Using the Airtable API to Get Users in Javascript
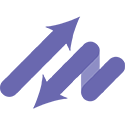
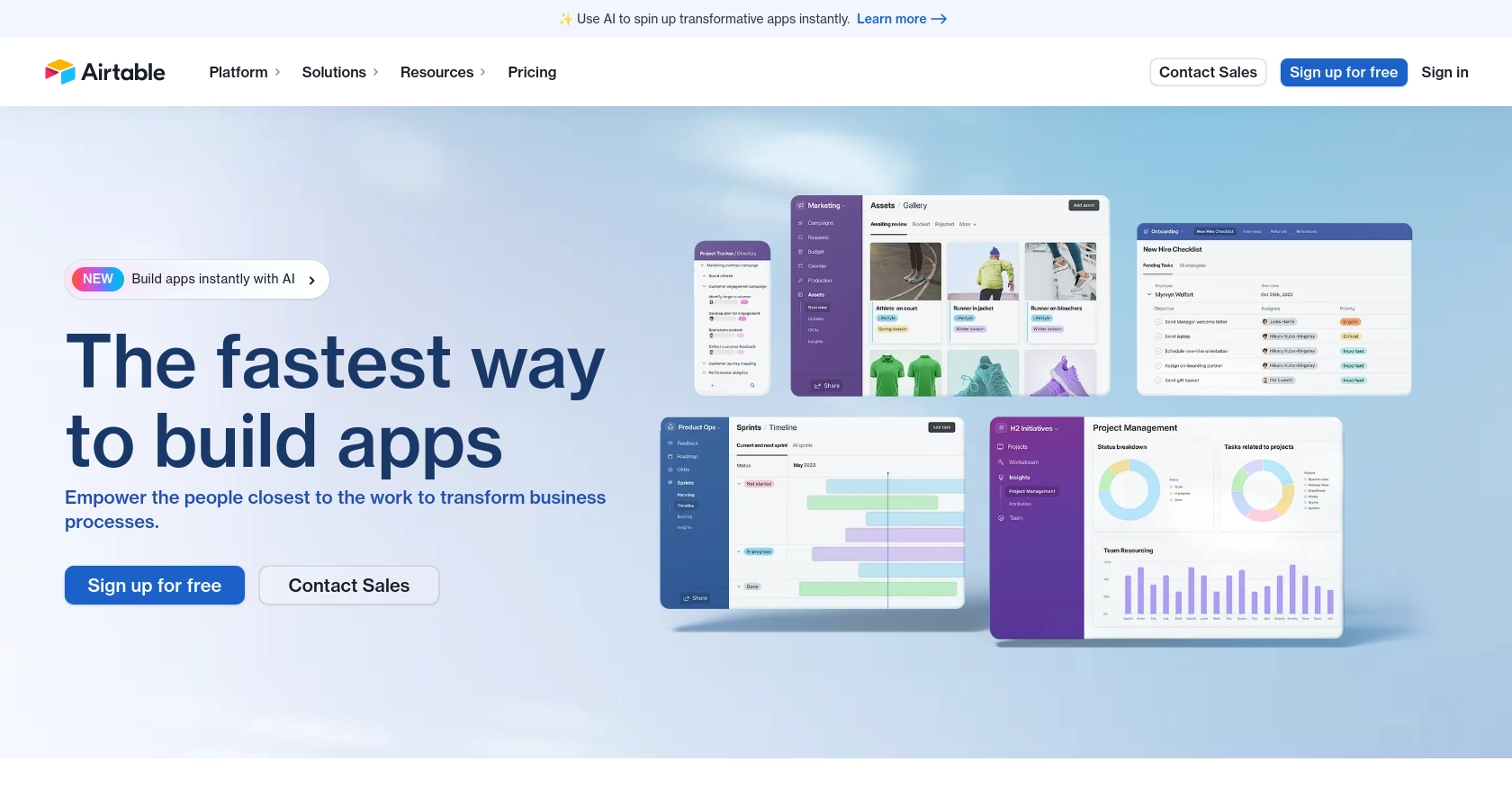
Introduction to Airtable API Integration
Airtable is a versatile platform that combines the simplicity of a spreadsheet with the power of a database, making it a popular choice for businesses looking to organize and manage data efficiently. Its user-friendly interface and robust API capabilities allow developers to create custom integrations that enhance productivity and streamline workflows.
