Using the Google Sheets API to Get Records (with Python examples)
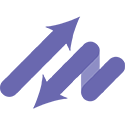
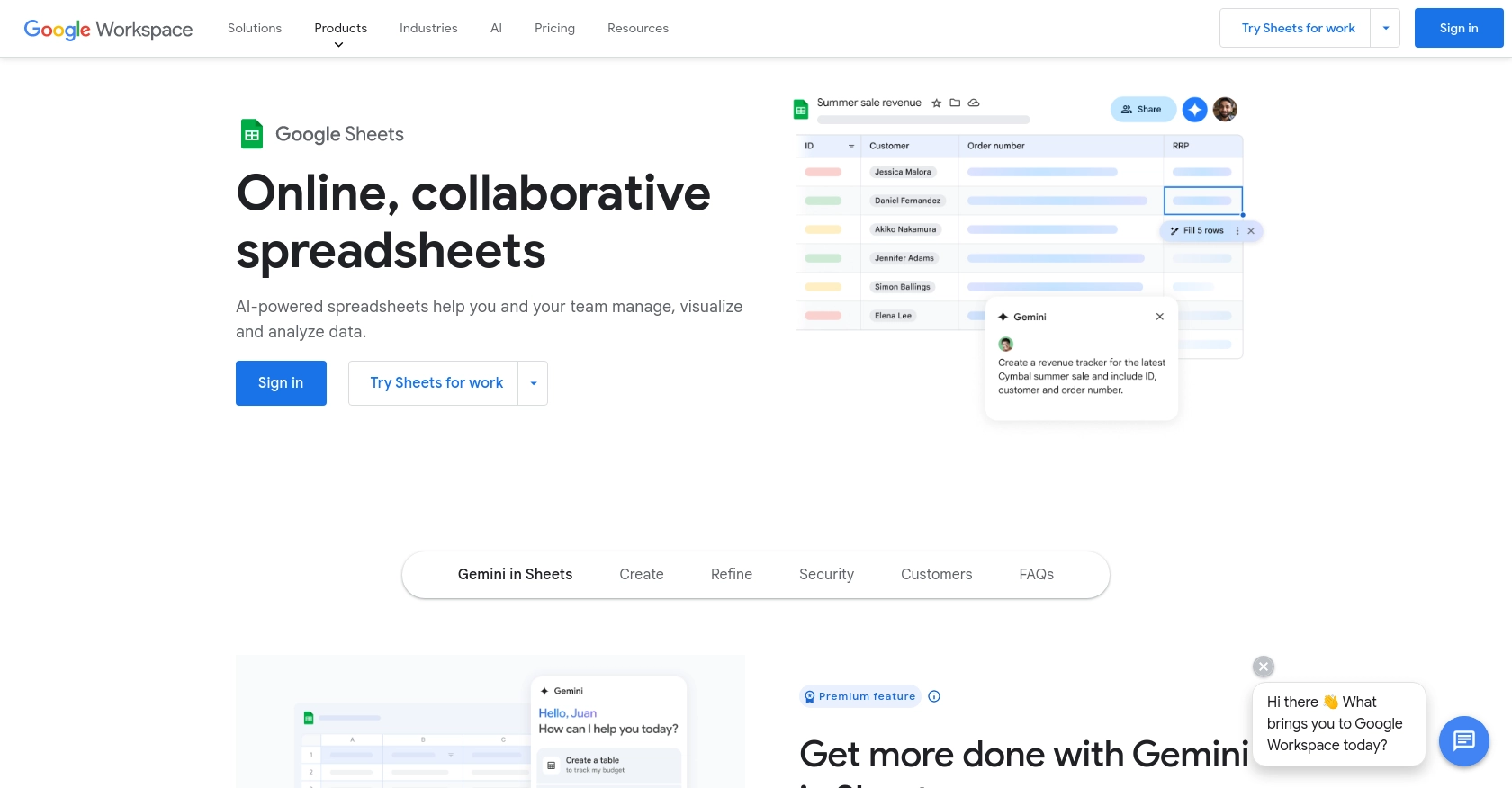
Introduction to Google Sheets API
Google Sheets is a powerful, cloud-based spreadsheet application that allows users to create, edit, and collaborate on spreadsheets in real-time. It is part of the Google Workspace suite and is widely used for data management, analysis, and visualization.
