Using the Apollo API to Get Users (with PHP examples)
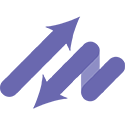
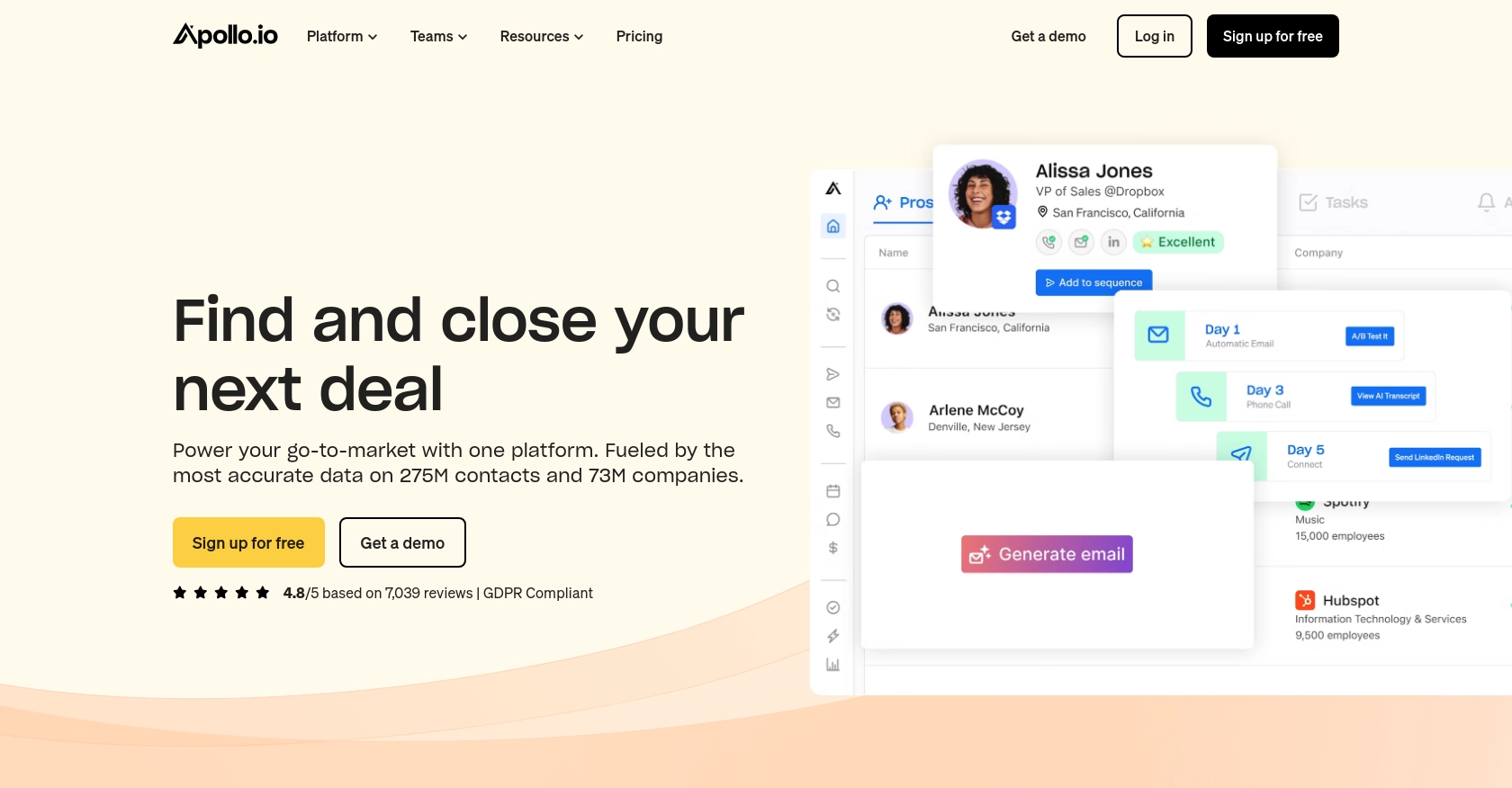
Introduction to Apollo API
Apollo is a robust sales intelligence and engagement platform that empowers businesses to streamline their sales processes. With a vast database of over 265 million contacts and advanced tools for lead generation, Apollo helps sales teams identify and connect with potential customers more effectively.
Integrating with the Apollo API allows developers to access and manage user data seamlessly. For example, you can use the Apollo API to retrieve a list of users, which can be beneficial for syncing user data with your internal systems or enhancing your CRM capabilities.
