Using the Intercom API to Get Users in Python
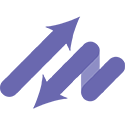
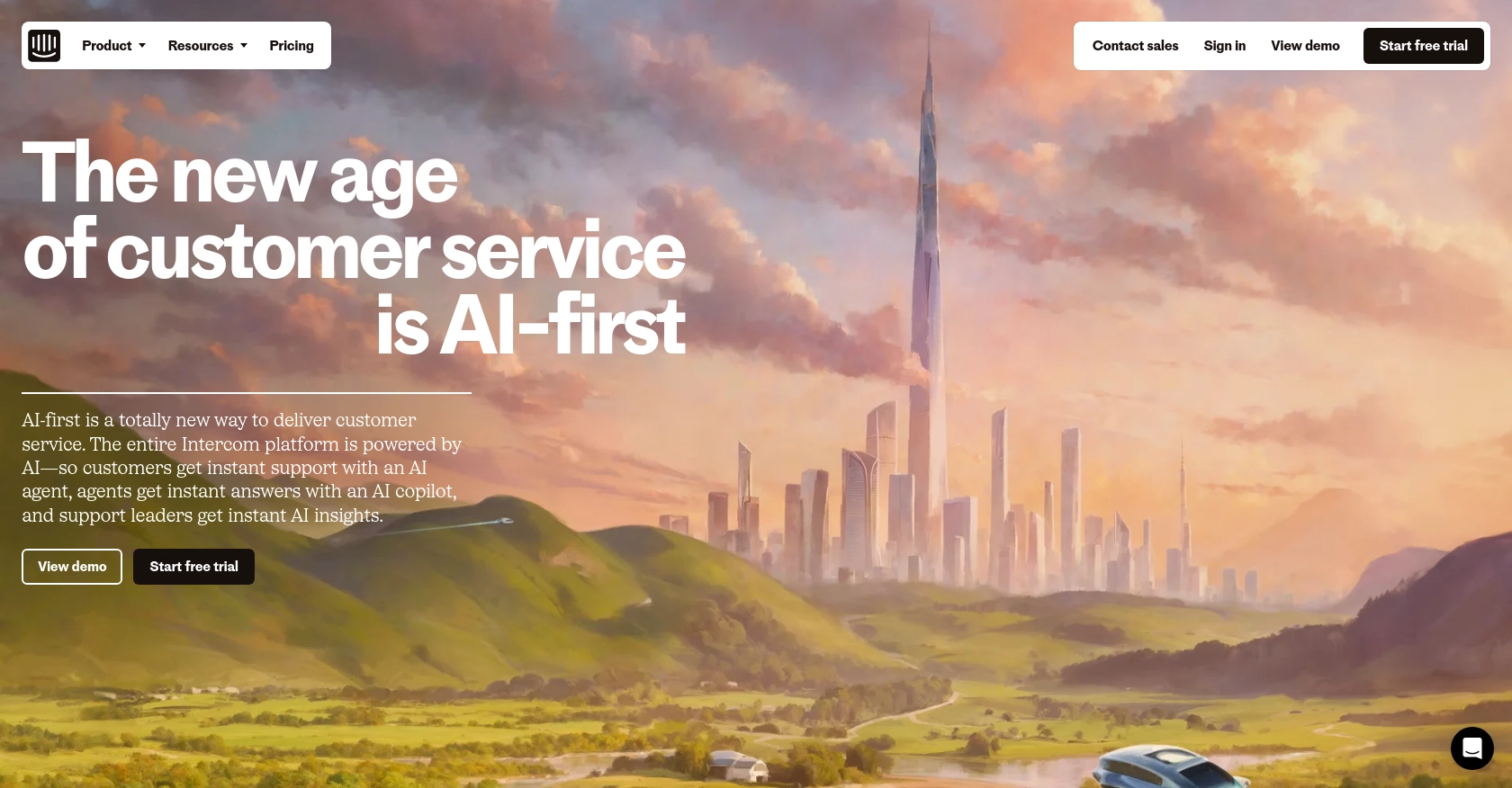
Introduction to Intercom API Integration
Intercom is a powerful customer communication platform that enables businesses to connect with their users through personalized messaging and support. It offers a suite of tools designed to enhance customer engagement, streamline support processes, and drive growth.