Integrating with the Google Sheets API enables developers to automate and enhance spreadsheet functionalities, such as retrieving and updating data programmatically. For example, a developer might use the Google Sheets API to fetch records from a spreadsheet and use that data to generate reports or populate dashboards, streamlining data workflows and improving efficiency.
Setting Up Your Google Sheets API Test Account
To begin interacting with the Google Sheets API, you need to set up a Google Cloud project and enable the API. This setup allows you to authenticate and authorize your application to access Google Sheets data securely.
Create a Google Cloud Project for Google Sheets API
- Visit the Google Cloud Console and sign in with your Google account.
- Navigate to Menu > IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Sheets API in Your Project
- In the Google Cloud Console, go to Menu > APIs & Services > Library.
- Search for "Google Sheets API" and click on it.
- Click the Enable button to activate the API for your project.
Configure OAuth Consent Screen for Google Sheets API
- In the Google Cloud Console, navigate to Menu > APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the app registration form and click Save and Continue.
Create OAuth Credentials for Google Sheets API
- Go to Menu > APIs & Services > Credentials in the Google Cloud Console.
- Click Create Credentials > OAuth client ID.
- Select Application type > Desktop app and provide a name for the credential.
- Click Create to generate your Client ID and Client Secret.
- Download the credentials as a JSON file and save it securely.
With these steps completed, your Google Cloud project is now set up to interact with the Google Sheets API using OAuth authentication. You can proceed to write Python code that utilizes these credentials to access and manipulate Google Sheets data.
For more detailed information, refer to the official Google Sheets API documentation: Google Sheets API Concepts, Create a Google Cloud Project, Enable Google Workspace APIs, Configure OAuth Consent, and Create Access Credentials.
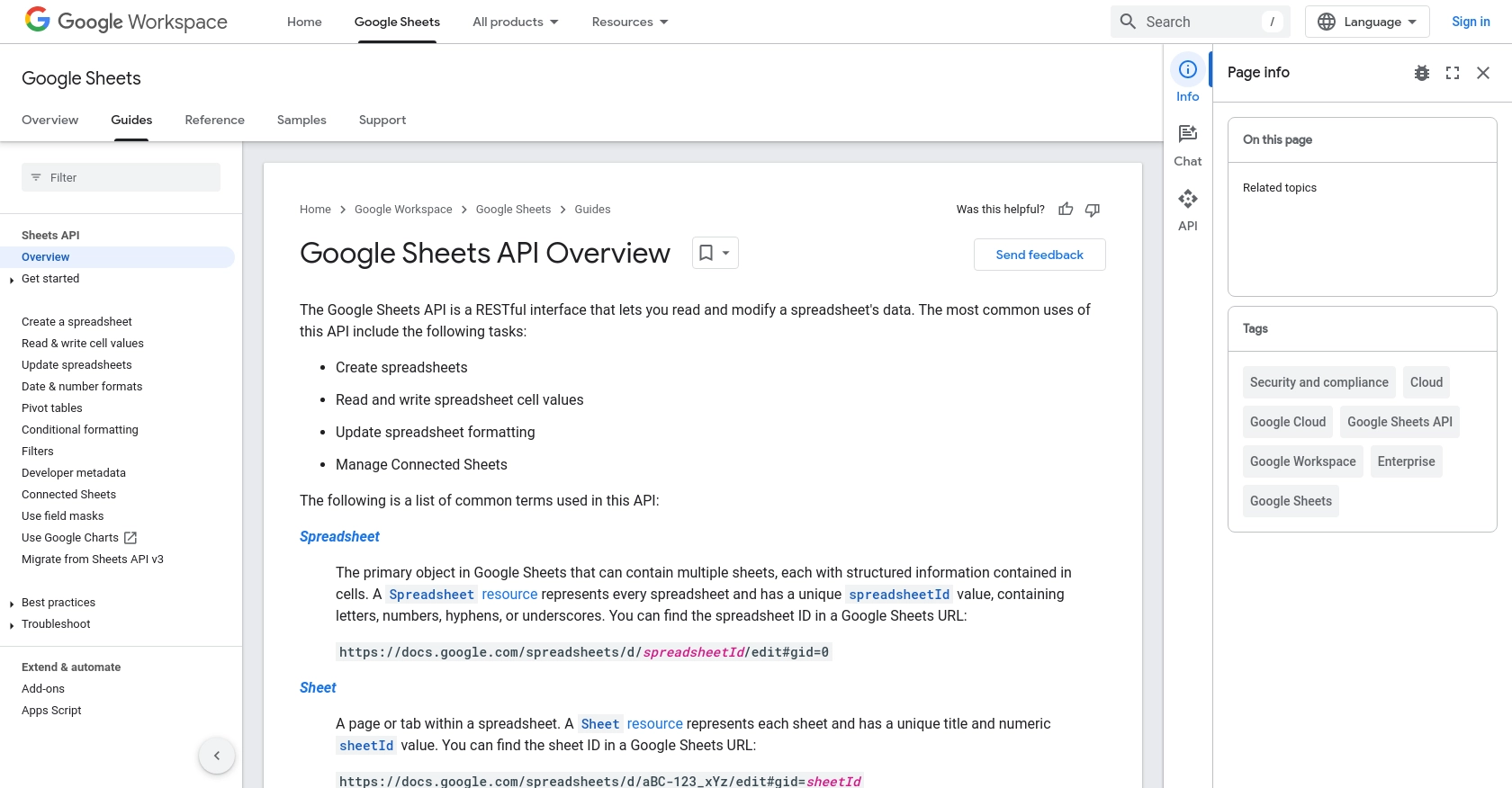
sbb-itb-96038d7
Making API Calls to Retrieve Google Sheets Data Using Python
