How to Get Vendors with the Sage 100 API in Python
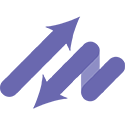
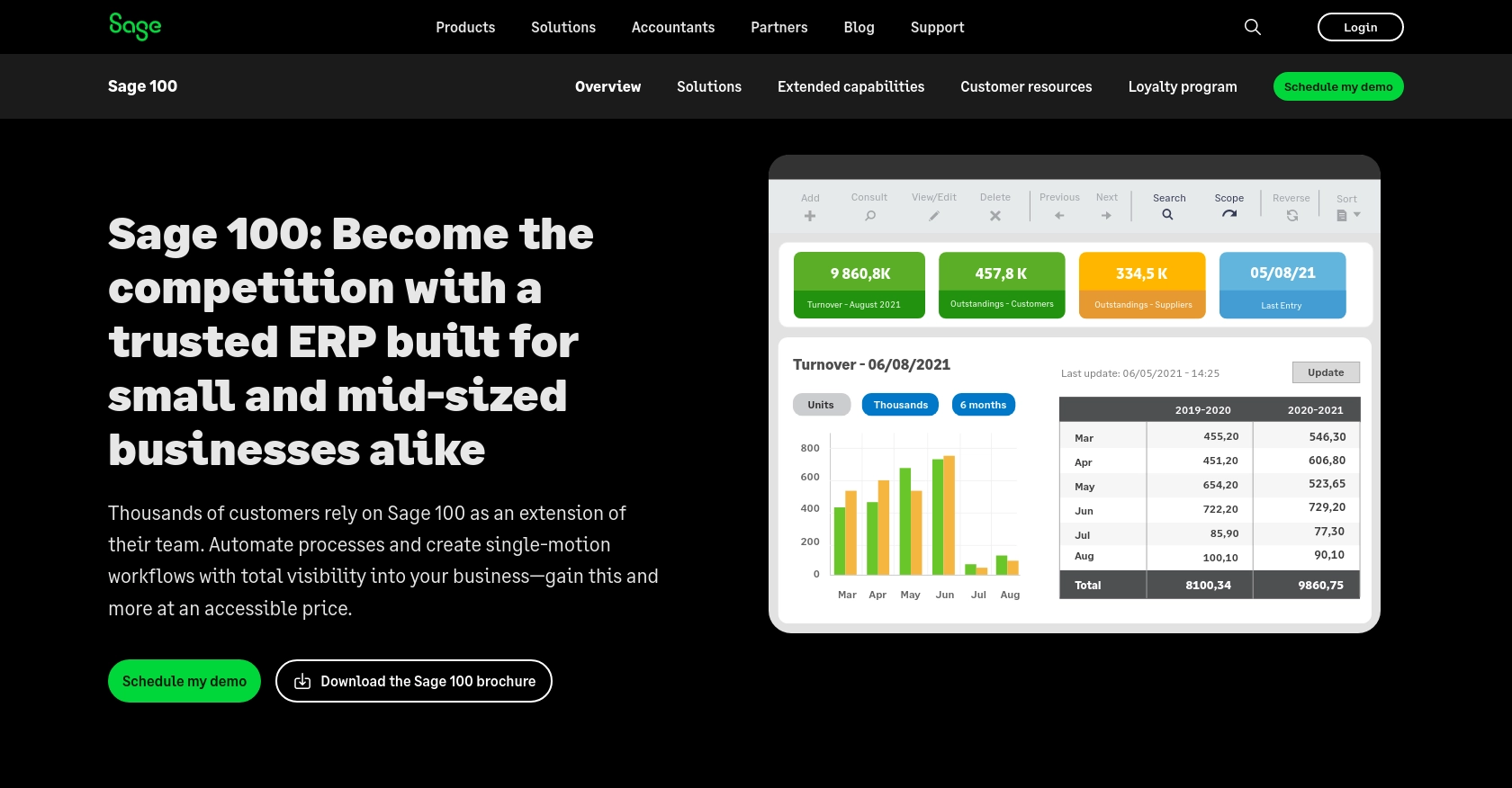
Introduction to Sage 100 Integration
Sage 100 is a comprehensive ERP solution designed to streamline business operations, including accounting, inventory management, and customer relationship management. It is widely used by small to medium-sized businesses seeking to enhance efficiency and gain better control over their financial processes.
Integrating with the Sage 100 API allows developers to access and manage various business data, such as vendor information, directly from the Sage 100 system. For example, a developer might want to retrieve vendor details to automate purchase order processes or synchronize vendor data with other business applications.
