Using the Freshsales API to Create or Update Contacts in Javascript
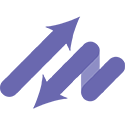
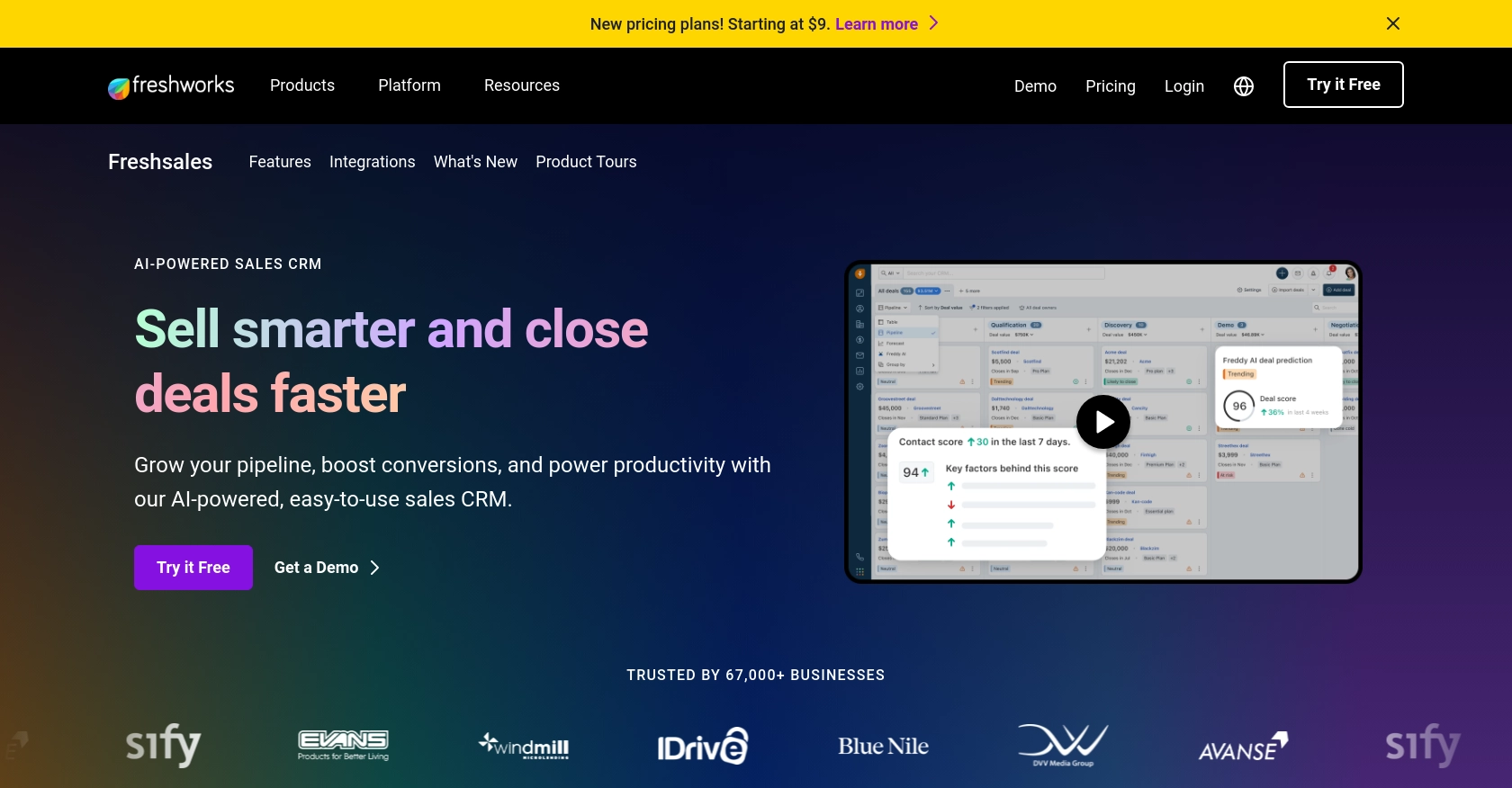
Introduction to Freshsales API Integration
Freshsales is a powerful customer relationship management (CRM) platform designed to help businesses manage their sales processes more effectively. With features like lead scoring, email tracking, and built-in phone capabilities, Freshsales offers a comprehensive solution for sales teams looking to enhance their productivity and close deals faster.