Developers may want to integrate with Intercom's API to access user data, automate interactions, and improve customer experience. For example, using the Intercom API, a developer can retrieve user information to personalize support responses or trigger automated messages based on user behavior.
This article will guide you through using Python to interact with the Intercom API, specifically focusing on retrieving user data. By following this tutorial, you'll learn how to efficiently access and manage user information within the Intercom platform using Python.
Setting Up Your Intercom Developer Account for API Integration
Before you can start interacting with the Intercom API, you'll need to set up a developer account. This involves creating a development workspace and configuring OAuth for authentication. Follow these steps to get started:
Create a Development Workspace on Intercom
To begin, you'll need to create a development workspace on Intercom. This workspace will allow you to build and test your integration:
- Visit the Intercom Developer Hub and sign up for a free account.
- Once registered, navigate to the Developer Hub and create a new app. This app will be associated with your development workspace.
Configure OAuth for Secure API Access
Intercom uses OAuth for secure access to its API. Follow these steps to set up OAuth:
- In your app's settings, enable the "Use OAuth" option.
- Provide the necessary redirect URLs. These URLs must use HTTPS and will be used to redirect users after they authorize your app.
- Select the required permissions for your app. Ensure you only request the scopes necessary for your use case.
- Save your client ID and client secret, which will be used to authenticate API requests.
For detailed instructions, refer to the Intercom OAuth setup guide.
Generate an Access Token for API Requests
After setting up OAuth, you'll need to generate an access token to authenticate your API requests:
- Direct users to the authorization URL:
https://app.intercom.com/oauth?client_id=YOUR_CLIENT_ID&state=YOUR_STATE
. - Once users authorize your app, they will be redirected to your specified redirect URL with an authorization code.
