Using the Cloze API to Create Or Update Contacts (with Javascript examples)
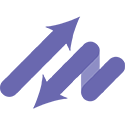
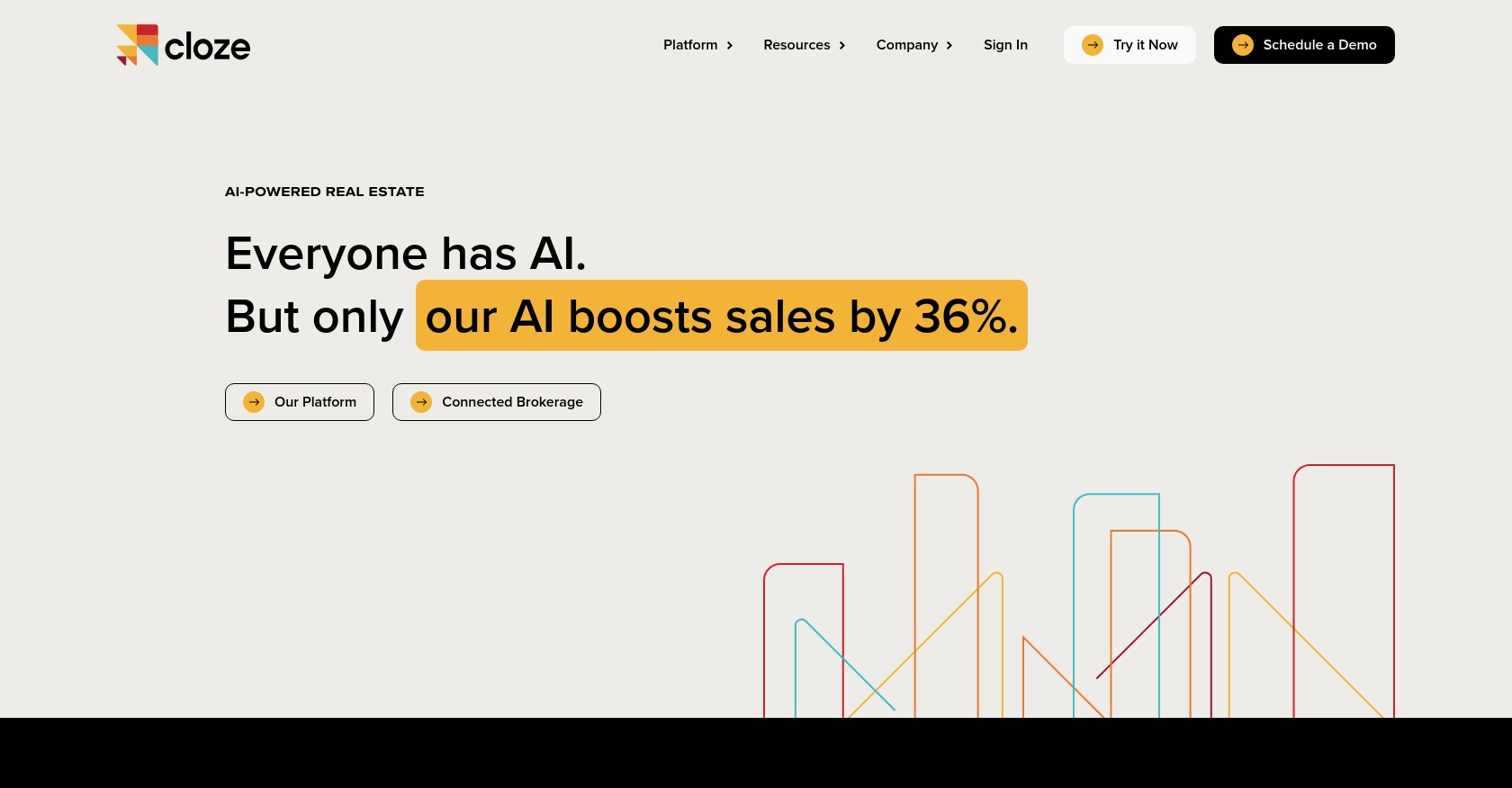
Introduction to Cloze API for Contact Management
Cloze is a powerful relationship management platform that helps businesses streamline their interactions with clients, partners, and team members. It offers a comprehensive suite of tools for managing contacts, projects, and communications, making it an essential tool for businesses looking to enhance their customer relationship management (CRM) efforts.
