Using the Google Analytics API to Get Analytics By Page (with PHP examples)
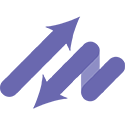
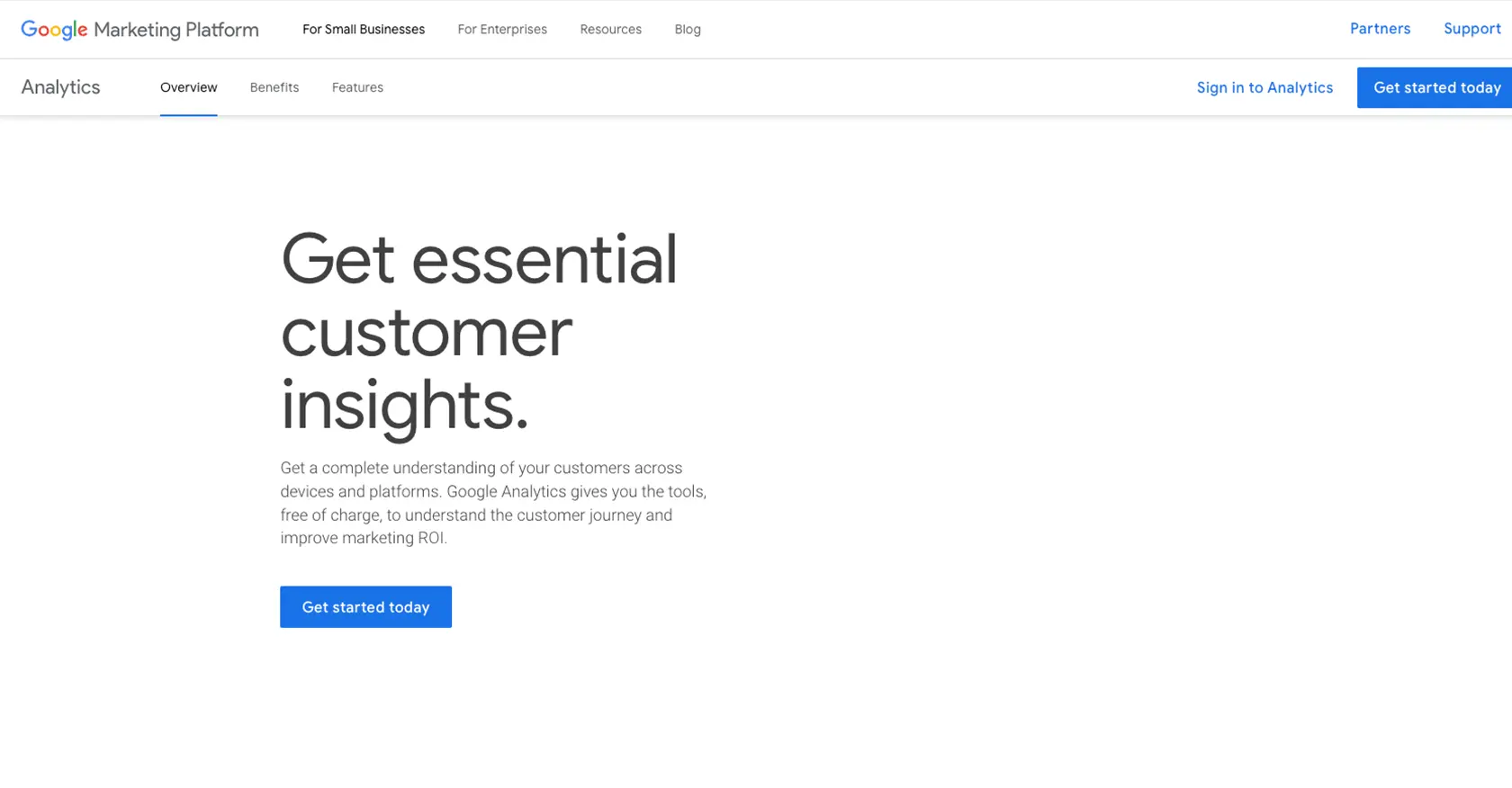
Introduction to Google Analytics API
Google Analytics is a powerful tool that provides insights into website traffic and user behavior, helping businesses make data-driven decisions. It offers a comprehensive suite of features to track and analyze various metrics, such as page views, user sessions, and conversion rates.
Developers often integrate with the Google Analytics API to automate reporting and gain deeper insights into user interactions. For example, you can use the API to retrieve analytics data by page, enabling you to understand which pages are performing well and which need improvement.
