Using the Keap API to Create or Update Companies in Javascript
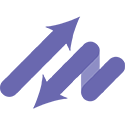
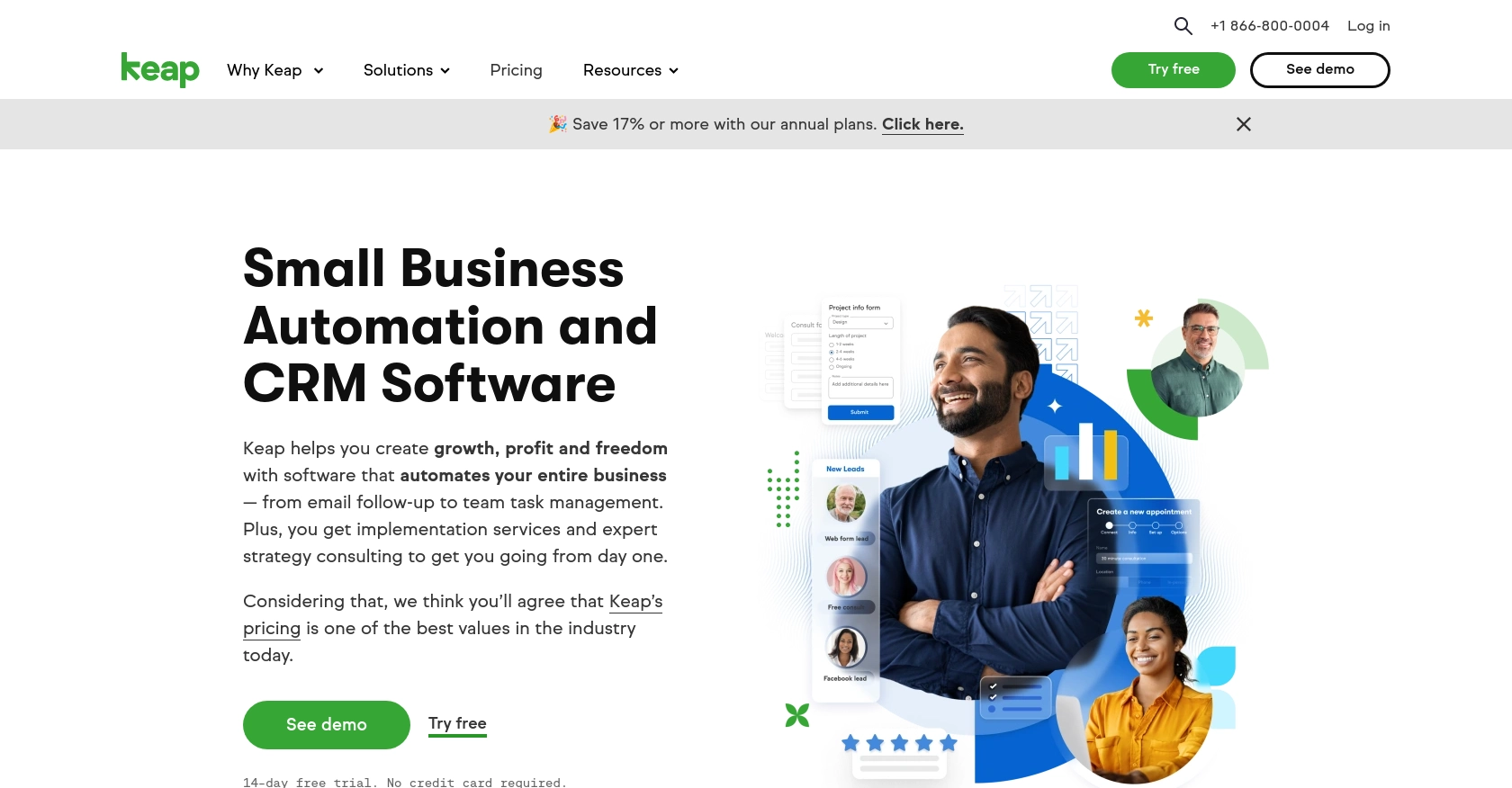
Introduction to Keap API Integration
Keap, formerly known as Infusionsoft, is a powerful CRM and marketing automation platform designed to help small businesses streamline their sales and marketing efforts. With features like contact management, email marketing, and sales automation, Keap provides a comprehensive solution for managing customer relationships.
Developers may want to integrate with Keap's API to automate and enhance business processes. For example, using the Keap API, developers can create or update company records directly from their applications, ensuring that customer data is always up-to-date and synchronized across platforms.
