Using the Close API to Get Users (with Javascript examples)
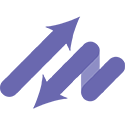
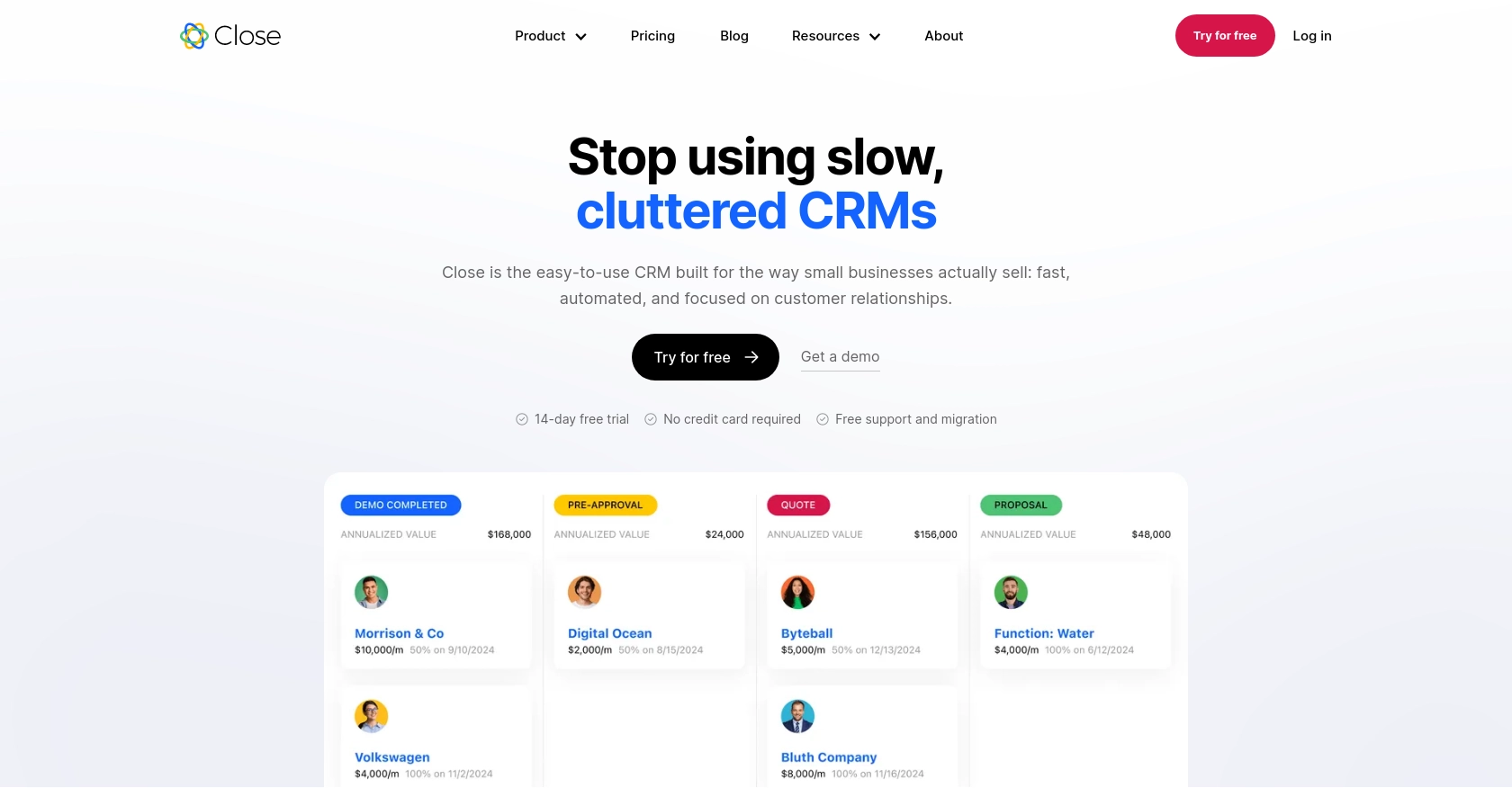
Introduction to Close CRM API
Close is a powerful CRM platform designed to help sales teams manage their leads, contacts, and sales pipelines more effectively. With its robust set of features, Close enables businesses to streamline their sales processes, improve communication, and ultimately close more deals.