Integrating with the Freshsales API allows developers to automate and streamline CRM tasks, such as creating or updating contact information. For example, a developer might use the Freshsales API to automatically update contact details in response to changes in an external database, ensuring that sales teams always have access to the most current information.
Setting Up Your Freshsales Test or Sandbox Account
Before you can start integrating with the Freshsales API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a Freshsales Account
If you don't already have a Freshsales account, you can sign up for a free trial on the Freshsales website. This trial will give you access to the platform's features, including API capabilities.
- Visit the Freshsales signup page.
- Fill in the required information, such as your name, email, and company details.
- Follow the instructions to complete the registration process.
Generating Freshsales API Key for Authentication
Freshsales uses a custom authentication method that requires an API key. Here's how you can generate your API key:
- Log in to your Freshsales account.
- Navigate to the settings by clicking on your profile icon in the top right corner.
- Select "API Settings" from the dropdown menu.
- Click on "Generate New API Key" and copy the key provided.
- Store this API key securely, as you'll need it to authenticate your API requests.
Configuring Freshsales API Access
Once you have your API key, you can configure your application to interact with the Freshsales API:
- Ensure you have access to the necessary permissions for creating or updating contacts.
- Review the Freshsales API documentation at Freshsales API Documentation for detailed information on endpoints and request formats.
With your Freshsales account and API key ready, you're all set to start making API calls to create or update contacts using JavaScript.
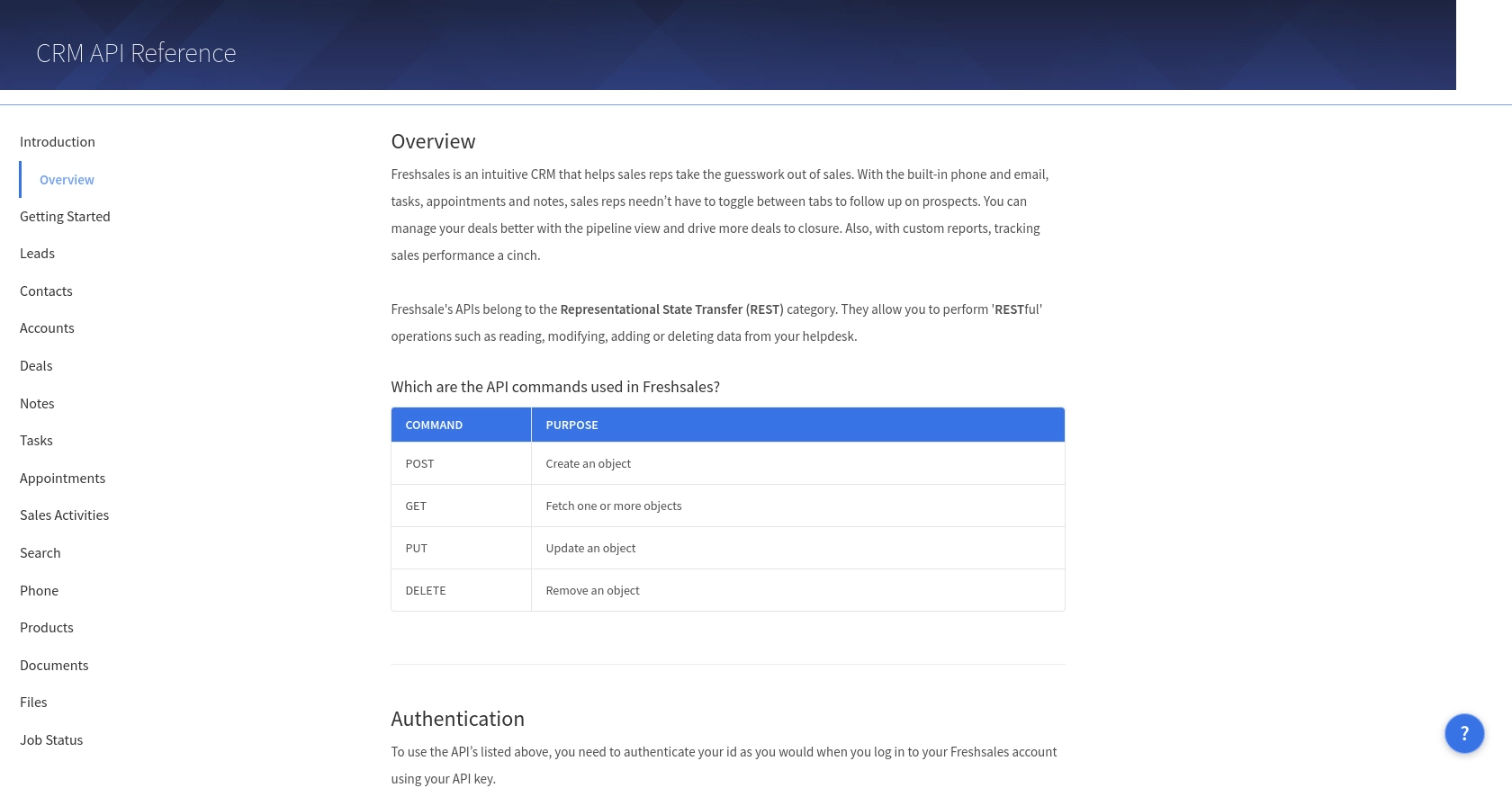
sbb-itb-96038d7
Making API Calls to Freshsales Using JavaScript
To interact with the Freshsales API using JavaScript, you'll need to set up your environment and write code to make HTTP requests. This section will guide you through the process of creating or updating contacts using the Freshsales API.
