Using the Apollo API to Get Contacts in PHP
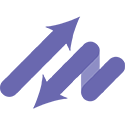
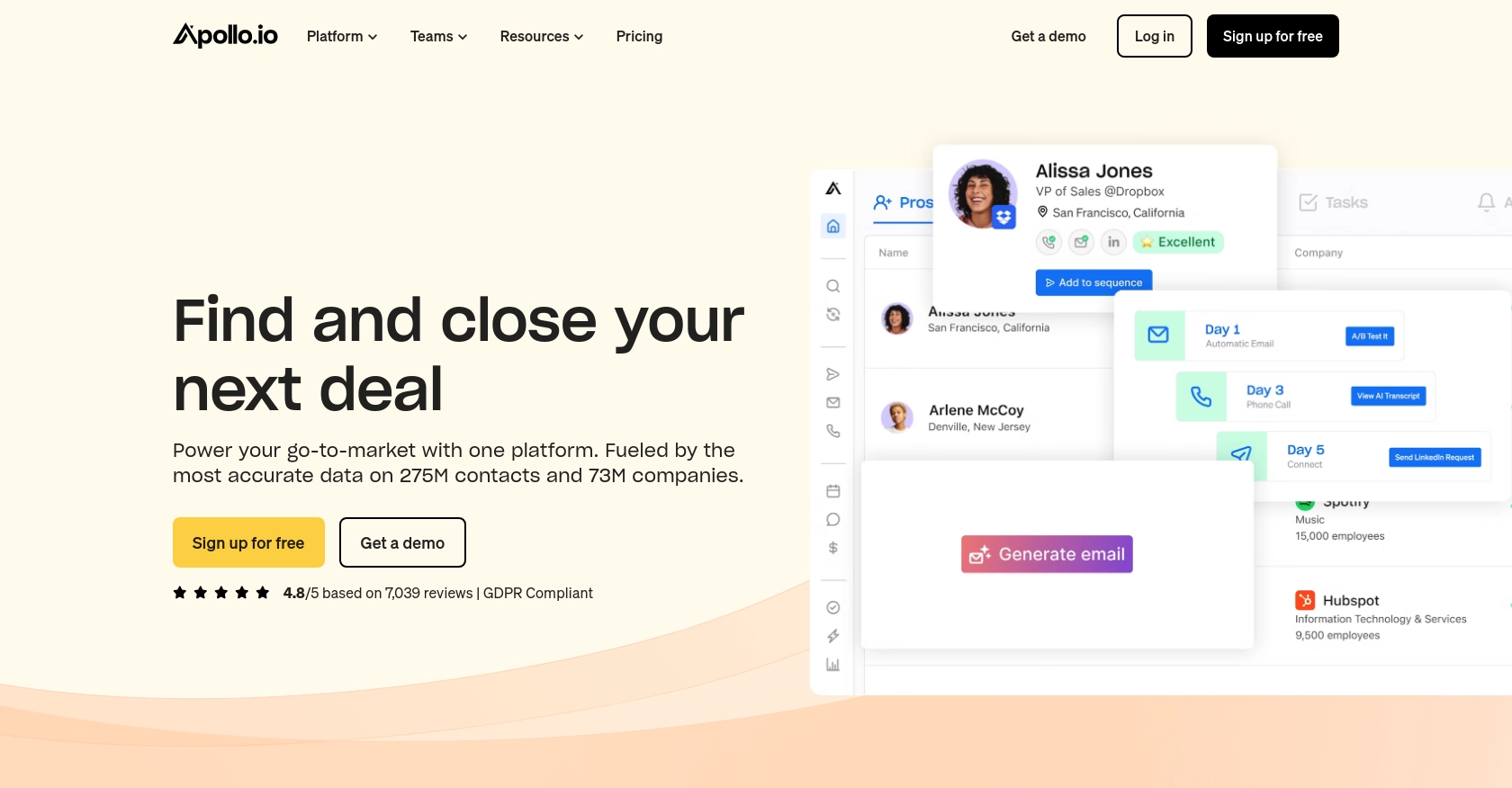
Introduction to Apollo API
Apollo is a comprehensive sales intelligence and engagement platform that empowers businesses to streamline their sales processes. By providing access to a vast database of over 265 million contacts and 70 million companies, Apollo enables sales teams to identify and connect with potential leads more effectively.
