Using the Nimble API to Create or Update Companies (with Python examples)
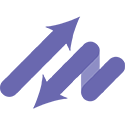
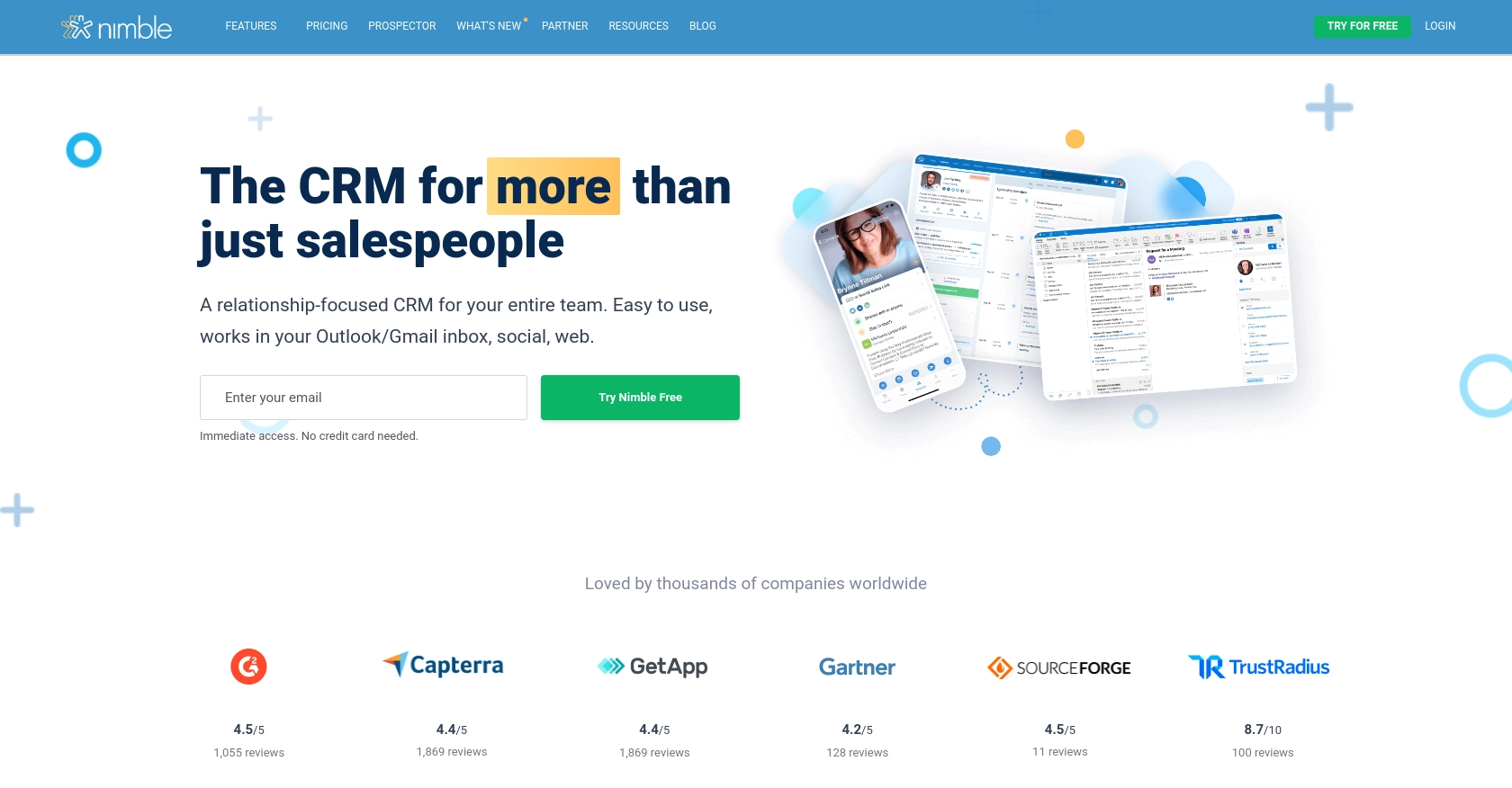
Introduction to Nimble CRM API Integration
Nimble is a relationship-focused CRM platform that helps businesses manage their contacts, tasks, and deals efficiently. With its seamless integration capabilities, Nimble allows for the synchronization of data across various platforms, making it an ideal choice for businesses looking to streamline their customer relationship management processes.
