Using the Confluence API to Get Space Pages in PHP
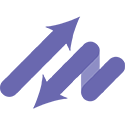
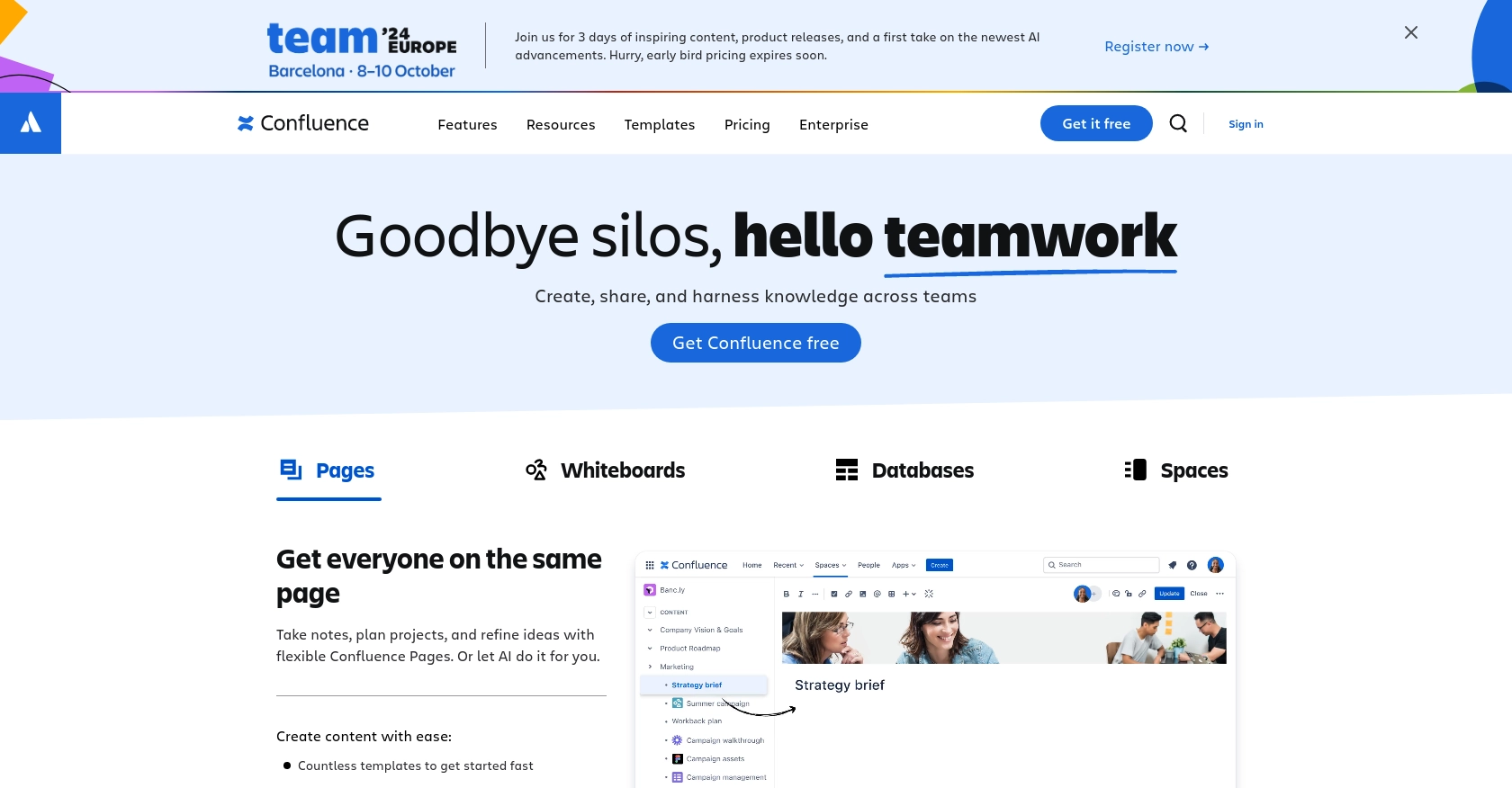
Introduction to Confluence API Integration
Confluence, developed by Atlassian, is a powerful collaboration tool used by teams to create, share, and manage content seamlessly. It serves as a centralized platform for documentation, project management, and knowledge sharing, making it an essential tool for businesses aiming to enhance productivity and communication.
