Using the Endear API to Create Tasks in Javascript
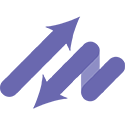
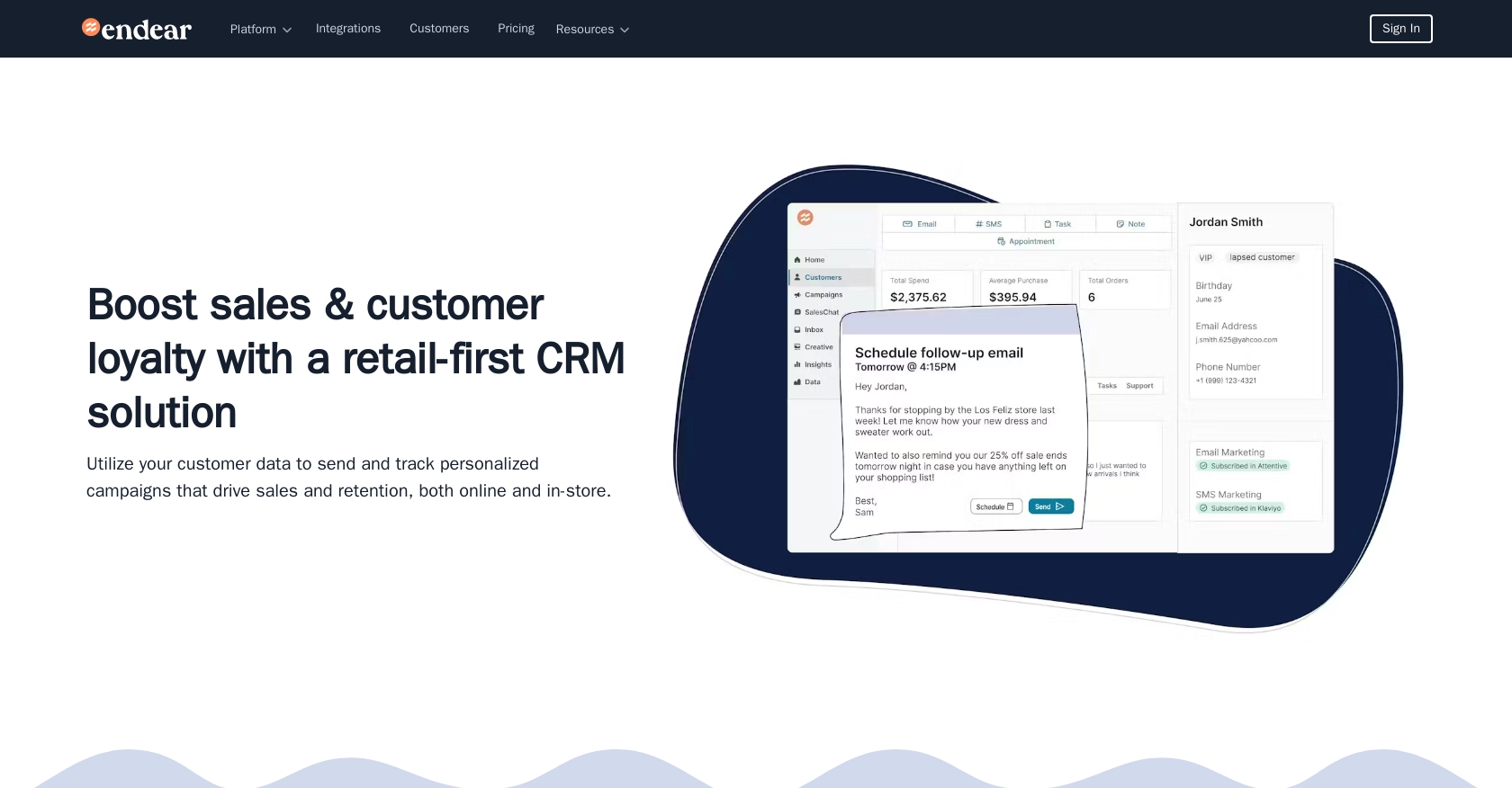
Introduction to Endear API for Task Management
Endear is a powerful CRM platform designed to enhance customer relationships through seamless integration and data management. It offers a comprehensive suite of tools that allow businesses to manage customer interactions effectively, making it an ideal choice for companies looking to streamline their operations.
