Using the Intercom API to Create or Update Contacts (with Python examples)
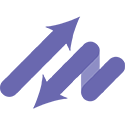
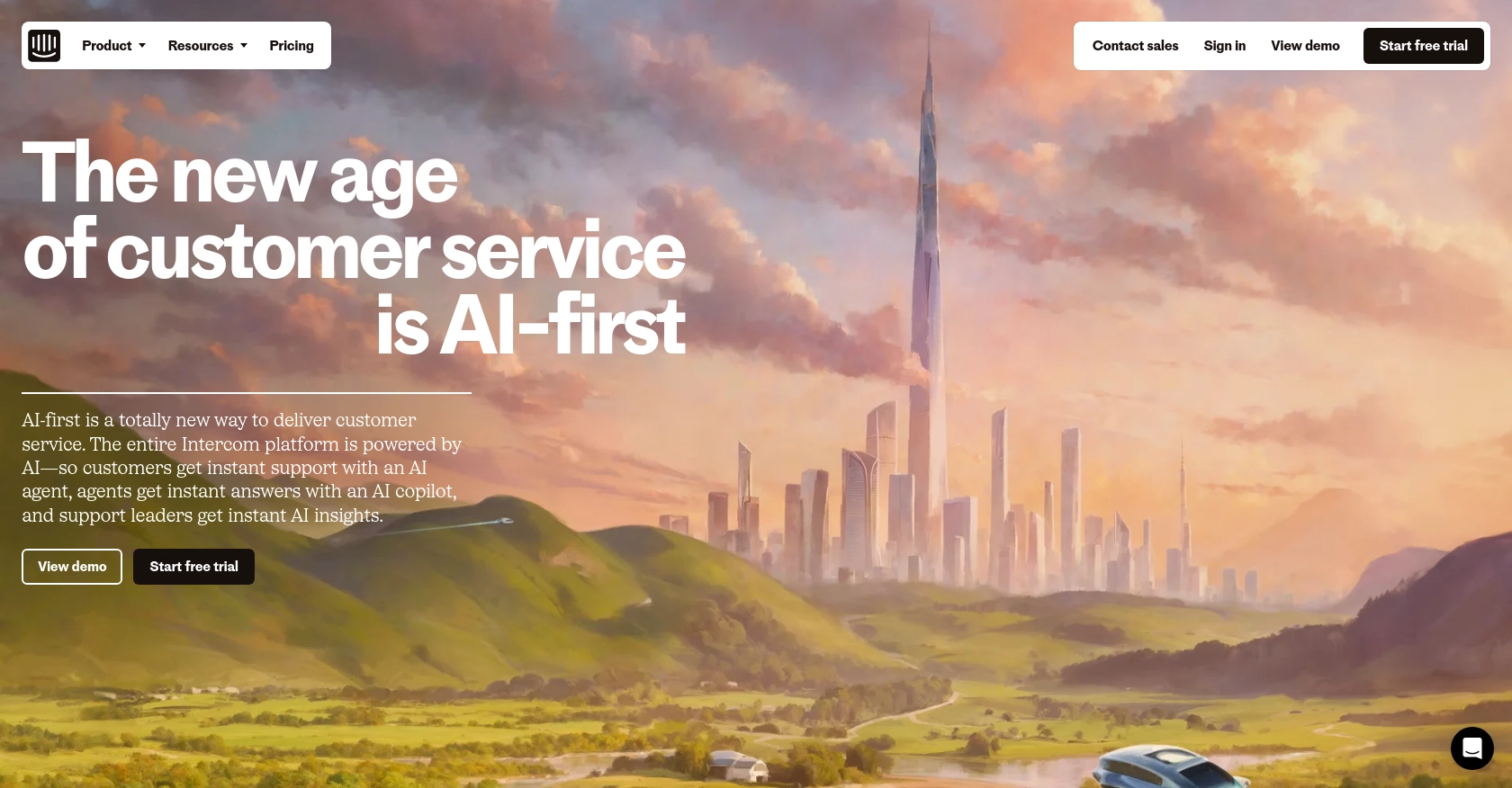
Introduction to Intercom API Integration
Intercom is a powerful customer communication platform that enables businesses to engage with their customers through messaging, email, and more. It offers a suite of tools designed to enhance customer support, marketing, and sales efforts, making it a popular choice for companies looking to improve their customer interactions.
