Using the AppFolio API to Get Report (with Javascript examples)
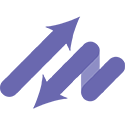
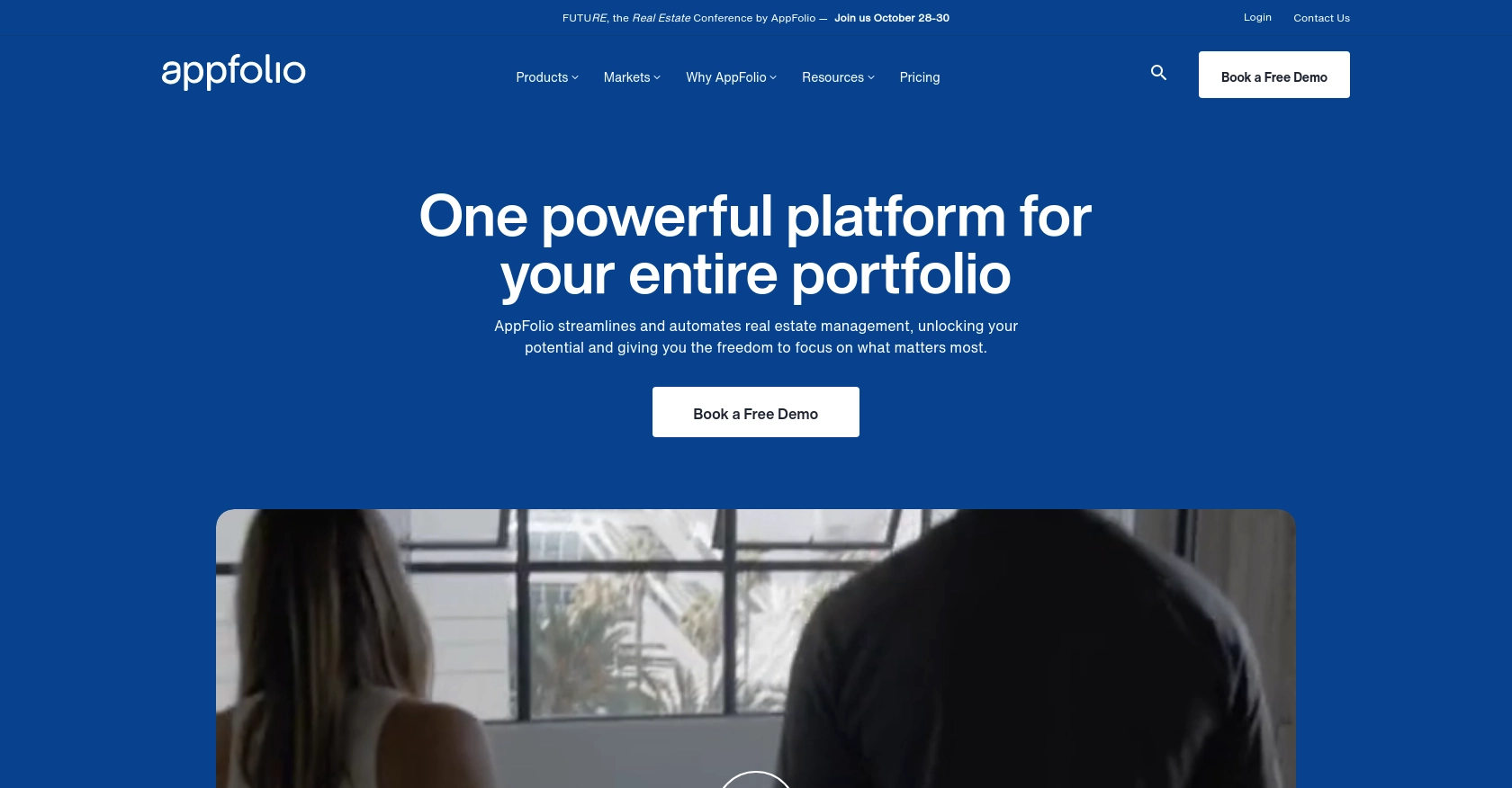
Introduction to AppFolio API Integration
AppFolio is a comprehensive property management software solution that caters to the needs of property managers and real estate professionals. It offers a wide range of features, including marketing, leasing, accounting, and reporting, all designed to streamline operations and enhance efficiency.