Setting Up Your JavaScript Environment for Freshsales API
Before making API calls, ensure you have a working JavaScript environment. You can use Node.js for server-side scripting or a browser-based environment for client-side scripting. For this tutorial, we'll use Node.js.
- Ensure you have Node.js installed on your machine. You can download it from the official Node.js website.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
axios
library to handle HTTP requests by runningnpm install axios
.
Creating or Updating Contacts with Freshsales API
With your environment set up, you can now write JavaScript code to create or update contacts in Freshsales. Here's a step-by-step guide:
const axios = require('axios');
// Set your Freshsales API key and domain
const apiKey = 'Your_API_Key';
const domain = 'yourdomain.freshsales.io';
// Function to create or update a contact
async function createOrUpdateContact(contactData) {
try {
const response = await axios({
method: 'post', // Use 'put' for updating an existing contact
url: `https://${domain}/api/contacts`,
headers: {
'Authorization': `Token token=${apiKey}`,
'Content-Type': 'application/json'
},
data: contactData
});
console.log('Contact created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating contact:', error.response ? error.response.data : error.message);
}
}
// Example contact data
const contactData = {
contact: {
first_name: 'John',
last_name: 'Doe',
email: 'john.doe@example.com'
}
};
// Call the function to create or update a contact
createOrUpdateContact(contactData);
In this code, we use the axios
library to make a POST request to the Freshsales API. Replace Your_API_Key
and yourdomain.freshsales.io
with your actual API key and Freshsales domain.
Verifying API Call Success in Freshsales
After running the script, you can verify the success of the API call by checking the Freshsales dashboard. Navigate to the contacts section to see if the contact has been created or updated as expected.
Handling Errors and Troubleshooting
When making API calls, it's crucial to handle potential errors. The code above includes a try-catch block to catch and log any errors that occur during the request. Common errors might include:
- Invalid API key or domain.
- Incorrect request format or missing required fields.
- Network issues or server errors.
Refer to the Freshsales API documentation for more details on error codes and troubleshooting tips.
Conclusion and Best Practices for Freshsales API Integration
Integrating with the Freshsales API using JavaScript can significantly enhance your CRM capabilities by automating contact management tasks. By following the steps outlined in this guide, you can efficiently create or update contacts, ensuring your sales team has access to the most accurate and up-to-date information.
Best Practices for Secure and Efficient Freshsales API Usage
- Securely Store API Keys: Always store your Freshsales API keys securely and avoid hardcoding them in your source code. Consider using environment variables or a secure vault.
- Handle Rate Limiting: Be mindful of Freshsales API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Validate Data: Ensure that the data being sent to the Freshsales API is validated and sanitized to prevent errors and maintain data integrity.
- Monitor API Usage: Regularly monitor your API usage and error logs to identify and resolve issues promptly.
Streamlining Integrations with Endgrate
While integrating with the Freshsales API can be straightforward, managing multiple integrations across different platforms can become complex. This is where Endgrate can be a valuable asset.
Endgrate offers a unified API endpoint that simplifies the integration process, allowing you to connect with multiple platforms, including Freshsales, through a single API call. By leveraging Endgrate, you can save time and resources, focusing on your core product development while ensuring a seamless integration experience for your customers.
Explore how Endgrate can transform your integration strategy and enhance your product's capabilities by visiting Endgrate's website today.
Read More
Ready to get started?