Developers might want to integrate with Intercom to manage customer data effectively, such as creating or updating contact information. For example, a developer could use the Intercom API to automatically update customer profiles with the latest interaction data, ensuring that sales and support teams have access to the most current information.
This article will guide you through using Python to interact with the Intercom API, focusing on creating and updating contacts. By following the steps outlined, you can streamline your customer data management processes and enhance your application's functionality.
Setting Up Your Intercom Test or Sandbox Account
Before you can start integrating with the Intercom API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data. Intercom provides a development workspace that you can use for this purpose.
Creating an Intercom Developer Account
If you don't already have an Intercom account, you'll need to create one. Follow these steps to set up your developer account:
- Visit the Intercom Developer Hub and sign up for a free account.
- Once registered, log in to your account and navigate to the Developer Hub.
- Create a new development workspace by selecting the option to set up a workspace. This will give you access to the necessary tools and resources for building your integration.
Configuring OAuth for Intercom API Access
Intercom uses OAuth for authentication, which requires setting up an app within your development workspace. Follow these steps to configure OAuth:
- In the Developer Hub, go to the Authentication page and enable the "Use OAuth" option.
- Provide the necessary details, including redirect URLs, which must use HTTPS.
- Select the permissions your app requires, such as access to people and conversation data.
- Save your settings to generate your client ID and client secret.
For more detailed instructions, refer to the Intercom OAuth setup guide.
Generating Access Tokens
Once OAuth is configured, you can generate access tokens to authenticate API requests:
- Redirect users to the Intercom authorization URL to obtain an authorization code.
- Exchange the authorization code for an access token by making a POST request to the Intercom token endpoint.
- Store the access token securely for use in API calls.
With your test account and OAuth setup complete, you're ready to start making API calls to create or update contacts in Intercom.
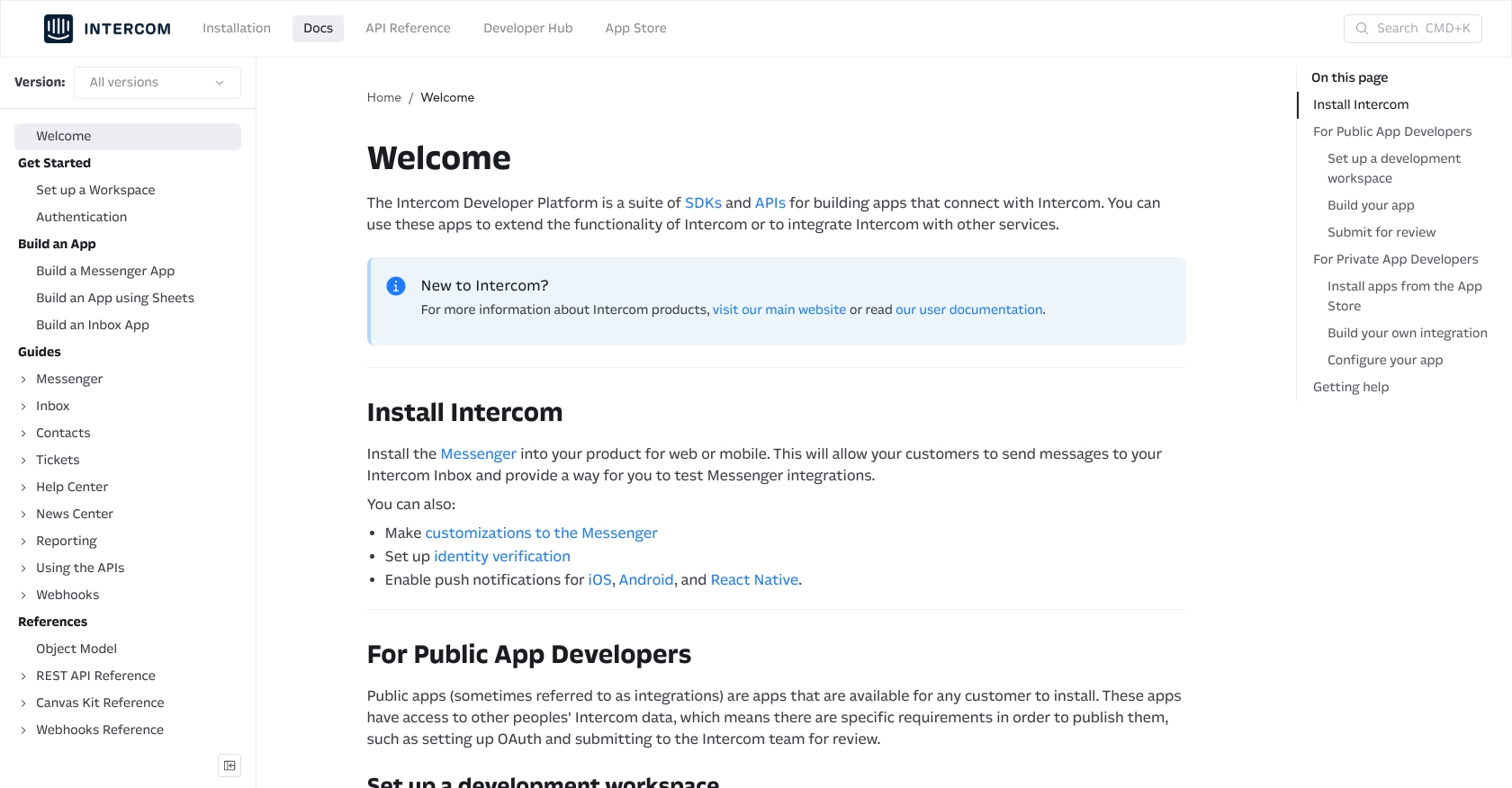
sbb-itb-96038d7
Making API Calls to Intercom for Creating or Updating Contacts Using Python
To interact with the Intercom API for creating or updating contacts, you'll need to use Python. This section will guide you through setting up your environment, writing the necessary code, and handling responses and errors effectively.