Integrating with the Confluence API allows developers to automate and streamline content management tasks. For example, you might want to retrieve all pages within a specific space to generate reports or synchronize content with other applications. This can be particularly useful for maintaining up-to-date documentation across various platforms.
Setting Up Your Confluence Test or Sandbox Account
Before diving into the Confluence API integration, it's essential to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your live data. Atlassian provides developers with the tools needed to create and manage these accounts efficiently.
Creating a Confluence Sandbox Account
If you don't already have a Confluence account, you can sign up for a free trial on the Atlassian website. This trial will give you access to a sandbox environment where you can test API interactions.
- Visit the Confluence Free Trial page.
- Follow the instructions to create your account.
- Once your account is set up, log in to access your Confluence dashboard.
Setting Up OAuth Authentication for Confluence API
The Confluence API uses OAuth 2.0 for authentication, ensuring secure access to your data. Follow these steps to set up OAuth authentication:
- Navigate to the Atlassian Developer Console and log in with your Atlassian account.
- Click on Create App to start a new application.
- Fill in the required details such as app name and description.
- Under the Authentication section, select OAuth 2.0.
- Note down the Client ID and Client Secret as you'll need these for API requests.
- Set the necessary scopes for your app, such as
read:page:confluence
to access page data. - Save your app settings and ensure your app is enabled.
For more detailed information on authentication, refer to the Confluence API Authentication Documentation.
Generating an Access Token
Once your app is set up, you'll need to generate an access token to authenticate API requests:
- Use the OAuth 2.0 Authorization Code Grant flow to obtain an access token.
- Direct users to the authorization URL with your client ID and requested scopes.
- After user consent, you'll receive an authorization code.
- Exchange this code for an access token using your client ID and client secret.
With your sandbox account and OAuth setup complete, you're ready to start making API calls to Confluence. This setup ensures that your integration is secure and compliant with Atlassian's standards.
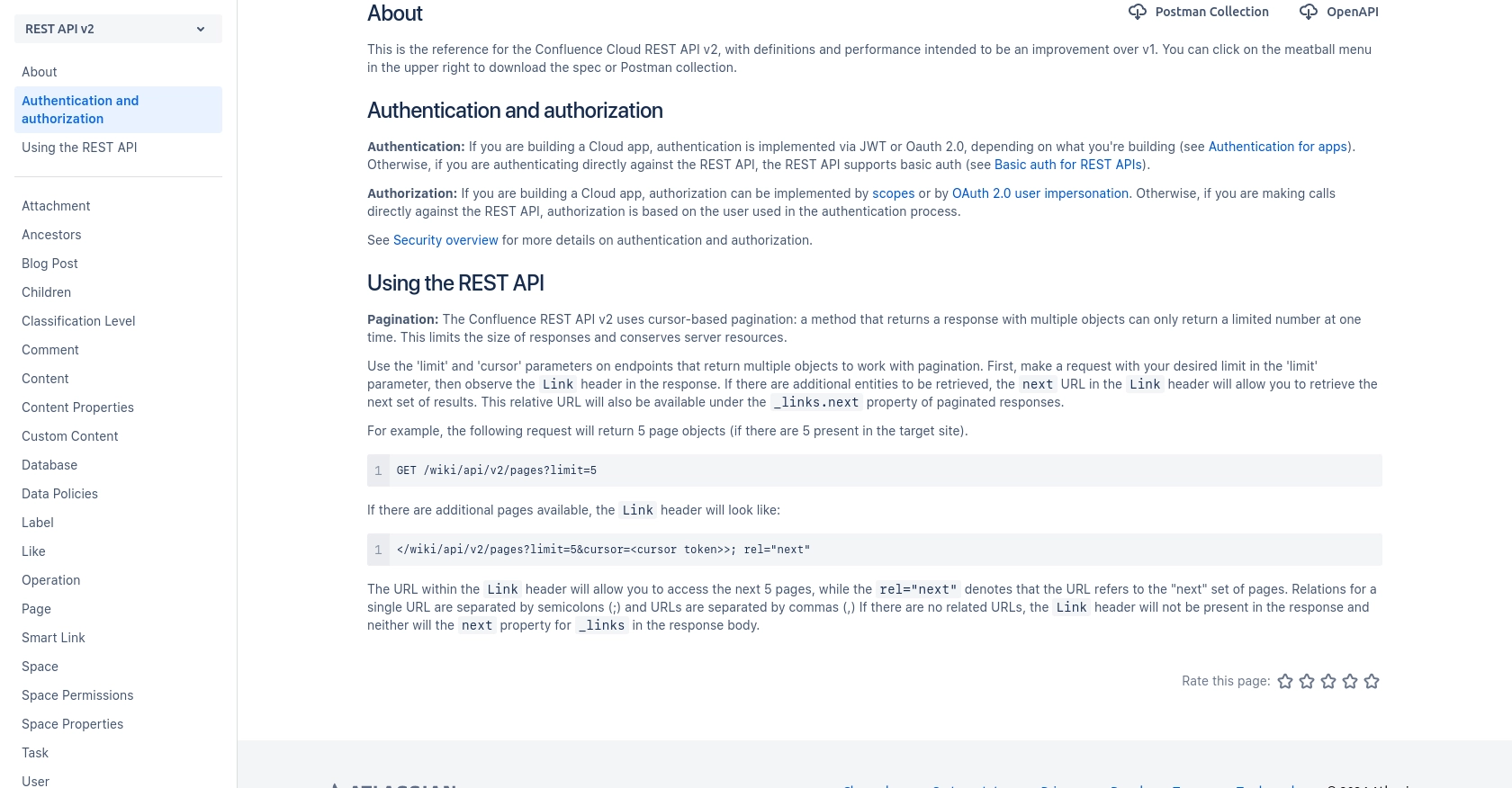
sbb-itb-96038d7
Making API Calls to Retrieve Confluence Space Pages Using PHP