Developers might want to integrate with the Close API to automate and enhance their sales workflows. For example, by using the Close API, a developer can retrieve user information to analyze team performance or synchronize user data with other business applications.
This article will guide you through using JavaScript to interact with the Close API, specifically focusing on retrieving user data. By the end of this tutorial, you'll be able to efficiently access user information from Close, enabling you to build more dynamic and integrated sales solutions.
Setting Up Your Close CRM Test Account
Before you can start interacting with the Close API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Close offers a straightforward way to create an account and generate the necessary API key for authentication.
Creating a Close CRM Account
If you don't already have a Close account, you can sign up for a free trial on the Close website. This will give you access to all the features you need to test your API integrations.
- Visit the Close website and click on "Start Free Trial."
- Fill out the required information to create your account.
- Once your account is set up, log in to access the Close dashboard.
Generating an API Key for Close CRM
Close uses API keys for authentication, which are ideal for internal scripts and integrations. Follow these steps to generate your API key:
- Navigate to the "Settings" page in your Close dashboard.
- Under the "API Keys" section, click on "Generate API Key."
- Copy the generated API key and store it securely, as you will need it to authenticate your API requests.
For more detailed information on authentication, refer to the Close API Authentication Documentation.
Testing Your API Key
To ensure your API key is working correctly, you can perform a simple test call to the Close API:
// Example using Fetch API in JavaScript
fetch('https://api.close.com/api/v1/me/', {
method: 'GET',
headers: {
'Authorization': 'Basic ' + btoa('yourapikey:'),
'Content-Type': 'application/json'
}
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Replace yourapikey
with the API key you generated. This call will fetch information about your user account, confirming that your API key is valid and correctly configured.
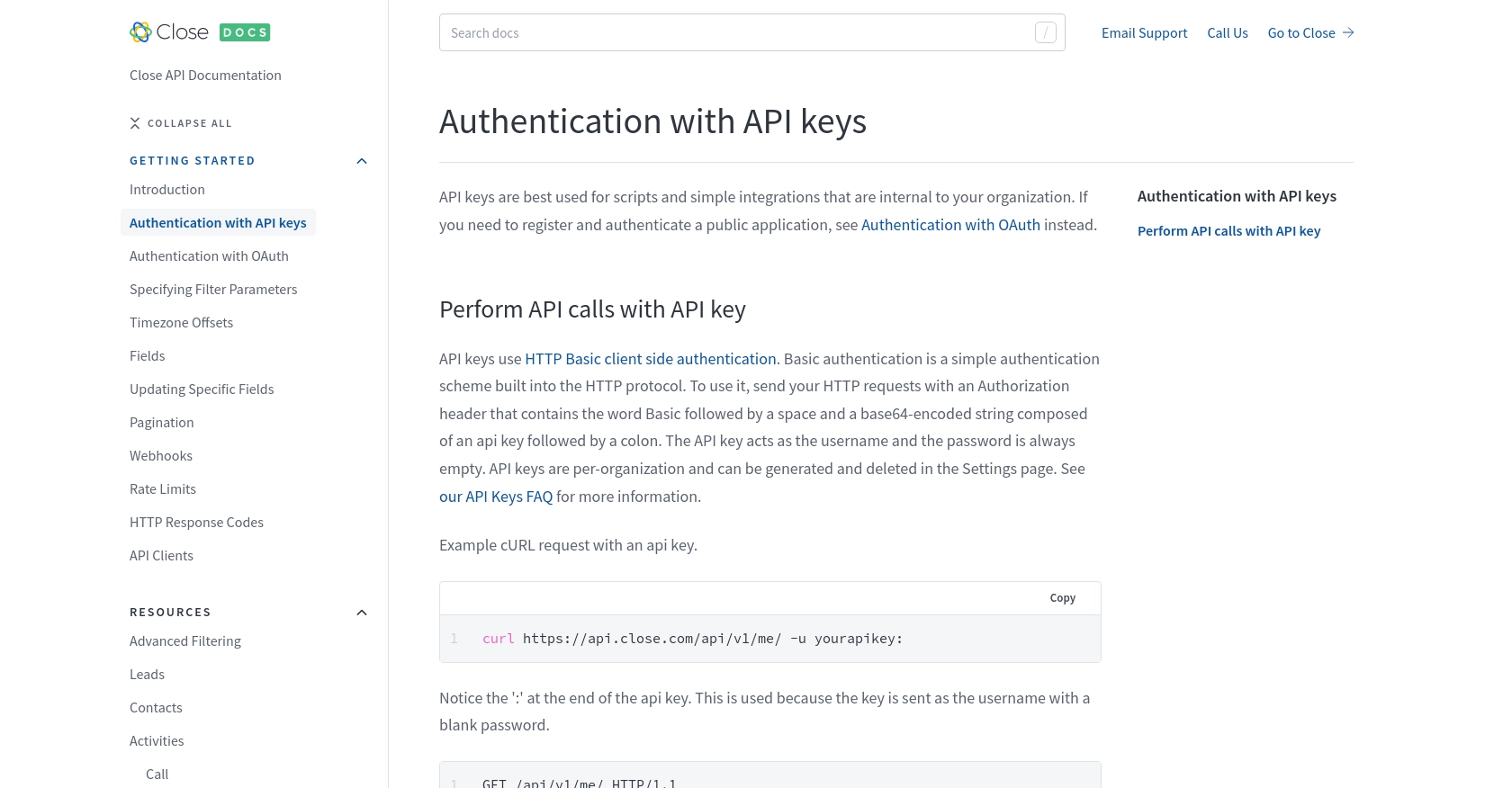
sbb-itb-96038d7
Making API Calls to Retrieve Users from Close CRM Using JavaScript
Now that you have your API key set up, it's time to make API calls to retrieve user data from Close CRM. This section will guide you through the process of setting up your JavaScript environment and executing the necessary API requests.