Connecting with the Airtable API can empower developers to access and manage user data seamlessly. For example, you might want to retrieve user information to synchronize it with another system, ensuring that your team has up-to-date data across platforms. This article will guide you through using the Airtable API to get user details using JavaScript, providing a practical example of how to leverage this integration in your projects.
Setting Up Your Airtable Test Account for API Integration
Before you can start interacting with the Airtable API using JavaScript, you need to set up a test account. This will allow you to safely experiment with API calls without affecting your production data. Airtable provides a straightforward process to create a sandbox environment for development purposes.
Creating an Airtable Account
If you don't already have an Airtable account, you can sign up for a free account on the Airtable website. Follow the on-screen instructions to complete the registration process. If you already have an account, simply log in.
Generating an OAuth Access Token for Airtable API
Since Airtable uses OAuth for authentication, you'll need to create an OAuth app to obtain the necessary credentials. Follow these steps to set up your OAuth app:
- Navigate to the Airtable OAuth creation page.
- Click on "Create an OAuth App" and fill in the required details, such as the app name, description, and redirect URI. Ensure that the redirect URI is HTTPS unless using localhost.
- Select the scopes you need for your integration. For accessing user data, ensure you include the
enterprise.user:read
scope. - Once your app is created, you'll receive a client ID and client secret. Keep these credentials secure as they are essential for obtaining an access token.
Obtaining the Access Token
With your OAuth app set up, you can now obtain an access token to authenticate your API requests:
- Direct your users to the authorization URL generated by your OAuth app. This URL will include your client ID and requested scopes.
- Once the user authorizes your app, they'll be redirected to your specified redirect URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to the Airtable token endpoint. Include your client ID, client secret, and the authorization code in the request.
Refer to the Airtable OAuth reference for detailed instructions on the token exchange process.
Testing Your Setup
After obtaining the access token, you can test your setup by making a simple API call to retrieve user information. This will confirm that your authentication process is correctly configured and that you can interact with the Airtable API.
