Using the Hubspot API to Create Deals (with Python examples)
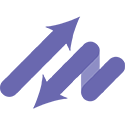
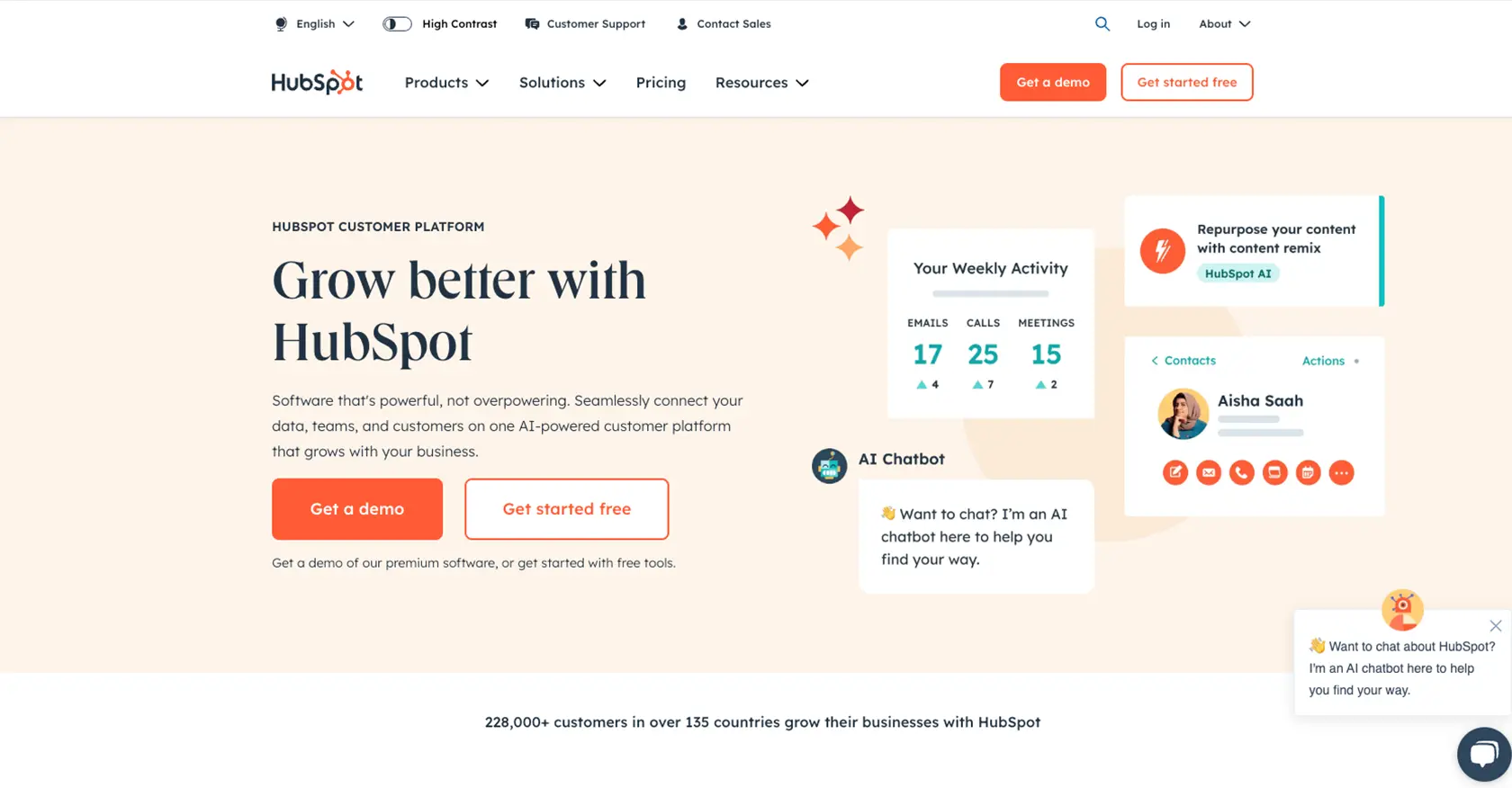
Introduction to HubSpot API for Deal Management
HubSpot is a comprehensive CRM platform that empowers businesses to manage their marketing, sales, and customer service efforts seamlessly. With its robust set of tools, HubSpot enables companies to enhance customer relationships and drive growth.
For developers, integrating with HubSpot's API offers the opportunity to automate and streamline various CRM processes. One such process is deal management, where developers can create, update, and manage deals programmatically. This integration is particularly useful for businesses looking to synchronize their sales data across multiple platforms or automate sales workflows.