Developers may want to integrate with Endear's API to automate task management processes, such as creating and assigning tasks within the platform. For example, a developer could use the Endear API to automatically generate tasks based on customer interactions, ensuring timely follow-ups and improving customer service efficiency.
Setting Up Your Endear Test Account for API Integration
Before you begin integrating with the Endear API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Endear provides a straightforward process to create an integration and obtain an API key, which is essential for authenticating your requests.
Steps to Create an Integration and Obtain Your Endear API Key
- Visit Your Endear Settings: Log in to your Endear account and navigate to the settings page.
- Access Integrations: Click on the "Integrations" section to view available options.
- Add a New Integration: Select "Add Integration" and choose the "API" option from the list.
- Fill in the Required Details: Provide the necessary information to create your integration. This may include naming your integration and specifying any additional settings.
- Generate Your API Key: Once the integration is set up, you'll receive an API key. Make sure to copy and store this key securely, as it will be used to authenticate your API requests.
With your API key in hand, you can now access the Endear API by including the header X-Endear-Api-Key
with the value of your generated API key in your requests. Remember, each API key is scoped to a specific brand, so you'll need a separate key for each brand you wish to integrate with.
For more detailed information, you can refer to the Endear Authentication Documentation.
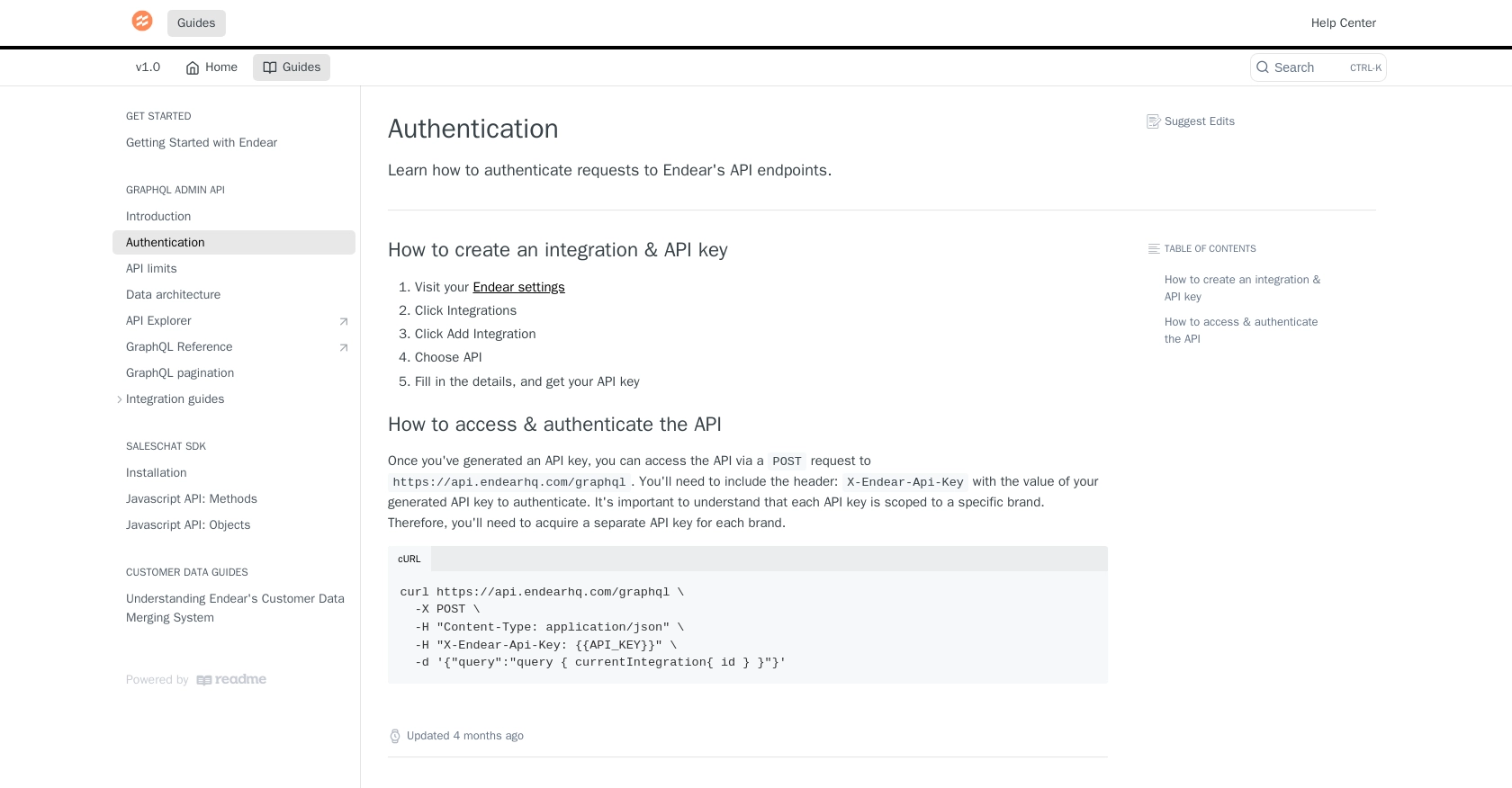
sbb-itb-96038d7
How to Make API Calls to Create Tasks Using Endear API in JavaScript
To interact with the Endear API for creating tasks, you'll need to use JavaScript to send HTTP requests. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses and errors effectively.