For more information on authentication, visit the Airtable authentication documentation.
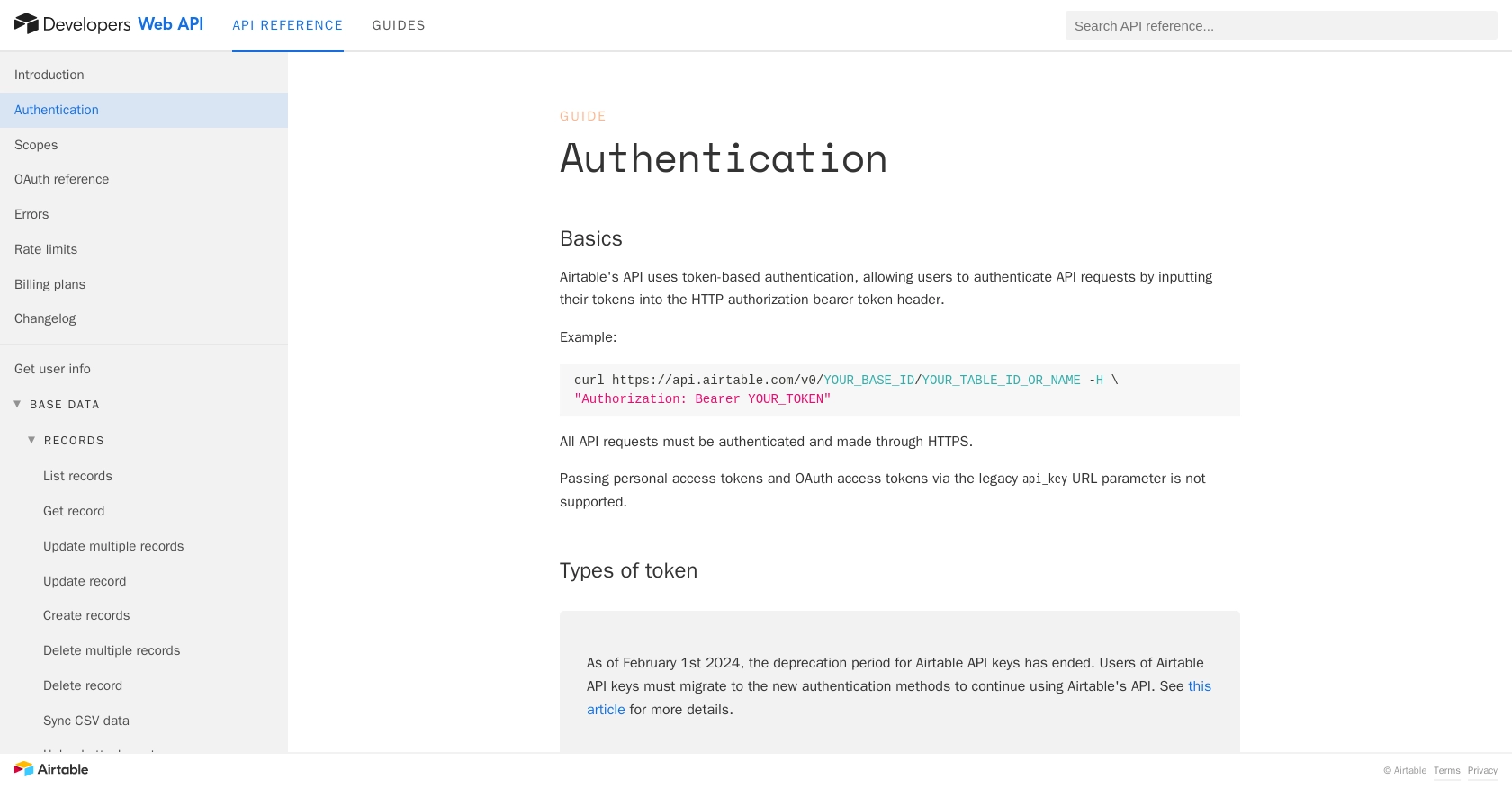
sbb-itb-96038d7
How to Make an Airtable API Call Using JavaScript
To interact with the Airtable API and retrieve user information, you'll need to use JavaScript to make HTTP requests. This section will guide you through setting up your environment and executing the API call to get user details.
Setting Up Your JavaScript Environment for Airtable API Integration
Before making API calls, ensure you have the necessary tools and libraries installed. You'll need Node.js and npm (Node Package Manager) to manage dependencies. Follow these steps to set up your environment:
- Ensure Node.js is installed on your machine. You can download it from the official Node.js website.
- Initialize a new Node.js project by running
npm init -y
in your project directory. - Install the Axios library to handle HTTP requests by executing
npm install axios
.
Executing the Airtable API Call to Retrieve User Information
With your environment set up, you can now write the JavaScript code to make an API call to Airtable. The following example demonstrates how to retrieve user details using Axios:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.airtable.com/v0/meta/enterpriseAccounts/{enterpriseAccountId}/users';
const headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
};
// Function to get users by email or ID
async function getUsers() {
try {
const response = await axios.get(endpoint, { headers });
const users = response.data.users;
// Display user information
users.forEach(user => {
console.log(`Name: ${user.name}, Email: ${user.email}`);
});
} catch (error) {
console.error('Error fetching users:', error.response.data);
}
}
// Call the function
getUsers();
Replace YOUR_ACCESS_TOKEN
with the access token obtained from the OAuth process and {enterpriseAccountId}
with your specific enterprise account ID.
Verifying the Success of Your Airtable API Request
After running the script, you should see the user details printed in the console. This confirms that your API call was successful. If you encounter any errors, refer to the Airtable error documentation for troubleshooting tips.
Handling Errors and Understanding Airtable API Error Codes
When making API calls, it's crucial to handle potential errors gracefully. Airtable's API provides specific error codes to help diagnose issues:
- 400 Bad Request: Check the request syntax and parameters.
- 401 Unauthorized: Verify your access token and permissions.
- 429 Too Many Requests: You have exceeded the rate limit. Wait and retry after 30 seconds.
For a complete list of error codes, visit the Airtable error documentation.