- Exchange this authorization code for an access token by making a POST request to
https://api.intercom.io/auth/eagle/token
with the required parameters.
Keep your access token secure, as it will be used to authenticate all API requests.
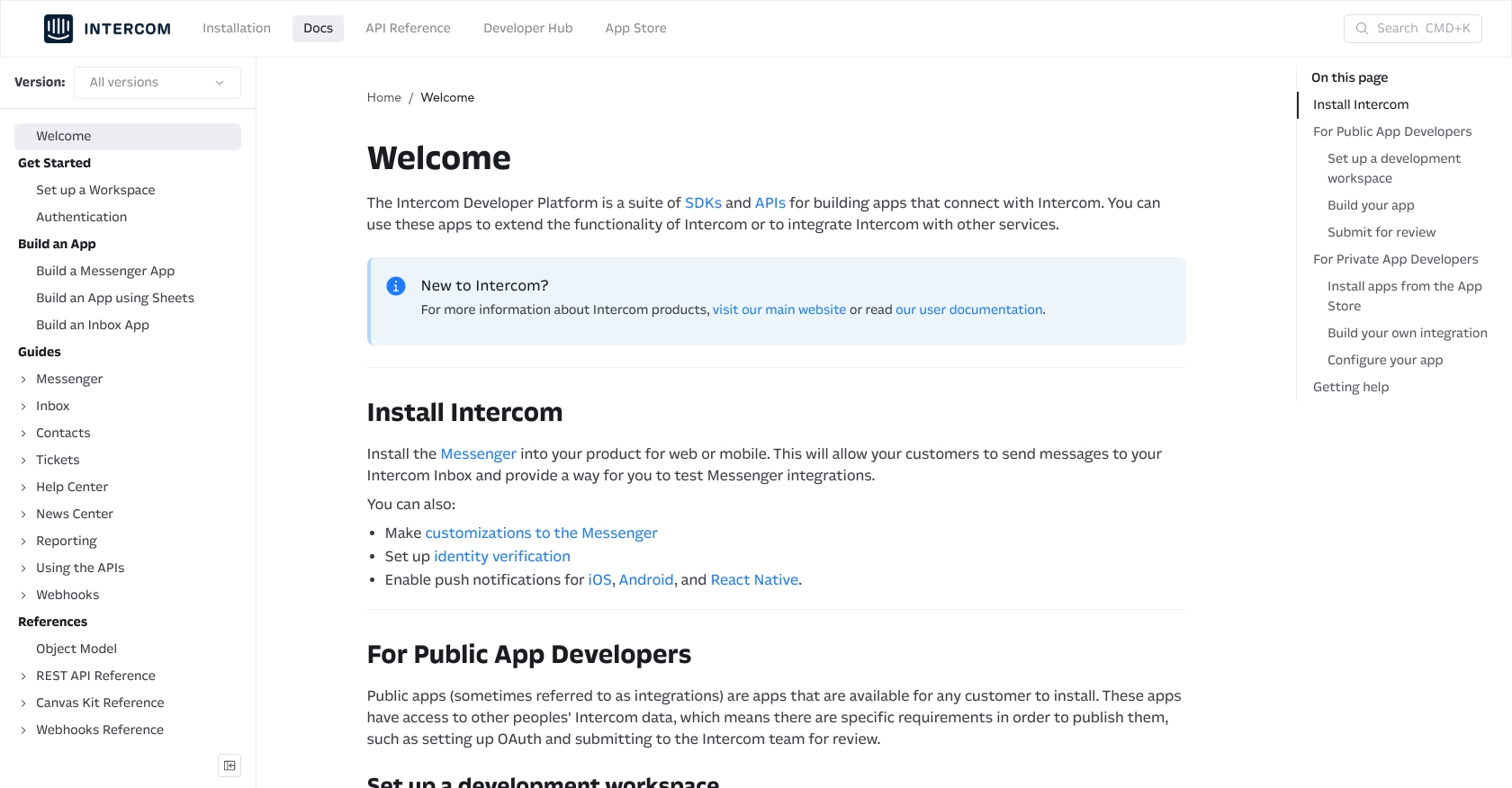
sbb-itb-96038d7
Making API Calls to Retrieve User Data from Intercom Using Python
To interact with the Intercom API and retrieve user data, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Intercom API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Writing Python Code to Retrieve Users from Intercom
Create a new Python file named get_intercom_users.py
and add the following code:
import requests
# Set the API endpoint and headers
url = "https://api.intercom.io/users"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Accept": "application/json",
"Intercom-Version": "2.11"
}
# Make a GET request to the API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
users = response.json()
for user in users.get("users", []):
print(f"User ID: {user['id']}, Email: {user['email']}")
else:
print(f"Failed to retrieve users: {response.status_code} - {response.text}")
Replace YOUR_ACCESS_TOKEN
with the access token you obtained during the OAuth setup. This script sends a GET request to the Intercom API to fetch user data and prints the user ID and email for each user.
Running the Python Script and Verifying Results
Execute the script from your terminal or command prompt using the following command:
python get_intercom_users.py
If successful, you should see a list of user IDs and emails printed to the console. If the request fails, the script will output an error message with the status code and response text.
Handling Errors and Troubleshooting Intercom API Requests
When working with the Intercom API, you may encounter errors. Here are some common error codes and their meanings:
- 401 Unauthorized: Check if your access token is correct and has the necessary permissions.
- 404 Not Found: Verify the API endpoint URL.
- 429 Too Many Requests: You have exceeded the rate limit. Wait before making more requests.
For more details on error handling, refer to the Intercom API documentation.