Setting Up Your Apollo API Test Account
Before you can start using the Apollo API to retrieve user data, you need to set up a test account. This will allow you to safely experiment with API calls without affecting your production data.
Creating an Apollo Account
If you don't already have an Apollo account, you can sign up for a free trial on the Apollo website. This will give you access to the platform's features and allow you to generate an API key for testing purposes.
- Visit the Apollo website and click on the "Sign Up" button.
- Follow the instructions to create your account. You'll need to provide some basic information such as your name, email, and company details.
- Once your account is set up, log in to access the Apollo dashboard.
Generating an Apollo API Key
To authenticate your API requests, you'll need an API key. Follow these steps to generate one:
- Navigate to the "API Settings" section in your Apollo dashboard.
- Click on "Generate API Key" to create a new key.
- Copy the generated API key and store it securely. You'll need it to authenticate your API requests.
Configuring API Key Authentication
With your API key ready, you can now configure your API requests. Apollo uses API key-based authentication, which requires you to include the key in the request headers.
// Sample PHP code to set up headers with API key
$headers = [
'Content-Type: application/json',
'Cache-Control: no-cache',
'X-Api-Key: YOUR_API_KEY_HERE'
];
Replace YOUR_API_KEY_HERE
with the API key you generated earlier. This header setup will be used in all your API requests to Apollo.
For more details on authentication, refer to the Apollo API documentation.
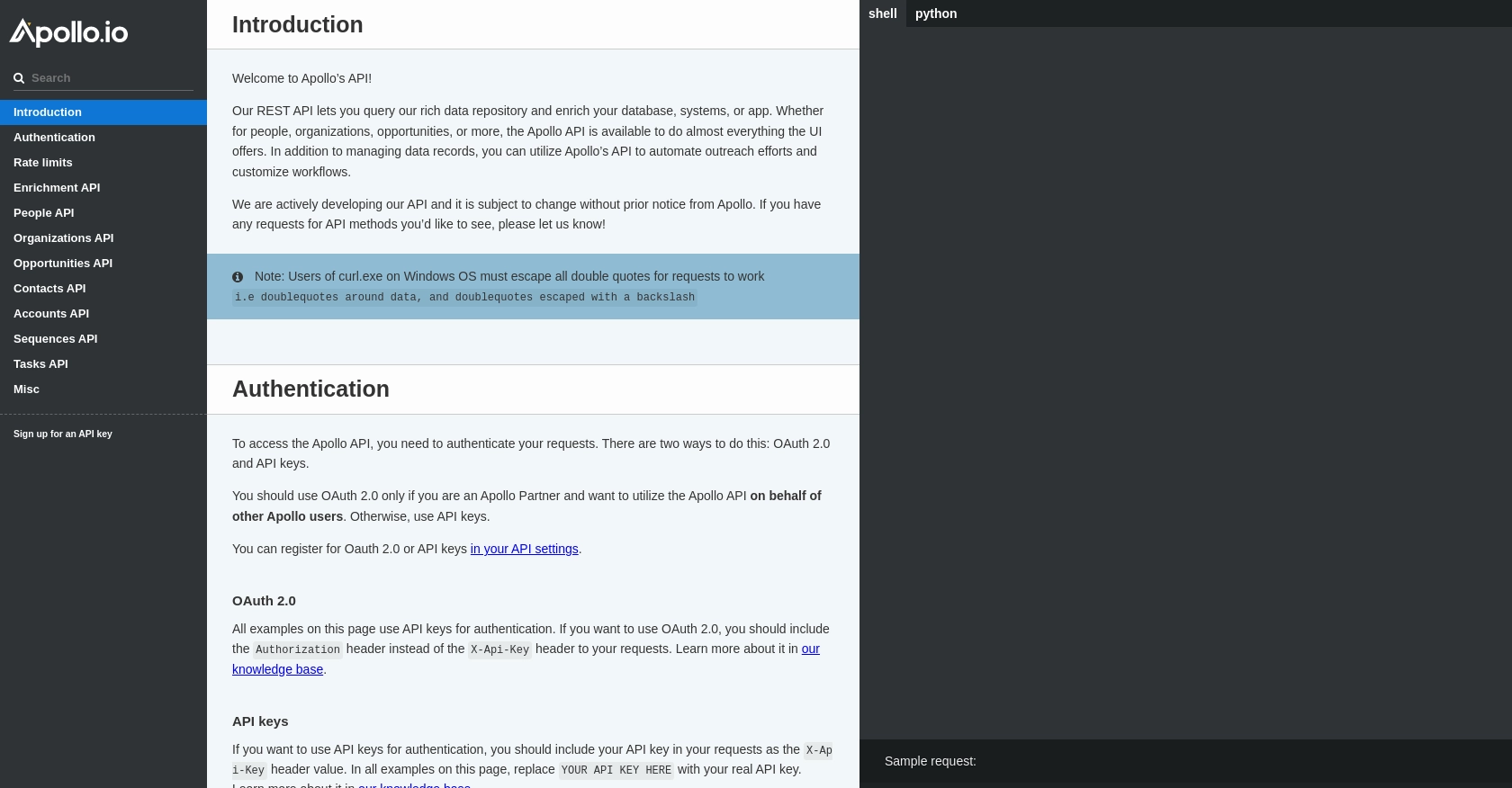
sbb-itb-96038d7
Making API Calls to Retrieve Users with Apollo API in PHP
To interact with the Apollo API and retrieve user data, you'll need to make HTTP requests using PHP. This section will guide you through the process of setting up your environment and executing the necessary API calls.