Developers may want to integrate with the Cloze API to automate and enhance contact management processes. For example, using the Cloze API, you can create or update contact information directly from your application, ensuring that your CRM data is always up-to-date and accurate.
This article will guide you through using JavaScript to interact with the Cloze API, specifically focusing on creating or updating contacts. By following this tutorial, you'll learn how to efficiently manage contact data within the Cloze platform, leveraging its robust API capabilities.
Setting Up Your Cloze API Test or Sandbox Account
Before you can start integrating with the Cloze API, it's essential to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data. Follow these steps to get started:
Create a Cloze Account
If you don't already have a Cloze account, you can sign up for a free trial on the Cloze website. This will give you access to the platform's features and allow you to test API interactions.
Generate a Cloze API Key
To authenticate your API requests, you'll need an API key. Follow these steps to generate one:
- Log in to your Cloze account.
- Navigate to the settings page by clicking on your profile icon in the top right corner.
- Select "API Keys" from the menu.
- Click on "Create New API Key" and provide a name for your key.
- Copy the generated API key and store it securely, as you'll need it for authentication.
Set Up OAuth for Public Integrations
If you're planning to build a public integration with Cloze, you'll need to use OAuth for authentication. Here's how to get started:
- Contact Cloze support at support@cloze.com to request OAuth access.
- Once approved, follow the instructions provided by Cloze to set up your OAuth credentials.
- Ensure you have your client ID and client secret ready for use in your application.
For more detailed instructions, refer to the Cloze API documentation.
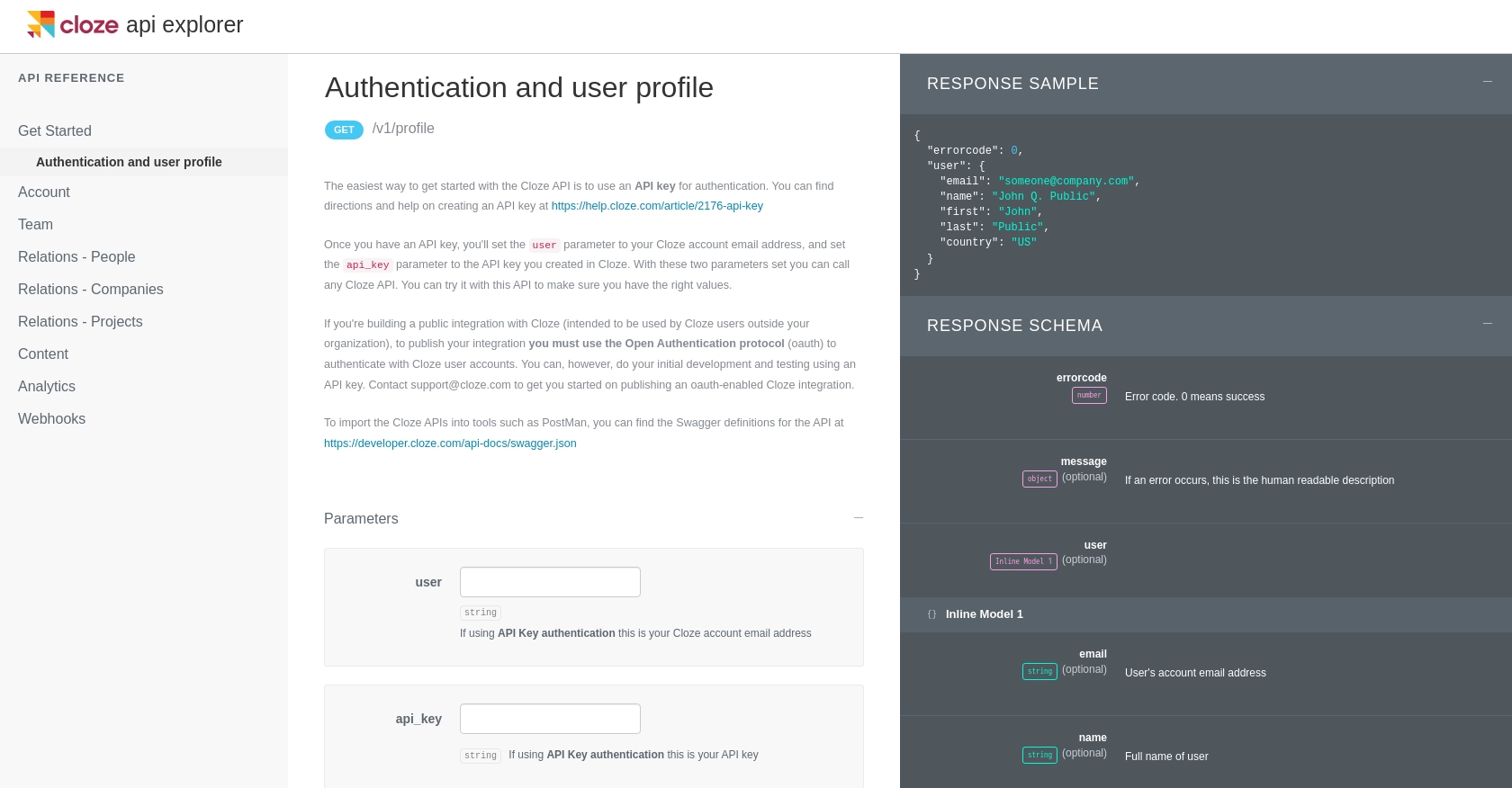
sbb-itb-96038d7
Making API Calls to Cloze for Contact Management Using JavaScript
To interact with the Cloze API for creating or updating contacts, you'll need to make HTTP requests using JavaScript. This section will guide you through the process, including setting up your environment and writing the necessary code.
Setting Up Your JavaScript Environment for Cloze API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- The
axios