Integrating with the AppFolio API allows developers to automate and optimize various property management tasks. For example, a developer might want to retrieve detailed reports from AppFolio to analyze property performance or financial data. By leveraging the AppFolio API, developers can seamlessly access and process this information, enabling more informed decision-making and improved operational workflows.
Setting Up Your AppFolio Test/Sandbox Account
Before diving into the integration process with AppFolio, it's essential to set up a test or sandbox account. This environment allows developers to experiment with API calls without affecting live data, ensuring a smooth and error-free integration experience.
Accessing the AppFolio Property Manager Portal
To begin, log in to your AppFolio Property Manager Portal. This portal serves as the central hub for managing your property data and accessing various features, including reporting.
- Visit the AppFolio Property Manager Portal and log in with your credentials.
Creating and Configuring Reports in AppFolio
Once logged in, you can create and configure reports to be used in your integration. Follow these steps to set up the necessary reports:
- Create the appropriate report within the portal.
- Click on Actions → Email and send the report in CSV format to the designated email address.
- Click on Actions → Save Layout and save the report layout with a preferred name.
Scheduling Reports in AppFolio
To automate the process, schedule the report to be sent regularly:
- Navigate back to the Property Manager portal and go to Reporting → Scheduled Reports.
- Create a new scheduled report and set it to send daily to the same email address in CSV format.
- The body and subject of the email can be customized as needed.
By following these steps, you ensure that the necessary data is consistently available for processing through the AppFolio API, enabling seamless integration and data analysis.
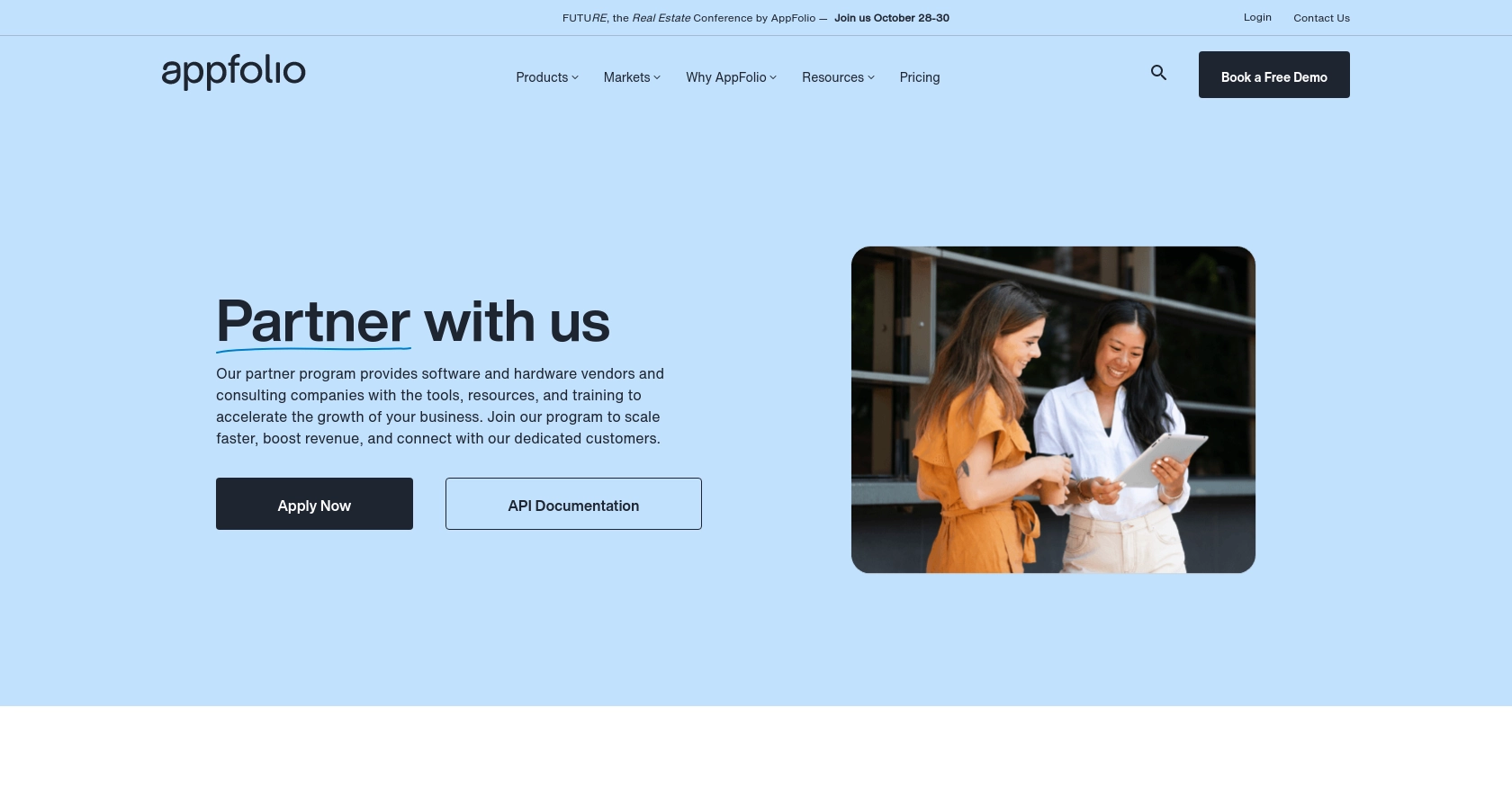
sbb-itb-96038d7
Making API Calls to Retrieve Reports from AppFolio Using JavaScript
To interact with the AppFolio API and retrieve reports, you will need to utilize JavaScript to automate the process of handling CSV reports sent via email. This section will guide you through setting up the necessary environment and writing the code to process these reports.