Integrating with Apollo's API allows developers to automate and enhance their sales workflows. For example, using the Apollo API, a developer can retrieve contact information to populate a CRM system, enabling sales teams to have up-to-date data at their fingertips. This integration can significantly improve lead management and outreach strategies.
Setting Up Your Apollo API Test Account
Before you can start using the Apollo API to retrieve contacts in PHP, you need to set up a test account. This involves obtaining an API key, which will allow you to authenticate your requests and access Apollo's extensive database.
Creating an Apollo Account
If you don't already have an Apollo account, you can sign up for a free trial on the Apollo website. This will give you access to the platform's features and allow you to explore its capabilities.
- Visit the Apollo website and click on the "Sign Up" button.
- Fill in the required information, such as your name, email, and company details.
- Follow the instructions to complete the registration process.
Generating an Apollo API Key
Once your account is set up, you'll need to generate an API key to authenticate your API requests. Follow these steps to obtain your API key:
- Log in to your Apollo account.
- Navigate to the "API Settings" section in your account dashboard.
- Click on "Generate API Key" and follow the prompts to create a new key.
- Copy the generated API key and store it securely, as you'll need it for making API requests.
Configuring API Key Authentication
With your API key ready, you can now configure your PHP application to authenticate requests to the Apollo API. The API uses API key-based authentication, which requires you to include the key in the request headers.
$apiKey = 'YOUR_API_KEY_HERE';
$headers = [
'Content-Type: application/json',
'Cache-Control: no-cache',
'X-Api-Key: ' . $apiKey
];
Replace YOUR_API_KEY_HERE
with the API key you generated earlier. This setup will allow you to authenticate your requests and interact with Apollo's API.
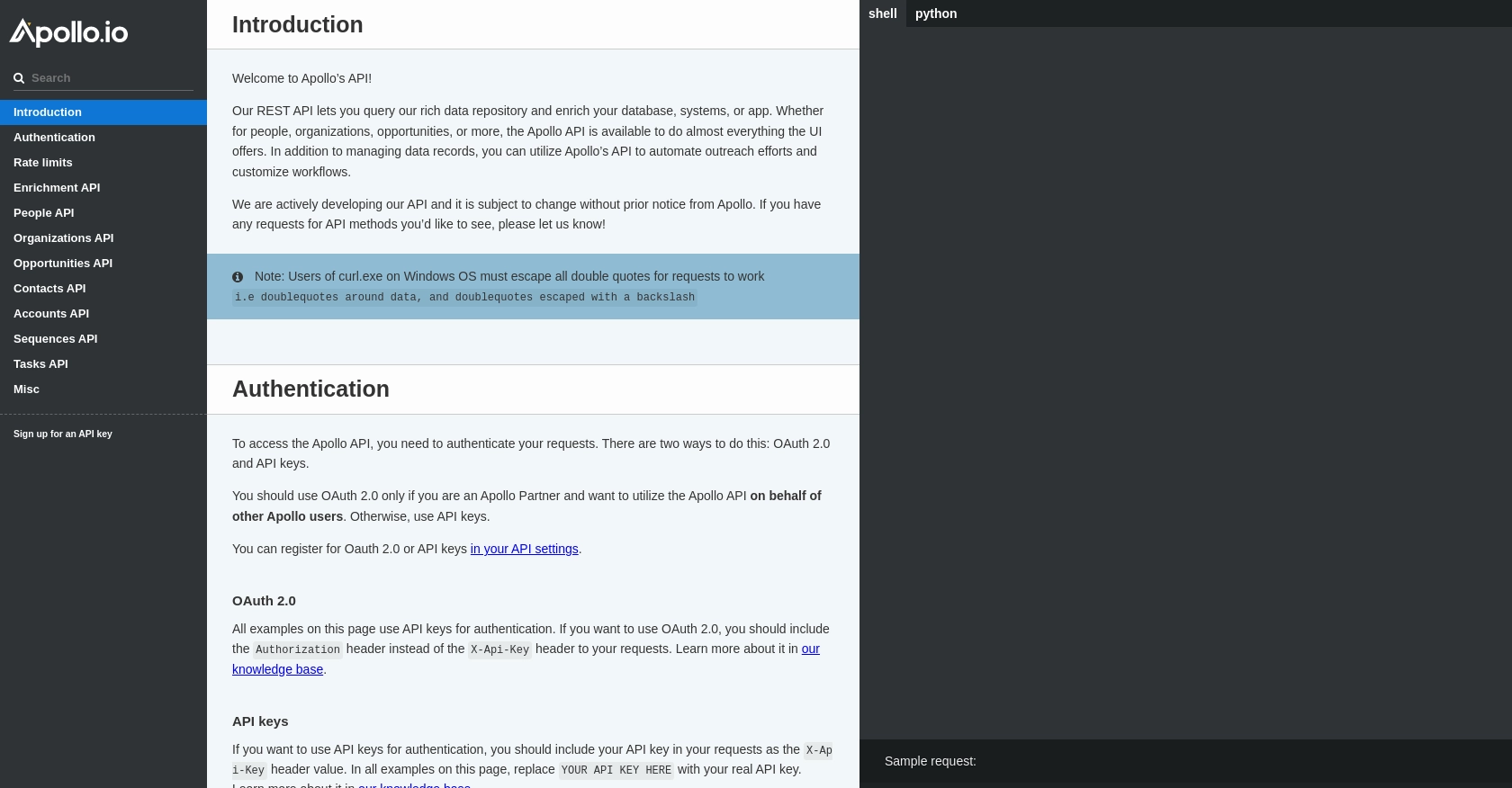
sbb-itb-96038d7
Making API Calls to Retrieve Contacts Using Apollo API in PHP
To interact with the Apollo API and retrieve contact information using PHP, you'll need to set up your environment and write the necessary code to make API requests. This section will guide you through the process, ensuring you have the right PHP version and dependencies installed.
Setting Up Your PHP Environment for Apollo API Integration
Before making API calls, ensure you have PHP installed on your machine. This tutorial uses PHP 7.4 or later. Additionally, you'll need the cURL
extension enabled, which is commonly used for making HTTP requests in PHP.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the cURL extension is enabled by checking your
php.ini
file or runningphp -m | grep curl
.
Writing PHP Code to Retrieve Contacts from Apollo API
