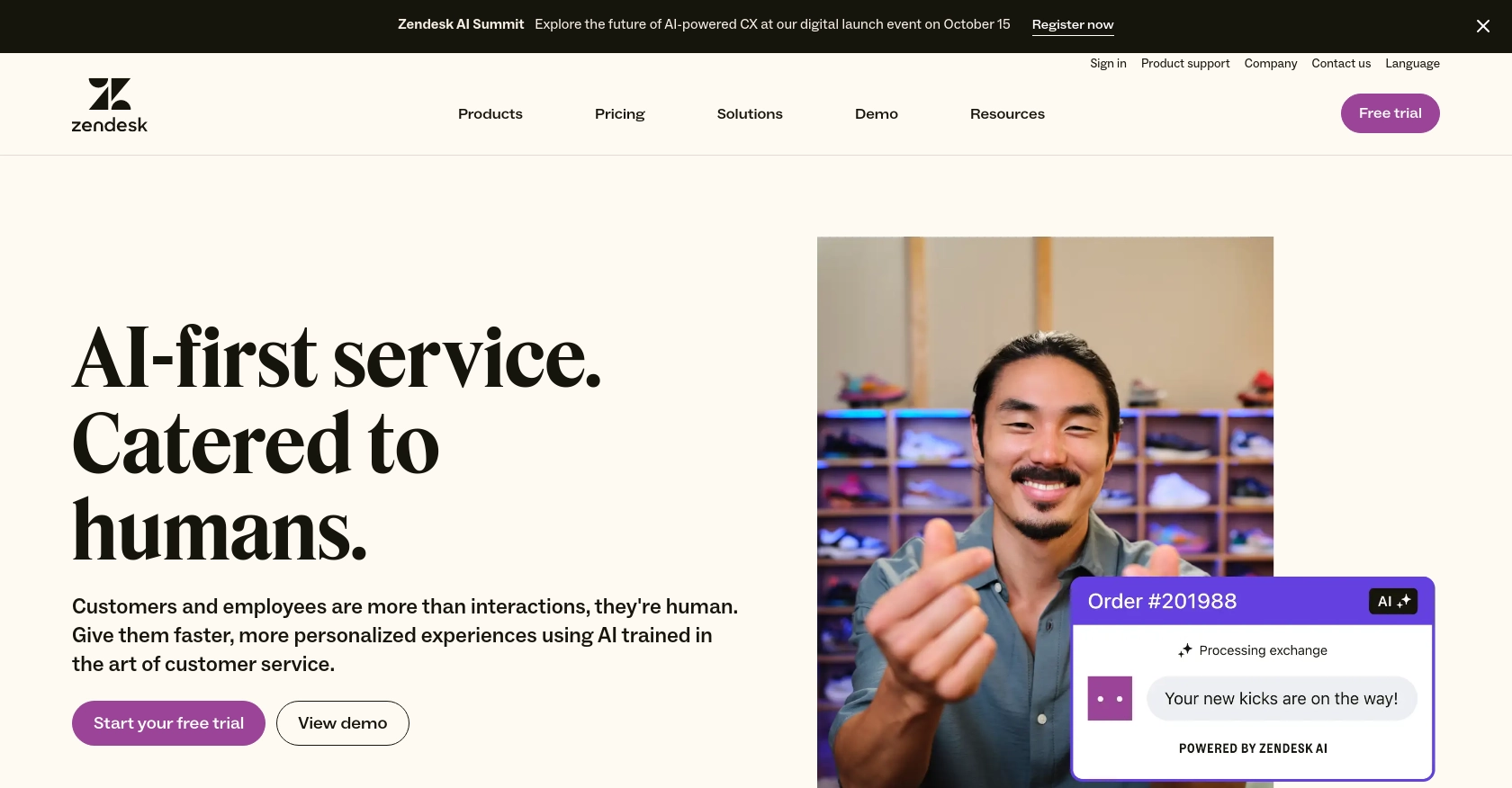
Introduction to Zendesk Support API
Zendesk Support is a powerful customer service platform that enables businesses to manage and enhance their customer support operations. It offers a suite of tools designed to streamline ticket management, customer interactions, and support workflows, making it a popular choice for companies aiming to improve their customer service experience.
Developers may want to integrate with the Zendesk Support API to access and manage user data, automate support processes, or enhance customer interaction capabilities. For example, a developer might use the Zendesk Support API to retrieve user information and integrate it with a CRM system, ensuring that support agents have access to the most up-to-date customer data.