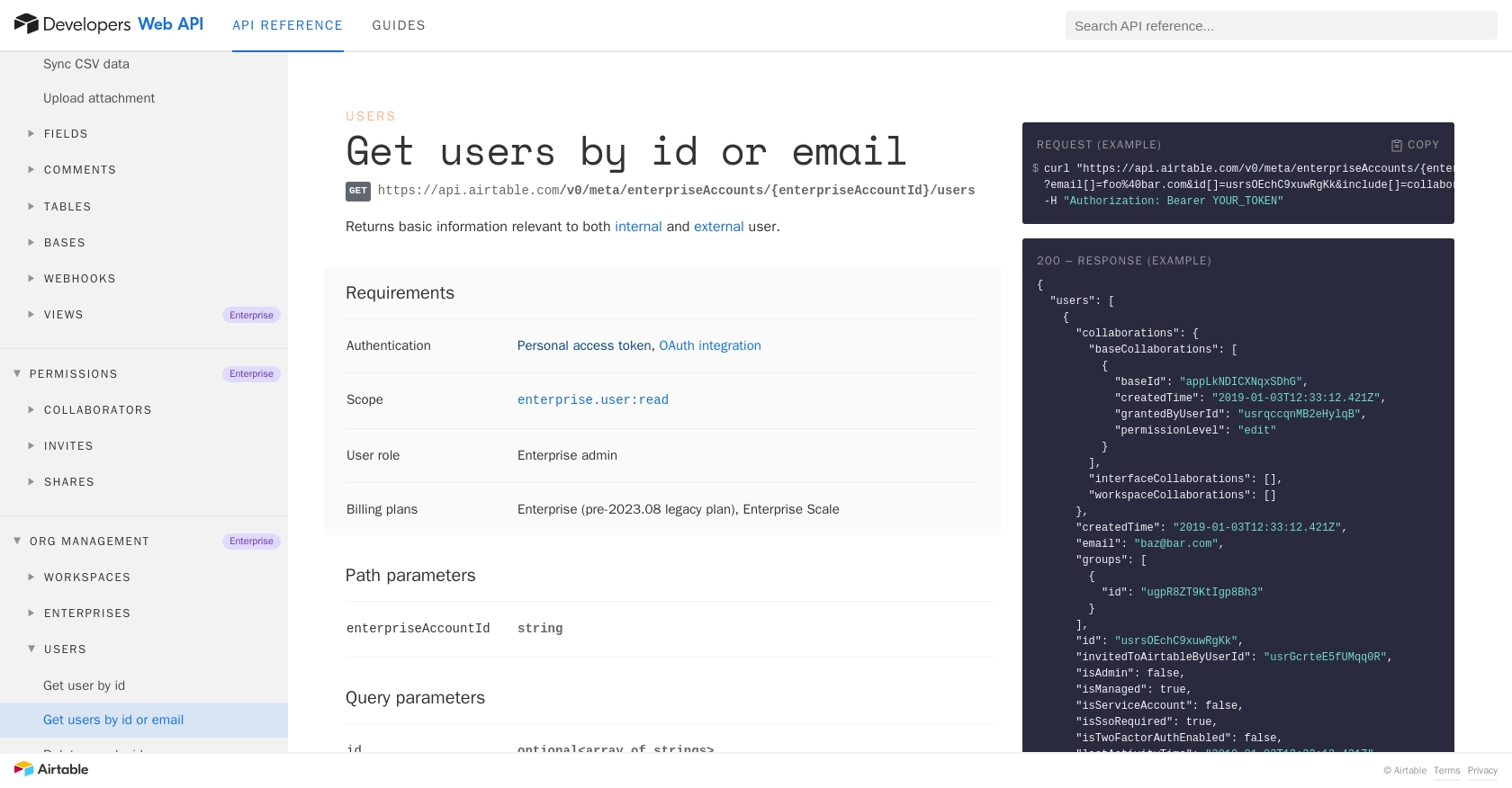
Conclusion and Best Practices for Using Airtable API with JavaScript
Integrating with the Airtable API using JavaScript provides a powerful way to manage and synchronize user data across platforms. By following the steps outlined in this guide, you can efficiently retrieve user information and ensure your applications remain up-to-date with the latest data.
Best Practices for Secure and Efficient Airtable API Integration
- Secure Storage of Credentials: Always store your OAuth credentials securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Airtable imposes a rate limit of 5 requests per second per base. Implement back-off strategies and retry logic to handle 429 status codes gracefully. For more details, refer to the Airtable rate limits documentation.
- Data Standardization: Ensure that data retrieved from Airtable is standardized and transformed as needed to fit your application's requirements.
Streamlining Integrations with Endgrate
For developers looking to simplify the integration process, consider using Endgrate. By leveraging Endgrate's unified API, you can connect to multiple platforms, including Airtable, with ease. This allows you to focus on your core product while outsourcing the complexities of integration management.
Visit Endgrate to learn how you can save time and resources by building once for each use case and providing an intuitive integration experience for your customers.
Read More
- https://endgrate.com/provider/airtable
- https://airtable.com/developers/web/api/authentication
- https://airtable.com/developers/web/api/scopes
- https://airtable.com/developers/web/api/oauth-reference
- https://airtable.com/developers/web/api/errors
- https://airtable.com/developers/web/api/rate-limits
- https://airtable.com/developers/web/api/get-users-by-id-or-email
Ready to get started?