Setting Up Your Python Environment for Intercom API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Install the required requests
library by running the following command in your terminal:
pip install requests
Writing Python Code to Create a Contact in Intercom
To create a contact in Intercom, you'll need to send a POST request to the Intercom API. Here's an example of how to do this using Python:
import requests
# Define the API endpoint and headers
url = "https://api.intercom.io/contacts"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json",
"Intercom-Version": "2.11"
}
# Define the contact data
contact_data = {
"email": "example@intercom.io",
"name": "Example User"
}
# Make the POST request to create a contact
response = requests.post(url, json=contact_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Contact created successfully:", response.json())
else:
print("Failed to create contact:", response.status_code, response.text)
Replace Your_Access_Token
with the access token obtained during the OAuth setup. The code above sends a POST request to create a new contact with the specified email and name.
Updating an Existing Contact in Intercom Using Python
To update an existing contact, use the PUT method. Here's an example:
import requests
# Define the API endpoint and headers
contact_id = "contact_id_here"
url = f"https://api.intercom.io/contacts/{contact_id}"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json",
"Intercom-Version": "2.11"
}
# Define the updated contact data
updated_data = {
"name": "Updated User Name"
}
# Make the PUT request to update the contact
response = requests.put(url, json=updated_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Contact updated successfully:", response.json())
else:
print("Failed to update contact:", response.status_code, response.text)
Replace contact_id_here
with the ID of the contact you wish to update. This code updates the contact's name and checks for a successful response.
Handling API Responses and Errors for Intercom Integration
When making API calls, it's crucial to handle responses and errors properly. The Intercom API returns various status codes to indicate success or failure:
- 200 OK: The request was successful.
- 401 Unauthorized: Authentication failed. Check your access token.
- 404 Not Found: The specified contact ID does not exist.
- 400 Bad Request: The request was malformed. Check your data.
Always log the response status and message to diagnose issues effectively. For more details on error codes, refer to the Intercom API documentation.