Setting Up Your JavaScript Environment for Endear API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Node.js (preferably version 14.x or higher)
- A code editor like Visual Studio Code
Once you have Node.js installed, you can use npm (Node Package Manager) to install any additional dependencies. For this tutorial, we'll use the node-fetch
library to make HTTP requests. Install it by running:
npm install node-fetch
Writing JavaScript Code to Create Tasks with Endear API
Now, let's write the JavaScript code to create a task using the Endear API. Create a new file named createTask.js
and add the following code:
const fetch = require('node-fetch');
// Define the API endpoint and headers
const endpoint = 'https://api.endearhq.com/graphql';
const headers = {
'Content-Type': 'application/json',
'X-Endear-Api-Key': 'Your_API_Key'
};
// Define the GraphQL mutation for creating a task
const mutation = `
mutation CreateTask($input: TaskInput!) {
createTask(input: $input) {
task {
id
created_at
note {
id
}
}
}
}`;
// Define the task details
const taskDetails = {
input: {
noteId: 'Note_ID',
assignees: ['User_ID'],
deadline: '2023-12-31T23:59:59Z'
}
};
// Make the POST request to the API
fetch(endpoint, {
method: 'POST',
headers: headers,
body: JSON.stringify({
query: mutation,
variables: taskDetails
})
})
.then(response => response.json())
.then(data => {
if (data.errors) {
console.error('Error creating task:', data.errors);
} else {
console.log('Task created successfully:', data.data.createTask.task);
}
})
.catch(error => console.error('Fetch error:', error));
Replace Your_API_Key
, Note_ID
, and User_ID
with your actual API key, note ID, and user ID respectively.
Running the JavaScript Code and Verifying Task Creation
To run the code, execute the following command in your terminal:
node createTask.js
If successful, you should see a confirmation message with the task details. You can verify the task creation by checking your Endear account.
Handling Errors and Understanding Endear API Rate Limits
When making API calls, it's crucial to handle potential errors. The Endear API may return HTTP status codes such as 429 if you exceed the rate limit of 120 requests per minute. Always check the response for errors and implement retry logic if necessary.
For more information on error handling and rate limits, refer to the Endear Rate Limits and Errors Documentation.
