Using the Close API to Get Users in Python
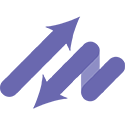
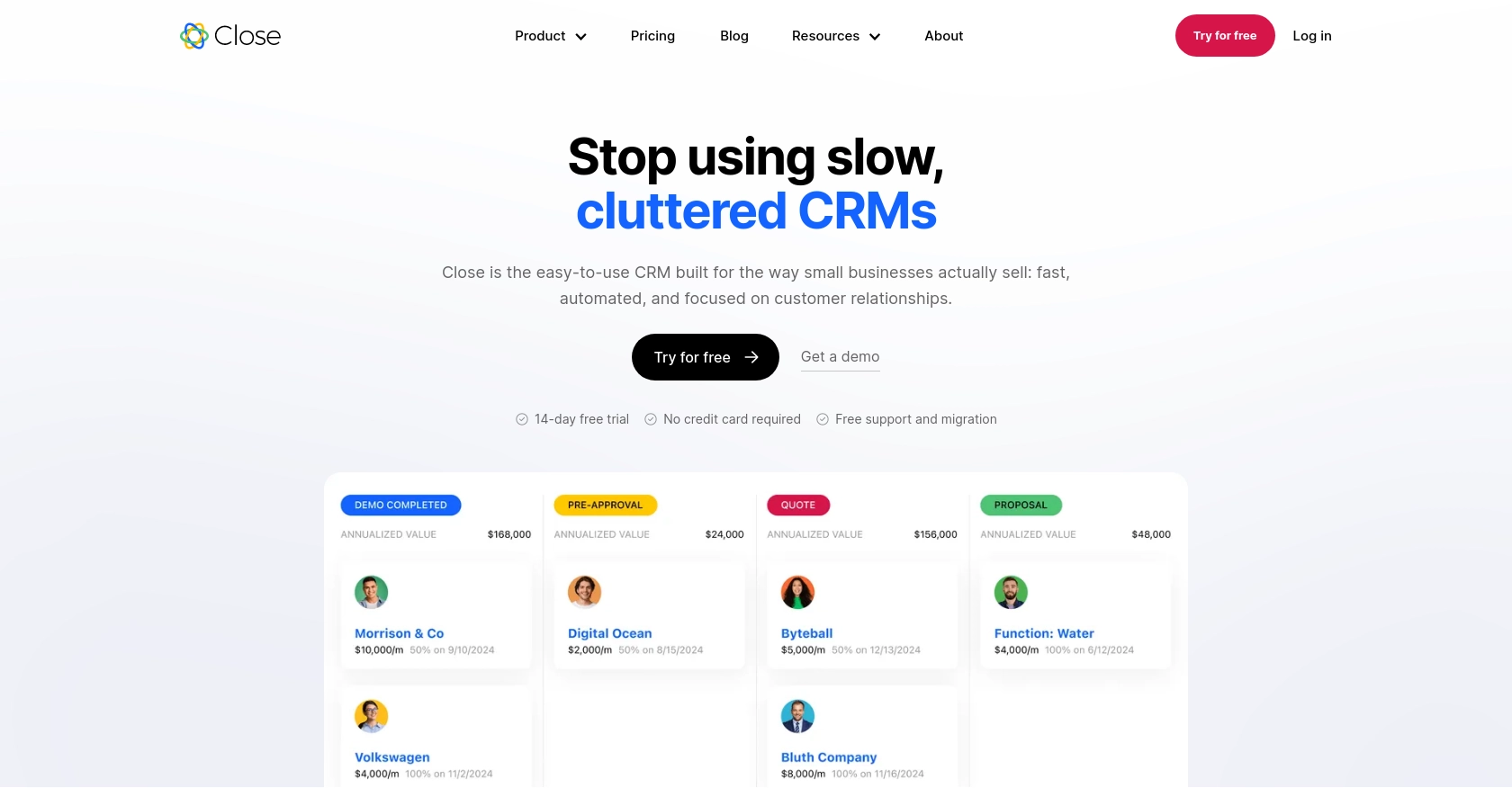
Introduction to Close CRM API
Close is a powerful CRM platform designed to enhance sales productivity and streamline communication for businesses. It offers a comprehensive suite of tools that help sales teams manage leads, track customer interactions, and close deals more efficiently.
