Using the Google Docs API to Create Document Texts in Javascript
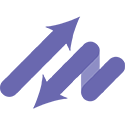
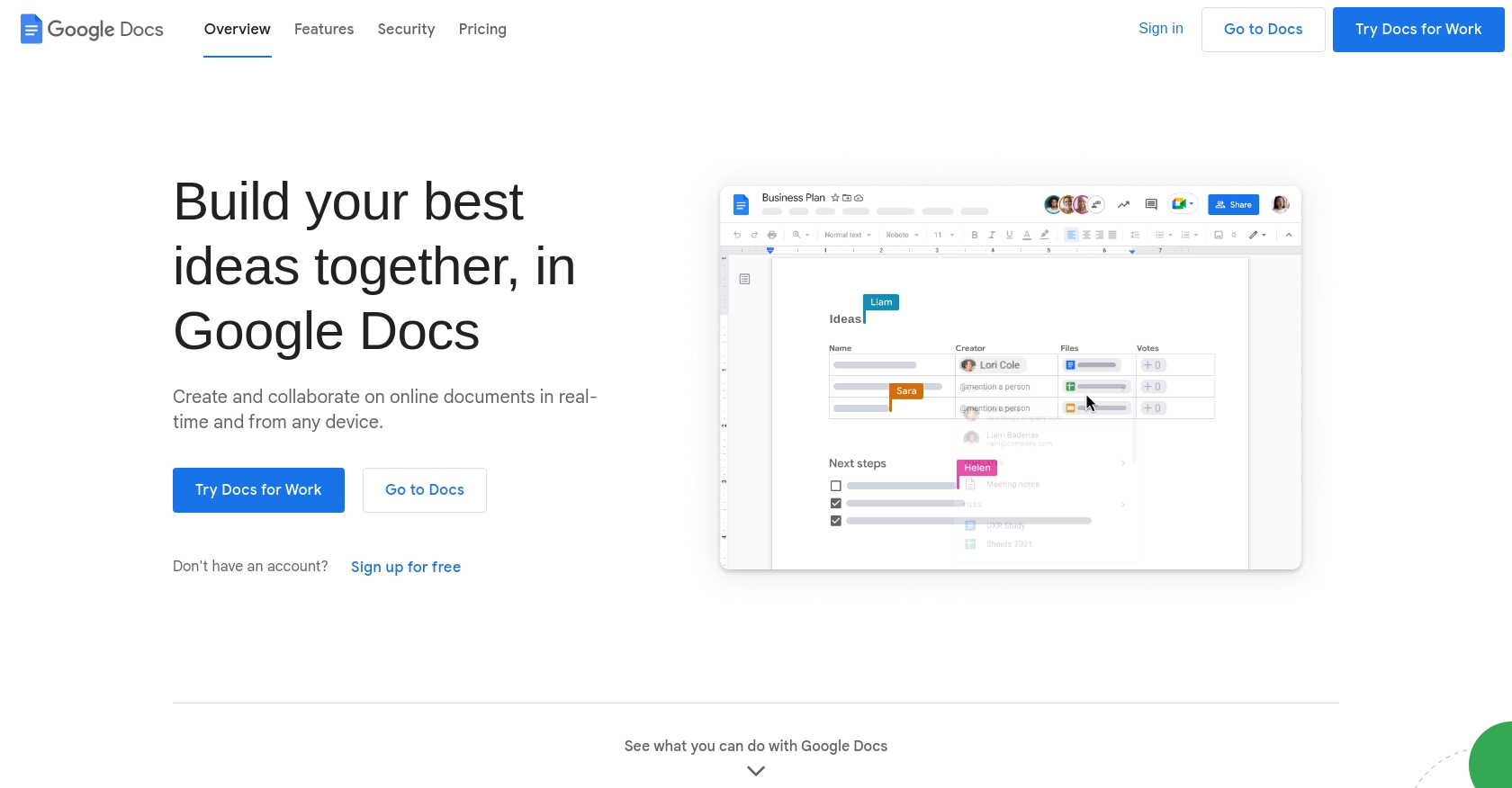
Introduction to Google Docs API
Google Docs is a widely used cloud-based word processing application that allows users to create, edit, and collaborate on documents in real-time. Its seamless integration with other Google Workspace apps makes it a powerful tool for both personal and professional use.
For developers, integrating with the Google Docs API opens up possibilities to automate document creation and management. By using the API, developers can programmatically create, edit, and format documents, enhancing productivity and enabling custom workflows.