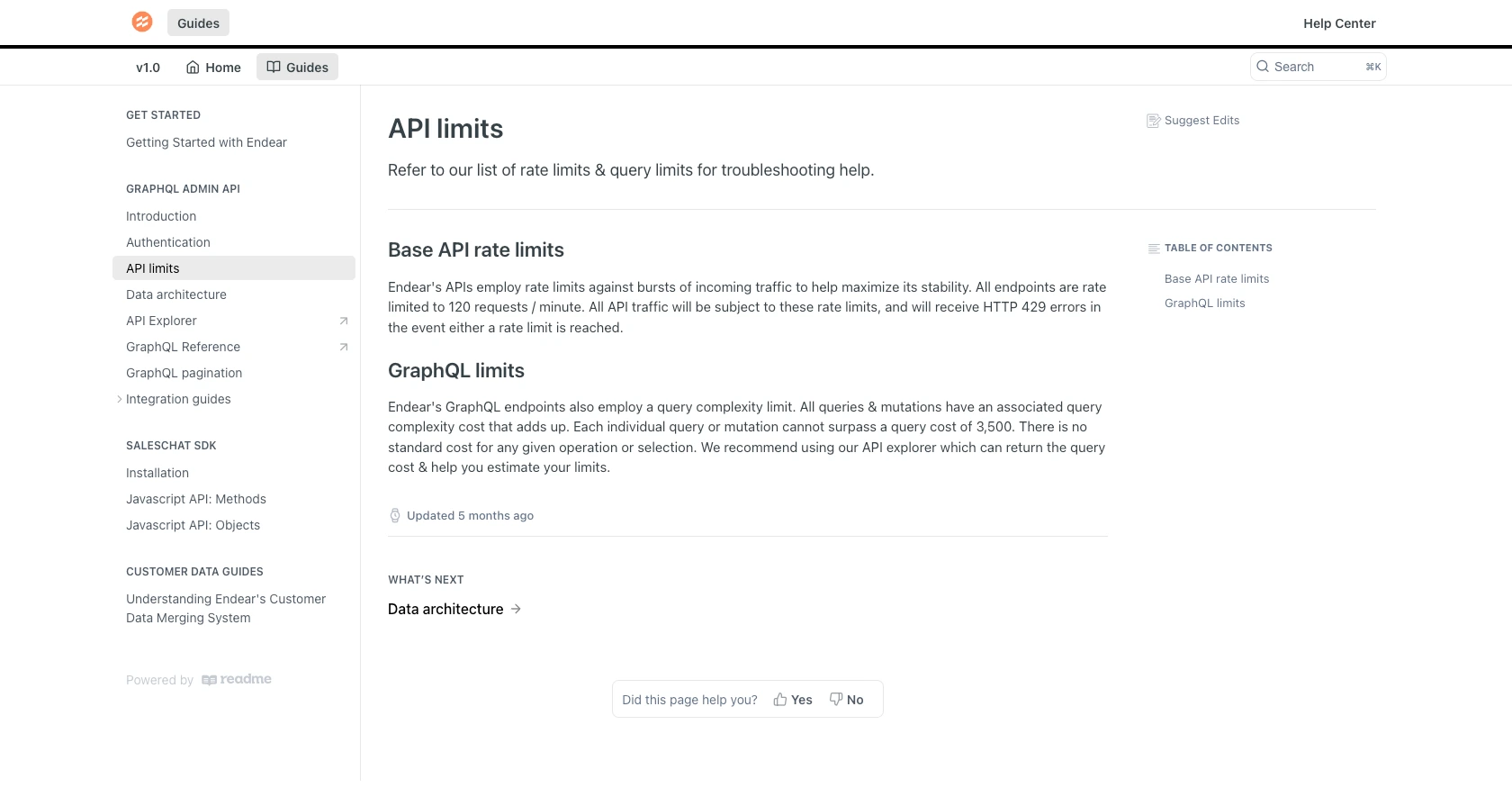
Conclusion and Best Practices for Using Endear API in JavaScript
Integrating with the Endear API to manage tasks in JavaScript can significantly enhance your workflow automation and improve customer relationship management. By following the steps outlined in this guide, you can efficiently create tasks and ensure seamless task management within the Endear platform.
Best Practices for Secure and Efficient Endear API Integration
- Secure API Key Storage: Always store your API keys securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Endear's rate limit of 120 requests per minute. Implement retry logic and exponential backoff to handle HTTP 429 errors gracefully.
- Data Standardization: Ensure that your task data is consistent and standardized to avoid conflicts and maintain data integrity across integrations.
Enhance Your Integration Strategy with Endgrate
While integrating with Endear's API provides powerful capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Endear. This allows you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration strategy by visiting Endgrate today.
Read More
- https://endgrate.com/provider/endear
- https://docs.endearhq.com/docs/authentication
- https://docs.endearhq.com/docs/rate-limits-status-codes-and-errors
- https://docs.endearhq.com/docs/disclaimers
- https://developers.endearhq.com/docs/graphql/objects/Task
- https://docs.endearhq.com/docs/send-your-first-record
Ready to get started?