Integrating with the Close API allows developers to access and manage user data, enabling seamless automation of sales processes. For example, a developer might use the Close API to retrieve user information and integrate it with other systems, enhancing team collaboration and data consistency across platforms.
This article will guide you through using Python to interact with the Close API, specifically focusing on retrieving user data. By following this tutorial, you'll learn how to efficiently access user information within your organization using Python.
Setting Up Your Close CRM Test Account
Before you can start interacting with the Close API, you'll need to set up a test account. This involves creating an account on Close and obtaining an API key for authentication purposes. The API key will allow you to make authorized requests to the Close API.
Creating a Close CRM Account
If you don't already have a Close account, you can sign up for a free trial on the Close website. This will give you access to the platform and allow you to explore its features.
- Visit the Close website and click on the "Sign Up" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the Close dashboard.
Generating an API Key for Close CRM
To interact with the Close API, you'll need to generate an API key. This key will be used to authenticate your requests.
- Log in to your Close account and navigate to the "Settings" page.
- Under the "API Keys" section, click on "Generate API Key."
- Copy the generated API key and store it securely, as you'll need it for making API calls.
For more information on API key authentication, refer to the Close API documentation.
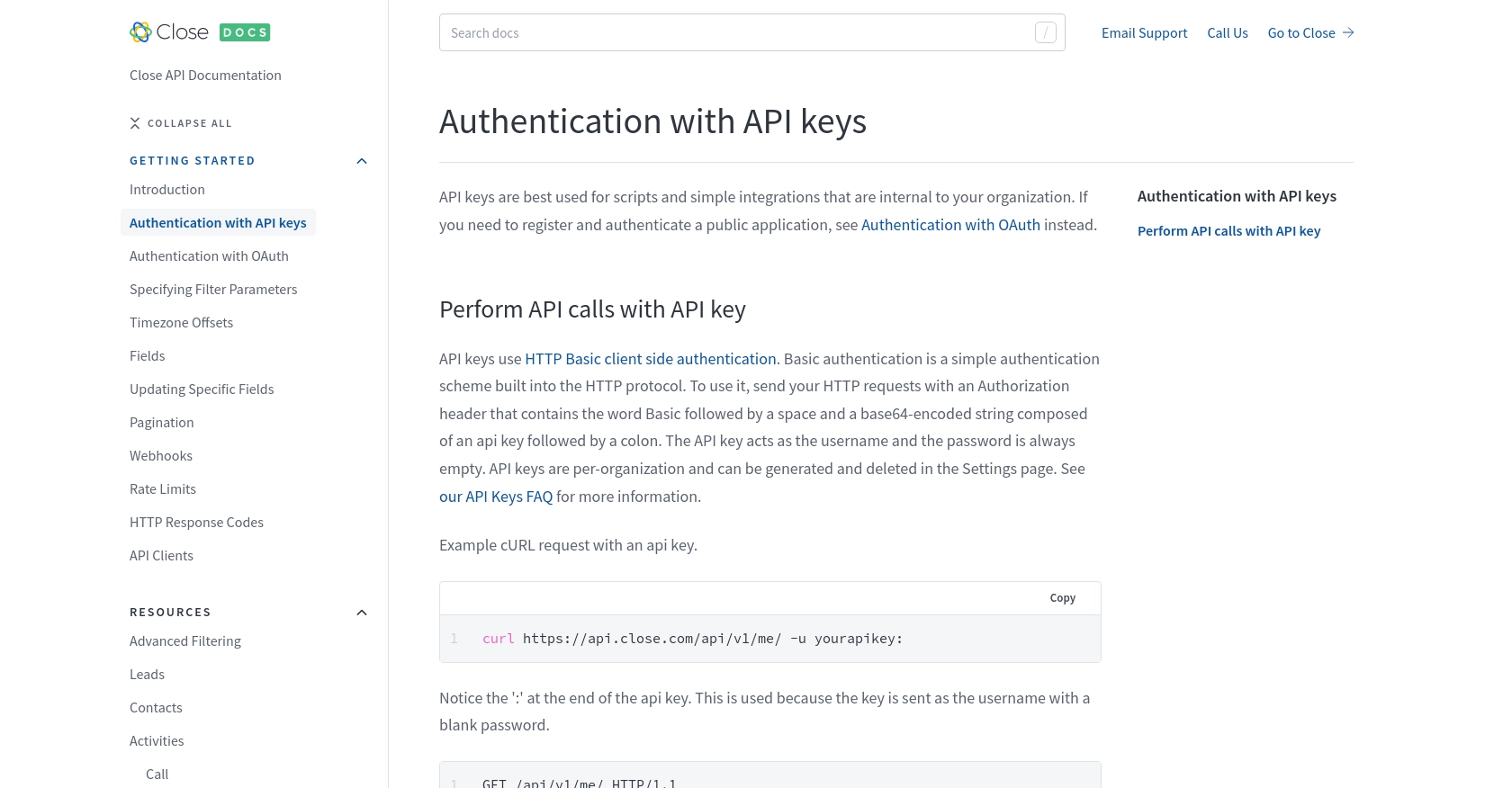
sbb-itb-96038d7
Making API Calls to Retrieve Users with Close API in Python
To interact with the Close API and retrieve user data, you'll need to use Python. This section will guide you through setting up your Python environment, making the necessary API call, and handling the response.