For example, a developer might use the Google Docs API to automatically generate reports by inserting dynamic data into a document template. This can be particularly useful for businesses that need to produce regular reports with updated information.
Setting Up Your Google Docs API Test Environment
To begin using the Google Docs API, you'll need to set up a Google Cloud project and configure OAuth 2.0 authentication. This allows your application to interact with Google Docs securely and access the necessary resources.
Create a Google Cloud Project
First, create a Google Cloud project to manage your API credentials and settings. Follow these steps:
- Go to the Google Cloud Console.
- Click on the Menu icon and select IAM & Admin > Create a Project.
- Enter a descriptive name for your project and click Create.
Enable Google Docs API
Next, enable the Google Docs API for your project:
- In the Google Cloud Console, navigate to APIs & Services > Library.
- Search for "Google Docs API" and click on it.
- Click the Enable button.
Configure OAuth Consent Screen
Set up the OAuth consent screen to define how your app will request permissions:
- Go to APIs & Services > OAuth consent screen.
- Select the user type for your app and click Create.
- Fill out the required fields and click Save and Continue.
Create OAuth 2.0 Credentials
Now, create the OAuth 2.0 credentials needed for authentication:
- Navigate to APIs & Services > Credentials.
- Click Create Credentials and select OAuth client ID.
- Choose Web application as the application type.
- Enter a name for your credentials and add authorized redirect URIs.
- Click Create to generate your Client ID and Client Secret.
Authorize Your Application
With your credentials ready, you can now authorize your application to access Google Docs:
- Use the Client ID and Client Secret to initiate the OAuth 2.0 flow.
- Request access to the necessary scopes for interacting with Google Docs.
- Upon successful authorization, you'll receive an access token to make API calls.
For more detailed instructions, refer to the official Google documentation on creating a project, enabling APIs, and configuring OAuth consent.
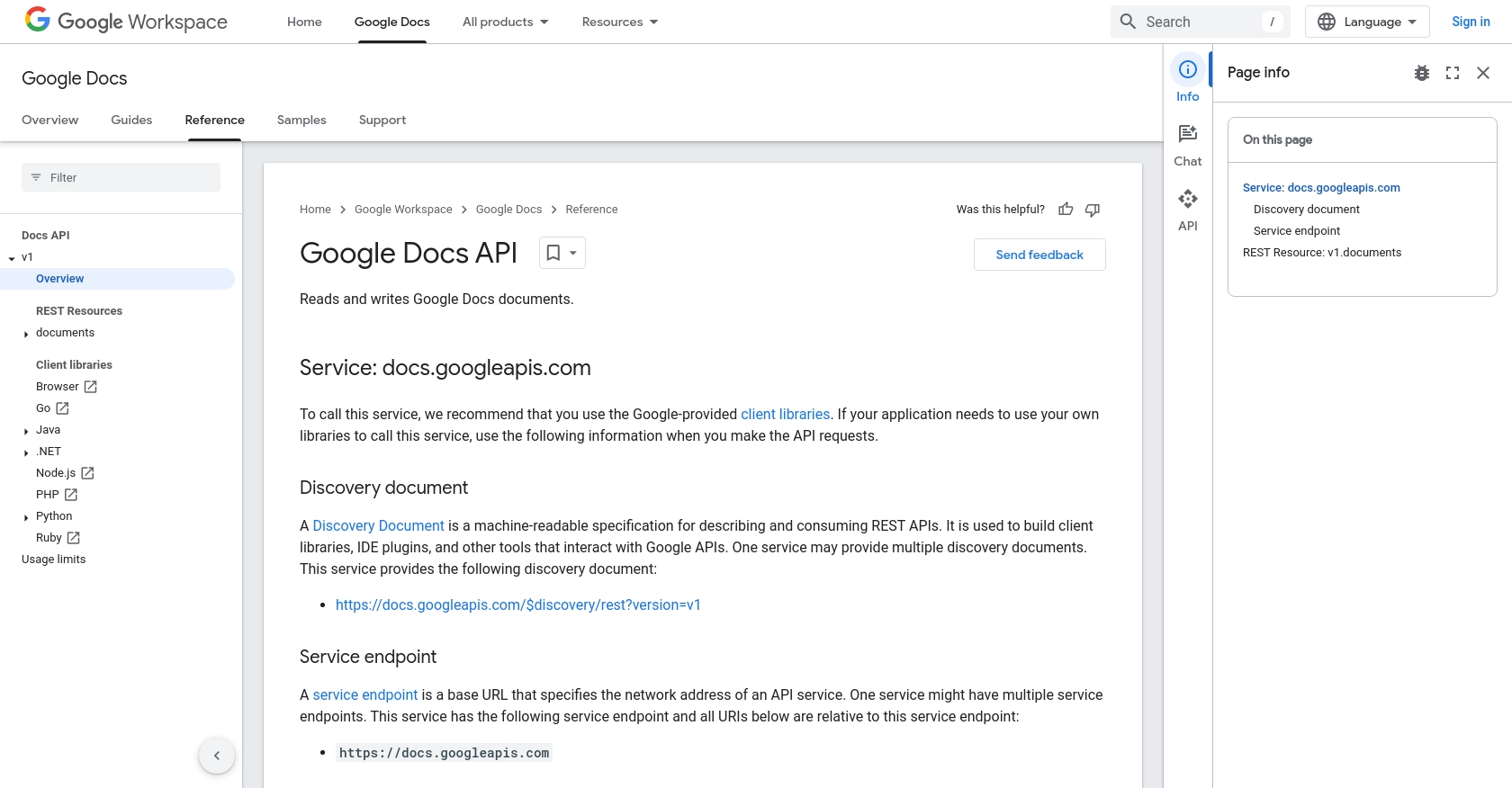
sbb-itb-96038d7
Making API Calls to Google Docs Using JavaScript
To interact with the Google Docs API using JavaScript, you'll need to set up your environment and write code to make API requests. This section will guide you through the process of creating and inserting text into a Google Doc programmatically.