To interact with the Confluence API and retrieve pages from a specific space, you'll need to make HTTP requests using PHP. This section will guide you through setting up your PHP environment and executing the necessary API calls.
Setting Up Your PHP Environment
Before making API calls, ensure your PHP environment is correctly configured. You'll need:
- PHP version 7.4 or higher
- cURL extension enabled in your PHP installation
To verify your PHP version and cURL extension, run the following command in your terminal:
php -v
php -m | grep curl
Installing Required PHP Dependencies
For making HTTP requests, we'll use the cURL library, which is typically included with PHP. If not, you can install it via your package manager. Additionally, Composer can be used to manage other dependencies if needed.
Example Code to Retrieve Confluence Space Pages
Below is a sample PHP script to retrieve pages from a specific Confluence space using the API:
<?php
// Set your access token and space ID
$accessToken = 'Your_Access_Token';
$spaceId = 'Your_Space_ID';
// Define the API endpoint
$url = "https://your-domain.atlassian.net/wiki/api/v2/spaces/$spaceId/pages";
// Initialize cURL session
$ch = curl_init($url);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $accessToken,
'Accept: application/json'
]);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Decode and display the response
$data = json_decode($response, true);
foreach ($data['results'] as $page) {
echo "Page Title: " . $page['title'] . "\n";
}
}
// Close cURL session
curl_close($ch);
?>
Replace Your_Access_Token
and Your_Space_ID
with your actual access token and space ID. This script initializes a cURL session, sets the necessary headers, and executes a GET request to the Confluence API to fetch pages.
Handling API Response and Errors
Upon executing the API call, the response will include the requested pages. If successful, the pages' titles will be printed. In case of errors, the script will output the error message. Common HTTP status codes to handle include:
- 200 OK: Request succeeded, and the pages are returned.
- 401 Unauthorized: Authentication failed. Check your access token.
- 404 Not Found: The specified space ID does not exist.
For more details on error handling, refer to the Confluence API Documentation.
Verifying API Call Success in Confluence
After running the script, verify the retrieved pages by checking your Confluence space. The pages listed in the API response should match those in your Confluence dashboard.
By following these steps, you can efficiently retrieve and manage Confluence space pages using PHP, streamlining your content management tasks.