Developers may want to integrate with Nimble's API to automate the management of company data, such as creating or updating company records. For example, a developer could use the Nimble API to automatically update company information from an external data source, ensuring that the CRM always contains the most current data.
Setting Up Your Nimble Test Account for API Integration
Before you can start using the Nimble API to create or update company records, you need to set up a test account. This will allow you to safely experiment with the API without affecting your live data.
Creating a Nimble Account
If you don't already have a Nimble account, you can sign up for a free trial on the Nimble website. Follow the instructions to create your account and log in.
Generating an API Key for Nimble
To interact with the Nimble API, you'll need to generate an API key. This key will authenticate your requests and allow you to access your account data programmatically.
- Log in to your Nimble account.
- Navigate to Settings and select API Token.
- Click on Generate New Token.
- Provide a description for your token and click Generate.
- Copy the generated API key and store it securely, as you'll need it for API requests.
Note: Only Nimble Account Administrators can generate API keys. Ensure that you have the necessary permissions.
Configuring API Access in Nimble
Once you have your API key, you need to configure your API requests to use it. All API requests must include the API key in the HTTP header for authentication.
import requests
apikey = "YOUR_API_KEY"
headers = {"Authorization": "Bearer " + apikey}
response = requests.get("https://app.nimble.com/api/v1/myself", headers=headers)
print(response.json())
Replace YOUR_API_KEY
with the API key you generated earlier. This example demonstrates how to make a simple request to verify your API setup.
For more detailed guidance on generating an API key, refer to the Nimble API documentation.
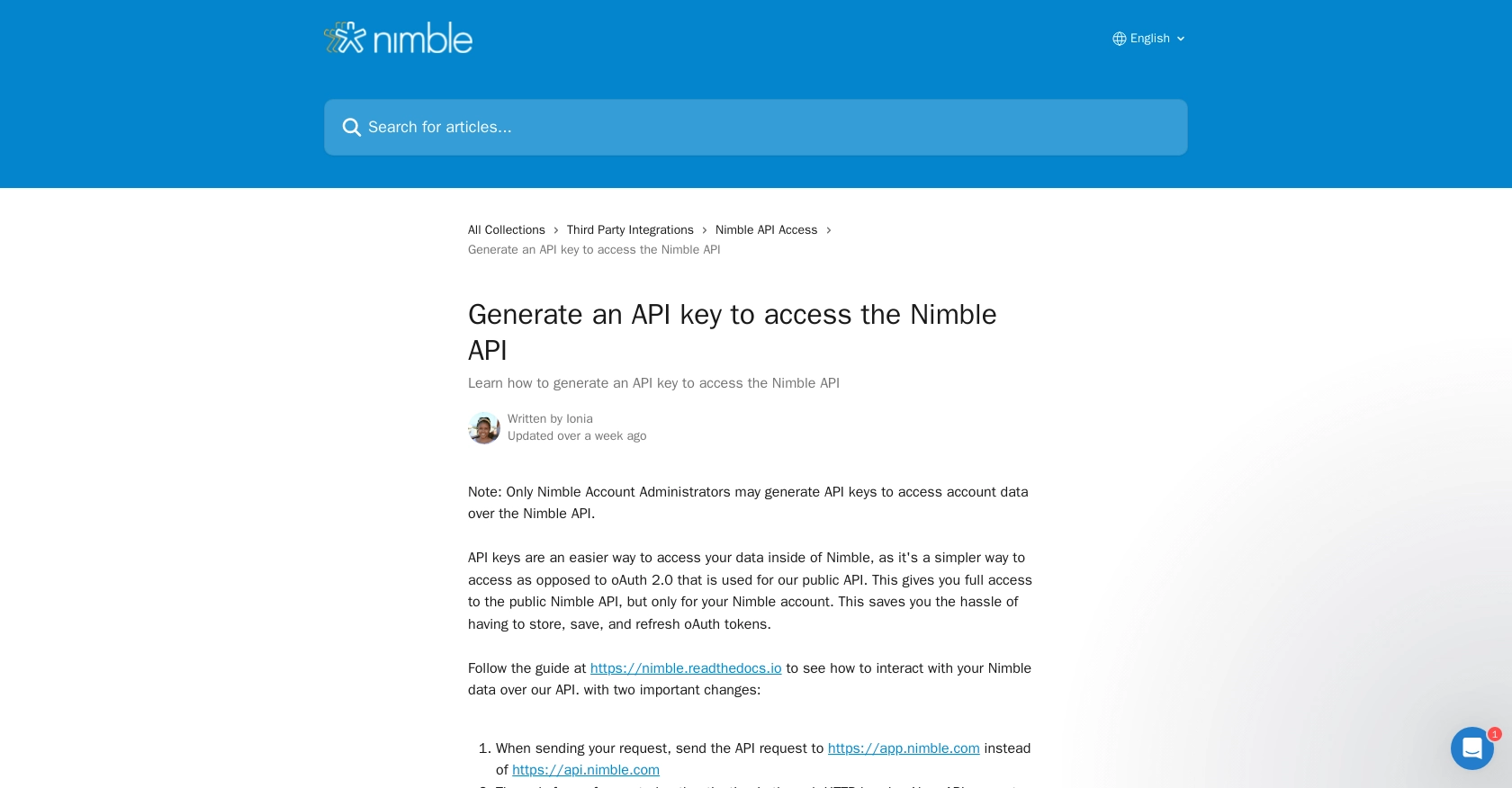
sbb-itb-96038d7
Making API Calls to Create or Update Companies in Nimble Using Python
To effectively interact with the Nimble API for creating or updating company records, you'll need to use Python. This section will guide you through the process, including setting up your environment and executing API calls.
Setting Up Your Python Environment for Nimble API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
pip install requests
Creating a Company Record in Nimble
To create a new company in Nimble, you'll need to send a POST request to the appropriate endpoint with the necessary company data. Here's a step-by-step guide:
import requests
# Set the API endpoint for creating a company
url = "https://app.nimble.com/api/v1/companies"
# Set the request headers with your API key
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_API_KEY"
}
# Define the company data
company_data = {
"fields": {
"company name": [{"value": "Example Company"}],
"description": [{"value": "A sample company description"}]