Setting Up Your PHP Environment for Apollo API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP version 7.4 or higher and the cURL
extension enabled. If you're using a package manager like Composer, you can also include the guzzlehttp/guzzle
library for easier HTTP requests.
composer require guzzlehttp/guzzle
Executing an API Call to Get Users from Apollo
With your environment ready, you can now proceed to make an API call to retrieve users. The following PHP code demonstrates how to perform a GET request to the Apollo API:
use GuzzleHttp\Client;
$client = new Client();
$response = $client->request('GET', 'https://api.apollo.io/v1/users/search', [
'headers' => [
'Content-Type' => 'application/json',
'Cache-Control' => 'no-cache',
'X-Api-Key' => 'YOUR_API_KEY_HERE'
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['users'] as $user) {
echo 'User ID: ' . $user['id'] . ', Name: ' . $user['name'] . '<br>';
}
Replace YOUR_API_KEY_HERE
with your actual API key. This script uses the Guzzle HTTP client to send a GET request to the Apollo API, retrieves the user data, and prints each user's ID and name.
Handling API Response and Errors
After executing the API call, it's crucial to handle the response correctly. Check the status code to ensure the request was successful, and handle any errors that may occur:
try {
$response = $client->request('GET', 'https://api.apollo.io/v1/users/search', [
'headers' => [
'Content-Type' => 'application/json',
'Cache-Control' => 'no-cache',
'X-Api-Key' => 'YOUR_API_KEY_HERE'
]
]);
if ($response->getStatusCode() === 200) {
$data = json_decode($response->getBody(), true);
// Process data
} else {
echo 'Error: ' . $response->getStatusCode();
}
} catch (Exception $e) {
echo 'Exception: ' . $e->getMessage();
}
This code snippet includes error handling using a try-catch block to manage exceptions and check the response status code.
Verifying Successful API Requests in Apollo Dashboard
To verify that your API requests are successful, you can check the Apollo dashboard. If the request retrieves user data, it should reflect in your test account. Additionally, monitor the API response headers for rate limit information to ensure you're within the allowed request limits.
For more information on handling errors and rate limits, refer to the Apollo API documentation.
Conclusion and Best Practices for Using Apollo API with PHP
Integrating with the Apollo API using PHP can significantly enhance your ability to manage and utilize user data within your applications. By following the steps outlined in this guide, you can efficiently retrieve user information and integrate it with your internal systems.
Best Practices for Secure and Efficient API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of the rate limits imposed by Apollo. Monitor the response headers to ensure your application stays within the allowed limits. Apollo's rate limits are detailed in their API documentation.
- Error Handling: Implement robust error handling to manage exceptions and unexpected responses from the API. This ensures your application remains stable and provides meaningful feedback to users.
- Data Standardization: Ensure that the data retrieved from Apollo is standardized and transformed as needed to fit your application's requirements.
Leverage Endgrate for Streamlined Integration
If managing multiple integrations becomes overwhelming, consider using Endgrate. With Endgrate, you can streamline your integration processes, allowing you to focus on your core product development. Endgrate provides a unified API endpoint that simplifies interactions with various platforms, including Apollo.
By using Endgrate, you can save time and resources, build integrations once for each use case, and offer an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can enhance your integration strategy.
Read More
Ready to get started?