This article will guide you through using JavaScript to interact with the Keap API, focusing on creating or updating company records. By following this tutorial, you'll learn how to efficiently manage company data within Keap, enhancing your application's functionality and user experience.
Setting Up Your Keap Developer Account and Sandbox Environment
Before you can start integrating with the Keap API, you'll need to set up a developer account and create a sandbox environment. This will allow you to test your API interactions without affecting live data. Follow these steps to get started:
Register for a Keap Developer Account
To begin, you'll need to register for a Keap developer account. This account will give you access to the necessary tools and resources to work with the Keap API.
- Visit the Keap Developer Portal.
- Click on the "Register" button and fill out the required information to create your developer account.
- Once registered, you'll have access to the developer dashboard where you can manage your applications and sandbox environments.
Create a Sandbox App for Testing
With your developer account set up, the next step is to create a sandbox app. This will allow you to test API calls in a safe environment.
- Log in to your Keap developer account.
- Navigate to the "Sandbox" section in the dashboard.
- Click on "Create Sandbox App" and provide the necessary details for your application.
- Once created, you'll receive a client ID and client secret, which are essential for authenticating your API requests.
Setting Up OAuth Authentication
The Keap API uses OAuth 2.0 for authentication. You'll need to create an app to obtain the client ID and client secret required for this process.
- In your sandbox app, navigate to the "OAuth" settings.
- Configure the redirect URI to match your application's callback URL.
- Save the settings and note down the client ID and client secret.
These credentials will be used to authorize API requests and access the Keap resources securely.
With your developer account and sandbox app set up, you're now ready to start making API calls to create or update company records in Keap using JavaScript. In the next section, we'll dive into the code and demonstrate how to perform these operations.
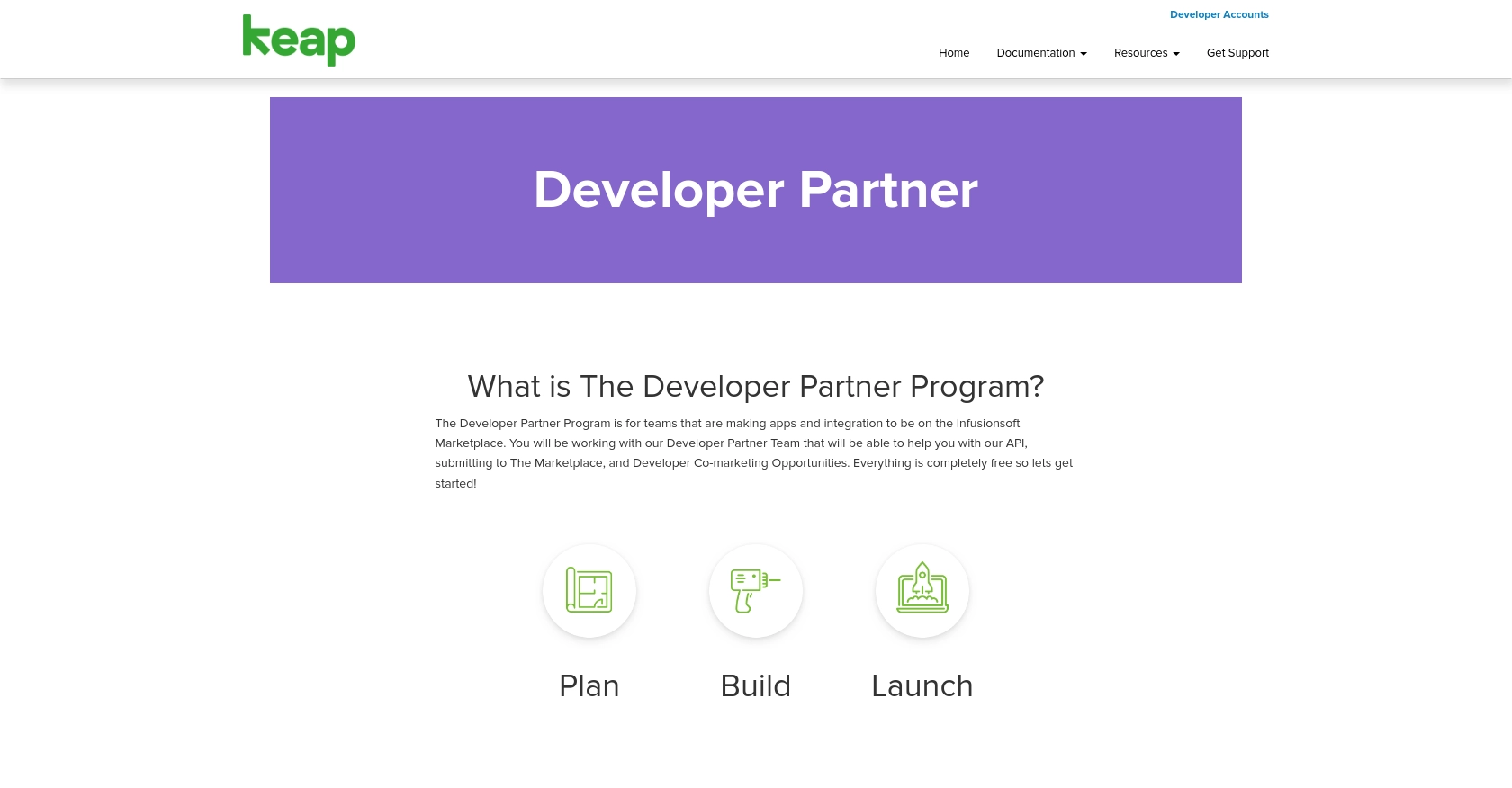
sbb-itb-96038d7
Making API Calls to Keap for Creating or Updating Companies Using JavaScript
Now that you have your Keap developer account and sandbox environment set up, it's time to dive into the code. In this section, we'll guide you through the process of making API calls to create or update company records in Keap using JavaScript. This involves setting up the necessary dependencies, writing the code to interact with the Keap API, and handling potential errors.
Setting Up Your JavaScript Environment for Keap API Integration
Before making API calls, ensure you have Node.js installed on your machine, as it provides the runtime environment for executing JavaScript code outside the browser. Additionally, you'll need to install the Axios library, which simplifies making HTTP requests.
- Download and install Node.js if you haven't already.
- Open your terminal and run the following command to install Axios:
npm install axios
Writing JavaScript Code to Create or Update Companies in Keap