library for making HTTP requests. Install it using the command:
npm install axios
Creating a New Contact in Cloze Using JavaScript
To create a new contact in Cloze, you'll need to send a POST request to the Cloze API endpoint. Here's a sample code snippet to help you get started:
const axios = require('axios');
const createContact = async () => {
const apiKey = 'Your_API_Key';
const userEmail = 'your_email@domain.com';
const endpoint = 'https://api.cloze.com/v1/people/create';
const contactData = {
name: 'John Doe',
emails: [{ value: 'johndoe@example.com', work: true }],
segment: 'customer',
stage: 'lead'
};
try {
const response = await axios.post(endpoint, contactData, {
params: {
user: userEmail,
api_key: apiKey
},
headers: {
'Content-Type': 'application/json'
}
});
console.log('Contact Created:', response.data);
} catch (error) {
console.error('Error creating contact:', error.response.data);
}
};
createContact();
Replace Your_API_Key
and your_email@domain.com
with your actual Cloze API key and email address. This code sends a POST request to create a new contact with the specified details.
Updating an Existing Contact in Cloze Using JavaScript
To update an existing contact, use the following code snippet to send a POST request to the appropriate API endpoint:
const updateContact = async () => {
const apiKey = 'Your_API_Key';
const userEmail = 'your_email@domain.com';
const endpoint = 'https://api.cloze.com/v1/people/update';
const contactData = {
name: 'John Doe',
emails: [{ value: 'johndoe@example.com', work: true }],
segment: 'partner',
stage: 'current'
};
try {
const response = await axios.post(endpoint, contactData, {
params: {
user: userEmail,
api_key: apiKey
},
headers: {
'Content-Type': 'application/json'
}
});
console.log('Contact Updated:', response.data);
} catch (error) {
console.error('Error updating contact:', error.response.data);
}
};
updateContact();
This code updates the contact's segment and stage. Ensure you replace the placeholders with actual values.
Verifying API Call Success and Handling Errors
After making an API call, it's crucial to verify its success. Check the response for an error code of 0
, which indicates success. If an error occurs, the response will contain a human-readable error message. Always handle errors gracefully to improve user experience.
For more information on error codes, refer to the Cloze API documentation.