Setting Up Your Sage 100 Test/Sandbox Account
Before you can start interacting with the Sage 100 API, it's essential to set up a test or sandbox account. This environment allows developers to safely experiment with API calls without affecting live data.
Installing and Configuring the Sage 100 ODBC Driver
To connect to the Sage 100 database, you need to install and configure the Sage 100 ODBC driver. Follow these steps to set it up:
- Access the ODBC Data Source Administrator on your system.
- Create a DSN (Data Source Name) that connects to the Sage 100 ERP system using the correct server, database, and authentication settings.
- Ensure the Sage 100 ODBC driver is installed and properly configured.
For detailed instructions, refer to the Sage 100 ODBC Driver Configuration Guide.
Creating a Sage 100 Test Environment
To create a test environment, follow these steps:
- Log in to your Sage 100 account.
- Navigate to the system configuration settings.
- Set up a test company or use an existing sandbox environment provided by Sage 100.
Configuring Authentication for Sage 100 API Access
Sage 100 uses a custom authentication method. Here's how to configure it:
- Ensure your DSN is correctly set up with the necessary authentication details.
- Use the
System.Data.Odbc
namespace in your Python code to establish a connection. - Create an
OdbcConnection
object, providing the DSN name in the connection string. - Open the connection using
OdbcConnection.Open()
.
Remember to handle exceptions using try-catch blocks to manage connection errors and timeouts.
Testing the ODBC Connection
After configuring the ODBC driver, test the connection to ensure everything is set up correctly:
- Open the ODBC Data Source Administrator.
- Select the DSN you created and click on "Test Connection."
- If the connection is successful, you are ready to proceed with API calls.
For more information, visit the Sage 100 ODBC Driver Configuration Guide.
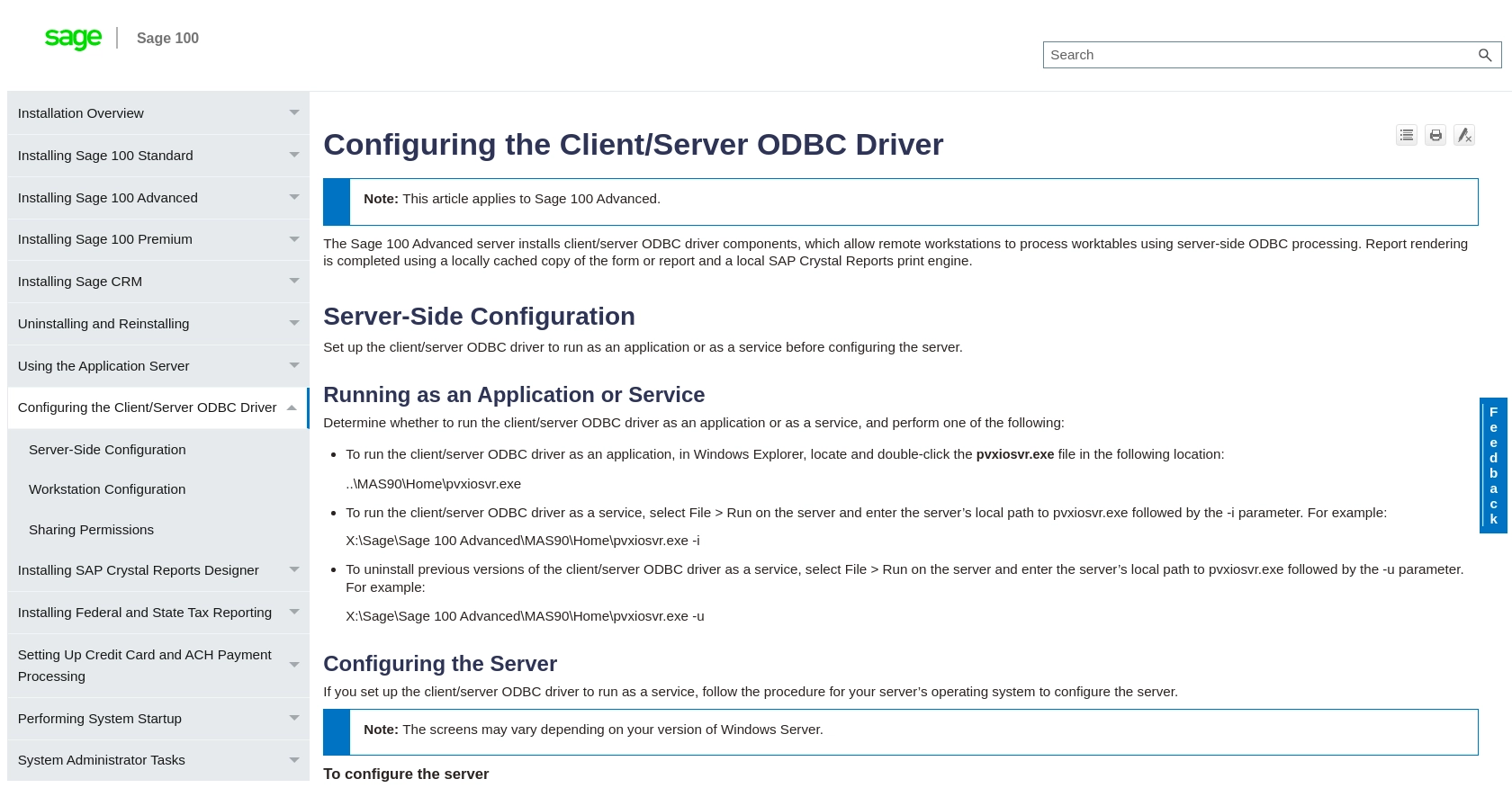
sbb-itb-96038d7
Making API Calls to Retrieve Vendors from Sage 100 Using Python
To interact with the Sage 100 API and retrieve vendor information, you'll need to use Python to establish a connection and execute the necessary queries. This section will guide you through the process of making API calls using Python, ensuring you have the right setup and code to get started.
Setting Up Your Python Environment for Sage 100 API