To interact with the Google Sheets API using Python, you need to set up your environment and write code that can authenticate and make requests to the API. This section will guide you through the process of retrieving records from a Google Sheet using Python.
Setting Up Your Python Environment for Google Sheets API
Before you begin coding, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. You will also need to install the google-auth
and google-auth-oauthlib
libraries to handle authentication, and the google-api-python-client
library to interact with the Google Sheets API.
pip install google-auth google-auth-oauthlib google-api-python-client
Writing Python Code to Fetch Records from Google Sheets
Once your environment is set up, you can write a Python script to authenticate and fetch data from a Google Sheet. Below is an example script:
from google.oauth2 import service_account
from googleapiclient.discovery import build
# Load credentials from the JSON file
creds = service_account.Credentials.from_service_account_file(
'path/to/credentials.json',
scopes=['https://www.googleapis.com/auth/spreadsheets.readonly']
)
# Build the service
service = build('sheets', 'v4', credentials=creds)
# Specify the spreadsheet ID and range
spreadsheet_id = 'your_spreadsheet_id'
range_name = 'Sheet1!A1:D10'
# Make the API call to fetch data
result = service.spreadsheets().values().get(
spreadsheetId=spreadsheet_id,
range=range_name
).execute()
# Get the values from the response
values = result.get('values', [])
# Print the fetched records
if not values:
print('No data found.')
else:
for row in values:
print(row)
Replace 'path/to/credentials.json'
with the path to your downloaded credentials JSON file, and 'your_spreadsheet_id'
with the ID of your Google Sheet. The range_name
specifies the range of cells you want to retrieve.
Verifying API Call Success and Handling Errors
After running the script, you should see the records from the specified range printed in your console. If the request is successful, the data will match the content in your Google Sheet. In case of errors, the API will return error codes that you can handle in your script. For more information on error codes, refer to the Google Sheets API documentation.
By following these steps, you can efficiently retrieve data from Google Sheets using Python, enabling you to automate data workflows and integrate Google Sheets data into your applications.