Setting Up Your Zendesk Support Sandbox Account
Before you can start interacting with the Zendesk Support API, you'll need to set up a sandbox account. This will allow you to test your API integrations without affecting your live data. Zendesk provides a sandbox environment that mirrors your production account, making it an ideal space for development and testing.
Creating a Zendesk Support Sandbox Account
If you don't already have a Zendesk account, you can sign up for a free trial on the Zendesk website. This trial will give you access to the sandbox environment. Follow these steps to create your sandbox account:
- Visit the Zendesk registration page and sign up for a free trial.
- Once your account is created, navigate to the Admin Center.
- In the Admin Center, go to Sandbox under the Manage section.
- Click on Create Sandbox to set up your sandbox environment.
Configuring OAuth Authentication for Zendesk Support API
The Zendesk Support API uses OAuth 2.0 for authentication, providing a secure way to access data without storing user passwords. To configure OAuth authentication, follow these steps:
- In the Admin Center, navigate to Apps and integrations > APIs > Zendesk API.
- Click on the OAuth Clients tab and then Add OAuth client.
- Fill in the required fields:
- Client Name: Enter a name for your application.
- Description: Optionally, provide a description of your app.
- Redirect URLs: Enter the URL where Zendesk should redirect users after they authorize your app. Ensure it's a secure URL (https).
- Click Save. Note down the Client ID and Client Secret as you'll need them for authentication.
Generating OAuth Access Tokens
With your OAuth client configured, you can now generate access tokens to authenticate API requests:
- Direct users to the Zendesk authorization page using the following URL format:
https://{subdomain}.zendesk.com/oauth/authorizations/new?response_type=code&client_id={your_client_id}&redirect_uri={your_redirect_url}&scope=read
- Handle the authorization response to retrieve the authorization code.
- Exchange the authorization code for an access token by making a POST request to:
https://{subdomain}.zendesk.com/oauth/tokens
- Include the following parameters in your request body:
- grant_type: "authorization_code"
- code: The authorization code received
- client_id: Your Client ID
- client_secret: Your Client Secret
- redirect_uri: The same redirect URL used in the authorization request
Once you have the access token, you can use it to authenticate your API requests by including it in the Authorization header:
Authorization: Bearer {access_token}
For more detailed information on OAuth authentication, refer to the Zendesk OAuth documentation.