Before making API calls, ensure your Python environment is properly configured. You'll need Python 3.x and the necessary libraries to interact with the Sage 100 ODBC driver.
- Ensure Python 3.x is installed on your machine. You can download it from the official Python website.
- Install the
pyodbc
library, which allows Python to connect to ODBC databases. Use the following command:
pip install pyodbc
Writing Python Code to Connect and Retrieve Vendor Data from Sage 100
With your environment set up, you can now write the Python code to connect to the Sage 100 database and retrieve vendor information. Follow these steps:
- Import the necessary libraries in your Python script:
import pyodbc
- Establish a connection to the Sage 100 database using the DSN you configured earlier:
# Define the DSN and connection details
dsn = 'Your_DSN_Name'
user = 'Your_Username'
password = 'Your_Password'
# Establish the connection
connection = pyodbc.connect(f'DSN={dsn};UID={user};PWD={password}')
- Create a cursor object and execute the SQL query to retrieve vendor data:
# Create a cursor from the connection
cursor = connection.cursor()
# Define the SQL query to retrieve vendors
query = "SELECT * FROM AP_Vendor"
# Execute the query
cursor.execute(query)
- Fetch and display the vendor data:
# Fetch all rows from the executed query
vendors = cursor.fetchall()
# Loop through the results and print vendor details
for vendor in vendors:
print(f"Vendor ID: {vendor.VendorID}, Name: {vendor.VendorName}")
- Close the connection after retrieving the data:
# Close the cursor and connection
cursor.close()
connection.close()
Verifying API Call Success and Handling Errors
After executing the API call, verify the success by checking the output in your terminal. The vendor details should match the data in your Sage 100 test environment. If you encounter any errors, ensure your DSN and authentication details are correct.
Implement error handling using try-except blocks to manage potential connection issues or query errors:
try:
# Connection and query execution code here
except pyodbc.Error as e:
print(f"Error: {e}")
finally:
# Ensure resources are freed
if cursor:
cursor.close()
if connection:
connection.close()
By following these steps, you can efficiently retrieve vendor data from Sage 100 using Python, enabling seamless integration with your business applications.