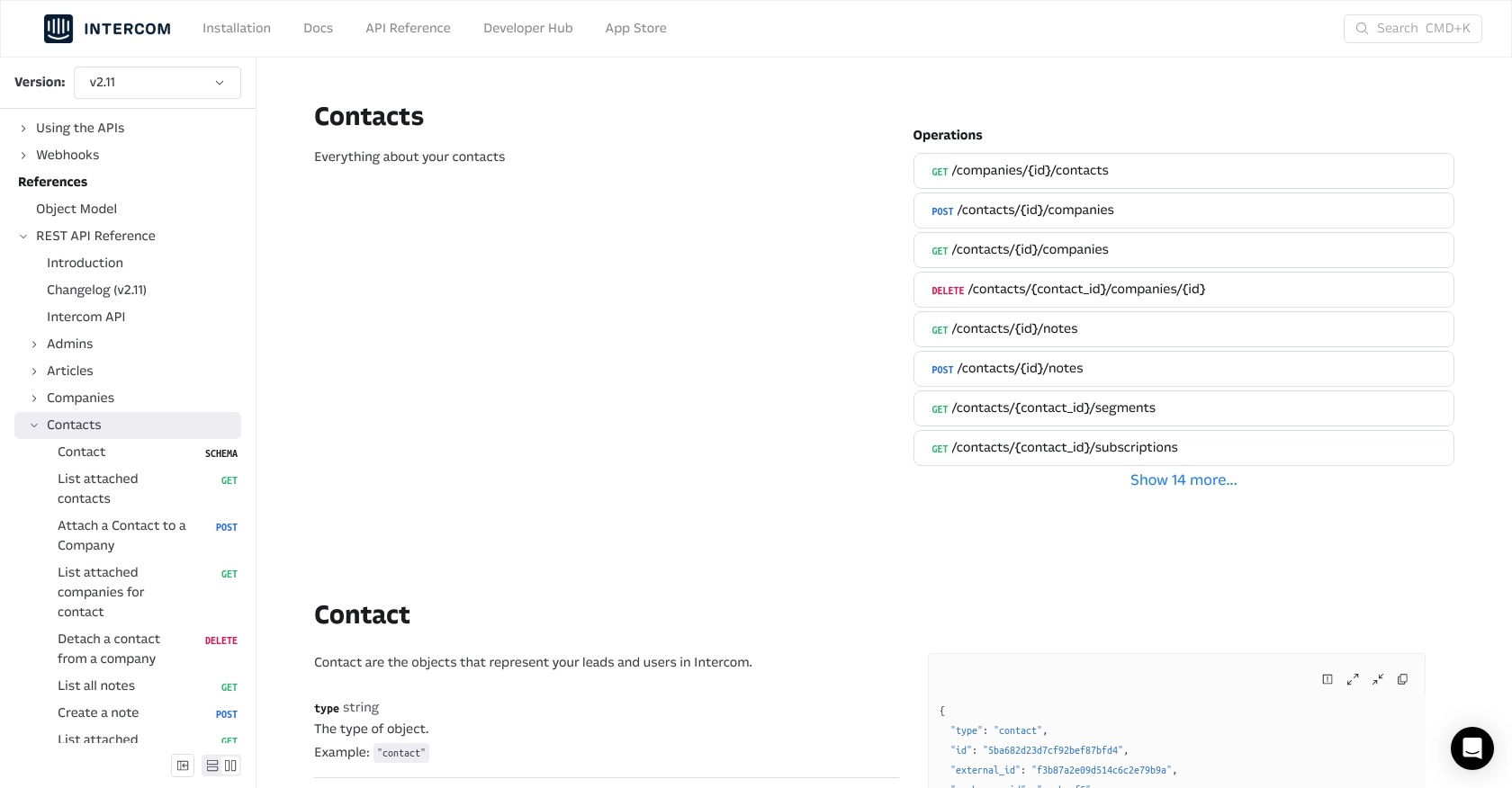
Conclusion and Best Practices for Intercom API Integration
Integrating with the Intercom API using Python allows developers to efficiently manage customer data by creating and updating contacts. This integration can significantly enhance your application's functionality by ensuring that customer information is always up-to-date and accessible to your sales and support teams.
Best Practices for Secure and Efficient Intercom API Usage
- Secure Storage of Access Tokens: Always store your access tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handling Rate Limits: Be mindful of Intercom's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Standardization: Ensure consistent data formats when creating or updating contacts to maintain data integrity across your systems.
- Error Handling: Implement robust error handling to manage different response codes effectively, ensuring your application can recover from API errors.
Streamlining Integrations with Endgrate
While integrating with Intercom can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. This is where Endgrate can be a valuable asset. By using Endgrate, you can:
- Save Time and Resources: Outsource your integration needs and focus on your core product development.
- Build Once, Use Everywhere: Create a single integration for each use case and apply it across multiple platforms.
- Enhance Customer Experience: Provide an intuitive and seamless integration experience for your users.
Explore how Endgrate can simplify your integration processes by visiting Endgrate's website and discover the benefits of a unified API solution.
Read More
Ready to get started?