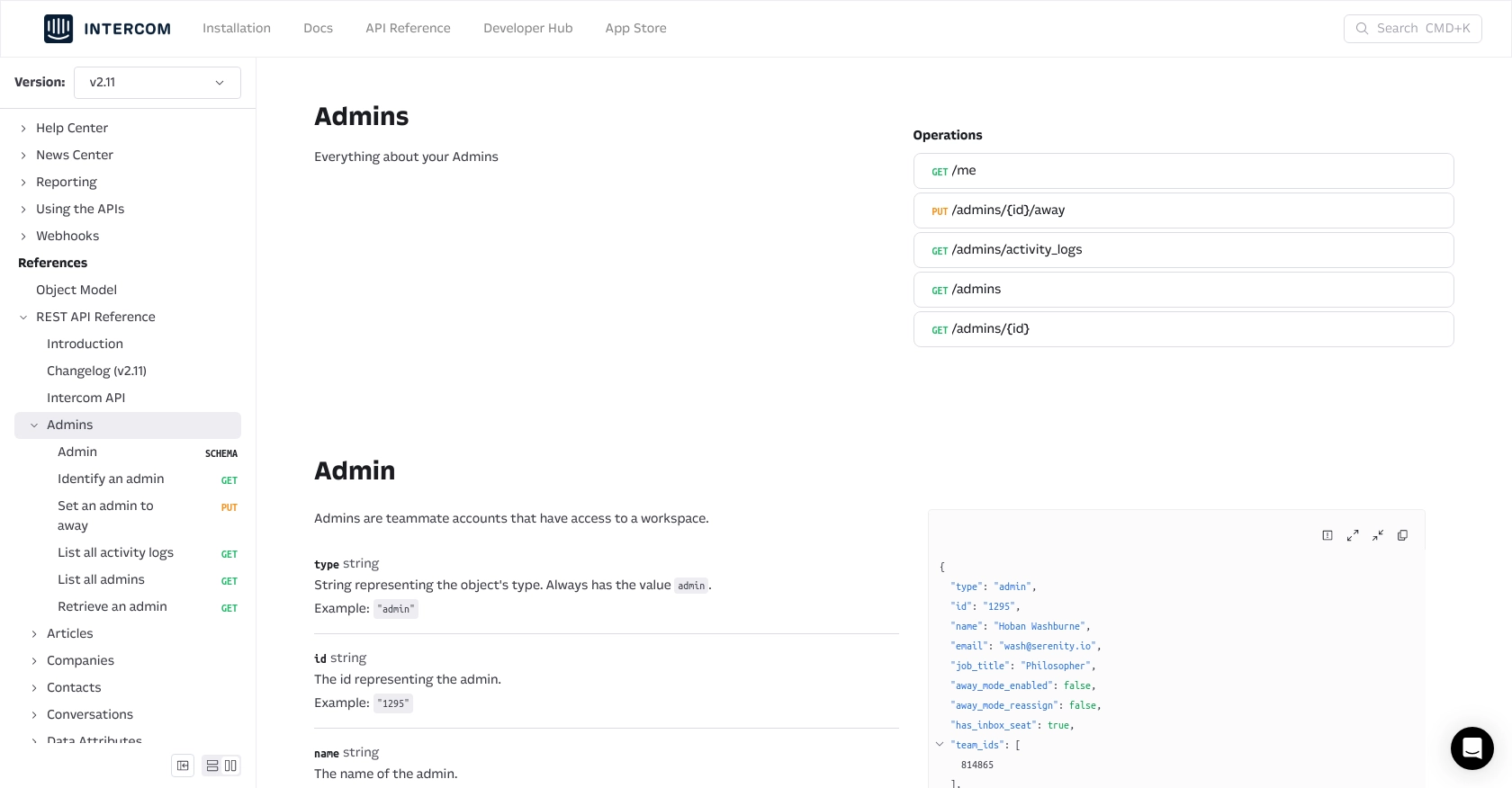
Best Practices for Using Intercom API in Python
When integrating with the Intercom API, following best practices ensures a secure and efficient implementation. Here are some key recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Intercom's API may impose rate limits. If you receive a
429 Too Many Requests
error, implement a retry mechanism with exponential backoff to handle rate limiting gracefully. - Optimize Data Handling: When retrieving user data, consider filtering and paginating results to manage large datasets efficiently. This reduces the load on both your application and the Intercom API.
- Monitor API Usage: Regularly monitor your API usage and error logs to identify any issues early. This helps in maintaining a smooth integration experience.
Streamlining Intercom Integrations with Endgrate
Building and maintaining integrations with platforms like Intercom can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint for multiple integrations, including Intercom.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the integration complexities.
- Build Once, Use Everywhere: Develop a single integration that works across various platforms, reducing redundant efforts.
- Enhance Customer Experience: Offer a seamless and intuitive integration experience to your users.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Read More
Ready to get started?