This article will guide you through using the Google Analytics API with PHP to extract analytics data by page, providing a step-by-step approach to setting up and executing API calls.
Setting Up Your Google Analytics API Test Account
Before you can start using the Google Analytics API, you need to set up a Google Cloud project and enable the necessary APIs. This setup will allow you to authenticate and interact with the Google Analytics API securely.
Create a Google Cloud Project
To begin, you need a Google Cloud project. This project will serve as the foundation for enabling APIs and managing credentials.
- Visit the Google Cloud Console.
- Click on the project dropdown at the top and select "New Project."
- Enter a name for your project and click "Create."
Enable Google Analytics API
Next, you need to enable the Google Analytics API for your project.
- In the Google Cloud Console, navigate to APIs & Services > Library.
- Search for "Google Analytics API" and click on it.
- Click the "Enable" button to activate the API for your project.
Configure OAuth Consent Screen
Setting up the OAuth consent screen is crucial for user authentication.
- Go to APIs & Services > OAuth consent screen in the Google Cloud Console.
- Select the user type and fill in the required fields, such as application name and support email.
- Click "Save and Continue" to proceed.
Create OAuth Credentials
Now, create OAuth credentials to authenticate your API requests.
- Navigate to APIs & Services > Credentials.
- Click "Create Credentials" and select "OAuth client ID."
- Choose "Web application" as the application type.
- Enter a name for your credentials and configure the authorized redirect URIs.
- Click "Create" to generate your client ID and client secret.
Make sure to securely store your client ID and client secret, as you'll need them for API authentication.
For more detailed instructions, refer to the official Google documentation: Create a Google Cloud Project, Enable APIs, Configure OAuth Consent, and Create Credentials.
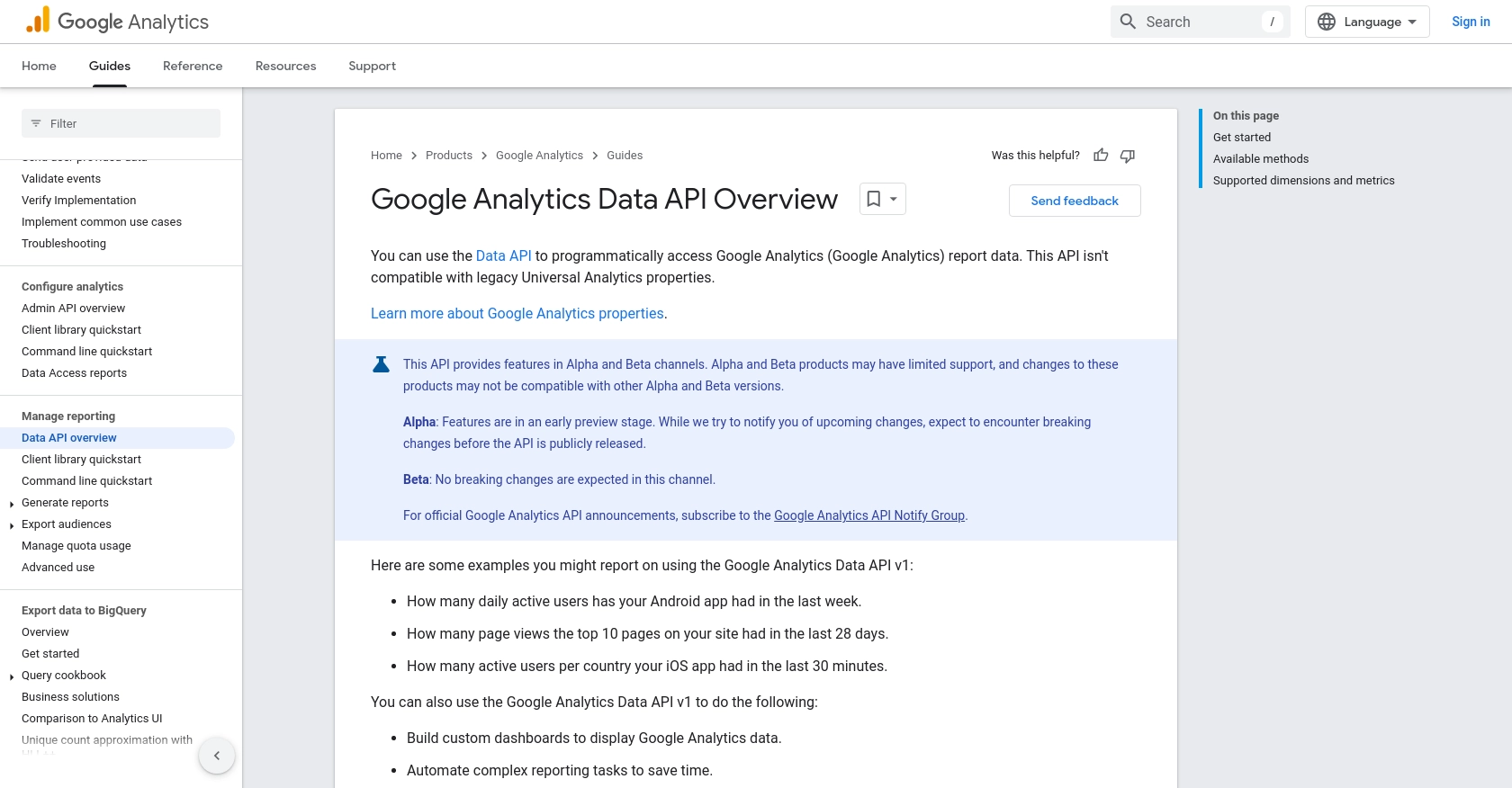
sbb-itb-96038d7
Making API Calls to Google Analytics Using PHP
To interact with the Google Analytics API and retrieve analytics data by page, you need to make API calls using PHP. This section will guide you through the necessary steps, including setting up your PHP environment, installing dependencies, and executing API requests.
Setting Up Your PHP Environment
Before making API calls, ensure your PHP environment is correctly configured. You'll need PHP 7.4 or later and the Composer package manager to manage dependencies.