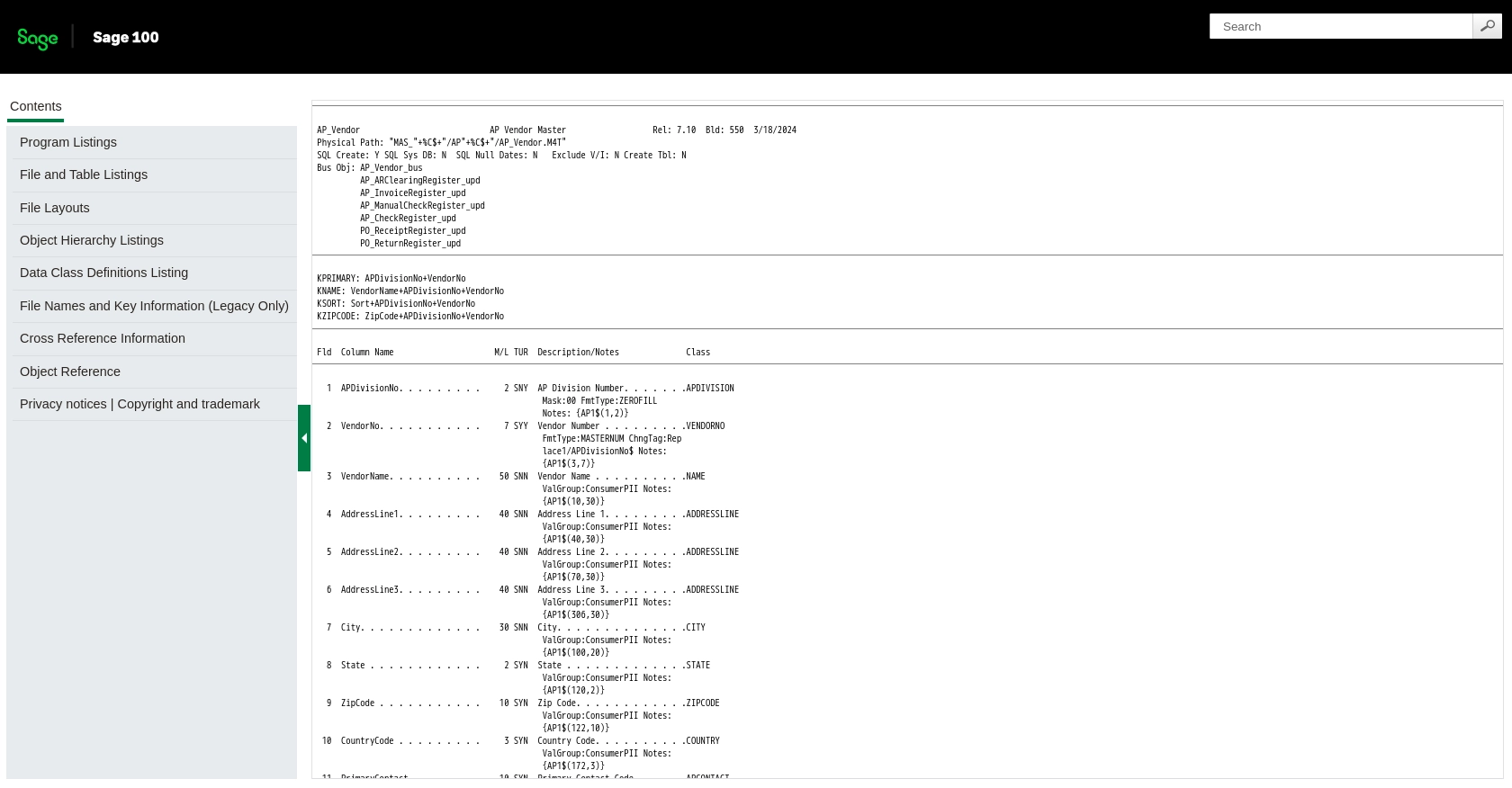
Conclusion and Best Practices for Integrating Sage 100 API with Python
Integrating with the Sage 100 API using Python provides a powerful way to automate and streamline business processes by accessing and managing vendor data directly from the Sage 100 system. By following the steps outlined in this guide, developers can efficiently retrieve vendor information and integrate it with other business applications.
Best Practices for Secure and Efficient Sage 100 API Integration
- Securely Store Credentials: Always store user credentials securely in your database, using encryption to protect sensitive information.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage 100 API and implement logic to handle these limits gracefully, ensuring your application remains responsive.
- Data Standardization: Transform and standardize data fields to ensure consistency across different systems and applications.
- Error Handling: Implement robust error handling to manage connection issues, timeouts, and query errors effectively.
Streamline Your Integration Process with Endgrate
While integrating with the Sage 100 API can be a rewarding endeavor, it can also be time-consuming and complex. Endgrate offers a solution to simplify this process by providing a unified API endpoint that connects to multiple platforms and services, including Sage 100.
By using Endgrate, developers can save time and resources, focusing on their core product while outsourcing integrations. This approach allows for a more intuitive integration experience for your customers and reduces the need to build multiple integrations for different platforms.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate and discover a more efficient way to manage your business integrations.
Read More
Ready to get started?