Setting Up Python Environment for Close API
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 if you haven't already.
- Use the following command to install the
requests
library:
pip install requests
Writing Python Code to Fetch Users from Close API
Now that your environment is set up, you can write a Python script to fetch user data from the Close API. Follow these steps:
import requests
import base64
# Set the API endpoint
url = "https://api.close.com/api/v1/user/"
# Your API key
api_key = "your_api_key_here"
# Encode the API key for Basic Authentication
encoded_api_key = base64.b64encode(f"{api_key}:".encode()).decode()
# Set the request headers
headers = {
"Authorization": f"Basic {encoded_api_key}"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
users = response.json()
for user in users['data']:
print(f"User ID: {user['id']}, Name: {user['display_name']}")
else:
print(f"Failed to retrieve users: {response.status_code} - {response.text}")
Replace your_api_key_here
with the API key you generated earlier.
Understanding the API Response and Handling Errors
When you run the script, it sends a GET request to the Close API to retrieve user data. If successful, it prints the user IDs and names. If the request fails, it prints an error message.
Common HTTP response codes you might encounter include:
- 200: Request was successful.
- 401: Authentication failed. Check your API key.
- 429: Too many requests. You need to wait before retrying.
For more details on HTTP response codes, refer to the Close API documentation.
Verifying the API Call in Close CRM
After running the script, you can verify the retrieved user data by checking your Close CRM dashboard. Ensure the users listed in the output match those in your organization.
By following these steps, you can efficiently retrieve user data from Close using Python, enabling seamless integration and automation of your sales processes.