- Verify your PHP installation by running
php -v
in your terminal. - Install Composer if it's not already installed. Follow the instructions on the Composer website.
Installing Required PHP Dependencies
You'll need the Google API Client Library for PHP to interact with the Google Analytics API. Install it using Composer:
composer require google/apiclient:^2.0
Executing Google Analytics API Calls in PHP
With your environment set up, you can now make API calls to Google Analytics. Below is a sample PHP script to retrieve analytics data by page:
require 'vendor/autoload.php';
use Google\Client;
use Google\Service\AnalyticsData;
// Initialize the Google Client
$client = new Client();
$client->setAuthConfig('path/to/your/client_credentials.json');
$client->addScope('https://www.googleapis.com/auth/analytics.readonly');
// Initialize the Analytics Data service
$analytics = new AnalyticsData($client);
// Define the request parameters
$request = new AnalyticsData\RunReportRequest([
'property' => 'properties/YOUR-GA4-PROPERTY-ID',
'dateRanges' => [
['startDate' => '30daysAgo', 'endDate' => 'today']
],
'dimensions' => [
['name' => 'pagePath']
],
'metrics' => [
['name' => 'pageviews']
]
]);
// Execute the request
$response = $analytics->properties->runReport($request);
// Output the results
foreach ($response->getRows() as $row) {
printf("Page: %s, Pageviews: %s\n", $row->getDimensionValues()[0]->getValue(), $row->getMetricValues()[0]->getValue());
}
Replace path/to/your/client_credentials.json
with the path to your OAuth credentials file and YOUR-GA4-PROPERTY-ID
with your Google Analytics 4 property ID.
Verifying API Call Success
After running the script, check the output to ensure it matches the data in your Google Analytics account. If the API call is successful, you should see a list of pages and their corresponding pageviews.
Handling Errors and Debugging
To handle errors, wrap your API calls in a try-catch block and log any exceptions. This will help you identify issues such as authentication errors or incorrect request parameters.
try {
// Execute the request
$response = $analytics->properties->runReport($request);
// Output the results
foreach ($response->getRows() as $row) {
printf("Page: %s, Pageviews: %s\n", $row->getDimensionValues()[0]->getValue(), $row->getMetricValues()[0]->getValue());
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
For more detailed information on error codes and handling, refer to the Google Analytics API documentation.