Prerequisites for Google Docs API Integration with JavaScript
Before you begin, ensure you have the following:
- Node.js installed on your machine.
- A text editor or IDE for writing JavaScript code.
- The
googleapis
package, which you can install using npm:
npm install googleapis
Setting Up Google Docs API Client in JavaScript
First, you'll need to set up the Google Docs API client in your JavaScript application. This involves importing the necessary modules and configuring the client with your credentials.
const { google } = require('googleapis');
const docs = google.docs('v1');
async function authenticate() {
const auth = new google.auth.GoogleAuth({
keyFile: 'path/to/your/credentials.json',
scopes: ['https://www.googleapis.com/auth/documents'],
});
return await auth.getClient();
}
Creating a New Google Document
To create a new document, use the create
method provided by the Google Docs API. This method requires a title for the document.
async function createDocument(auth) {
const request = {
auth: auth,
resource: {
title: 'My New Document',
},
};
const response = await docs.documents.create(request);
console.log(`Document created with ID: ${response.data.documentId}`);
return response.data.documentId;
}
Inserting Text into a Google Document
Once you have created a document, you can insert text using the batchUpdate
method. This method allows you to specify the text and location for insertion.
async function insertText(auth, documentId) {
const requests = [
{
insertText: {
location: {
index: 1,
},
text: 'Hello, this is a sample text!',
},
},
];
const request = {
auth: auth,
documentId: documentId,
resource: {
requests: requests,
},
};
await docs.documents.batchUpdate(request);
console.log('Text inserted successfully.');
}
Executing the Google Docs API Integration
Finally, execute the functions to authenticate, create a document, and insert text. This sequence will demonstrate the complete process of interacting with the Google Docs API using JavaScript.
async function main() {
const auth = await authenticate();
const documentId = await createDocument(auth);
await insertText(auth, documentId);
}
main().catch(console.error);
By following these steps, you can automate document creation and text insertion in Google Docs using JavaScript. For more details, refer to the official Google Docs API documentation and API call guidelines.