For example, a developer might use the HubSpot API to automatically create deals when a new lead reaches a certain stage in the sales funnel. This ensures that the sales team can focus on nurturing leads rather than manually entering data, thereby increasing efficiency and accuracy.
Setting Up Your HubSpot Test or Sandbox Account for API Integration
Before diving into the HubSpot API to create deals, it's essential to set up a test or sandbox account. This environment allows developers to experiment with API calls without affecting live data, ensuring a safe and controlled testing space.
Creating a HubSpot Developer Account
To begin, you'll need a HubSpot developer account. If you don't have one, follow these steps:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill in the required information.
- Once registered, log in to your developer account to access the HubSpot developer tools.
Setting Up a HubSpot Sandbox Account
HubSpot provides sandbox accounts for testing purposes. Here's how to set one up:
- In your HubSpot developer account, navigate to the "Test Accounts" section.
- Click on "Create a test account" to generate a new sandbox environment.
- Follow the prompts to configure your sandbox account settings.
Configuring OAuth for HubSpot API Access
The HubSpot API uses OAuth for authentication, ensuring secure access to your data. Follow these steps to configure OAuth:
- In your developer account, go to the "Apps" section and click "Create an app."
- Fill in the app details, including name and description.
- Navigate to the "Auth" tab and select "OAuth" as the authentication method.
- Specify the necessary scopes for your app, such as
crm.objects.deals.write
for deal creation. - Save your app settings to generate a client ID and client secret.
Generating OAuth Tokens
With your app configured, you can now generate OAuth tokens to authenticate API requests:
- Direct users to the OAuth authorization URL to obtain an authorization code.
- Exchange the authorization code for an access token using the following Python code:
import requests
# Set the token endpoint and payload
token_url = "https://api.hubapi.com/oauth/v1/token"
payload = {
"grant_type": "authorization_code",
"client_id": "Your_Client_ID",
"client_secret": "Your_Client_Secret",
"redirect_uri": "Your_Redirect_URI",
"code": "Authorization_Code"
}
# Make a POST request to obtain the access token
response = requests.post(token_url, data=payload)
tokens = response.json()
# Print the access token
print(tokens['access_token'])
Replace Your_Client_ID
, Your_Client_Secret
, Your_Redirect_URI
, and Authorization_Code
with your actual values.