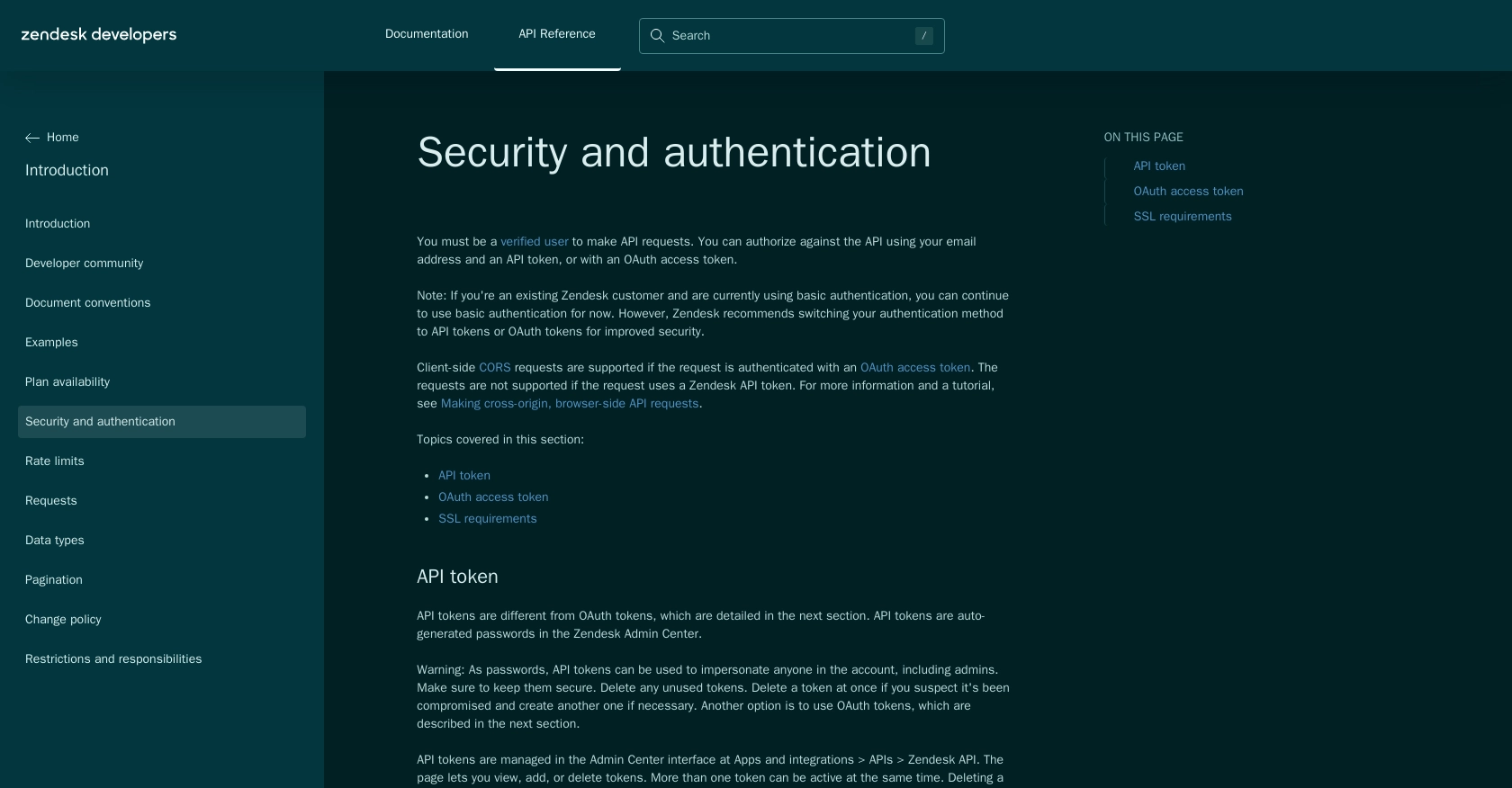
sbb-itb-96038d7
Making API Calls to Retrieve Users with Zendesk Support API in JavaScript
To interact with the Zendesk Support API using JavaScript, you'll need to ensure you have the correct setup and dependencies. This section will guide you through the process of making API calls to retrieve user data, including setting up your environment and handling responses.
Setting Up Your JavaScript Environment for Zendesk Support API
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment needed to execute JavaScript code outside of a browser.
Additionally, you'll need the Axios library to handle HTTP requests. Install Axios using npm (Node Package Manager) with the following command:
npm install axios
Example Code to Retrieve Users from Zendesk Support API
Once your environment is set up, you can proceed to write the JavaScript code to retrieve users from the Zendesk Support API. Below is a sample code snippet demonstrating how to make a GET request to the API:
const axios = require('axios');
// Define the API endpoint and headers
const endpoint = 'https://{subdomain}.zendesk.com/api/v2/users.json';
const headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer {access_token}' // Replace with your access token
};
// Make a GET request to the API
axios.get(endpoint, { headers })
.then(response => {
// Handle the response data
const users = response.data.users;
users.forEach(user => {
console.log(`ID: ${user.id}, Name: ${user.name}`);
});
})
.catch(error => {
// Handle errors
if (error.response) {
console.error(`Error: ${error.response.status} - ${error.response.data.error}`);
} else {
console.error(`Error: ${error.message}`);
}
});
Replace {subdomain}
with your Zendesk subdomain and {access_token}
with the access token you obtained during the OAuth setup.
Verifying API Call Success and Handling Errors
After running the code, you should see a list of users printed to the console. This indicates a successful API call. If the request fails, the error handling code will output the error status and message, helping you diagnose the issue.
Common error codes include:
- 401 Unauthorized: Check if your access token is valid and not expired.
- 404 Not Found: Ensure the API endpoint URL is correct.
- 429 Too Many Requests: You have exceeded the rate limit. Refer to the Zendesk rate limits documentation for more details.
Checking the Retrieved User Data in Zendesk Support
To verify the retrieved data, log in to your Zendesk Support sandbox account and navigate to the Users section. The users listed in the console output should match those in your Zendesk account.
For more information on the Users API, refer to the Zendesk Users API documentation.