Setting Up Your JavaScript Environment
Before you begin, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser, making it ideal for server-side scripting and automation tasks.
- Download and install Node.js from the official website.
- Once installed, verify the installation by running
node -v
andnpm -v
in your terminal or command prompt.
Installing Required Node.js Packages
To handle email processing and CSV parsing, you'll need to install a few Node.js packages. Use the following command to install the necessary dependencies:
npm install nodemailer csv-parser
Nodemailer will help you send and receive emails, while csv-parser will allow you to parse CSV files.
Writing JavaScript Code to Process AppFolio Reports
Now that your environment is set up, you can write the JavaScript code to process the reports. Create a new file named processReports.js
and add the following code:
const nodemailer = require('nodemailer');
const csvParser = require('csv-parser');
const fs = require('fs');
// Configure your email transporter
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: 'your-email@gmail.com',
pass: 'your-email-password'
}
});
// Function to process CSV report
function processCSVReport(filePath) {
const results = [];
fs.createReadStream(filePath)
.pipe(csvParser())
.on('data', (data) => results.push(data))
.on('end', () => {
console.log('CSV Report Processed:', results);
// Further processing logic here
});
}
// Example function to fetch and process email
function fetchAndProcessEmail() {
// Logic to fetch email and extract CSV attachment
const csvFilePath = 'path/to/your/report.csv';
processCSVReport(csvFilePath);
}
// Call the function to start processing
fetchAndProcessEmail();
In this code, you configure nodemailer
to connect to your email service. The processCSVReport
function reads and parses the CSV file, allowing you to handle the data as needed.
Running Your JavaScript Script
To execute your script and process the reports, run the following command in your terminal:
node processReports.js
This command will initiate the script, fetch the email, and process the CSV report, displaying the parsed data in the console.
Handling Errors and Verifying Success
Ensure to implement error handling in your script to manage any issues that may arise during email fetching or CSV parsing. You can add error listeners to the streams and log any errors for troubleshooting.
Verify the success of your API calls and data processing by checking the output in your console and ensuring the data matches the expected results from your AppFolio reports.
Conclusion: Best Practices for AppFolio API Integration
Integrating with the AppFolio API to retrieve reports using JavaScript can significantly enhance your property management operations by automating data retrieval and analysis. To ensure a successful integration, consider the following best practices:
Securely Storing Credentials
- Always store sensitive information such as email credentials securely. Use environment variables or secure vaults to manage these details.
Handling Rate Limiting and API Limits
- While the AppFolio API documentation does not specify rate limits, it's crucial to implement logic to handle potential rate limiting gracefully. Consider using retry mechanisms with exponential backoff to manage API call limits effectively.
Transforming and Standardizing Data
- Ensure that the data retrieved from AppFolio is transformed and standardized to fit your application's requirements. This might involve converting data formats or normalizing field names for consistency.
Leverage Endgrate for Seamless Integrations
For developers looking to streamline their integration processes, consider using Endgrate. By outsourcing integrations, you can focus on your core product while benefiting from a unified API endpoint that connects to multiple platforms, including AppFolio. This approach not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website and discover how you can build once for each use case instead of multiple times for different integrations.
Read More
Ready to get started?