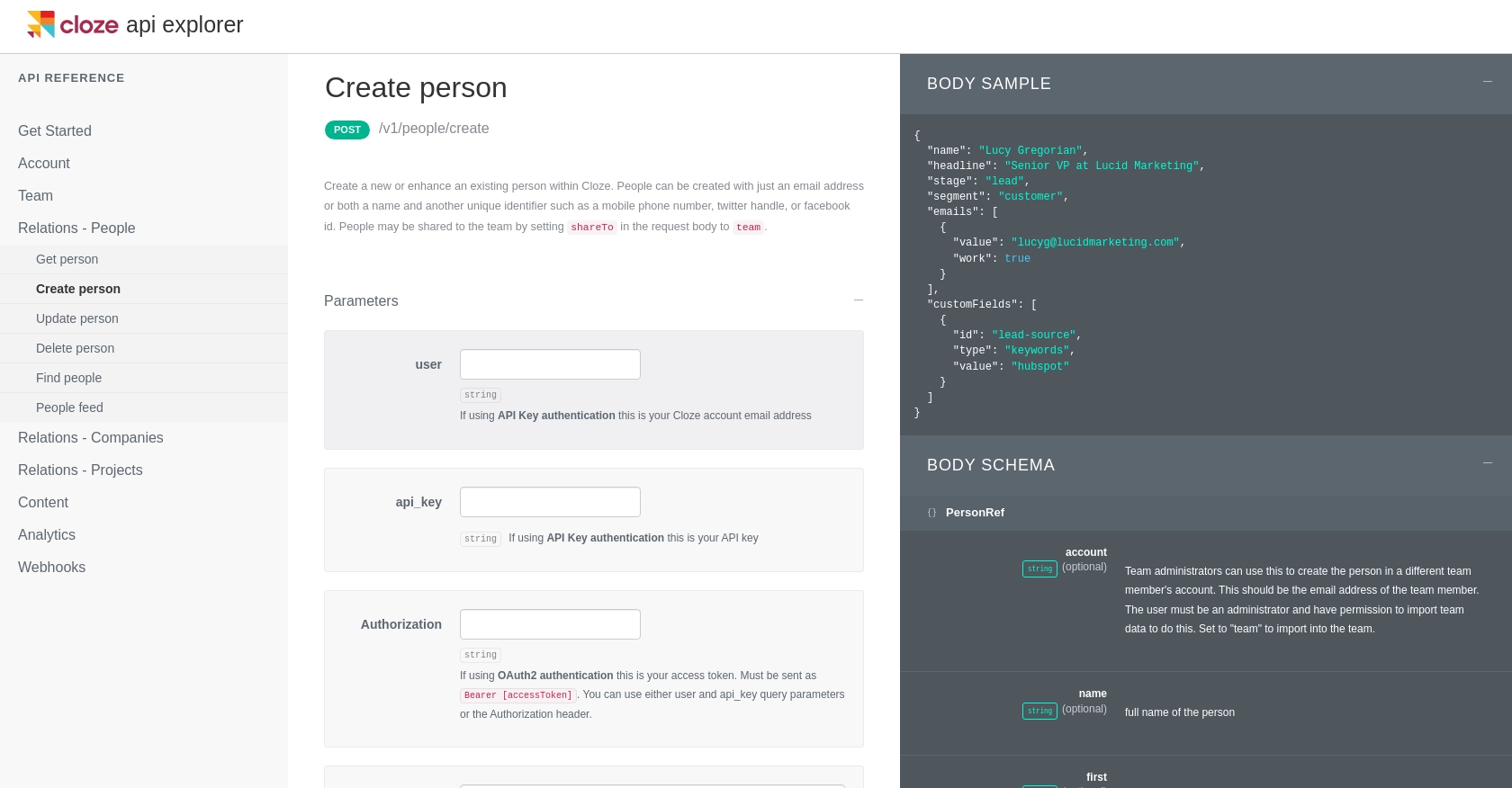
Conclusion and Best Practices for Using the Cloze API with JavaScript
Integrating with the Cloze API using JavaScript offers a powerful way to automate and streamline contact management within your applications. By following the steps outlined in this guide, you can efficiently create and update contacts, ensuring your CRM data remains accurate and up-to-date.
Best Practices for Secure and Efficient API Integration with Cloze
- Secure Storage of API Keys: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure consistent data formats when sending and receiving data to maintain data integrity across systems.
- Error Handling: Implement robust error handling to capture and log errors, providing meaningful feedback to users and aiding in debugging.
Leverage Endgrate for Simplified Integration Management
For developers looking to streamline their integration processes further, consider using Endgrate. Endgrate allows you to manage multiple integrations through a single API endpoint, saving time and resources. By outsourcing integration management to Endgrate, you can focus on your core product development while ensuring a seamless integration experience for your users.
Visit Endgrate to learn more about how it can enhance your integration capabilities and simplify your development workflow.
Read More
Ready to get started?