Setting Up Your JavaScript Environment for Close API
To interact with the Close API using JavaScript, ensure you have a modern JavaScript environment. You can use Node.js or a browser-based environment with support for the Fetch API. Ensure you have the following:
- A code editor like Visual Studio Code.
- Node.js installed if you plan to run scripts outside the browser.
- Basic knowledge of JavaScript and asynchronous programming.
Installing Necessary JavaScript Dependencies
If you're using Node.js, you might want to install additional packages to handle HTTP requests, such as Axios. However, for simplicity, we'll use the Fetch API, which is built into modern browsers and Node.js environments.
JavaScript Code Example to Fetch Users from Close CRM
Below is a JavaScript example using the Fetch API to retrieve a list of users from Close CRM:
// Fetch users from Close CRM
fetch('https://api.close.com/api/v1/user/', {
method: 'GET',
headers: {
'Authorization': 'Basic ' + btoa('yourapikey:'),
'Content-Type': 'application/json'
}
})
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok: ' + response.statusText);
}
return response.json();
})
.then(data => {
console.log('Users:', data);
})
.catch(error => console.error('Error fetching users:', error));
Replace yourapikey
with your actual API key. This code sends a GET request to the Close API to retrieve user data. If successful, it logs the user information to the console.
Handling API Response and Errors for Close API
When making API calls, it's crucial to handle potential errors and verify the response. The Close API uses standard HTTP response codes to indicate success or failure:
- 200: Request was successful.
- 400: Bad request, check your parameters.
- 401: Unauthorized, check your API key.
- 429: Too many requests, respect rate limits.
For more details on HTTP response codes, refer to the Close API HTTP Response Codes Documentation.
Verifying API Call Success in Close CRM
To verify that your API call was successful, check the console output for user data. Additionally, you can log into your Close CRM account and navigate to the user management section to confirm the data matches the API response.
Handling Rate Limits in Close API
The Close API enforces rate limits to ensure fair usage. If you receive a 429 status code, pause your requests as specified by the rate_reset
value in the response headers. For more information, see the Close API Rate Limits Documentation.