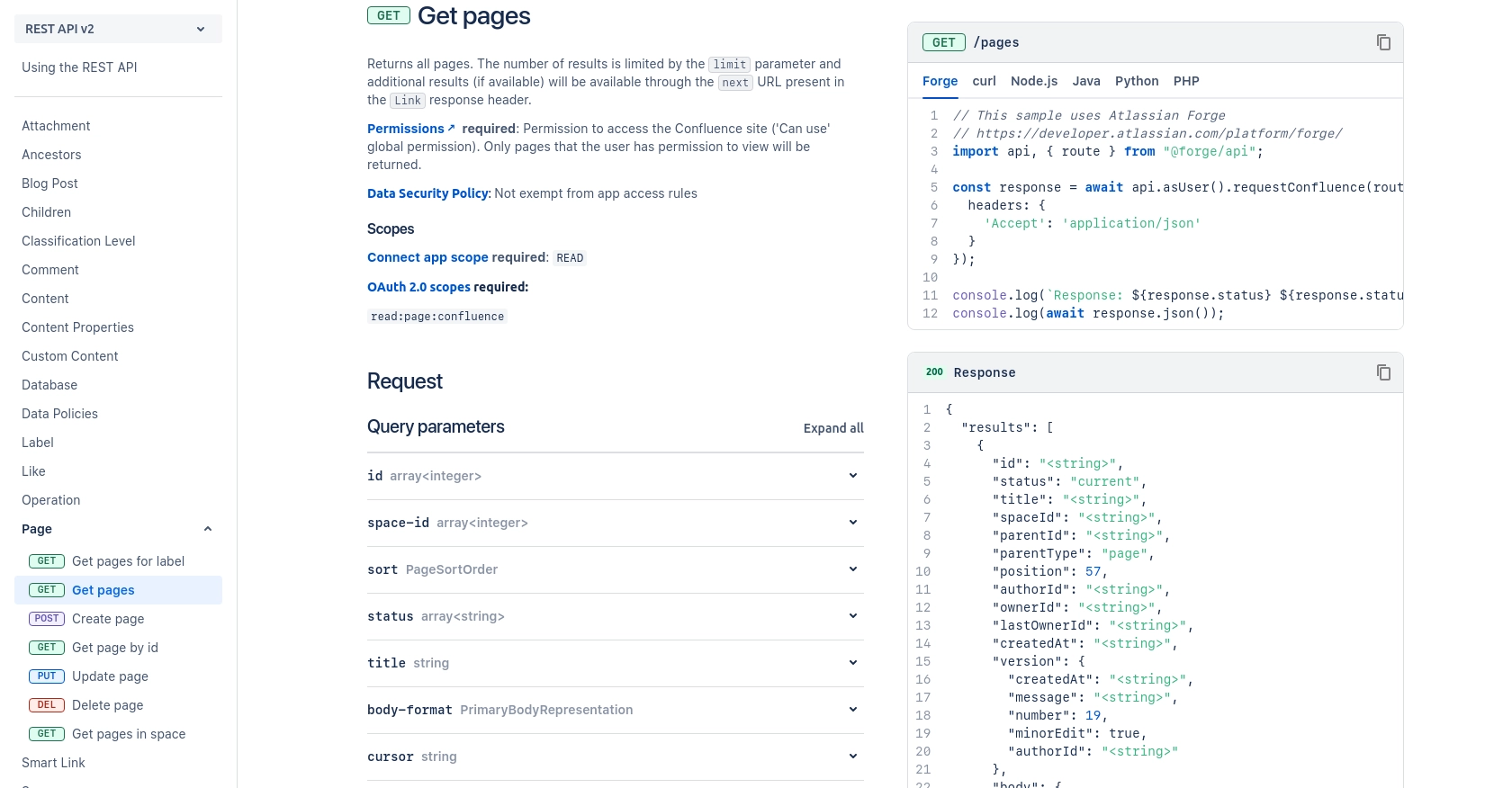
Conclusion and Best Practices for Using Confluence API with PHP
Integrating with the Confluence API using PHP provides a robust solution for automating content management tasks within your organization. By following the steps outlined in this guide, you can efficiently retrieve and manage pages within specific Confluence spaces, enhancing your team's productivity and collaboration.
Best Practices for Secure and Efficient Confluence API Integration
- Secure Storage of Credentials: Always store your access tokens and client secrets securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of Confluence's API rate limits to avoid throttling. Implement retry logic with exponential backoff to manage API requests efficiently.
- Data Transformation and Standardization: Ensure that data retrieved from Confluence is transformed and standardized to fit your application's requirements, maintaining consistency across platforms.
Streamlining Integrations with Endgrate
While building custom integrations can be rewarding, it can also be time-consuming and complex. Endgrate offers a unified API solution that simplifies the process of connecting with multiple platforms, including Confluence. By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development while providing an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate and discover the benefits of a streamlined, efficient integration process.
Read More
Ready to get started?