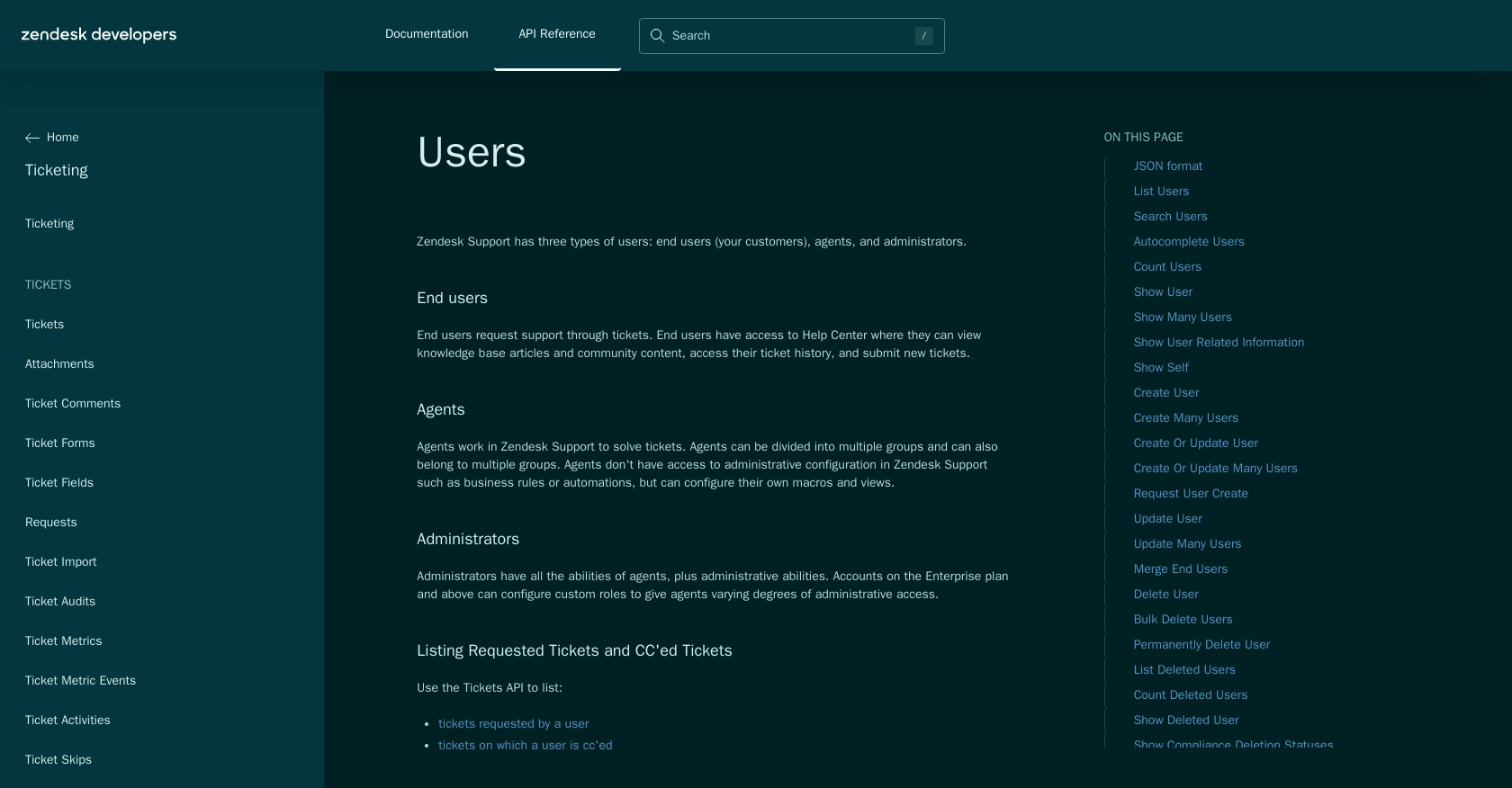
Conclusion and Best Practices for Using Zendesk Support API in JavaScript
Integrating with the Zendesk Support API using JavaScript can significantly enhance your customer support capabilities by providing seamless access to user data and automating support processes. By following the steps outlined in this guide, you can efficiently retrieve user information and integrate it into your applications.
Best Practices for Managing Zendesk Support API Integrations
- Securely Store Credentials: Always store your OAuth credentials, such as client ID and client secret, securely. Avoid hardcoding them in your source code.
- Handle Rate Limiting: Be mindful of Zendesk's rate limits. If you encounter a 429 error, implement a retry mechanism that respects the
Retry-After
header. For more details, refer to the Zendesk rate limits documentation. - Data Standardization: Ensure that the data retrieved from Zendesk is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage API call failures gracefully. Log errors for further analysis and debugging.
Enhancing Your Integration Experience with Endgrate
While building integrations with the Zendesk Support API can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution for managing multiple integrations with a unified API endpoint. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration management.
- Focus on your core product development instead of handling multiple integration complexities.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration processes by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zendesksupport
- https://developer.zendesk.com/api-reference/introduction/security-and-auth/
- https://support.zendesk.com/hc/en-us/articles/4408845965210-Using-OAuth-authentication-with-your-application
- https://developer.zendesk.com/api-reference/introduction/rate-limits/
- https://developer.zendesk.com/api-reference/introduction/pagination/
- https://developer.zendesk.com/api-reference/ticketing/users/users/
Ready to get started?