With your sandbox account and OAuth tokens ready, you're all set to start making API calls to create deals in HubSpot.
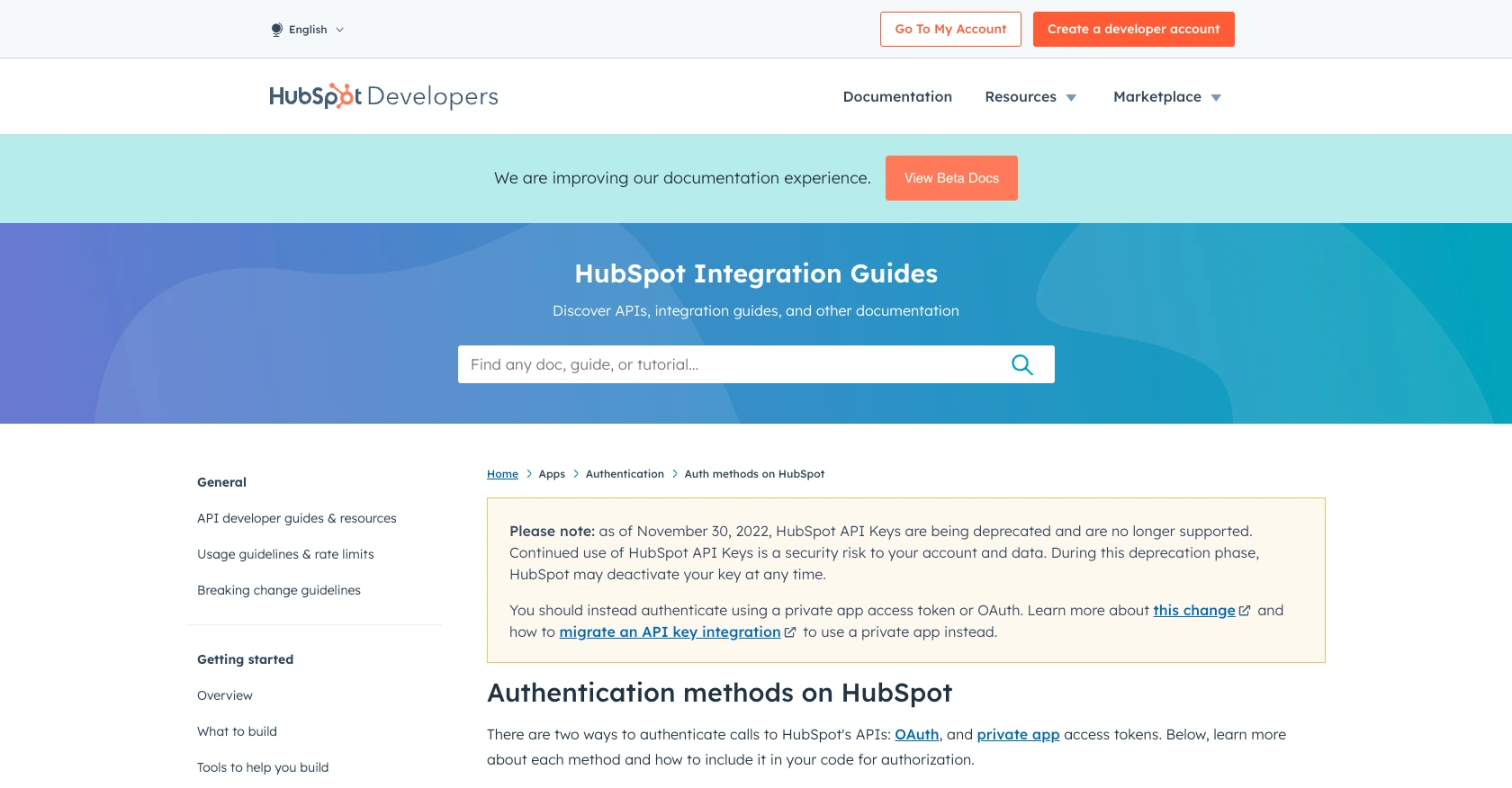
sbb-itb-96038d7
Making API Calls to Create Deals in HubSpot Using Python
With your HubSpot sandbox account and OAuth tokens ready, you can now proceed to create deals using the HubSpot API. This section will guide you through the necessary steps and provide Python code examples to help you interact with the API effectively.
Prerequisites for Using Python with HubSpot API
Before making API calls, ensure you have the following prerequisites:
- Python 3.11.1 installed on your machine.
- The Python package installer
pip
to manage dependencies.
Install the requests
library to handle HTTP requests by running the following command:
pip install requests
Creating a Deal in HubSpot Using Python
To create a deal in HubSpot, you'll need to make a POST request to the HubSpot API endpoint. Here's a step-by-step guide with example code:
import requests
# Set the API endpoint for creating deals
url = "https://api.hubapi.com/crm/v3/objects/deals"
# Set the request headers with the OAuth access token
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the deal properties
deal_data = {
"properties": {
"dealname": "New Deal",
"amount": "5000",
"pipeline": "default",
"dealstage": "contractsent",
"closedate": "2023-12-31T23:59:59Z"
}
}
# Make the POST request to create the deal
response = requests.post(url, json=deal_data, headers=headers)
# Check if the request was successful
if response.status_code == 201:
print("Deal created successfully:", response.json())
else:
print("Failed to create deal:", response.status_code, response.text)
Replace Your_Access_Token
with the OAuth token you obtained earlier. The deal_data
dictionary contains the properties of the deal you wish to create, such as dealname
, amount
, pipeline
, and dealstage
.
Verifying Deal Creation in HubSpot
After running the code, verify that the deal has been created by checking your HubSpot sandbox account. Navigate to the Deals section to see the newly created deal with the specified properties.
Handling Errors and Understanding Error Codes
When making API calls, it's crucial to handle potential errors. The HubSpot API may return various error codes, such as:
- 401 Unauthorized: Indicates an issue with authentication. Ensure your OAuth token is valid.
- 429 Too Many Requests: You've hit the rate limit. Implement rate limiting strategies to avoid this error.
- 400 Bad Request: Check the request payload for any missing or incorrect fields.
For more detailed error information, refer to the HubSpot Deals API documentation.