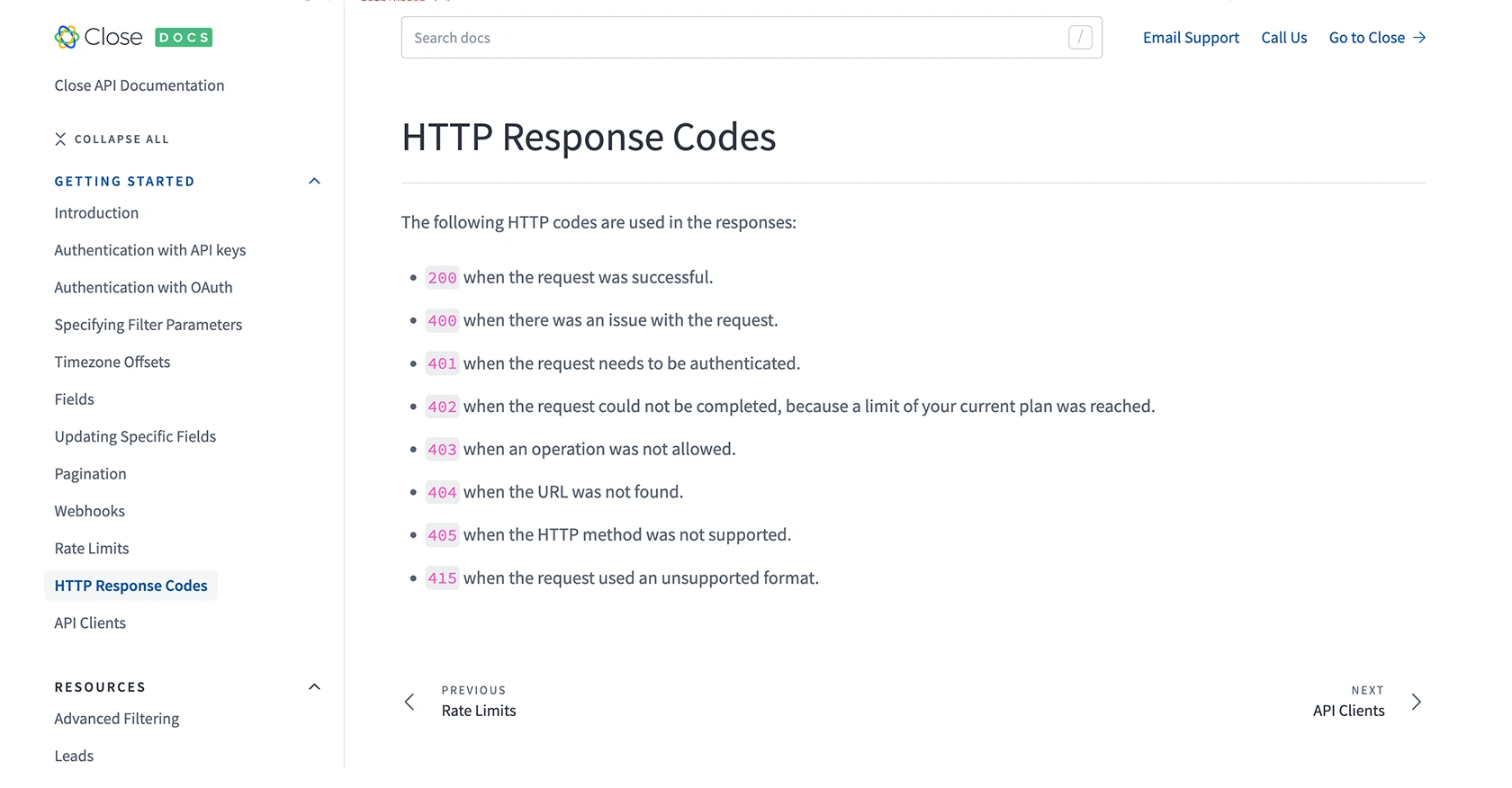
Best Practices for Using Close API in Python
When working with the Close API, it's essential to follow best practices to ensure efficient and secure integration. Here are some recommendations:
- Securely Store API Keys: Always store your API keys securely. Avoid hardcoding them in your scripts. Consider using environment variables or a secure vault.
- Handle Rate Limits Gracefully: Close API enforces rate limits. If you receive a 429 status code, pause your requests for the duration specified in the
rate_reset
value. For more details, refer to the Close API rate limits documentation. - Implement Error Handling: Always check the response status codes and handle errors appropriately. This will help you identify issues quickly and maintain a robust integration.
- Optimize Data Handling: Consider transforming and standardizing data fields to match your application's requirements, ensuring consistency across platforms.
Enhance Your Integration Experience with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Close. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
- https://endgrate.com/provider/close
- https://developer.close.com/topics/authentication/
- https://developer.close.com/topics/authentication-oauth2/
- https://developer.close.com/topics/pagination/
- https://developer.close.com/topics/rate-limits/
- https://developer.close.com/topics/http-response-codes/
- https://developer.close.com/resources/users/
Ready to get started?