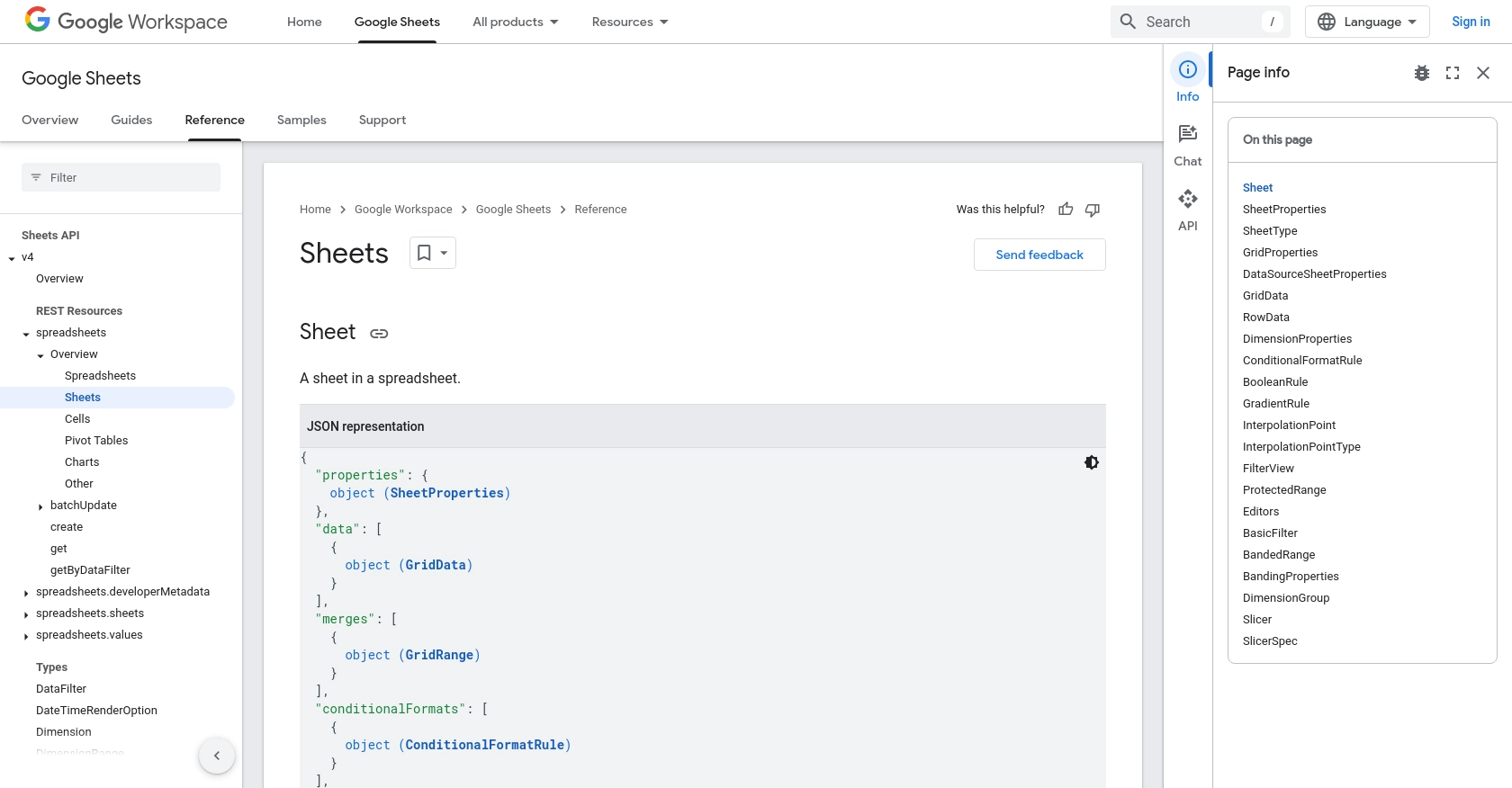
Conclusion and Best Practices for Using Google Sheets API with Python
Integrating with the Google Sheets API using Python provides a powerful way to automate data retrieval and manipulation tasks, enhancing productivity and streamlining workflows. By following the steps outlined in this guide, you can efficiently access and manage Google Sheets data programmatically.
Best Practices for Secure and Efficient Google Sheets API Integration
- Securely Store Credentials: Ensure that your OAuth credentials and API keys are stored securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid exceeding the allowed number of requests. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Optimize Data Requests: When possible, limit the range of data you request to minimize response size and improve performance. Use specific ranges instead of fetching entire sheets.
- Implement Error Handling: Incorporate robust error handling in your scripts to manage API errors effectively. Refer to the Google Sheets API documentation for detailed error code explanations.
Streamline Your Integration Process with Endgrate
While integrating with the Google Sheets API can significantly enhance your application's capabilities, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this process by offering a unified API endpoint that connects to various platforms, including Google Sheets.
With Endgrate, you can focus on your core product while outsourcing the integration work. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can streamline your integration processes and save valuable time and resources.
Read More
- https://endgrate.com/provider/googlesheets
- https://developers.google.com/sheets/api/guides/concepts
- https://developers.google.com/sheets/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/sheets/api/reference/rest/v4/spreadsheets/sheets
- https://developers.google.com/sheets/api/reference/rest/v4/spreadsheets/cells
Ready to get started?