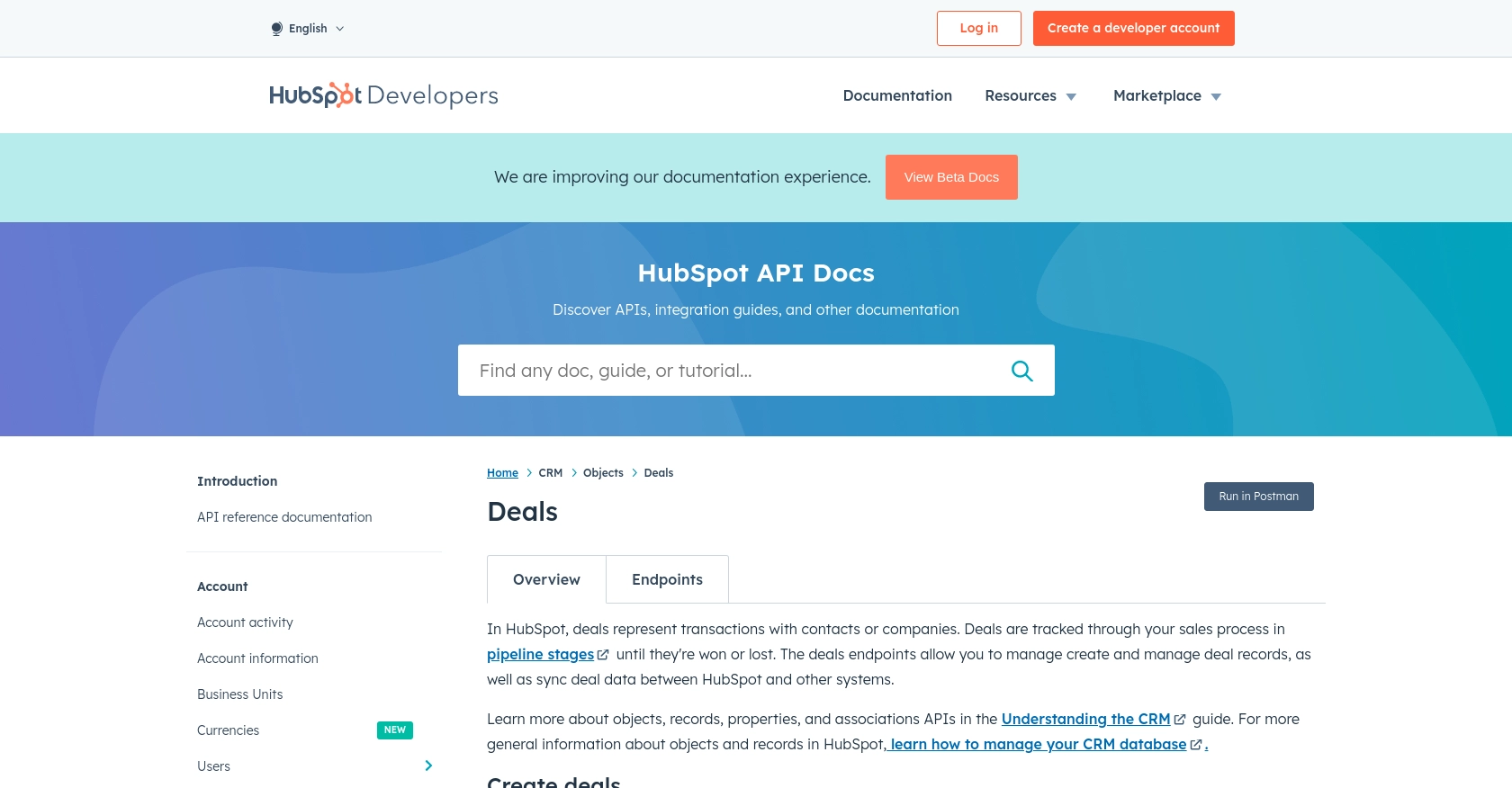
Conclusion and Best Practices for Using HubSpot API to Create Deals
Integrating with the HubSpot API to create deals can significantly enhance your sales processes by automating tasks and ensuring data consistency across platforms. By following the steps outlined in this guide, you can effectively manage deals within HubSpot using Python, streamlining your workflow and improving efficiency.
Best Practices for Secure and Efficient HubSpot API Integration
- Securely Store Credentials: Always store your OAuth tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Implement Rate Limiting: HubSpot's API has rate limits, such as 100 requests every 10 seconds for OAuth apps. Ensure your application respects these limits to avoid 429 errors. Consider implementing exponential backoff strategies for retrying requests.
- Data Standardization: Consistently format and standardize data fields to maintain data integrity across different systems and integrations.
- Error Handling: Implement robust error handling to manage API response codes effectively. Log errors for monitoring and debugging purposes.
Enhance Your Integration Strategy with Endgrate
While building custom integrations can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution for managing multiple integrations through a single API endpoint. By leveraging Endgrate, you can save time and resources, allowing your team to focus on core product development while ensuring a seamless integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website and discover how you can build once for each use case instead of multiple times for different integrations.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/deals
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?