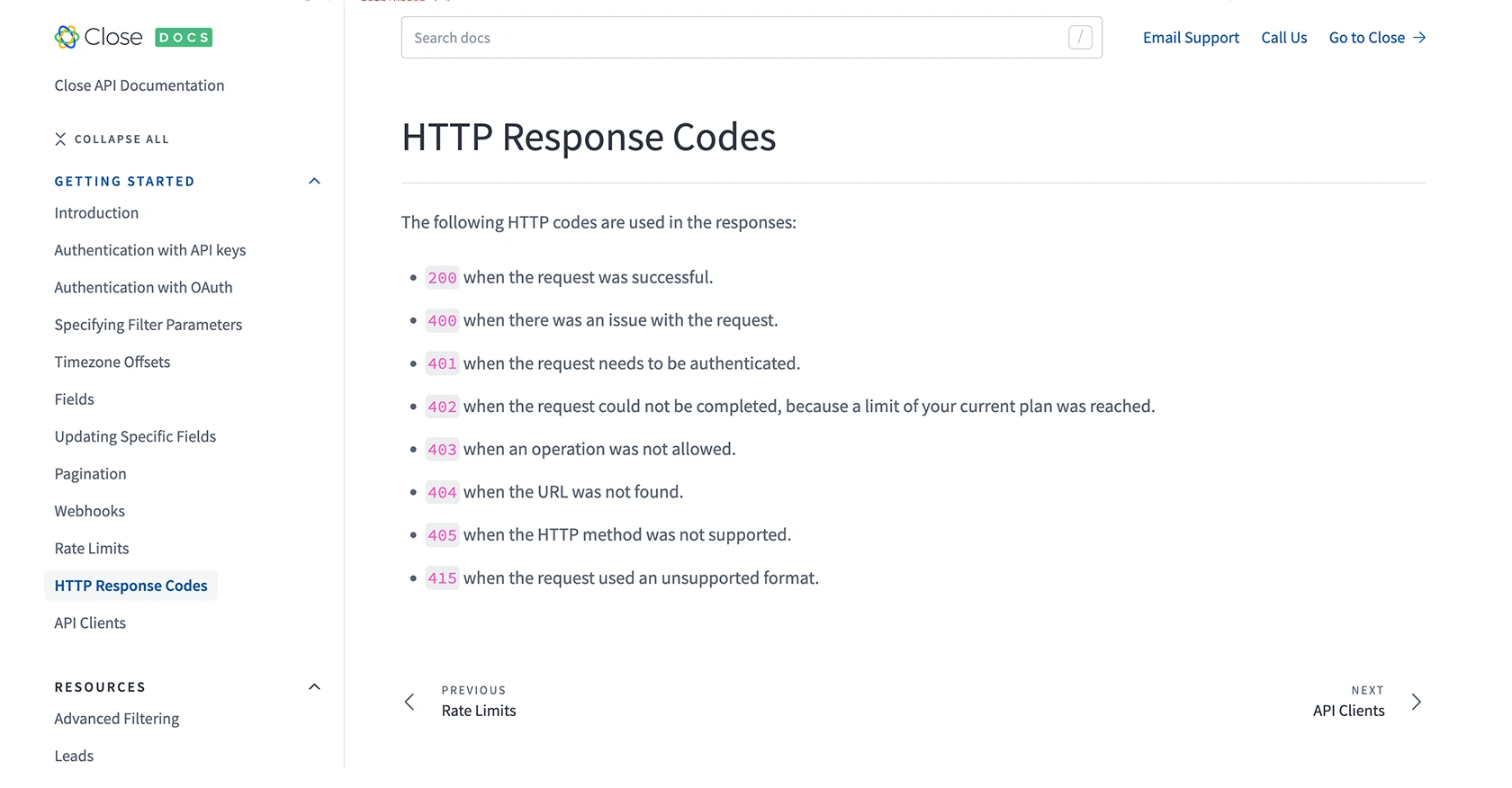
Conclusion and Best Practices for Using Close API with JavaScript
Integrating with the Close API using JavaScript can significantly enhance your sales processes by automating user data retrieval and synchronization. By following the steps outlined in this guide, you can efficiently access user information, enabling more dynamic and integrated sales solutions.
Best Practices for Secure and Efficient Close API Integration
- Secure API Key Storage: Always store your API keys securely. Avoid hardcoding them in your scripts. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits Gracefully: Respect the Close API rate limits to ensure smooth operation. Implement logic to pause requests when a 429 status code is received, using the
rate_reset
value to determine the wait time. - Standardize Data Fields: When integrating with multiple systems, ensure that data fields are standardized for consistency and ease of use across platforms.
Enhancing Your Integration Strategy with Endgrate
While integrating with the Close API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Close.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
- https://endgrate.com/provider/close
- https://developer.close.com/topics/authentication/
- https://developer.close.com/topics/authentication-oauth2/
- https://developer.close.com/topics/pagination/
- https://developer.close.com/topics/rate-limits/
- https://developer.close.com/topics/http-response-codes/
- https://developer.close.com/resources/users/
Ready to get started?