With your environment ready, you can now write the PHP code to make a GET request to the Apollo API and retrieve contacts. Below is a sample script to help you get started:
'CEO, Apollo',
'sort_by_field' => 'contact_last_activity_date',
'sort_ascending' => false
];
$options = [
CURLOPT_URL => $url,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_HTTPHEADER => $headers,
CURLOPT_POST => true,
CURLOPT_POSTFIELDS => json_encode($data)
];
$ch = curl_init();
curl_setopt_array($ch, $options);
$response = curl_exec($ch);
curl_close($ch);
$contacts = json_decode($response, true);
print_r($contacts);
?>
Replace YOUR_API_KEY_HERE
with your actual API key. This script sends a POST request to the Apollo API to search for contacts with the specified keywords and sorts them by the last activity date.
Handling API Responses and Errors in Apollo API
After executing the API call, you should verify the response to ensure the request was successful. The Apollo API will return a JSON response containing the contact data or an error message if the request fails.
- Check the HTTP status code to determine if the request was successful (status code 200).
- Handle errors by inspecting the response for any error messages or codes.
For more details on error codes and handling, refer to the Apollo API documentation.
Verifying Successful Contact Retrieval in Apollo API
Once you have retrieved the contact data, you can verify the results by checking the output in your PHP application. The returned data should match the contacts available in your Apollo account.
By following these steps, you can efficiently integrate the Apollo API into your PHP application, allowing you to automate contact retrieval and enhance your sales processes.
Conclusion and Best Practices for Using Apollo API in PHP
Integrating the Apollo API into your PHP applications can significantly enhance your sales processes by automating contact retrieval and ensuring your CRM systems are always up-to-date. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate your requests, and handle API responses effectively.
Best Practices for Secure and Efficient Apollo API Integration
- Secure API Keys: Always store your API keys securely and avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Apollo's rate limits, which are 50 requests per minute, 100 requests per hour, and 300 requests per day. Implement logic to handle rate limit responses gracefully.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your systems.
- Error Handling: Implement robust error handling to manage API errors and exceptions, ensuring your application can recover gracefully.
Enhancing Your Integration with Endgrate
For developers looking to streamline their integration processes further, consider leveraging Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can simplify your integration efforts and enhance your development workflow.
Read More
Ready to get started?