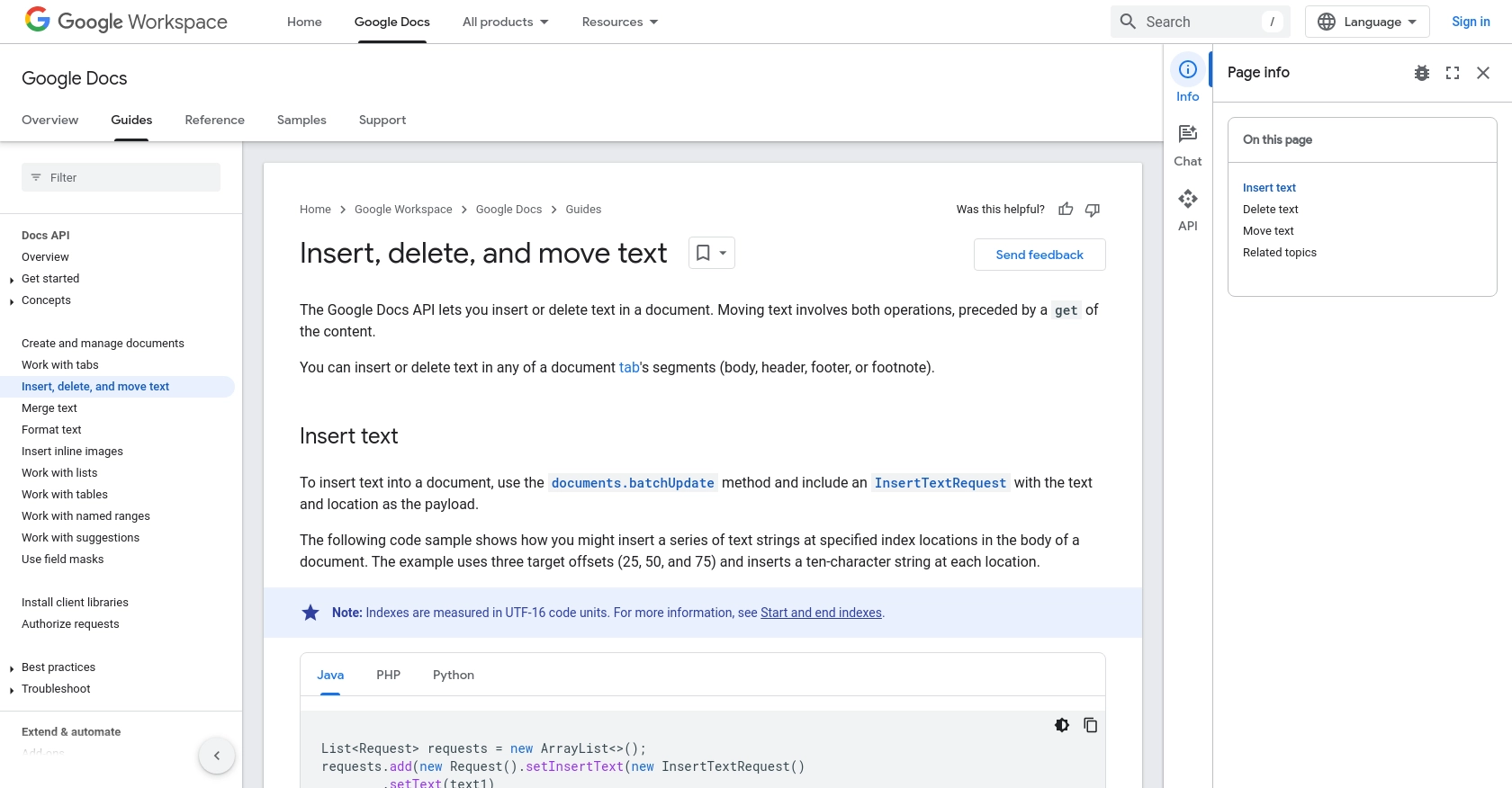
Conclusion and Best Practices for Google Docs API Integration with JavaScript
Integrating with the Google Docs API using JavaScript provides developers with powerful tools to automate document creation and management. By following the steps outlined in this guide, you can efficiently create and insert text into Google Docs, enhancing productivity and enabling custom workflows.
Best Practices for Secure and Efficient Google Docs API Usage
- Securely Store Credentials: Always store your OAuth 2.0 credentials securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be aware of Google API rate limits. Implement exponential backoff strategies to handle rate limit errors gracefully and ensure your application remains responsive.
- Optimize API Calls: Batch multiple requests into a single API call where possible to reduce the number of requests and improve performance.
- Monitor API Usage: Regularly monitor your API usage through the Google Cloud Console to identify any unusual patterns or potential issues.
Enhancing Integration Capabilities with Endgrate
While integrating with the Google Docs API directly is powerful, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Google Docs.
By using Endgrate, you can:
- Save time and resources by outsourcing integration management and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, streamlining your development process.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate and discover the benefits of a unified API approach.
Read More
- https://endgrate.com/provider/googledocs
- https://developers.google.com/docs/api/reference/rest
- https://developers.google.com/workspace/guides/create-project
- https://developers.google.com/workspace/guides/enable-apis
- https://developers.google.com/workspace/guides/configure-oauth-consent
- https://developers.google.com/workspace/guides/create-credentials
- https://developers.google.com/docs/api/how-tos/move-text
Ready to get started?