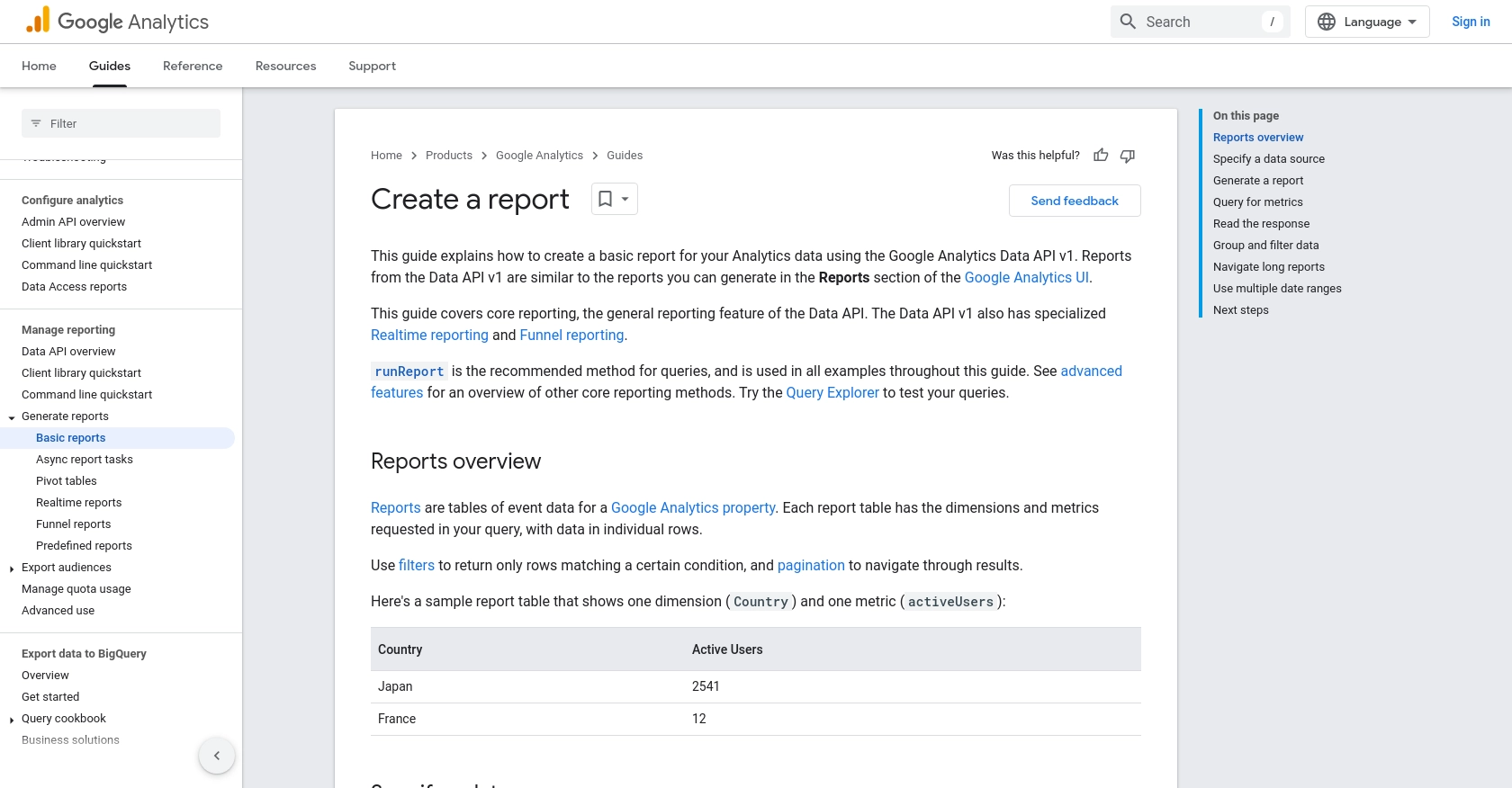
Conclusion: Best Practices for Using Google Analytics API with PHP
Integrating Google Analytics API with PHP offers a powerful way to automate data retrieval and gain insights into your website's performance. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate your requests, and execute API calls to gather valuable analytics data.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always keep your OAuth credentials secure. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of Google Analytics API rate limits. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Optimize Data Requests: Request only the data you need by specifying precise dimensions and metrics. This will help reduce response times and improve efficiency.
- Monitor API Usage: Regularly review your API usage in the Google Cloud Console to ensure compliance with quotas and to optimize performance.
Transforming and Standardizing Data for Business Insights
Once you retrieve data from the Google Analytics API, consider transforming and standardizing it to align with your business needs. This might involve aggregating data, normalizing metrics, or integrating it with other data sources for comprehensive analysis.
Streamline Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate provides a unified API endpoint that connects to various platforms, including Google Analytics, allowing you to focus on your core product while outsourcing integration complexities.
Explore how Endgrate can save you time and resources by visiting Endgrate today.
Read More
- https://endgrate.com/provider/googleanalytics
- https://developers.google.com/analytics/devguides/reporting/data/v1
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/analytics/devguides/reporting/data/v1/basics
Ready to get started?