With your environment ready, you can now write the code to interact with the Keap API. The following example demonstrates how to create or update a company record using Axios for HTTP requests.
const axios = require('axios');
// Replace with your actual client ID, client secret, and access token
const clientId = 'YOUR_CLIENT_ID';
const clientSecret = 'YOUR_CLIENT_SECRET';
const accessToken = 'YOUR_ACCESS_TOKEN';
// Function to create or update a company
async function createOrUpdateCompany(companyData) {
try {
const response = await axios({
method: 'post', // Use 'put' for updating
url: 'https://api.infusionsoft.com/crm/rest/v1/companies',
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
},
data: companyData
});
console.log('Company created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating company:', error.response ? error.response.data : error.message);
}
}
// Example company data
const companyData = {
company_name: 'Example Company',
email: 'contact@example.com',
phone: '123-456-7890'
};
// Call the function
createOrUpdateCompany(companyData);
In this code, replace YOUR_CLIENT_ID
, YOUR_CLIENT_SECRET
, and YOUR_ACCESS_TOKEN
with your actual credentials. The function createOrUpdateCompany
sends a POST request to the Keap API to create a new company. If you need to update an existing company, change the method to PUT and provide the company ID in the URL.
Verifying API Request Success and Handling Errors
After executing the code, you should verify that the company record was successfully created or updated in your Keap sandbox environment. Log in to your Keap account and check the company records to confirm the changes.
Handling errors is crucial for robust API integration. The example code includes error handling to log any issues that occur during the API call. Common error codes include:
- 400 Bad Request: The request was invalid. Check the request data and parameters.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 404 Not Found: The specified resource could not be found.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more detailed error information, refer to the Keap API documentation.
Best Practices for Keap API Integration and Error Handling
Successfully integrating with the Keap API requires attention to best practices, particularly in handling authentication, rate limits, and data management. Here are some key considerations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of Keap's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully. For specific rate limit details, refer to the Keap API documentation.
- Standardize Data Fields: Ensure that data fields are consistent and standardized across your application and Keap. This helps maintain data integrity and simplifies data processing.
Enhancing Your Application with Keap API and Endgrate
Integrating with the Keap API can significantly enhance your application's capabilities by automating CRM processes and ensuring data consistency. However, managing multiple integrations can be complex and time-consuming.
Endgrate offers a streamlined solution by providing a unified API endpoint for various platforms, including Keap. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration management.
- Focus on your core product development instead of handling multiple integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate and discover the benefits of a unified API approach.
Read More
Ready to get started?