}
}
# Send the POST request to create the company
response = requests.post(url, json=company_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Company created successfully:", response.json())
else:
print("Failed to create company:", response.json())
Replace YOUR_API_KEY
with your actual API key. This code snippet demonstrates how to create a company by specifying its name and description.
Updating an Existing Company Record in Nimble
To update an existing company, you'll need the company's ID. Use a PUT request to modify the company's details:
import requests
# Set the API endpoint for updating a company
company_id = "COMPANY_ID"
url = f"https://app.nimble.com/api/v1/companies/{company_id}"
# Set the request headers with your API key
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer YOUR_API_KEY"
}
# Define the updated company data
updated_data = {
"fields": {
"description": [{"value": "Updated company description"}]
}
}
# Send the PUT request to update the company
response = requests.put(url, json=updated_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Company updated successfully:", response.json())
else:
print("Failed to update company:", response.json())
Replace COMPANY_ID
and YOUR_API_KEY
with the respective values. This example updates the company's description.
Handling API Response and Errors
After making an API call, it's crucial to handle the response correctly. A successful request will return a status code of 200. If the request fails, check the response for error details. Common error codes include:
- 409: Validation error, often due to missing required fields.
- 404: Resource not found, typically due to an incorrect company ID.
For more information on error handling, refer to the Nimble API documentation.
Conclusion and Best Practices for Nimble API Integration
Integrating with the Nimble API to create or update company records can significantly enhance your CRM capabilities by automating data management tasks. By following the steps outlined in this guide, you can efficiently manage company data within Nimble using Python.
Best Practices for Secure and Efficient API Usage
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Validation: Ensure that all required fields are included in your API requests to avoid validation errors.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users.
Transforming and Standardizing Data Fields
When integrating with Nimble, consider transforming and standardizing data fields to ensure consistency across different platforms. This practice can help maintain data integrity and improve the quality of your CRM data.
Enhancing Integration with Endgrate
For developers looking to streamline their integration processes further, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Endgrate provides a unified API endpoint that simplifies the integration experience, making it easier for your team to manage multiple platforms efficiently.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
Ready to get started?